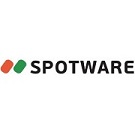
Topics
Forum Topics not found
Replies
cAlgo_Fanatic
26 Jun 2013, 12:17
cTrader Web will work on IE10 soon. We also have plans for improving the magnification as well as linking charts together. Stay tuned.
@cAlgo_Fanatic
cAlgo_Fanatic
26 Jun 2013, 12:07
It will not work for backtesting. You can test it only by running it live with a demo account, that is why it is suggested to use a small interval for the timed event.
The code seems ok at first glance, please confirm that the robot is being tested live.
Also note that if the computer to goes to sleep it will also not work so check the power settings.
@cAlgo_Fanatic
cAlgo_Fanatic
26 Jun 2013, 11:47
Yes, see the example here: ModifyPosition
We will make a modified version and upload it to the Robots section. Just to be clear on the definition of this, setting take profit to a position will close the position once the price reaches that level, that is, according to the definition of the algorithm, if it has not already been closed, by an opposite trade having been generated.
@cAlgo_Fanatic
cAlgo_Fanatic
24 Jun 2013, 16:50
Dear Trader,
Trading strategies are rarely successful for all symbols, timeframes and other input parameters. They are usually intended for one set of those and developers usually post that in the descriptions of those Robots. If this information is not provided you may leave a comment to ask the developers of those Robots to provide it. Alternatively, you can optimize them yourself as well as experiment with the code. If you need help with the cAlgo API we are always here to help.
Best Regards
@cAlgo_Fanatic
cAlgo_Fanatic
24 Jun 2013, 14:30
The following code maybe what you need or point you in the right direction. We will investigate any issues regarding duplicate emails sent.
The logic of the following code is that it keeps a list of all the positions in the account which is updated on each tick.
If a position that existed in the previous tick is not found an email is sent and the position is removed from the list.
// ------------------------------------------------------------------------------- // // This is a Template used as a guideline to build your own Robot. // Please use the “Feedback” tab to provide us with your suggestions about cAlgo’s API. // // The logic of the following code is that it keeps a list of all the positions in the account // which is updated on each tick. // If a position that existed in the previous tick is not found an email is sent with the balance info // and the position is removed from the list. // // ------------------------------------------------------------------------------- using System; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Requests; using cAlgo.Indicators; using System.Collections.Generic; using System.Linq; namespace cAlgo.Robots { [Robot] public class NewRobot : Robot { private List<Position> _listPosition = new List<Position>(); private List<Position> _listPositionToBeRemoved = new List<Position>(); protected override void OnStart() { foreach (Position pos in Account.Positions) _listPosition.Add(pos); } protected override void OnTick() { // Put your core logic here foreach (var position in _listPosition) { if (Account.Positions.Contains(position)) continue; Notifications.SendEmail("...", "...", "Position Closed", Account.Balance.ToString()); _listPositionToBeRemoved.Add(position); } UpdateList(); } private void UpdateList() { foreach (var position in _listPositionToBeRemoved) _listPosition.Remove(position); foreach (var position in Account.Positions) { if (_listPosition.Contains(position)) continue; _listPosition.Add(position); } } protected override void OnStop() { // Put your deinitialization logic here } } }
@cAlgo_Fanatic
cAlgo_Fanatic
24 Jun 2013, 12:12
Hello,
We will consider adding a default sound file into the API. In the meantime:
It seems that you may be trying to access files in a folder other than the one used in the indicator you downloaded.
To confirm please modify the code to the sample code below so that you will be providing the full path.
Notifications.PlaySound(@"C:\SampleDestination\SampleSound.mp3"); // for instance with the above example it would be: Notifications.PlaySound(@"C:\Windows\Media\tada.wav");
What other issues are you having with cTrader? We appreciate and always take user feedback into consideration.
@cAlgo_Fanatic
cAlgo_Fanatic
24 Jun 2013, 11:02
Please restart your pc and try reinstalling. If it fails again, send us an email at engage@spotware.com.
@cAlgo_Fanatic
cAlgo_Fanatic
24 Jun 2013, 09:53
Hello,
You cannot run indicators or robots from Visual Studio. You need to use cAlgo or Ctrader (indicators only) to view the results.
@cAlgo_Fanatic
cAlgo_Fanatic
21 Jun 2013, 17:04
( Updated at: 21 Dec 2023, 09:20 )
This feature has been added to the platform. Thank you for your feedback.
@cAlgo_Fanatic
cAlgo_Fanatic
21 Jun 2013, 09:54
Did you try adding the notification in the OnPositionClosed event?
protected override void OnPositionClosed(Position position) { var balance = Account.Balance; Print("{0}", balance); Notifications.SendEmail("from_email@somedomain.com", "to_email@somedomain.com", "Position Closed", Account.Balance.ToString()); }
@cAlgo_Fanatic
cAlgo_Fanatic
19 Jun 2013, 14:43
Ports 80 and 443.
We need some more information to investigate this.
Does cTrader function properly or do both cTrader and cAlgo have a problem?
@cAlgo_Fanatic
cAlgo_Fanatic
19 Jun 2013, 10:09
There are no plans currently to support OSX or create a cAlgo web version. You may however use cAlgo with a virual PC.
@cAlgo_Fanatic
cAlgo_Fanatic
18 Jun 2013, 14:22
Do you mean the robot should not execute any trades until there is one open position?
@cAlgo_Fanatic
cAlgo_Fanatic
18 Jun 2013, 10:55
You can calculate the net profit (double) for each label like so:
foreach (var pos in Account.Positions) { if (pos.Label == "123") netProfit += pos.NetProfit; }
or using a Linq expression:
var netProfit = Account.Positions.Where(pos => pos.Label == "123").Sum(pos => pos.NetProfit);
You can assign that each robot has a unique label or you may check which is the position in the account by the symbol instead of the label:
foreach (var pos in Account.Positions) { if (pos.SymbolCode == "EURUSD") netProfit += pos.NetProfit; }
The net profit is equal to the equity - balance.
@cAlgo_Fanatic
cAlgo_Fanatic
18 Jun 2013, 10:18
Are you using using a firewall or caching http proxy?
@cAlgo_Fanatic
cAlgo_Fanatic
18 Jun 2013, 10:08
This will be available in the next release, in approximately one week.
@cAlgo_Fanatic
cAlgo_Fanatic
17 Jun 2013, 17:02
We will consider adding this in the future. Thank you for the suggestion.
@cAlgo_Fanatic
cAlgo_Fanatic
26 Jun 2013, 12:48
1. There is an extra curly bracket which will be a reason the program will not build here:
Remove that "}" from the start of the line.
2. The getVolume property is not being used only defined.
One way to use it is by adding this line prior to creating the request object:
right before this line for instance:
The getVolume property will return a negative number if SL is not set (if SL = 0) . So, either modify the code or initialize SL to a value greater than zero.
Usefull C# tutorial: http://www.csharp-station.com/Tutorial.aspx
@cAlgo_Fanatic