Description
Quicksort is an efficient sorting algorithm based on the divide-and-conquer technique. This algorithm sorts an array by partitioning it. Here are some of the main steps of quicksort:
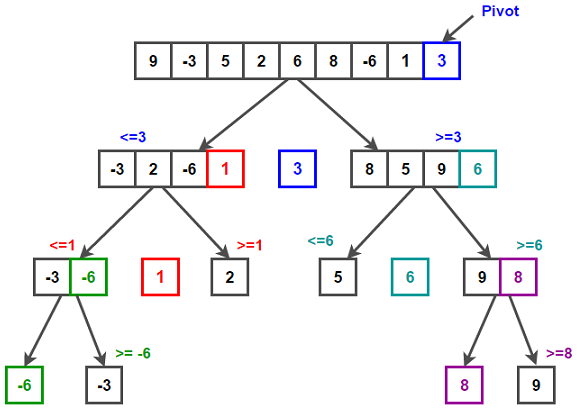
Pivot Selection:
- The most important step in QuickSort is selecting the pivot element. An axis is an element that divides the array into two parts.
- The middle element is often selected as the pivot, but some implementations use random or other methods.
Division:
- After selecting the pivot, the array is divided into two parts, one smaller than the pivot and the other larger than the pivot.
- Partitioning uses pointers, one that points to the beginning of the array and the other that points to the last element of the array.
- The elements have to be arranged in the form of an axis.
Reversion:
- Now the array has been divided into two parts. QuickSort is applied recursively to both parts.
- Each part is divided into two parts by selecting a pivot, which is repeated until the array is sorted.
Combine:
- When each part is sorted, they are merged, and the entire array is sorted.
The time complexity of QuickSort is O(n log n) in the average case, but can also be O(n^2) in the worst-case scenario, in which the pivot is always the minimum or maximum value of the array. But, its average performance is very high and it is usually used in sorting problems.
https://www.geeksforgeeks.org/quick-sort/
Thanks
Best Regards
Salesforce Developer Training
@rajatsharma0918
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
// https://ctrader.com/users/profile/64575
namespace cAlgo.Indicators
{
[Cloud("CCI", "CCI 2", FirstColor = "Blue", Opacity = 0.15, SecondColor = "Red")]
[Indicator(IsOverlay = false, AccessRights = AccessRights.None)]
public class CCiTrend : Indicator
{
[Output("CCI", LineColor = "Black")]
public IndicatorDataSeries CCIa { get; set; }
[Output("CCI 2", LineColor = "Black")]
public IndicatorDataSeries CCIb { get; set; }
[Output("Zero Line", Color = Colors.Black, LineStyle = LineStyle.Dots)]
public IndicatorDataSeries ZeroLine { get; set; }
[Output("Level +100", Color = Colors.Black, LineStyle = LineStyle.DotsRare)]
public IndicatorDataSeries LevelPlus200 { get; set; }
[Output("Level -100", Color = Colors.Black, LineStyle = LineStyle.DotsRare)]
public IndicatorDataSeries LevelMinus200 { get; set; }
[Parameter("CCi Period 1", DefaultValue = 21)]
public int Period { get; set; }
[Parameter("CCi Period 2", DefaultValue = 48)]
public int Period2 { get; set; }
private CommodityChannelIndex cci;
private CommodityChannelIndex cci2;
protected override void Initialize()
{
cci = Indicators.CommodityChannelIndex(Period);
cci2 = Indicators.CommodityChannelIndex(Period2);
}
public override void Calculate(int index)
{
CCIa[index] = cci.Result[index];
CCIb[index] = cci2.Result[index];
ZeroLine[index] = 0;
LevelPlus200[index] = 200;
LevelMinus200[index] = -200;
}
}
}
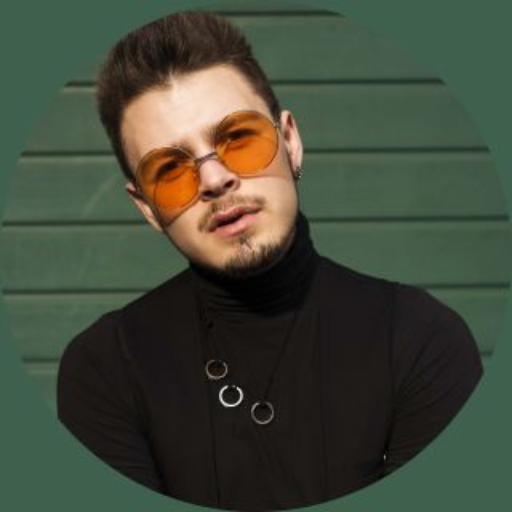
rajatsharma0918
Joined on 16.12.2023
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: ig.algo
- Rating: 0
- Installs: 478
- Modified: 16/12/2023 08:13
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
argh… again, more clutter and non cTrader targetted rubbish…
moderators, please occasionally do a bit of erm, moderation. the uploads area is becoming a sh!tfest of rubbish due to a complete lack of oversight and supervised moderation.