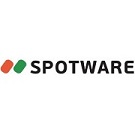
Topics
Forum Topics not found
Replies
cAlgo_Fanatic
29 Aug 2013, 17:46
We understand the need to set those levels freely. We are considering adding this for upcoming versions of cTrader.
@cAlgo_Fanatic
cAlgo_Fanatic
29 Aug 2013, 17:40
We are working on tick charts and more tabs. As far as the rest of the suggestions, we will evaluate them and consider them for implementation in the future.
@cAlgo_Fanatic
cAlgo_Fanatic
29 Aug 2013, 15:52
RE: RE:
coolutm said:
Support,
Thank you for the reply. This one works perfect.
Just one more question (or request), what if I want to set up different amount for the profit side and stop loss side?
I simply added one more if phrase....if (NetProfit <= -200) but didn't work.
Please help me.
if ( netProfit >= 300.0) || netProfit <= -200 )
@cAlgo_Fanatic
cAlgo_Fanatic
29 Aug 2013, 10:34
You can use a try...catch block to catch the exception.
@cAlgo_Fanatic
cAlgo_Fanatic
29 Aug 2013, 09:57
RE:
forexner12 said:
Please look in to the calculation of the break even function. most of the time when it hit the break even i have loose money due to miscalculation. I have set my break even after 10pips.
thanks
Please send us an email providing some information about the platform and account type you use (e.g. Spotware cAlgo, demo), the commissions and more details regarding the transaction to engage@spotware.com so that we can investigate this.
@cAlgo_Fanatic
cAlgo_Fanatic
29 Aug 2013, 09:28
You may switch "Auto Formatting" off from the Preferences in the top menu. Please send us the code or the code snippet to engage@spotware.com, or describe it a little further so that we can fix this.
@cAlgo_Fanatic
cAlgo_Fanatic
19 Aug 2013, 17:54
We've made a change: cAlgo will no longer restore samples, so one can remove them permanently.
@cAlgo_Fanatic
cAlgo_Fanatic
19 Aug 2013, 17:04
The sample robots and indicators are part of the API and cannot be removed permanently. We are planning to separate samples in a different tab in the future.
@cAlgo_Fanatic
cAlgo_Fanatic
19 Aug 2013, 13:01
Thank you for the feedback and kind words. We will seriously consider your proposals for upcoming versions of cTrader. We understand the need to set those levels freely.
@cAlgo_Fanatic
cAlgo_Fanatic
19 Aug 2013, 10:19
( Updated at: 23 Jan 2024, 13:14 )
We do not have belkhayate in the platform for the time being, you can use a custom indicator instead. The indicator [Belkhayate Polynomial Regression] has been uploaded by another user, it could be the one you are looking for. Custom indicators can be loaded from cTrader just as easily as build in indicators.
For more info see:http://help.spotware.com/calgo/custom-indicators
@cAlgo_Fanatic
cAlgo_Fanatic
19 Aug 2013, 10:06
You can use MarketDepth and the related methods/properties. See /api/marketdepth, /api/marketdepthentry
@cAlgo_Fanatic
cAlgo_Fanatic
16 Aug 2013, 16:04
( Updated at: 21 Dec 2023, 09:20 )
When the number circled in red, which is equal to the net profit minus the commission (23.16 -15 =11.16) will be greater than 300 or less than -300, then the position will close.
protected override void OnTick() { var netProfit = 0.0; foreach (var openedPosition in Account.Positions) { netProfit += openedPosition.NetProfit + openedPosition.Commissions; } ChartObjects.DrawText("a", netProfit.ToString(), StaticPosition.BottomLeft); if (Math.Abs(netProfit) >= 300.0) { foreach (var openedPosition in Account.Positions) { Trade.Close(openedPosition); } } }
@cAlgo_Fanatic
cAlgo_Fanatic
16 Aug 2013, 12:27
RE:
lostvik said:
Want a Compass on the charts
Can you please elaborate? Is this an indicator you are referring to?
@cAlgo_Fanatic
cAlgo_Fanatic
16 Aug 2013, 11:34
Most probably your mouse moves down to menu items and the editor looses focus. Keep the mouse above the menu for example. Let us know if this persists.
We will improve this functionality in the future releases.
@cAlgo_Fanatic
cAlgo_Fanatic
16 Aug 2013, 10:52
We will investigate this promptly. We apologize for the inconvenience and thank you for reporting it.
@cAlgo_Fanatic
cAlgo_Fanatic
13 Aug 2013, 17:53
RE:
citikot said:
Could You, please, clarify if my broker is Roboforex it means that I'm sending a pending order to them, they are sending my order to Spotware, where the order stored until criteria met?
Your order is sent directly to cTrader server, it is stored there. Then the server sends it to the liquidity provider.
@cAlgo_Fanatic
cAlgo_Fanatic
03 Sep 2013, 10:48
This is an example of a workaround to modifying a pending order's target price.
To be used as a guideline to build your own Robot.
Input Parameters:
OnStart:
OnTick: The robot stops if the pending order field is null (The order has been successfully deleted or not found).
@cAlgo_Fanatic