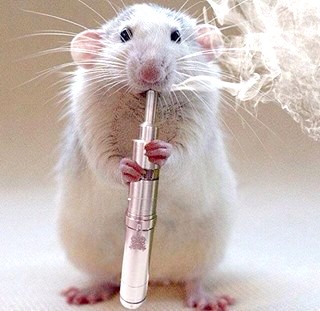
Topics
Replies
cyfer
14 Jul 2016, 10:23
I usually do this through a Bool Swtich
Lets say your signal is a Long Trade and before it's opened the Bot is waiting for the Signal
In this case The Bool Switch (Lets Call it CanOpenLongTrade) will be true
To initialize
//initialize private bool CanOpenLongTrade ; //OnStart CanOpenLongTrade = True
Now Your cBot Got the Signal , Let's say on OnTick Method , You test for the Validity of opening a Long Trade
if(Condition1 && Condition2 && CanOpenLongTrade) { // Here you Execute the opening of the long trade }
and at the same OnTick or OnBar method you Swich it Off
if(Condition1 && Condition2 && CanOpenLongTrade) { // Here you Execute the opening of the long trade CanOpenLongTrade = false ; }
Now it will not take the long signals again
Still you must turn it back on , maybe after the Trade is closed , According to specific time or what ever you want
The point is you :
-Start with the bool switch True
-Check for being true before a position is opened
-Turn it off immediately after you open the trade
-Turn it back on again to allow the cBot to open another position
@cyfer
cyfer
29 Jun 2016, 02:53
Hello Marc
This Uses Your Windows Time
DateTime.Now.Hour
And Server.Time Sure uses the Server Time
There was something about the difference between Time.Hour & Server.Time.Hour that Spotware mentioned in a Thread but I Can't remember what it was .
Is your bot using other TimeZone than UTC ? If no , I think Time.Hour & Server.Time.Hour will be the same . but i'm not sure
best way is to Log them(Print their Values) to see the difference between them .
@cyfer
cyfer
12 Jun 2016, 23:10
( Updated at: 21 Dec 2023, 09:20 )
Hello
First you should set your EMAs at Start of the Bot , not on each Bar
I'm trying to reproduce your code here in a way I can understand
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class SampleTrendcBot : Robot { [Parameter()] public DataSeries SourceSeries { get; set; } [Parameter("Slow Periods", DefaultValue = 21)] public int SlowPeriods { get; set; } [Parameter("Fast Periods", DefaultValue = 8)] public int FastPeriods { get; set; } [Parameter("Closing Periods", DefaultValue = 3)] public int ClosePeriods { get; set; } [Parameter("Quantity (Lots)", DefaultValue = 1, MinValue = 0.01, Step = 0.01)] public double Quantity { get; set; } private ExponentialMovingAverage slowMa; private ExponentialMovingAverage fastMa; private ExponentialMovingAverage closeMa; private const string label = "Sample Trend cBot"; private Position longPosition; private Position shortPosition; protected override void OnStart() { fastMa = Indicators.ExponentialMovingAverage(SourceSeries, 8); slowMa = Indicators.ExponentialMovingAverage(SourceSeries, 21); closeMa = Indicators.ExponentialMovingAverage(SourceSeries, 3); } protected override void OnBar() { longPosition = Positions.Find(label, Symbol, TradeType.Buy); shortPosition = Positions.Find(label, Symbol, TradeType.Sell); var currentCloseMa = closeMa.Result.Last(0); // var currentSlowMa = slowMa.Result.Last(1); // var currentFastMa = fastMa.Result.Last(1); // var previousSlowMa = slowMa.Result.Last(2); // var previousFastMa = fastMa.Result.Last(2); var currentcandleclose = MarketSeries.Close.Last(0); if (fastMa.Result.Last(1) < slowMa.Result.Last(1) && fastMa.Result.Last(0) > slowMa.Result.Last(0) && longPosition == null) { ExecuteMarketOrder(TradeType.Buy, Symbol, VolumeInUnits, label); } else if (fastMa.Result.Last(1) > slowMa.Result.Last(1) && fastMa.Result.Last(0) < slowMa.Result.Last(0) && shortPosition == null) { ExecuteMarketOrder(TradeType.Sell, Symbol, VolumeInUnits, label); } if (longPosition != null & currentcandleclose < currentCloseMa) ClosePosition(longPosition); if (shortPosition != null & currentcandleclose > currentCloseMa) ClosePosition(shortPosition); } private long VolumeInUnits { get { return Symbol.QuantityToVolume(Quantity); } } } }
Now , assuming you want to open a Sell/Buy order not after the Cross of EMAs but after the Cross and on the start of the new candle
I Think you should then a Bool Switch
For example : When 2 EMAs cross , Bool value is true (OpenBuyTrade = True) and When this value is tested on the next Bar , it will open the trade
same for Sell order
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class SampleTrendcBot : Robot { private bool OpenBuyTrade; private bool OpenSellTrade; [Parameter()] public DataSeries SourceSeries { get; set; } [Parameter("Slow Periods", DefaultValue = 21)] public int SlowPeriods { get; set; } [Parameter("Fast Periods", DefaultValue = 8)] public int FastPeriods { get; set; } [Parameter("Closing Periods", DefaultValue = 3)] public int ClosePeriods { get; set; } [Parameter("Quantity (Lots)", DefaultValue = 1, MinValue = 0.01, Step = 0.01)] public double Quantity { get; set; } private ExponentialMovingAverage slowMa; private ExponentialMovingAverage fastMa; private ExponentialMovingAverage closeMa; private const string label = "Sample Trend cBot"; private Position longPosition; private Position shortPosition; protected override void OnStart() { fastMa = Indicators.ExponentialMovingAverage(SourceSeries, 8); slowMa = Indicators.ExponentialMovingAverage(SourceSeries, 21); closeMa = Indicators.ExponentialMovingAverage(SourceSeries, 3); OpenBuyTrade = false; OpenSellTrade = false; } protected override void OnBar() { longPosition = Positions.Find(label, Symbol, TradeType.Buy); shortPosition = Positions.Find(label, Symbol, TradeType.Sell); var currentCloseMa = closeMa.Result.Last(0); // var currentSlowMa = slowMa.Result.Last(1); // var currentFastMa = fastMa.Result.Last(1); // var previousSlowMa = slowMa.Result.Last(2); // var previousFastMa = fastMa.Result.Last(2); var currentcandleclose = MarketSeries.Close.Last(0); if (OpenBuyTrade) { ExecuteMarketOrder(TradeType.Buy, Symbol, VolumeInUnits, label); OpenBuyTrade = false; } if (OpenSellTrade) { ExecuteMarketOrder(TradeType.Sell, Symbol, VolumeInUnits, label); OpenSellTrade = false; } if (fastMa.Result.Last(1) < slowMa.Result.Last(1) && fastMa.Result.Last(0) > slowMa.Result.Last(0) && longPosition == null) { OpenBuyTrade = true; } else if (fastMa.Result.Last(1) > slowMa.Result.Last(1) && fastMa.Result.Last(0) < slowMa.Result.Last(0) && shortPosition == null) { OpenSellTrade = true; } if (longPosition != null & currentcandleclose < currentCloseMa) ClosePosition(longPosition); if (shortPosition != null & currentcandleclose > currentCloseMa) ClosePosition(shortPosition); } private long VolumeInUnits { get { return Symbol.QuantityToVolume(Quantity); } } } }
I wish that answers your question
@cyfer
cyfer
31 May 2016, 16:03
You can go for something like this
protected override void OnBar() { if (Time.Hour == 21 && Time.Minute >= 55) { Print("Execution Time"); } else { Print("We wait for the proper time"); } }
You can also test Server.Time.Hour instead of Time.Hour and see what suites you
If your strategy is based on a specific time of the day , I strongly advise against using OnTick() , its an overhead for nothing
@cyfer
cyfer
22 May 2016, 01:35
Swing ---> Ichimoku + ADX (DMS)
Scalp---> The Squeeze , Matrix
But my Scalping Beast is .. Eclipse & Eclipse Scanner .. "proprietary Indies"
Eventually .. Indicators are great aid if you use them in the right market condition , but I don't think they can make any one a successful trader
@cyfer
cyfer
10 May 2016, 23:54
RE:
cyfer said:
Basically , It will be something Like this
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class Cyf_ROB_BollyBand_Bounce : Robot { private BollingerBands bb; private Position position; private bool CanOpenTrade; [Parameter(DefaultValue = 20)] public int bb_Periods { get; set; } [Parameter(DefaultValue = 2.0)] public double bb_stdDev { get; set; } [Parameter("Source")] public DataSeries bb_Source { get; set; } [Parameter("Volume", DefaultValue = 10000, MinValue = 1000, Step = 1000)] public int Volume { get; set; } [Parameter("Stop at", DefaultValue = 20)] public int StopLoss { get; set; } protected override void OnStart() { CanOpenTrade = true; bb_Source = CreateDataSeries(); bb = Indicators.BollingerBands(MarketSeries.Close, bb_Periods, bb_stdDev, MovingAverageType.Simple); } protected override void OnBar() { if (MarketSeries.Close.Last(0) < bb.Top.Last(0) && MarketSeries.Close.Last(1) > bb.Top.Last(1) && CanOpenTrade) { var result = ExecuteMarketOrder(TradeType.Sell, Symbol, Volume, "BollyBand_Sell", StopLoss, null); if (result.IsSuccessful) { position = result.Position; CanOpenTrade = false; } } /////////////////// if (MarketSeries.Close.Last(0) > bb.Bottom.Last(0) && MarketSeries.Close.Last(1) < bb.Bottom.Last(1) && CanOpenTrade) { var result = ExecuteMarketOrder(TradeType.Buy, Symbol, Volume, "BollyBand_Buy", StopLoss, null); if (result.IsSuccessful) { position = result.Position; CanOpenTrade = false; } } } protected override void OnTick() { // Put your core logic here if (Positions.Count != 0 && position.TradeType == TradeType.Sell) { if (MarketSeries.Close.Last(0) >= bb.Main.Last(0))// Main or Bottom ???? ClosePosition(position); CanOpenTrade = true; } if (Positions.Count != 0 && position.TradeType == TradeType.Buy) { if (MarketSeries.Close.Last(0) <= bb.Main.Last(0)) // Main or TOP ????? ClosePosition(position); CanOpenTrade = true; } } protected override void OnStop() { // Put your deinitialization logic here } } }But This is so simplified and there is no Real TP or SL Targets here .
usually the best SL & TP is related to ATR of that TF .
I Don't think it's a winning strategy , Bollinger bands are based on volatility .. So if Price Goes UP till it hits the upper band and then reverses to the lower band .. and then
exceeds the lower band and then reverses and Goes Up again and hit the upper band again .. and keep doing this imaginary cycle .. This could be a winning strategy
obviously this doesn't happen
@cyfer
cyfer
10 May 2016, 23:53
Basically , It will be something Like this
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class Cyf_ROB_BollyBand_Bounce : Robot { private BollingerBands bb; private Position position; private bool CanOpenTrade; [Parameter(DefaultValue = 20)] public int bb_Periods { get; set; } [Parameter(DefaultValue = 2.0)] public double bb_stdDev { get; set; } [Parameter("Source")] public DataSeries bb_Source { get; set; } [Parameter("Volume", DefaultValue = 10000, MinValue = 1000, Step = 1000)] public int Volume { get; set; } [Parameter("Stop at", DefaultValue = 20)] public int StopLoss { get; set; } protected override void OnStart() { CanOpenTrade = true; bb_Source = CreateDataSeries(); bb = Indicators.BollingerBands(MarketSeries.Close, bb_Periods, bb_stdDev, MovingAverageType.Simple); } protected override void OnBar() { if (MarketSeries.Close.Last(0) < bb.Top.Last(0) && MarketSeries.Close.Last(1) > bb.Top.Last(1) && CanOpenTrade) { var result = ExecuteMarketOrder(TradeType.Sell, Symbol, Volume, "BollyBand_Sell", StopLoss, null); if (result.IsSuccessful) { position = result.Position; CanOpenTrade = false; } } /////////////////// if (MarketSeries.Close.Last(0) > bb.Bottom.Last(0) && MarketSeries.Close.Last(1) < bb.Bottom.Last(1) && CanOpenTrade) { ChartObjects.DrawText("Buy_Signal" + MarketSeries.Open.Count, Bullet, MarketSeries.Open.Count, MarketSeries.High.LastValue, vAlign, hAlign, Colors.Orange); var result = ExecuteMarketOrder(TradeType.Buy, Symbol, Volume, "BollyBand_Buy", StopLoss, null); if (result.IsSuccessful) { position = result.Position; CanOpenTrade = false; } } } protected override void OnTick() { // Put your core logic here if (Positions.Count != 0 && position.TradeType == TradeType.Sell) { if (MarketSeries.Close.Last(0) >= bb.Main.Last(0))// Main or Bottom ???? ClosePosition(position); CanOpenTrade = true; } if (Positions.Count != 0 && position.TradeType == TradeType.Buy) { if (MarketSeries.Close.Last(0) <= bb.Main.Last(0)) // Main or TOP ????? ClosePosition(position); CanOpenTrade = true; } } protected override void OnStop() { // Put your deinitialization logic here } } }
But This is so simplified and there is no Real TP or SL Targets here .
usually the best SL & TP is related to ATR of that TF .
I Don't think it's a winning strategy , Bollinger bands are based on volatility .. So if Price Goes UP till it hits the upper band and then reverses to the lower band .. and then
exceeds the lower band and then reverses and Goes Up again and hit the upper band again .. and keep doing this imaginary cycle .. This could be a winning strategy
obviously this doesn't happen
@cyfer
cyfer
29 Mar 2016, 09:34
( Updated at: 21 Dec 2023, 09:20 )
I can't really see what you mean .. it is working as it should
a simpler way to look at this is to set the chart time frame to Monthly and see where your lines are drawn .. if they match the H & L of the monthly candle .. then its working fine
@cyfer
cyfer
29 Mar 2016, 08:45
( Updated at: 21 Dec 2023, 09:20 )
I Can't find anything wrong with Hi & Lo from GetSeries
private MarketSeries M_Series; protected override void Initialize() { M_Series = MarketData.GetSeries(Symbol, TimeFrame.Monthly); } public override void Calculate(int index) { ChartObjects.DrawHorizontalLine("HI", M_Series.High.Last(0), Colors.Green); ChartObjects.DrawHorizontalLine("LO", M_Series.Low.Last(0), Colors.Yellow); }
Just this code works very fine with no problem , and if you set it to .Last(whatever) it works accurately .
One thing though that may cause confusion here , The Time Zone you set at the lower right end of cTrader Interface
here is an example of My Zone +2 & then ServerTime Zone (Pepperstone )
@cyfer
cyfer
25 Mar 2016, 21:08
Save yourself the headache and do this
using System; using cAlgo.API; using cAlgo.API.Internals; using cAlgo.API.Indicators; namespace cAlgo.Indicators { [Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AutoRescale = true)] public class MultiSymbolMarketInfo : Indicator { private Symbol symbol1; private Symbol symbol2; private Symbol symbol3; private Symbol symbol4; private Symbol symbol5; private Symbol symbol6; private Symbol symbol7; [Parameter(DefaultValue = "EURGBP")] public string Symbol1 { get; set; } [Parameter(DefaultValue = "GBPUSD")] public string Symbol2 { get; set; } [Parameter(DefaultValue = "EURUSD")] public string Symbol3 { get; set; } [Parameter(DefaultValue = "EURJPY")] public string Symbol4 { get; set; } [Parameter(DefaultValue = "AUDJPY")] public string Symbol5 { get; set; } [Parameter(DefaultValue = "EURCAD")] public string Symbol6 { get; set; } [Parameter(DefaultValue = "GBPJPY")] public string Symbol7 { get; set; } protected override void Initialize() { symbol1 = MarketData.GetSymbol(Symbol1); symbol2 = MarketData.GetSymbol(Symbol2); symbol3 = MarketData.GetSymbol(Symbol3); symbol4 = MarketData.GetSymbol(Symbol4); symbol5 = MarketData.GetSymbol(Symbol5); symbol6 = MarketData.GetSymbol(Symbol6); symbol7 = MarketData.GetSymbol(Symbol7); } public override void Calculate(int index) { if (!IsLastBar) return; var text = FormatSymbol(symbol1) + "\n" + FormatSymbol(symbol2) + "\n" + FormatSymbol(symbol3) + "\n" + FormatSymbol(symbol4) + "\n" + FormatSymbol(symbol5) + "\n" + FormatSymbol(symbol6) + "\n" + FormatSymbol(symbol7); ChartObjects.RemoveObject(text); ChartObjects.DrawText("symbol1", text, StaticPosition.TopLeft, Colors.Lime); } private string FormatSymbol(Symbol symbol) { var spread = Math.Round(symbol.Spread / symbol.PipSize, 1); if (symbol.Code.Contains("JPY")) { return string.Format("{0}\t\t Ask: {1}\t Bid: {2}\t Spread: {3}", symbol.Code, symbol.Ask, symbol.Bid, spread); } else return string.Format("{0}\t Ask: {1}\t Bid: {2}\t Spread: {3}", symbol.Code, symbol.Ask, symbol.Bid, spread); } } }
*I have nothing to say about your browser issue , I'm using Opera browser
@cyfer
cyfer
07 Mar 2016, 00:03
A Cross is only considered a Cross after the Bar Closes not before
A Cross can happen before the candle closes and then retreats and becomes a Touch not a Cross .
You can't count on a momentary Cross .. You'll get too many false signals
make your Calculations in OnBar() method , So it will happen on the beginning of each new bar , and yes you should test Bar(2)&Bar(1)
Bar(0) Doesn't exist till it Closes
**********************************
Even if your indicator is working on 1M TimeFrame & your Bot is working on 5M or higher , you'll still test Bar(2)&Bar(1) but then you should put the calculations
in OnTick() method
@cyfer
cyfer
06 Mar 2016, 05:02
With such little code , it's impossible to tell
However , You should be aware that Last(0) represents the Current Bar , I.e .. It didn't finish yet .. so with each tick you'll have different values
the only value you can count on before the bar closes is the Open Value .. the other 3 values may change completely in 1 tick .
@cyfer
cyfer
06 Mar 2016, 01:47
How to round decimal numbers in C# like you do in C language ?
Print(Math.Round(14.125,0)); //--> Returns a 14 in C#
Print(Math.Round(14.935, 0)); //--> Returns 15
and If you want to Round it so it has some decimal points you can specify that in the 2nd parameter of Round Method .
@cyfer
cyfer
04 Mar 2016, 16:41
public override void Calculate(int index) { var daily = MarketData.GetSeries(TimeFrame.Daily); var indexDaily = daily.OpenTime.GetIndexByTime(MarketSeries.OpenTime.LastValue); double daily_range1 = Math.Abs(daily.High[index - 1] - daily.Low[index - 1]); double daily_range2 = Math.Abs(daily.High[index - 2] - daily.Low[index - 2]); if (daily_range1 > daily_range2 && daily.High[indexDaily - 1] < daily.High[indexDaily - 2] && daily.Low[indexDaily - 1] < daily.Low[indexDaily - 2]) ChartObjects.DrawText(index.ToString(), "X", index - 1, MarketSeries.High[index], VerticalAlignment.Center, HorizontalAlignment.Center, Colors.Red); }
- Get the Daily Series in Initialize not Calculate
- I also see no good reason to Get the Ranges At Calculate Method, eventually you're comparing the Past 2 days ranges .. so they should be done once
- Index in Calculate method is the Index of the Time Frame you're working on ., so currently you're using the index of that time frame "Just like you use in the draw Method"
@cyfer
cyfer
01 Mar 2016, 16:36
Because Spotware provided us "Retail Traders/Developers" with a limited API
you can use the Currency Pair & TimeFrame because they built those as a collection
even when you want to make a collection of Strings[] , you can't
the only way is (int) with a Max & Min values .. so you'll get the slider but other than that , AFAIK there is nothing .
Speaking of Limitations , you'll face the need for a scanner that goes through various currency pairs , then you'll meet another limitations
for example , we should've had the ability to create a custom window that docks into the platform "like news in FXpro"platform or Favorites in currency tab
we also should be able to make Algos without the need to inherit from indicators or Bots / or inherit specific Functions only "Like OnBar & OnTick"
did you notice that we can't even display a visual alert "like the one you see when you set a Price Alert" and we have to go for winAPI & Extern methods ?
unfortunately , that's the way it is .
I hope I'm wrong .. but I don't think so .
@cyfer
cyfer
01 Mar 2016, 13:03
I tried it so many times with enum and array and not a single Success
Spotware is not providing a Parameter that can hold a collection , so no matter what hacks you can do .. you just can't get around this limitation
there is no type we can access that can hold a collection , only Spotware built-in types like TimeFrame & Symbol
@cyfer
cyfer
02 Aug 2016, 18:50
Don't know if your trade is still open , If it is Try to run this code
@cyfer