An update for this algorithm is currently pending moderation. Please revisit this page shortly to access the algorithm's latest version.
Description
The Trend Continuation Factor (TCF) indicator was developed to assist in identifying the market's trend and direction. It was introduced by M.H. Pee in an article titled 'Trend Continuation Factor' in the Stocks & Commodities magazine.
One common strategy for using this indicator involves seeking confluence across four periods (e.g., 20, 50, 100, 200).
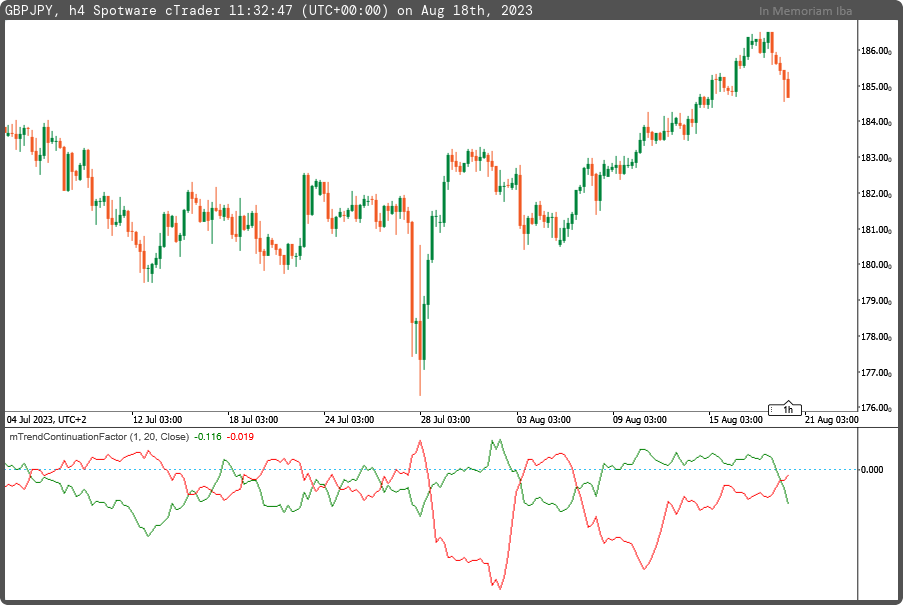
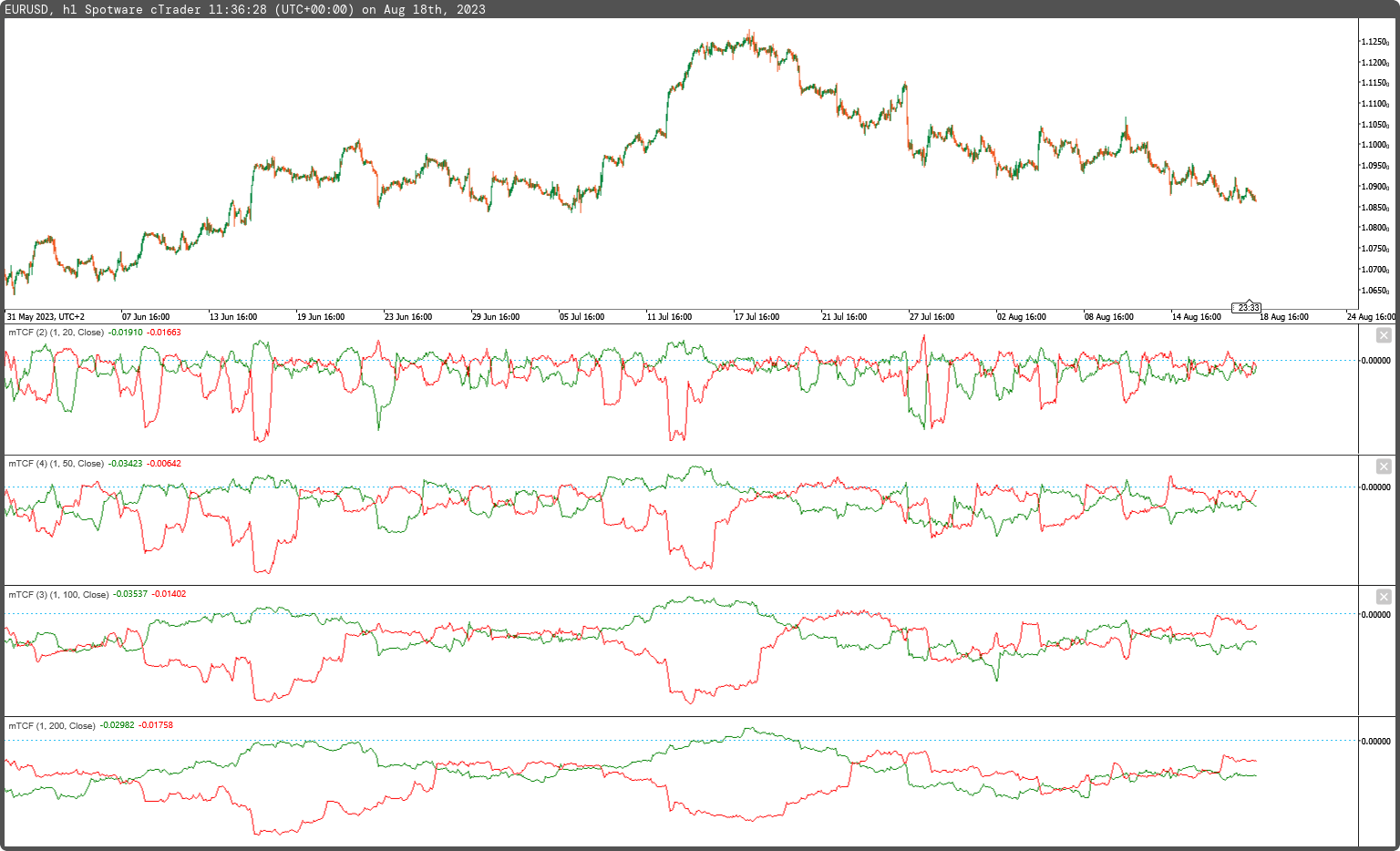
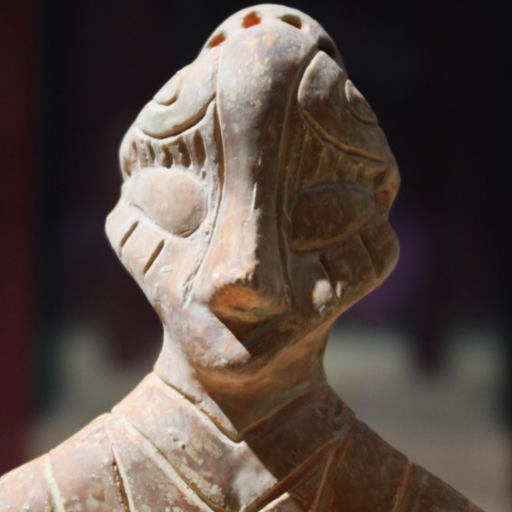
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mTrendContinuationFactor.algo
- Rating: 5
- Installs: 441
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
MF
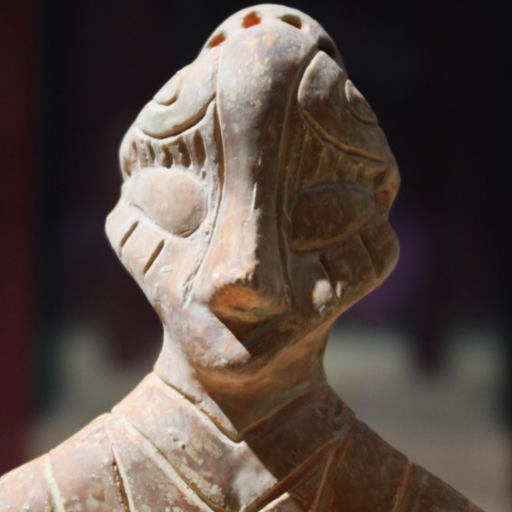
mfejza
·
10 months ago