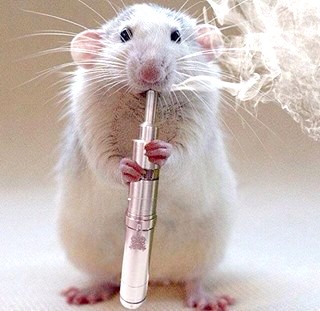
Indicator lines (2 colors)
16 Feb 2016, 02:21
Is there any hack to color the overbough & oversold parts with different colors than the rest of the line ?
I.e normal color : green .. overbought : red
I tried filling these areas with vertical lines .. but it looks horrible .
Replies
whis.gg
16 Feb 2016, 13:17
RE:
cyfer said:
it does catch some values outside the range (30-70 or whatever the range)
If you want to filter that, you might apply this method, although it's kinda sketchy.
using System; using cAlgo.API; using cAlgo.API.Internals; using cAlgo.API.Indicators; using cAlgo.Indicators; namespace cAlgo { [Levels(20, 80)] [Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class StochasticColored : Indicator { [Output("Result", Color = Colors.Gray)] public IndicatorDataSeries Result { get; set; } [Output("Overbought", Color = Colors.LimeGreen, Thickness = 2, PlotType = PlotType.DiscontinuousLine)] public IndicatorDataSeries Overbought { get; set; } [Output("Oversold", Color = Colors.Red, Thickness = 2, PlotType = PlotType.DiscontinuousLine)] public IndicatorDataSeries Oversold { get; set; } private StochasticOscillator stoch; protected override void Initialize() { stoch = Indicators.StochasticOscillator(9, 3, 9, MovingAverageType.Simple); } public override void Calculate(int index) { Result[index] = stoch.PercentK.LastValue; // Overbought if (Result[index] > 80) { if (Result[index - 1] < 80) Overbought[index - 1] = 80; Overbought[index] = Result[index]; } else if (Result[index - 1] > 80 && Result[index] < 80) Overbought[index] = 80; // Oversold if (Result[index] < 20) { if (Result[index - 1] > 20) Oversold[index - 1] = 20; Oversold[index] = Result[index]; } else if (Result[index - 1] < 20 && Result[index] > 20) Oversold[index] = 20; } } }
@whis.gg
whis.gg
16 Feb 2016, 02:43
Hey, here is workaround I use. Notice that I define previous value of series as well to make sure discontinuous line are plotted properly.
Result:
@whis.gg