Description
This indicator is commonly used to confirm market trends and generate buy/sell signals.
The main advantage of a smoothed moving average is that it removes short-term fluctuations, and allows us to view the price trends much easier, which is why they are widely used in trending markets.
Definitions
The Smoothed Moving Average (SMMA) is a combination of an SMA and an EMA. It gives the recent prices an equal weighting as the historic prices as it takes all available price data into account.
Calculations
SMMA0 = SMA
SMMAn = (SMMAn-1 * (Length - 1) + Source) / Length
where SMA = Simple Moving Average, SMMA = Smoothed Moving Average
SMMA0 = First SMMA, SMMAn = Current SMMA, SMMAn-1 = Previous SMMA
Parameters
- Source - Data Source, e.g. Close, High …
- Length - Smoothing Period
Outputs
- SMMA - Smoothed Moving Average line
Chartshot
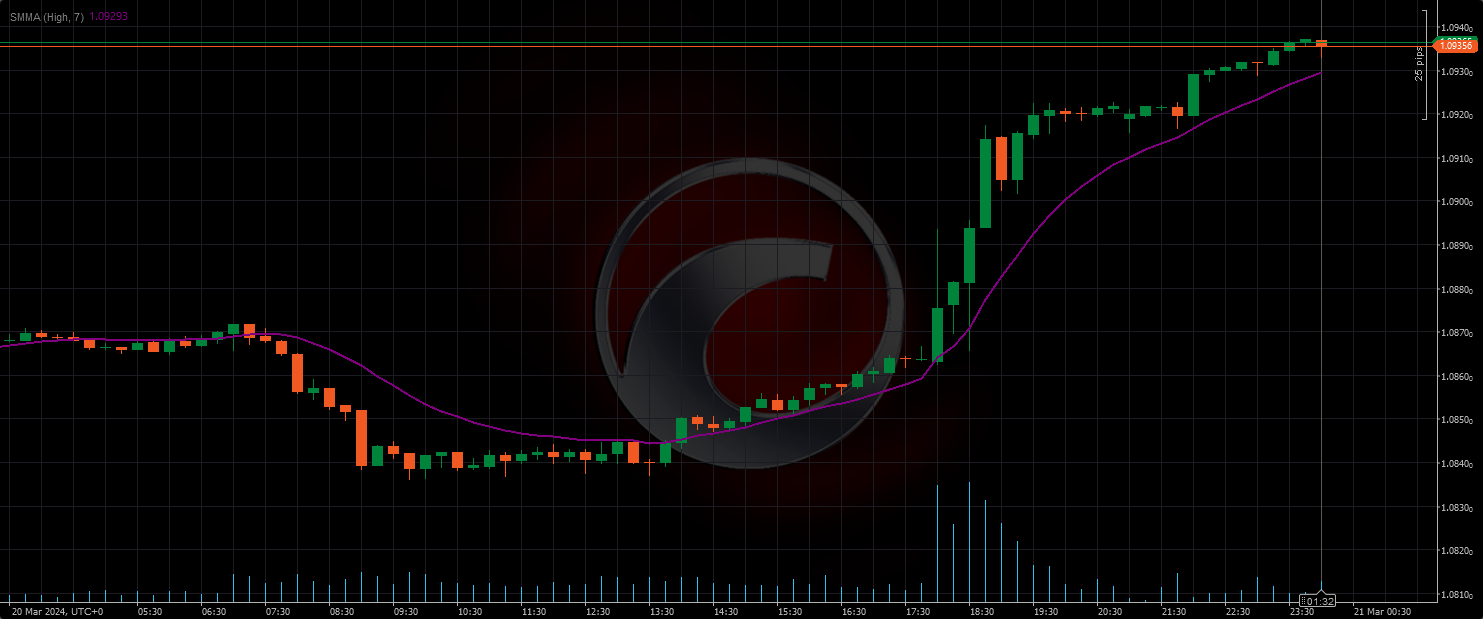
soskrr
Joined on 20.08.2023
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: SMMA.algo
- Rating: 0
- Installs: 769
- Modified: 21/03/2024 00:42
To whom it may concern