Description
This sample indicator allows you to draw most popular Fibonacci drawing tools on your chart.
It's designed to work like a built-in feature of cTrader platform, you can draw any of the Fibonacci drawing tools by clicking on the indicator buttons menu for that specific tool and then click on your chart to plot it.
The Fibonacci Drawing is open source and if you are a cTrader automate API developer you can use this as a sample for developing highly sophisticated add-ons for cTrader by using the full power of cTrader automate API.
Features
Have almost all popular Fibonacci drawing tools like retracement, expansion, speed resistance fan, time zone, trend based time, and channel
Works like a built-in feature of cTrader
Doesn't occupy too much space on your chart, you can expand/collapse it anytime
Save/load drawings, you can share your drawings with other cTrader users and they can just load it on their charts
Full customizable, you can change each drawing specific settings based on your needs
Show or hide all drawn objects by clicking on indicator show/hide buttons
Remove all drawn objects with one click
Multi time frame filter
This indicator is open source, if you are interested you can contribute on Github:
Changelog
Version 1.2.0.0
Release Date: January 26, 2023
Changed: String color parameters to new Color type parameter
Note: This version only works on cTrader 4.6 or above.
Version 1.1.0.0
Release Date: December 20, 2022
Fixed: Issues with new versions of cTrader
Changed: Target framework from .NET 4 to 6
Version 1.0.2.0
Release Date: June 10, 2021
Added: New option for showing/hiding the price and time levels of Fibonacci Resistance fan
Fixed: A major bug on Fan patterns
Version 1.0.1.0
Release Date: June 8, 2021
Added: Fibonacci Expansion drawing tool
Changed: Improved logger
using cAlgo.API;
using cAlgo.Controls;
using cAlgo.Helpers;
using cAlgo.Patterns;
using System.Collections.Generic;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)]
public class FibonacciDrawing : Indicator
{
private StackPanel _mainButtonsPanel;
private StackPanel _groupButtonsPanel;
private StackPanel _mainPanel;
private Style _buttonsStyle;
private readonly List<Button> _buttons = new List<Button>();
private Button _expandButton;
#region Patterns color parameters
[Parameter("Color", DefaultValue = "70FFC000", Group = "Patterns Color")]
public Color PatternsColor { get; set; }
#endregion Patterns color parameters
#region Patterns Label parameters
[Parameter("Show", DefaultValue = true, Group = "Patterns Label")]
public bool PatternsLabelShow { get; set; }
[Parameter("Color", DefaultValue = "Yellow", Group = "Patterns Label")]
public Color PatternsLabelColor { get; set; }
[Parameter("Locked", DefaultValue = true, Group = "Patterns Label")]
public bool PatternsLabelLocked { get; set; }
[Parameter("Link Style", DefaultValue = true, Group = "Patterns Label")]
public bool PatternsLabelLinkStyle { get; set; }
#endregion Patterns Label parameters
#region Container Panel parameters
[Parameter("Orientation", DefaultValue = Orientation.Vertical, Group = "Container Panel")]
public Orientation PanelOrientation { get; set; }
[Parameter("Horizontal Alignment", DefaultValue = HorizontalAlignment.Left, Group = "Container Panel")]
public HorizontalAlignment PanelHorizontalAlignment { get; set; }
[Parameter("Vertical Alignment", DefaultValue = VerticalAlignment.Top, Group = "Container Panel")]
public VerticalAlignment PanelVerticalAlignment { get; set; }
[Parameter("Margin", DefaultValue = 3, Group = "Container Panel")]
public double PanelMargin { get; set; }
#endregion Container Panel parameters
#region Buttons parameters
[Parameter("Disable Color", DefaultValue = "#FFCCCCCC", Group = "Buttons")]
public Color ButtonsBackgroundDisableColor { get; set; }
[Parameter("Enable Color", DefaultValue = "Red", Group = "Buttons")]
public Color ButtonsBackgroundEnableColor { get; set; }
[Parameter("Text Color", DefaultValue = "Blue", Group = "Buttons")]
public Color ButtonsForegroundColor { get; set; }
[Parameter("Margin", DefaultValue = 1, Group = "Buttons")]
public double ButtonsMargin { get; set; }
[Parameter("Transparency", DefaultValue = 0.5, MinValue = 0, MaxValue = 1, Group = "Buttons")]
public double ButtonsTransparency { get; set; }
#endregion Buttons parameters
#region TimeFrame Visibility parameters
[Parameter("Enable", DefaultValue = false, Group = "TimeFrame Visibility")]
public bool IsTimeFrameVisibilityEnabled { get; set; }
[Parameter("TimeFrame", Group = "TimeFrame Visibility")]
public TimeFrame VisibilityTimeFrame { get; set; }
[Parameter("Only Buttons", Group = "TimeFrame Visibility")]
public bool VisibilityOnlyButtons { get; set; }
#endregion TimeFrame Visibility parameters
#region Fibonacci Retracement parameters
[Parameter("Show 1st Level", DefaultValue = true, Group = "Fibonacci Retracement")]
public bool ShowFirstFibonacciRetracement { get; set; }
[Parameter("Fill 1st Level", DefaultValue = true, Group = "Fibonacci Retracement")]
public bool FillFirstFibonacciRetracement { get; set; }
[Parameter("1st Level Percent", DefaultValue = 0, Group = "Fibonacci Retracement")]
public double FirstFibonacciRetracementPercent { get; set; }
[Parameter("1st Level Color", DefaultValue = "Gray", Group = "Fibonacci Retracement")]
public Color FirstFibonacciRetracementColor { get; set; }
[Parameter("1st Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Retracement")]
public int FirstFibonacciRetracementThickness { get; set; }
[Parameter("1st Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Retracement")]
public LineStyle FirstFibonacciRetracementStyle { get; set; }
[Parameter("1st Level Extend To Infinity", DefaultValue = false, Group = "Fibonacci Retracement")]
public bool FirstFibonacciRetracementExtendToInfinity { get; set; }
[Parameter("Show 2nd Level", DefaultValue = true, Group = "Fibonacci Retracement")]
public bool ShowSecondFibonacciRetracement { get; set; }
[Parameter("Fill 2nd Level", DefaultValue = true, Group = "Fibonacci Retracement")]
public bool FillSecondFibonacciRetracement { get; set; }
[Parameter("2nd Level Percent", DefaultValue = 0.236, Group = "Fibonacci Retracement")]
public double SecondFibonacciRetracementPercent { get; set; }
[Parameter("2nd Level Color", DefaultValue = "Red", Group = "Fibonacci Retracement")]
public Color SecondFibonacciRetracementColor { get; set; }
[Parameter("2nd Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Retracement")]
public int SecondFibonacciRetracementThickness { get; set; }
[Parameter("2nd Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Retracement")]
public LineStyle SecondFibonacciRetracementStyle { get; set; }
[Parameter("2nd Level Extend To Infinity", DefaultValue = false, Group = "Fibonacci Retracement")]
public bool SecondFibonacciRetracementExtendToInfinity { get; set; }
[Parameter("Show 3rd Level", DefaultValue = true, Group = "Fibonacci Retracement")]
public bool ShowThirdFibonacciRetracement { get; set; }
[Parameter("Fill 3rd Level", DefaultValue = true, Group = "Fibonacci Retracement")]
public bool FillThirdFibonacciRetracement { get; set; }
[Parameter("3rd Level Percent", DefaultValue = 0.382, Group = "Fibonacci Retracement")]
public double ThirdFibonacciRetracementPercent { get; set; }
[Parameter("3rd Level Color", DefaultValue = "GreenYellow", Group = "Fibonacci Retracement")]
public Color ThirdFibonacciRetracementColor { get; set; }
[Parameter("3rd Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Retracement")]
public int ThirdFibonacciRetracementThickness { get; set; }
[Parameter("3rd Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Retracement")]
public LineStyle ThirdFibonacciRetracementStyle { get; set; }
[Parameter("3rd Level Extend To Infinity", DefaultValue = false, Group = "Fibonacci Retracement")]
public bool ThirdFibonacciRetracementExtendToInfinity { get; set; }
[Parameter("Show 4th Level", DefaultValue = true, Group = "Fibonacci Retracement")]
public bool ShowFourthFibonacciRetracement { get; set; }
[Parameter("Fill 4th Level", DefaultValue = true, Group = "Fibonacci Retracement")]
public bool FillFourthFibonacciRetracement { get; set; }
[Parameter("4th Level Percent", DefaultValue = 0.5, Group = "Fibonacci Retracement")]
public double FourthFibonacciRetracementPercent { get; set; }
[Parameter("4th Level Color", DefaultValue = "DarkGreen", Group = "Fibonacci Retracement")]
public Color FourthFibonacciRetracementColor { get; set; }
[Parameter("4th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Retracement")]
public int FourthFibonacciRetracementThickness { get; set; }
[Parameter("4th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Retracement")]
public LineStyle FourthFibonacciRetracementStyle { get; set; }
[Parameter("4th Level Extend To Infinity", DefaultValue = false, Group = "Fibonacci Retracement")]
public bool FourthFibonacciRetracementExtendToInfinity { get; set; }
[Parameter("Show 5th Level", DefaultValue = true, Group = "Fibonacci Retracement")]
public bool ShowFifthFibonacciRetracement { get; set; }
[Parameter("Fill 5th Level", DefaultValue = true, Group = "Fibonacci Retracement")]
public bool FillFifthFibonacciRetracement { get; set; }
[Parameter("5th Level Percent", DefaultValue = 0.618, Group = "Fibonacci Retracement")]
public double FifthFibonacciRetracementPercent { get; set; }
[Parameter("5th Level Color", DefaultValue = "BlueViolet", Group = "Fibonacci Retracement")]
public Color FifthFibonacciRetracementColor { get; set; }
[Parameter("5th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Retracement")]
public int FifthFibonacciRetracementThickness { get; set; }
[Parameter("5th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Retracement")]
public LineStyle FifthFibonacciRetracementStyle { get; set; }
[Parameter("5th Level Extend To Infinity", DefaultValue = false, Group = "Fibonacci Retracement")]
public bool FifthFibonacciRetracementExtendToInfinity { get; set; }
[Parameter("Show 6th Level", DefaultValue = true, Group = "Fibonacci Retracement")]
public bool ShowSixthFibonacciRetracement { get; set; }
[Parameter("Fill 6th Level", DefaultValue = true, Group = "Fibonacci Retracement")]
public bool FillSixthFibonacciRetracement { get; set; }
[Parameter("6th Level Percent", DefaultValue = 0.786, Group = "Fibonacci Retracement")]
public double SixthFibonacciRetracementPercent { get; set; }
[Parameter("6th Level Color", DefaultValue = "AliceBlue", Group = "Fibonacci Retracement")]
public Color SixthFibonacciRetracementColor { get; set; }
[Parameter("6th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Retracement")]
public int SixthFibonacciRetracementThickness { get; set; }
[Parameter("6th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Retracement")]
public LineStyle SixthFibonacciRetracementStyle { get; set; }
[Parameter("6th Level Extend To Infinity", DefaultValue = false, Group = "Fibonacci Retracement")]
public bool SixthFibonacciRetracementExtendToInfinity { get; set; }
[Parameter("Show 7th Level", DefaultValue = true, Group = "Fibonacci Retracement")]
public bool ShowSeventhFibonacciRetracement { get; set; }
[Parameter("Fill 7th Level", DefaultValue = true, Group = "Fibonacci Retracement")]
public bool FillSeventhFibonacciRetracement { get; set; }
[Parameter("7th Level Percent", DefaultValue = 1, Group = "Fibonacci Retracement")]
public double SeventhFibonacciRetracementPercent { get; set; }
[Parameter("7th Level Color", DefaultValue = "Bisque", Group = "Fibonacci Retracement")]
public Color SeventhFibonacciRetracementColor { get; set; }
[Parameter("7th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Retracement")]
public int SeventhFibonacciRetracementThickness { get; set; }
[Parameter("7th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Retracement")]
public LineStyle SeventhFibonacciRetracementStyle { get; set; }
[Parameter("7th Level Extend To Infinity", DefaultValue = false, Group = "Fibonacci Retracement")]
public bool SeventhFibonacciRetracementExtendToInfinity { get; set; }
[Parameter("Show 8th Level", DefaultValue = true, Group = "Fibonacci Retracement")]
public bool ShowEighthFibonacciRetracement { get; set; }
[Parameter("Fill 8th Level", DefaultValue = true, Group = "Fibonacci Retracement")]
public bool FillEighthFibonacciRetracement { get; set; }
[Parameter("8th Level Percent", DefaultValue = 1.618, Group = "Fibonacci Retracement")]
public double EighthFibonacciRetracementPercent { get; set; }
[Parameter("8th Level Color", DefaultValue = "Azure", Group = "Fibonacci Retracement")]
public Color EighthFibonacciRetracementColor { get; set; }
[Parameter("8th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Retracement")]
public int EighthFibonacciRetracementThickness { get; set; }
[Parameter("8th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Retracement")]
public LineStyle EighthFibonacciRetracementStyle { get; set; }
[Parameter("8th Level Extend To Infinity", DefaultValue = false, Group = "Fibonacci Retracement")]
public bool EighthFibonacciRetracementExtendToInfinity { get; set; }
[Parameter("Show 9th Level", DefaultValue = true, Group = "Fibonacci Retracement")]
public bool ShowNinthFibonacciRetracement { get; set; }
[Parameter("Fill 9th Level", DefaultValue = true, Group = "Fibonacci Retracement")]
public bool FillNinthFibonacciRetracement { get; set; }
[Parameter("9th Level Percent", DefaultValue = 2.618, Group = "Fibonacci Retracement")]
public double NinthFibonacciRetracementPercent { get; set; }
[Parameter("9th Level Color", DefaultValue = "Aqua", Group = "Fibonacci Retracement")]
public Color NinthFibonacciRetracementColor { get; set; }
[Parameter("9th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Retracement")]
public int NinthFibonacciRetracementThickness { get; set; }
[Parameter("9th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Retracement")]
public LineStyle NinthFibonacciRetracementStyle { get; set; }
[Parameter("9th Level Extend To Infinity", DefaultValue = false, Group = "Fibonacci Retracement")]
public bool NinthFibonacciRetracementExtendToInfinity { get; set; }
[Parameter("Show 10th Level", DefaultValue = true, Group = "Fibonacci Retracement")]
public bool ShowTenthFibonacciRetracement { get; set; }
[Parameter("Fill 10th Level", DefaultValue = true, Group = "Fibonacci Retracement")]
public bool FillTenthFibonacciRetracement { get; set; }
[Parameter("10th Level Percent", DefaultValue = 3.618, Group = "Fibonacci Retracement")]
public double TenthFibonacciRetracementPercent { get; set; }
[Parameter("10th Level Color", DefaultValue = "Aquamarine", Group = "Fibonacci Retracement")]
public Color TenthFibonacciRetracementColor { get; set; }
[Parameter("10th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Retracement")]
public int TenthFibonacciRetracementThickness { get; set; }
[Parameter("10th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Retracement")]
public LineStyle TenthFibonacciRetracementStyle { get; set; }
[Parameter("10th Level Extend To Infinity", DefaultValue = false, Group = "Fibonacci Retracement")]
public bool TenthFibonacciRetracementExtendToInfinity { get; set; }
[Parameter("Show 11th Level", DefaultValue = true, Group = "Fibonacci Retracement")]
public bool ShowEleventhFibonacciRetracement { get; set; }
[Parameter("Fill 11th Level", DefaultValue = true, Group = "Fibonacci Retracement")]
public bool FillEleventhFibonacciRetracement { get; set; }
[Parameter("11th Level Percent", DefaultValue = 4.236, Group = "Fibonacci Retracement")]
public double EleventhFibonacciRetracementPercent { get; set; }
[Parameter("11th Level Color", DefaultValue = "Chocolate", Group = "Fibonacci Retracement")]
public Color EleventhFibonacciRetracementColor { get; set; }
[Parameter("11th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Retracement")]
public int EleventhFibonacciRetracementThickness { get; set; }
[Parameter("11th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Retracement")]
public LineStyle EleventhFibonacciRetracementStyle { get; set; }
[Parameter("11th Level Extend To Infinity", DefaultValue = false, Group = "Fibonacci Retracement")]
public bool EleventhFibonacciRetracementExtendToInfinity { get; set; }
#endregion Fibonacci Retracement parameters
#region Fibonacci Expansion parameters
[Parameter("Show 1st Level", DefaultValue = true, Group = "Fibonacci Expansion")]
public bool ShowFirstFibonacciExpansion { get; set; }
[Parameter("Fill 1st Level", DefaultValue = true, Group = "Fibonacci Expansion")]
public bool FillFirstFibonacciExpansion { get; set; }
[Parameter("1st Level Percent", DefaultValue = 0, Group = "Fibonacci Expansion")]
public double FirstFibonacciExpansionPercent { get; set; }
[Parameter("1st Level Color", DefaultValue = "Gray", Group = "Fibonacci Expansion")]
public Color FirstFibonacciExpansionColor { get; set; }
[Parameter("1st Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Expansion")]
public int FirstFibonacciExpansionThickness { get; set; }
[Parameter("1st Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Expansion")]
public LineStyle FirstFibonacciExpansionStyle { get; set; }
[Parameter("1st Level Extend To Infinity", DefaultValue = false, Group = "Fibonacci Expansion")]
public bool FirstFibonacciExpansionExtendToInfinity { get; set; }
[Parameter("Show 2nd Level", DefaultValue = true, Group = "Fibonacci Expansion")]
public bool ShowSecondFibonacciExpansion { get; set; }
[Parameter("Fill 2nd Level", DefaultValue = true, Group = "Fibonacci Expansion")]
public bool FillSecondFibonacciExpansion { get; set; }
[Parameter("2nd Level Percent", DefaultValue = 0.236, Group = "Fibonacci Expansion")]
public double SecondFibonacciExpansionPercent { get; set; }
[Parameter("2nd Level Color", DefaultValue = "Red", Group = "Fibonacci Expansion")]
public Color SecondFibonacciExpansionColor { get; set; }
[Parameter("2nd Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Expansion")]
public int SecondFibonacciExpansionThickness { get; set; }
[Parameter("2nd Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Expansion")]
public LineStyle SecondFibonacciExpansionStyle { get; set; }
[Parameter("2nd Level Extend To Infinity", DefaultValue = false, Group = "Fibonacci Expansion")]
public bool SecondFibonacciExpansionExtendToInfinity { get; set; }
[Parameter("Show 3rd Level", DefaultValue = true, Group = "Fibonacci Expansion")]
public bool ShowThirdFibonacciExpansion { get; set; }
[Parameter("Fill 3rd Level", DefaultValue = true, Group = "Fibonacci Expansion")]
public bool FillThirdFibonacciExpansion { get; set; }
[Parameter("3rd Level Percent", DefaultValue = 0.382, Group = "Fibonacci Expansion")]
public double ThirdFibonacciExpansionPercent { get; set; }
[Parameter("3rd Level Color", DefaultValue = "GreenYellow", Group = "Fibonacci Expansion")]
public Color ThirdFibonacciExpansionColor { get; set; }
[Parameter("3rd Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Expansion")]
public int ThirdFibonacciExpansionThickness { get; set; }
[Parameter("3rd Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Expansion")]
public LineStyle ThirdFibonacciExpansionStyle { get; set; }
[Parameter("3rd Level Extend To Infinity", DefaultValue = false, Group = "Fibonacci Expansion")]
public bool ThirdFibonacciExpansionExtendToInfinity { get; set; }
[Parameter("Show 4th Level", DefaultValue = true, Group = "Fibonacci Expansion")]
public bool ShowFourthFibonacciExpansion { get; set; }
[Parameter("Fill 4th Level", DefaultValue = true, Group = "Fibonacci Expansion")]
public bool FillFourthFibonacciExpansion { get; set; }
[Parameter("4th Level Percent", DefaultValue = 0.5, Group = "Fibonacci Expansion")]
public double FourthFibonacciExpansionPercent { get; set; }
[Parameter("4th Level Color", DefaultValue = "DarkGreen", Group = "Fibonacci Expansion")]
public Color FourthFibonacciExpansionColor { get; set; }
[Parameter("4th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Expansion")]
public int FourthFibonacciExpansionThickness { get; set; }
[Parameter("4th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Expansion")]
public LineStyle FourthFibonacciExpansionStyle { get; set; }
[Parameter("4th Level Extend To Infinity", DefaultValue = false, Group = "Fibonacci Expansion")]
public bool FourthFibonacciExpansionExtendToInfinity { get; set; }
[Parameter("Show 5th Level", DefaultValue = true, Group = "Fibonacci Expansion")]
public bool ShowFifthFibonacciExpansion { get; set; }
[Parameter("Fill 5th Level", DefaultValue = true, Group = "Fibonacci Expansion")]
public bool FillFifthFibonacciExpansion { get; set; }
[Parameter("5th Level Percent", DefaultValue = 0.618, Group = "Fibonacci Expansion")]
public double FifthFibonacciExpansionPercent { get; set; }
[Parameter("5th Level Color", DefaultValue = "BlueViolet", Group = "Fibonacci Expansion")]
public Color FifthFibonacciExpansionColor { get; set; }
[Parameter("5th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Expansion")]
public int FifthFibonacciExpansionThickness { get; set; }
[Parameter("5th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Expansion")]
public LineStyle FifthFibonacciExpansionStyle { get; set; }
[Parameter("5th Level Extend To Infinity", DefaultValue = false, Group = "Fibonacci Expansion")]
public bool FifthFibonacciExpansionExtendToInfinity { get; set; }
[Parameter("Show 6th Level", DefaultValue = true, Group = "Fibonacci Expansion")]
public bool ShowSixthFibonacciExpansion { get; set; }
[Parameter("Fill 6th Level", DefaultValue = true, Group = "Fibonacci Expansion")]
public bool FillSixthFibonacciExpansion { get; set; }
[Parameter("6th Level Percent", DefaultValue = 0.786, Group = "Fibonacci Expansion")]
public double SixthFibonacciExpansionPercent { get; set; }
[Parameter("6th Level Color", DefaultValue = "AliceBlue", Group = "Fibonacci Expansion")]
public Color SixthFibonacciExpansionColor { get; set; }
[Parameter("6th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Expansion")]
public int SixthFibonacciExpansionThickness { get; set; }
[Parameter("6th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Expansion")]
public LineStyle SixthFibonacciExpansionStyle { get; set; }
[Parameter("6th Level Extend To Infinity", DefaultValue = false, Group = "Fibonacci Expansion")]
public bool SixthFibonacciExpansionExtendToInfinity { get; set; }
[Parameter("Show 7th Level", DefaultValue = true, Group = "Fibonacci Expansion")]
public bool ShowSeventhFibonacciExpansion { get; set; }
[Parameter("Fill 7th Level", DefaultValue = true, Group = "Fibonacci Expansion")]
public bool FillSeventhFibonacciExpansion { get; set; }
[Parameter("7th Level Percent", DefaultValue = 1, Group = "Fibonacci Expansion")]
public double SeventhFibonacciExpansionPercent { get; set; }
[Parameter("7th Level Color", DefaultValue = "Bisque", Group = "Fibonacci Expansion")]
public Color SeventhFibonacciExpansionColor { get; set; }
[Parameter("7th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Expansion")]
public int SeventhFibonacciExpansionThickness { get; set; }
[Parameter("7th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Expansion")]
public LineStyle SeventhFibonacciExpansionStyle { get; set; }
[Parameter("7th Level Extend To Infinity", DefaultValue = false, Group = "Fibonacci Expansion")]
public bool SeventhFibonacciExpansionExtendToInfinity { get; set; }
[Parameter("Show 8th Level", DefaultValue = true, Group = "Fibonacci Expansion")]
public bool ShowEighthFibonacciExpansion { get; set; }
[Parameter("Fill 8th Level", DefaultValue = true, Group = "Fibonacci Expansion")]
public bool FillEighthFibonacciExpansion { get; set; }
[Parameter("8th Level Percent", DefaultValue = 1.618, Group = "Fibonacci Expansion")]
public double EighthFibonacciExpansionPercent { get; set; }
[Parameter("8th Level Color", DefaultValue = "Azure", Group = "Fibonacci Expansion")]
public Color EighthFibonacciExpansionColor { get; set; }
[Parameter("8th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Expansion")]
public int EighthFibonacciExpansionThickness { get; set; }
[Parameter("8th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Expansion")]
public LineStyle EighthFibonacciExpansionStyle { get; set; }
[Parameter("8th Level Extend To Infinity", DefaultValue = false, Group = "Fibonacci Expansion")]
public bool EighthFibonacciExpansionExtendToInfinity { get; set; }
[Parameter("Show 9th Level", DefaultValue = true, Group = "Fibonacci Expansion")]
public bool ShowNinthFibonacciExpansion { get; set; }
[Parameter("Fill 9th Level", DefaultValue = true, Group = "Fibonacci Expansion")]
public bool FillNinthFibonacciExpansion { get; set; }
[Parameter("9th Level Percent", DefaultValue = 2.618, Group = "Fibonacci Expansion")]
public double NinthFibonacciExpansionPercent { get; set; }
[Parameter("9th Level Color", DefaultValue = "Aqua", Group = "Fibonacci Expansion")]
public Color NinthFibonacciExpansionColor { get; set; }
[Parameter("9th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Expansion")]
public int NinthFibonacciExpansionThickness { get; set; }
[Parameter("9th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Expansion")]
public LineStyle NinthFibonacciExpansionStyle { get; set; }
[Parameter("9th Level Extend To Infinity", DefaultValue = false, Group = "Fibonacci Expansion")]
public bool NinthFibonacciExpansionExtendToInfinity { get; set; }
[Parameter("Show 10th Level", DefaultValue = true, Group = "Fibonacci Expansion")]
public bool ShowTenthFibonacciExpansion { get; set; }
[Parameter("Fill 10th Level", DefaultValue = true, Group = "Fibonacci Expansion")]
public bool FillTenthFibonacciExpansion { get; set; }
[Parameter("10th Level Percent", DefaultValue = 3.618, Group = "Fibonacci Expansion")]
public double TenthFibonacciExpansionPercent { get; set; }
[Parameter("10th Level Color", DefaultValue = "Aquamarine", Group = "Fibonacci Expansion")]
public Color TenthFibonacciExpansionColor { get; set; }
[Parameter("10th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Expansion")]
public int TenthFibonacciExpansionThickness { get; set; }
[Parameter("10th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Expansion")]
public LineStyle TenthFibonacciExpansionStyle { get; set; }
[Parameter("10th Level Extend To Infinity", DefaultValue = false, Group = "Fibonacci Expansion")]
public bool TenthFibonacciExpansionExtendToInfinity { get; set; }
[Parameter("Show 11th Level", DefaultValue = true, Group = "Fibonacci Expansion")]
public bool ShowEleventhFibonacciExpansion { get; set; }
[Parameter("Fill 11th Level", DefaultValue = true, Group = "Fibonacci Expansion")]
public bool FillEleventhFibonacciExpansion { get; set; }
[Parameter("11th Level Percent", DefaultValue = 4.236, Group = "Fibonacci Expansion")]
public double EleventhFibonacciExpansionPercent { get; set; }
[Parameter("11th Level Color", DefaultValue = "Chocolate", Group = "Fibonacci Expansion")]
public Color EleventhFibonacciExpansionColor { get; set; }
[Parameter("11th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Expansion")]
public int EleventhFibonacciExpansionThickness { get; set; }
[Parameter("11th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Expansion")]
public LineStyle EleventhFibonacciExpansionStyle { get; set; }
[Parameter("11th Level Extend To Infinity", DefaultValue = false, Group = "Fibonacci Expansion")]
public bool EleventhFibonacciExpansionExtendToInfinity { get; set; }
#endregion Fibonacci Expansion parameters
#region Fibonacci Speed Resistance Fan parameters
[Parameter("Rectangle Thickness", DefaultValue = 1, MinValue = 1, Group = "Fibonacci Speed Resistance Fan")]
public int FibonacciSpeedResistanceFanRectangleThickness { get; set; }
[Parameter("Rectangle Style", DefaultValue = LineStyle.Dots, Group = "Fibonacci Speed Resistance Fan")]
public LineStyle FibonacciSpeedResistanceFanRectangleStyle { get; set; }
[Parameter("Rectangle Color", DefaultValue = "Blue", Group = "Fibonacci Speed Resistance Fan")]
public Color FibonacciSpeedResistanceFanRectangleColor { get; set; }
[Parameter("Extended Lines Thickness", DefaultValue = 1, MinValue = 1, Group = "Fibonacci Speed Resistance Fan")]
public int FibonacciSpeedResistanceFanExtendedLinesThickness { get; set; }
[Parameter("Extended Lines Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Speed Resistance Fan")]
public LineStyle FibonacciSpeedResistanceFanExtendedLinesStyle { get; set; }
[Parameter("Extended Lines Color", DefaultValue = "Blue", Group = "Fibonacci Speed Resistance Fan")]
public Color FibonacciSpeedResistanceFanExtendedLinesColor { get; set; }
[Parameter("Show Price Levels", DefaultValue = true, Group = "Fibonacci Speed Resistance Fan")]
public bool FibonacciSpeedResistanceFanShowPriceLevels { get; set; }
[Parameter("Price Levels Thickness", DefaultValue = 1, MinValue = 1, Group = "Fibonacci Speed Resistance Fan")]
public int FibonacciSpeedResistanceFanPriceLevelsThickness { get; set; }
[Parameter("Price Levels Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Speed Resistance Fan")]
public LineStyle FibonacciSpeedResistanceFanPriceLevelsStyle { get; set; }
[Parameter("Price Levels Color", DefaultValue = "Magenta", Group = "Fibonacci Speed Resistance Fan")]
public Color FibonacciSpeedResistanceFanPriceLevelsColor { get; set; }
[Parameter("Show Time Levels", DefaultValue = true, Group = "Fibonacci Speed Resistance Fan")]
public bool FibonacciSpeedResistanceFanShowTimeLevels { get; set; }
[Parameter("Time Levels Thickness", DefaultValue = 1, MinValue = 1, Group = "Fibonacci Speed Resistance Fan")]
public int FibonacciSpeedResistanceFanTimeLevelsThickness { get; set; }
[Parameter("Time Levels Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Speed Resistance Fan")]
public LineStyle FibonacciSpeedResistanceFanTimeLevelsStyle { get; set; }
[Parameter("Time Levels Color", DefaultValue = "Yellow", Group = "Fibonacci Speed Resistance Fan")]
public Color FibonacciSpeedResistanceFanTimeLevelsColor { get; set; }
[Parameter("Main Fan Thickness", DefaultValue = 1, MinValue = 1, Group = "Fibonacci Speed Resistance Fan")]
public int FibonacciSpeedResistanceFanMainFanThickness { get; set; }
[Parameter("Main Fan Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Speed Resistance Fan")]
public LineStyle FibonacciSpeedResistanceFanMainFanStyle { get; set; }
[Parameter("Main Fan Color", DefaultValue = "Yellow", Group = "Fibonacci Speed Resistance Fan")]
public Color FibonacciSpeedResistanceFanMainFanColor { get; set; }
[Parameter("1st Fan Percent", DefaultValue = 0.25, Group = "Fibonacci Speed Resistance Fan")]
public double FibonacciSpeedResistanceFanFirstFanPercent { get; set; }
[Parameter("1st Fan Thickness", DefaultValue = 1, MinValue = 1, Group = "Fibonacci Speed Resistance Fan")]
public int FibonacciSpeedResistanceFanFirstFanThickness { get; set; }
[Parameter("1st Fan Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Speed Resistance Fan")]
public LineStyle FibonacciSpeedResistanceFanFirstFanStyle { get; set; }
[Parameter("1st Fan Color", DefaultValue = "Red", Group = "Fibonacci Speed Resistance Fan")]
public Color FibonacciSpeedResistanceFanFirstFanColor { get; set; }
[Parameter("2nd Fan Percent", DefaultValue = 0.382, Group = "Fibonacci Speed Resistance Fan")]
public double FibonacciSpeedResistanceFanSecondFanPercent { get; set; }
[Parameter("2nd Fan Thickness", DefaultValue = 1, MinValue = 1, Group = "Fibonacci Speed Resistance Fan")]
public int FibonacciSpeedResistanceFanSecondFanThickness { get; set; }
[Parameter("2nd Fan Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Speed Resistance Fan")]
public LineStyle FibonacciSpeedResistanceFanSecondFanStyle { get; set; }
[Parameter("2nd Fan Color", DefaultValue = "Brown", Group = "Fibonacci Speed Resistance Fan")]
public Color FibonacciSpeedResistanceFanSecondFanColor { get; set; }
[Parameter("3rd Fan Percent", DefaultValue = 0.5, Group = "Fibonacci Speed Resistance Fan")]
public double FibonacciSpeedResistanceFanThirdFanPercent { get; set; }
[Parameter("3rd Fan Thickness", DefaultValue = 1, MinValue = 1, Group = "Fibonacci Speed Resistance Fan")]
public int FibonacciSpeedResistanceFanThirdFanThickness { get; set; }
[Parameter("3rd Fan Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Speed Resistance Fan")]
public LineStyle FibonacciSpeedResistanceFanThirdFanStyle { get; set; }
[Parameter("3rd Fan Color", DefaultValue = "Lime", Group = "Fibonacci Speed Resistance Fan")]
public Color FibonacciSpeedResistanceFanThirdFanColor { get; set; }
[Parameter("4th Fan Percent", DefaultValue = 0.618, Group = "Fibonacci Speed Resistance Fan")]
public double FibonacciSpeedResistanceFanFourthFanPercent { get; set; }
[Parameter("4th Fan Thickness", DefaultValue = 1, MinValue = 1, Group = "Fibonacci Speed Resistance Fan")]
public int FibonacciSpeedResistanceFanFourthFanThickness { get; set; }
[Parameter("4th Fan Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Speed Resistance Fan")]
public LineStyle FibonacciSpeedResistanceFanFourthFanStyle { get; set; }
[Parameter("4th Fan Color", DefaultValue = "Magenta", Group = "Fibonacci Speed Resistance Fan")]
public Color FibonacciSpeedResistanceFanFourthFanColor { get; set; }
[Parameter("5th Fan Percent", DefaultValue = 0.75, Group = "Fibonacci Speed Resistance Fan")]
public double FibonacciSpeedResistanceFanFifthFanPercent { get; set; }
[Parameter("5th Fan Thickness", DefaultValue = 1, MinValue = 1, Group = "Fibonacci Speed Resistance Fan")]
public int FibonacciSpeedResistanceFanFifthFanThickness { get; set; }
[Parameter("5th Fan Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Speed Resistance Fan")]
public LineStyle FibonacciSpeedResistanceFanFifthFanStyle { get; set; }
[Parameter("5th Fan Color", DefaultValue = "Blue", Group = "Fibonacci Speed Resistance Fan")]
public Color FibonacciSpeedResistanceFanFifthFanColor { get; set; }
#endregion Fibonacci Speed Resistance Fan parameters
#region Fibonacci Time Zone parameters
[Parameter("Show 1st Level", DefaultValue = true, Group = "Fibonacci Time Zone")]
public bool ShowFirstFibonacciTimeZone { get; set; }
[Parameter("1st Level Percent", DefaultValue = 0, Group = "Fibonacci Time Zone")]
public double FirstFibonacciTimeZonePercent { get; set; }
[Parameter("1st Level Color", DefaultValue = "Gray", Group = "Fibonacci Time Zone")]
public Color FirstFibonacciTimeZoneColor { get; set; }
[Parameter("1st Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Time Zone")]
public int FirstFibonacciTimeZoneThickness { get; set; }
[Parameter("1st Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Time Zone")]
public LineStyle FirstFibonacciTimeZoneStyle { get; set; }
[Parameter("Show 2nd Level", DefaultValue = true, Group = "Fibonacci Time Zone")]
public bool ShowSecondFibonacciTimeZone { get; set; }
[Parameter("2nd Level Percent", DefaultValue = 1, Group = "Fibonacci Time Zone")]
public double SecondFibonacciTimeZonePercent { get; set; }
[Parameter("2nd Level Color", DefaultValue = "Red", Group = "Fibonacci Time Zone")]
public Color SecondFibonacciTimeZoneColor { get; set; }
[Parameter("2nd Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Time Zone")]
public int SecondFibonacciTimeZoneThickness { get; set; }
[Parameter("2nd Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Time Zone")]
public LineStyle SecondFibonacciTimeZoneStyle { get; set; }
[Parameter("Show 3rd Level", DefaultValue = true, Group = "Fibonacci Time Zone")]
public bool ShowThirdFibonacciTimeZone { get; set; }
[Parameter("3rd Level Percent", DefaultValue = 2, Group = "Fibonacci Time Zone")]
public double ThirdFibonacciTimeZonePercent { get; set; }
[Parameter("3rd Level Color", DefaultValue = "GreenYellow", Group = "Fibonacci Time Zone")]
public Color ThirdFibonacciTimeZoneColor { get; set; }
[Parameter("3rd Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Time Zone")]
public int ThirdFibonacciTimeZoneThickness { get; set; }
[Parameter("3rd Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Time Zone")]
public LineStyle ThirdFibonacciTimeZoneStyle { get; set; }
[Parameter("Show 4th Level", DefaultValue = true, Group = "Fibonacci Time Zone")]
public bool ShowFourthFibonacciTimeZone { get; set; }
[Parameter("4th Level Percent", DefaultValue = 3, Group = "Fibonacci Time Zone")]
public double FourthFibonacciTimeZonePercent { get; set; }
[Parameter("4th Level Color", DefaultValue = "DarkGreen", Group = "Fibonacci Time Zone")]
public Color FourthFibonacciTimeZoneColor { get; set; }
[Parameter("4th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Time Zone")]
public int FourthFibonacciTimeZoneThickness { get; set; }
[Parameter("4th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Time Zone")]
public LineStyle FourthFibonacciTimeZoneStyle { get; set; }
[Parameter("Show 5th Level", DefaultValue = true, Group = "Fibonacci Time Zone")]
public bool ShowFifthFibonacciTimeZone { get; set; }
[Parameter("5th Level Percent", DefaultValue = 5, Group = "Fibonacci Time Zone")]
public double FifthFibonacciTimeZonePercent { get; set; }
[Parameter("5th Level Color", DefaultValue = "BlueViolet", Group = "Fibonacci Time Zone")]
public Color FifthFibonacciTimeZoneColor { get; set; }
[Parameter("5th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Time Zone")]
public int FifthFibonacciTimeZoneThickness { get; set; }
[Parameter("5th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Time Zone")]
public LineStyle FifthFibonacciTimeZoneStyle { get; set; }
[Parameter("Show 6th Level", DefaultValue = true, Group = "Fibonacci Time Zone")]
public bool ShowSixthFibonacciTimeZone { get; set; }
[Parameter("6th Level Percent", DefaultValue = 8, Group = "Fibonacci Time Zone")]
public double SixthFibonacciTimeZonePercent { get; set; }
[Parameter("6th Level Color", DefaultValue = "AliceBlue", Group = "Fibonacci Time Zone")]
public Color SixthFibonacciTimeZoneColor { get; set; }
[Parameter("6th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Time Zone")]
public int SixthFibonacciTimeZoneThickness { get; set; }
[Parameter("6th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Time Zone")]
public LineStyle SixthFibonacciTimeZoneStyle { get; set; }
[Parameter("Show 7th Level", DefaultValue = true, Group = "Fibonacci Time Zone")]
public bool ShowSeventhFibonacciTimeZone { get; set; }
[Parameter("7th Level Percent", DefaultValue = 13, Group = "Fibonacci Time Zone")]
public double SeventhFibonacciTimeZonePercent { get; set; }
[Parameter("7th Level Color", DefaultValue = "Bisque", Group = "Fibonacci Time Zone")]
public Color SeventhFibonacciTimeZoneColor { get; set; }
[Parameter("7th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Time Zone")]
public int SeventhFibonacciTimeZoneThickness { get; set; }
[Parameter("7th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Time Zone")]
public LineStyle SeventhFibonacciTimeZoneStyle { get; set; }
[Parameter("Show 8th Level", DefaultValue = true, Group = "Fibonacci Time Zone")]
public bool ShowEighthFibonacciTimeZone { get; set; }
[Parameter("8th Level Percent", DefaultValue = 21, Group = "Fibonacci Time Zone")]
public double EighthFibonacciTimeZonePercent { get; set; }
[Parameter("8th Level Color", DefaultValue = "Azure", Group = "Fibonacci Time Zone")]
public Color EighthFibonacciTimeZoneColor { get; set; }
[Parameter("8th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Time Zone")]
public int EighthFibonacciTimeZoneThickness { get; set; }
[Parameter("8th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Time Zone")]
public LineStyle EighthFibonacciTimeZoneStyle { get; set; }
[Parameter("Show 9th Level", DefaultValue = true, Group = "Fibonacci Time Zone")]
public bool ShowNinthFibonacciTimeZone { get; set; }
[Parameter("9th Level Percent", DefaultValue = 34, Group = "Fibonacci Time Zone")]
public double NinthFibonacciTimeZonePercent { get; set; }
[Parameter("9th Level Color", DefaultValue = "Aqua", Group = "Fibonacci Time Zone")]
public Color NinthFibonacciTimeZoneColor { get; set; }
[Parameter("9th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Time Zone")]
public int NinthFibonacciTimeZoneThickness { get; set; }
[Parameter("9th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Time Zone")]
public LineStyle NinthFibonacciTimeZoneStyle { get; set; }
[Parameter("Show 10th Level", DefaultValue = true, Group = "Fibonacci Time Zone")]
public bool ShowTenthFibonacciTimeZone { get; set; }
[Parameter("10th Level Percent", DefaultValue = 55, Group = "Fibonacci Time Zone")]
public double TenthFibonacciTimeZonePercent { get; set; }
[Parameter("10th Level Color", DefaultValue = "Aquamarine", Group = "Fibonacci Time Zone")]
public Color TenthFibonacciTimeZoneColor { get; set; }
[Parameter("10th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Time Zone")]
public int TenthFibonacciTimeZoneThickness { get; set; }
[Parameter("10th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Time Zone")]
public LineStyle TenthFibonacciTimeZoneStyle { get; set; }
[Parameter("Show 11th Level", DefaultValue = true, Group = "Fibonacci Time Zone")]
public bool ShowEleventhFibonacciTimeZone { get; set; }
[Parameter("11th Level Percent", DefaultValue = 89, Group = "Fibonacci Time Zone")]
public double EleventhFibonacciTimeZonePercent { get; set; }
[Parameter("11th Level Color", DefaultValue = "Chocolate", Group = "Fibonacci Time Zone")]
public Color EleventhFibonacciTimeZoneColor { get; set; }
[Parameter("11th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Time Zone")]
public int EleventhFibonacciTimeZoneThickness { get; set; }
[Parameter("11th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Time Zone")]
public LineStyle EleventhFibonacciTimeZoneStyle { get; set; }
#endregion Fibonacci Time Zone parameters
#region Trend Based Fibonacci Time Parameters
[Parameter("Show 1st Level", DefaultValue = true, Group = "Trend Based Fibonacci Time")]
public bool ShowFirstTrendBasedFibonacciTime { get; set; }
[Parameter("1st Level Percent", DefaultValue = 0, Group = "Trend Based Fibonacci Time")]
public double FirstTrendBasedFibonacciTimePercent { get; set; }
[Parameter("1st Level Color", DefaultValue = "Gray", Group = "Trend Based Fibonacci Time")]
public Color FirstTrendBasedFibonacciTimeColor { get; set; }
[Parameter("1st Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Trend Based Fibonacci Time")]
public int FirstTrendBasedFibonacciTimeThickness { get; set; }
[Parameter("1st Level Style", DefaultValue = LineStyle.Solid, Group = "Trend Based Fibonacci Time")]
public LineStyle FirstTrendBasedFibonacciTimeStyle { get; set; }
[Parameter("Show 2nd Level", DefaultValue = true, Group = "Trend Based Fibonacci Time")]
public bool ShowSecondTrendBasedFibonacciTime { get; set; }
[Parameter("2nd Level Percent", DefaultValue = 0.382, Group = "Trend Based Fibonacci Time")]
public double SecondTrendBasedFibonacciTimePercent { get; set; }
[Parameter("2nd Level Color", DefaultValue = "Red", Group = "Trend Based Fibonacci Time")]
public Color SecondTrendBasedFibonacciTimeColor { get; set; }
[Parameter("2nd Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Trend Based Fibonacci Time")]
public int SecondTrendBasedFibonacciTimeThickness { get; set; }
[Parameter("2nd Level Style", DefaultValue = LineStyle.Solid, Group = "Trend Based Fibonacci Time")]
public LineStyle SecondTrendBasedFibonacciTimeStyle { get; set; }
[Parameter("Show 3rd Level", DefaultValue = true, Group = "Trend Based Fibonacci Time")]
public bool ShowThirdTrendBasedFibonacciTime { get; set; }
[Parameter("3rd Level Percent", DefaultValue = 0.5, Group = "Trend Based Fibonacci Time")]
public double ThirdTrendBasedFibonacciTimePercent { get; set; }
[Parameter("3rd Level Color", DefaultValue = "GreenYellow", Group = "Trend Based Fibonacci Time")]
public Color ThirdTrendBasedFibonacciTimeColor { get; set; }
[Parameter("3rd Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Trend Based Fibonacci Time")]
public int ThirdTrendBasedFibonacciTimeThickness { get; set; }
[Parameter("3rd Level Style", DefaultValue = LineStyle.Solid, Group = "Trend Based Fibonacci Time")]
public LineStyle ThirdTrendBasedFibonacciTimeStyle { get; set; }
[Parameter("Show 4th Level", DefaultValue = true, Group = "Trend Based Fibonacci Time")]
public bool ShowFourthTrendBasedFibonacciTime { get; set; }
[Parameter("4th Level Percent", DefaultValue = 0.618, Group = "Trend Based Fibonacci Time")]
public double FourthTrendBasedFibonacciTimePercent { get; set; }
[Parameter("4th Level Color", DefaultValue = "DarkGreen", Group = "Trend Based Fibonacci Time")]
public Color FourthTrendBasedFibonacciTimeColor { get; set; }
[Parameter("4th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Trend Based Fibonacci Time")]
public int FourthTrendBasedFibonacciTimeThickness { get; set; }
[Parameter("4th Level Style", DefaultValue = LineStyle.Solid, Group = "Trend Based Fibonacci Time")]
public LineStyle FourthTrendBasedFibonacciTimeStyle { get; set; }
[Parameter("Show 5th Level", DefaultValue = true, Group = "Trend Based Fibonacci Time")]
public bool ShowFifthTrendBasedFibonacciTime { get; set; }
[Parameter("5th Level Percent", DefaultValue = 1, Group = "Trend Based Fibonacci Time")]
public double FifthTrendBasedFibonacciTimePercent { get; set; }
[Parameter("5th Level Color", DefaultValue = "BlueViolet", Group = "Trend Based Fibonacci Time")]
public Color FifthTrendBasedFibonacciTimeColor { get; set; }
[Parameter("5th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Trend Based Fibonacci Time")]
public int FifthTrendBasedFibonacciTimeThickness { get; set; }
[Parameter("5th Level Style", DefaultValue = LineStyle.Solid, Group = "Trend Based Fibonacci Time")]
public LineStyle FifthTrendBasedFibonacciTimeStyle { get; set; }
[Parameter("Show 6th Level", DefaultValue = true, Group = "Trend Based Fibonacci Time")]
public bool ShowSixthTrendBasedFibonacciTime { get; set; }
[Parameter("6th Level Percent", DefaultValue = 1.382, Group = "Trend Based Fibonacci Time")]
public double SixthTrendBasedFibonacciTimePercent { get; set; }
[Parameter("6th Level Color", DefaultValue = "AliceBlue", Group = "Trend Based Fibonacci Time")]
public Color SixthTrendBasedFibonacciTimeColor { get; set; }
[Parameter("6th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Trend Based Fibonacci Time")]
public int SixthTrendBasedFibonacciTimeThickness { get; set; }
[Parameter("6th Level Style", DefaultValue = LineStyle.Solid, Group = "Trend Based Fibonacci Time")]
public LineStyle SixthTrendBasedFibonacciTimeStyle { get; set; }
[Parameter("Show 7th Level", DefaultValue = true, Group = "Trend Based Fibonacci Time")]
public bool ShowSeventhTrendBasedFibonacciTime { get; set; }
[Parameter("7th Level Percent", DefaultValue = 1.618, Group = "Trend Based Fibonacci Time")]
public double SeventhTrendBasedFibonacciTimePercent { get; set; }
[Parameter("7th Level Color", DefaultValue = "Bisque", Group = "Trend Based Fibonacci Time")]
public Color SeventhTrendBasedFibonacciTimeColor { get; set; }
[Parameter("7th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Trend Based Fibonacci Time")]
public int SeventhTrendBasedFibonacciTimeThickness { get; set; }
[Parameter("7th Level Style", DefaultValue = LineStyle.Solid, Group = "Trend Based Fibonacci Time")]
public LineStyle SeventhTrendBasedFibonacciTimeStyle { get; set; }
[Parameter("Show 8th Level", DefaultValue = true, Group = "Trend Based Fibonacci Time")]
public bool ShowEighthTrendBasedFibonacciTime { get; set; }
[Parameter("8th Level Percent", DefaultValue = 2, Group = "Trend Based Fibonacci Time")]
public double EighthTrendBasedFibonacciTimePercent { get; set; }
[Parameter("8th Level Color", DefaultValue = "Azure", Group = "Trend Based Fibonacci Time")]
public Color EighthTrendBasedFibonacciTimeColor { get; set; }
[Parameter("8th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Trend Based Fibonacci Time")]
public int EighthTrendBasedFibonacciTimeThickness { get; set; }
[Parameter("8th Level Style", DefaultValue = LineStyle.Solid, Group = "Trend Based Fibonacci Time")]
public LineStyle EighthTrendBasedFibonacciTimeStyle { get; set; }
[Parameter("Show 9th Level", DefaultValue = true, Group = "Trend Based Fibonacci Time")]
public bool ShowNinthTrendBasedFibonacciTime { get; set; }
[Parameter("9th Level Percent", DefaultValue = 2.382, Group = "Trend Based Fibonacci Time")]
public double NinthTrendBasedFibonacciTimePercent { get; set; }
[Parameter("9th Level Color", DefaultValue = "Aqua", Group = "Trend Based Fibonacci Time")]
public Color NinthTrendBasedFibonacciTimeColor { get; set; }
[Parameter("9th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Trend Based Fibonacci Time")]
public int NinthTrendBasedFibonacciTimeThickness { get; set; }
[Parameter("9th Level Style", DefaultValue = LineStyle.Solid, Group = "Trend Based Fibonacci Time")]
public LineStyle NinthTrendBasedFibonacciTimeStyle { get; set; }
[Parameter("Show 10th Level", DefaultValue = true, Group = "Trend Based Fibonacci Time")]
public bool ShowTenthTrendBasedFibonacciTime { get; set; }
[Parameter("10th Level Percent", DefaultValue = 2.618, Group = "Trend Based Fibonacci Time")]
public double TenthTrendBasedFibonacciTimePercent { get; set; }
[Parameter("10th Level Color", DefaultValue = "Aquamarine", Group = "Trend Based Fibonacci Time")]
public Color TenthTrendBasedFibonacciTimeColor { get; set; }
[Parameter("10th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Trend Based Fibonacci Time")]
public int TenthTrendBasedFibonacciTimeThickness { get; set; }
[Parameter("10th Level Style", DefaultValue = LineStyle.Solid, Group = "Trend Based Fibonacci Time")]
public LineStyle TenthTrendBasedFibonacciTimeStyle { get; set; }
[Parameter("Show 11th Level", DefaultValue = true, Group = "Trend Based Fibonacci Time")]
public bool ShowEleventhTrendBasedFibonacciTime { get; set; }
[Parameter("11th Level Percent", DefaultValue = 3, Group = "Trend Based Fibonacci Time")]
public double EleventhTrendBasedFibonacciTimePercent { get; set; }
[Parameter("11th Level Color", DefaultValue = "Chocolate", Group = "Trend Based Fibonacci Time")]
public Color EleventhTrendBasedFibonacciTimeColor { get; set; }
[Parameter("11th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Trend Based Fibonacci Time")]
public int EleventhTrendBasedFibonacciTimeThickness { get; set; }
[Parameter("11th Level Style", DefaultValue = LineStyle.Solid, Group = "Trend Based Fibonacci Time")]
public LineStyle EleventhTrendBasedFibonacciTimeStyle { get; set; }
#endregion Trend Based Fibonacci Time Parameters
#region Fibonacci Channel parameters
[Parameter("Show 1st Level", DefaultValue = true, Group = "Fibonacci Channel")]
public bool ShowFirstFibonacciChannel { get; set; }
[Parameter("Fill 1st Level", DefaultValue = true, Group = "Fibonacci Channel")]
public bool FillFirstFibonacciChannel { get; set; }
[Parameter("1st Level Percent", DefaultValue = 0, Group = "Fibonacci Channel")]
public double FirstFibonacciChannelPercent { get; set; }
[Parameter("1st Level Color", DefaultValue = "Gray", Group = "Fibonacci Channel")]
public Color FirstFibonacciChannelColor { get; set; }
[Parameter("1st Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Channel")]
public int FirstFibonacciChannelThickness { get; set; }
[Parameter("1st Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Channel")]
public LineStyle FirstFibonacciChannelStyle { get; set; }
[Parameter("Show 2nd Level", DefaultValue = true, Group = "Fibonacci Channel")]
public bool ShowSecondFibonacciChannel { get; set; }
[Parameter("Fill 2nd Level", DefaultValue = true, Group = "Fibonacci Channel")]
public bool FillSecondFibonacciChannel { get; set; }
[Parameter("2nd Level Percent", DefaultValue = 0.236, Group = "Fibonacci Channel")]
public double SecondFibonacciChannelPercent { get; set; }
[Parameter("2nd Level Color", DefaultValue = "Red", Group = "Fibonacci Channel")]
public Color SecondFibonacciChannelColor { get; set; }
[Parameter("2nd Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Channel")]
public int SecondFibonacciChannelThickness { get; set; }
[Parameter("2nd Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Channel")]
public LineStyle SecondFibonacciChannelStyle { get; set; }
[Parameter("Show 3rd Level", DefaultValue = true, Group = "Fibonacci Channel")]
public bool ShowThirdFibonacciChannel { get; set; }
[Parameter("Fill 3rd Level", DefaultValue = true, Group = "Fibonacci Channel")]
public bool FillThirdFibonacciChannel { get; set; }
[Parameter("3rd Level Percent", DefaultValue = 0.382, Group = "Fibonacci Channel")]
public double ThirdFibonacciChannelPercent { get; set; }
[Parameter("3rd Level Color", DefaultValue = "GreenYellow", Group = "Fibonacci Channel")]
public Color ThirdFibonacciChannelColor { get; set; }
[Parameter("3rd Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Channel")]
public int ThirdFibonacciChannelThickness { get; set; }
[Parameter("3rd Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Channel")]
public LineStyle ThirdFibonacciChannelStyle { get; set; }
[Parameter("Show 4th Level", DefaultValue = true, Group = "Fibonacci Channel")]
public bool ShowFourthFibonacciChannel { get; set; }
[Parameter("Fill 4th Level", DefaultValue = true, Group = "Fibonacci Channel")]
public bool FillFourthFibonacciChannel { get; set; }
[Parameter("4th Level Percent", DefaultValue = 0.5, Group = "Fibonacci Channel")]
public double FourthFibonacciChannelPercent { get; set; }
[Parameter("4th Level Color", DefaultValue = "DarkGreen", Group = "Fibonacci Channel")]
public Color FourthFibonacciChannelColor { get; set; }
[Parameter("4th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Channel")]
public int FourthFibonacciChannelThickness { get; set; }
[Parameter("4th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Channel")]
public LineStyle FourthFibonacciChannelStyle { get; set; }
[Parameter("Show 5th Level", DefaultValue = true, Group = "Fibonacci Channel")]
public bool ShowFifthFibonacciChannel { get; set; }
[Parameter("Fill 5th Level", DefaultValue = true, Group = "Fibonacci Channel")]
public bool FillFifthFibonacciChannel { get; set; }
[Parameter("5th Level Percent", DefaultValue = 0.618, Group = "Fibonacci Channel")]
public double FifthFibonacciChannelPercent { get; set; }
[Parameter("5th Level Color", DefaultValue = "BlueViolet", Group = "Fibonacci Channel")]
public Color FifthFibonacciChannelColor { get; set; }
[Parameter("5th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Channel")]
public int FifthFibonacciChannelThickness { get; set; }
[Parameter("5th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Channel")]
public LineStyle FifthFibonacciChannelStyle { get; set; }
[Parameter("Show 6th Level", DefaultValue = true, Group = "Fibonacci Channel")]
public bool ShowSixthFibonacciChannel { get; set; }
[Parameter("Fill 6th Level", DefaultValue = true, Group = "Fibonacci Channel")]
public bool FillSixthFibonacciChannel { get; set; }
[Parameter("6th Level Percent", DefaultValue = 0.786, Group = "Fibonacci Channel")]
public double SixthFibonacciChannelPercent { get; set; }
[Parameter("6th Level Color", DefaultValue = "AliceBlue", Group = "Fibonacci Channel")]
public Color SixthFibonacciChannelColor { get; set; }
[Parameter("6th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Channel")]
public int SixthFibonacciChannelThickness { get; set; }
[Parameter("6th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Channel")]
public LineStyle SixthFibonacciChannelStyle { get; set; }
[Parameter("Show 7th Level", DefaultValue = true, Group = "Fibonacci Channel")]
public bool ShowSeventhFibonacciChannel { get; set; }
[Parameter("Fill 7th Level", DefaultValue = true, Group = "Fibonacci Channel")]
public bool FillSeventhFibonacciChannel { get; set; }
[Parameter("7th Level Percent", DefaultValue = 1, Group = "Fibonacci Channel")]
public double SeventhFibonacciChannelPercent { get; set; }
[Parameter("7th Level Color", DefaultValue = "Bisque", Group = "Fibonacci Channel")]
public Color SeventhFibonacciChannelColor { get; set; }
[Parameter("7th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Channel")]
public int SeventhFibonacciChannelThickness { get; set; }
[Parameter("7th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Channel")]
public LineStyle SeventhFibonacciChannelStyle { get; set; }
[Parameter("Show 8th Level", DefaultValue = true, Group = "Fibonacci Channel")]
public bool ShowEighthFibonacciChannel { get; set; }
[Parameter("Fill 8th Level", DefaultValue = true, Group = "Fibonacci Channel")]
public bool FillEighthFibonacciChannel { get; set; }
[Parameter("8th Level Percent", DefaultValue = 1.618, Group = "Fibonacci Channel")]
public double EighthFibonacciChannelPercent { get; set; }
[Parameter("8th Level Color", DefaultValue = "Azure", Group = "Fibonacci Channel")]
public Color EighthFibonacciChannelColor { get; set; }
[Parameter("8th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Channel")]
public int EighthFibonacciChannelThickness { get; set; }
[Parameter("8th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Channel")]
public LineStyle EighthFibonacciChannelStyle { get; set; }
[Parameter("Show 9th Level", DefaultValue = true, Group = "Fibonacci Channel")]
public bool ShowNinthFibonacciChannel { get; set; }
[Parameter("Fill 9th Level", DefaultValue = true, Group = "Fibonacci Channel")]
public bool FillNinthFibonacciChannel { get; set; }
[Parameter("9th Level Percent", DefaultValue = 2.618, Group = "Fibonacci Channel")]
public double NinthFibonacciChannelPercent { get; set; }
[Parameter("9th Level Color", DefaultValue = "Aqua", Group = "Fibonacci Channel")]
public Color NinthFibonacciChannelColor { get; set; }
[Parameter("9th Level Thickness", DefaultValue = 1, MinValue = 0, Group = "Fibonacci Channel")]
public int NinthFibonacciChannelThickness { get; set; }
[Parameter("9th Level Style", DefaultValue = LineStyle.Solid, Group = "Fibonacci Channel")]
public LineStyle NinthFibonacciChannelStyle { get; set; }
[Parameter("Show 10th Level", DefaultValue = true, Group = "Fibonacci Channel")]
public bool ShowTenthFibonacciChannel { get; set; }
[Parameter("Fill 10th Level", DefaultValue = true, Group = "Fibonacci Channe
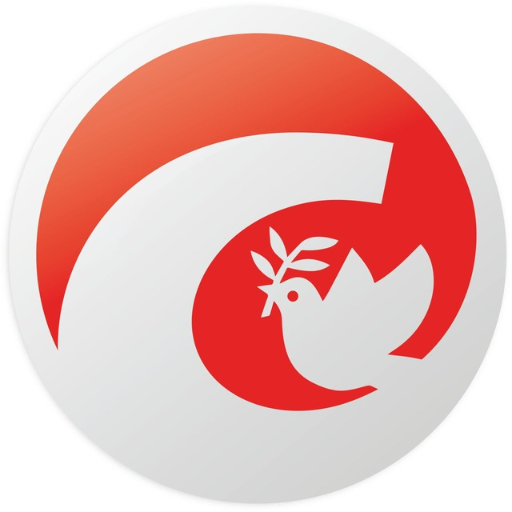
Spotware
Joined on 23.09.2013
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Fibonacci Drawing.algo
- Rating: 5
- Installs: 4107
- Modified: 26/01/2023 10:57
Comments
Hello, I downloaded and installed this indicator. I can see it in the "object manager / indicators " list but I see nothing on my chart.
Did I miss something ?
Best regards.
All the indicators are formed and challenging for the top of the norms. The way out for the change is fit for the fabrications. The issues of the best dissertation writing services reviews are marked for the joyful means for the good and normative means for the ideal path for the generations.
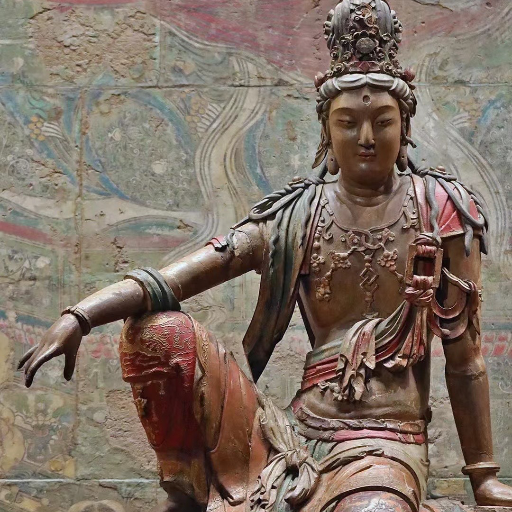
When I draw using the system comes with the cross cursor can not consider the baseline problem, but after using it automatically jumped back to the mouse arrow mode, so I hope that the next version of the update to increase the cross cursor dashed, so that any future indicators do not need to consider the baseline problem, and secondly, in the software level, if I choose the cross cursor mode, in I do not choose the mouse arrow mode do not automatically jump to the mouse arrow mode.
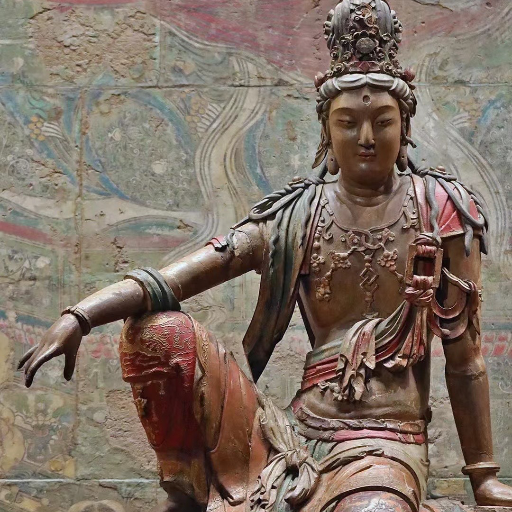
There is another problem, when using Fibonacci channel, if the first point is on the trend line it will show a cross cursor when it moves up. In fact, you can refer to the trendview, they draw the channel where the first point will not be selected, and they are cross cursor, there will be no baseline alignment problems.
What reference should be added for it co compile ? Thanks
Hello, I downloaded and installed this indicator. I can see it in the "object manager / indicators " list but I see nothing on my chart and also there´s no button that allows me to activate the indicator.
Did I miss something ?
Best regards.