An update for this algorithm is currently pending moderation. Please revisit this page shortly to access the algorithm's latest version.
Description
The Volume Price Momentum Oscillator combines volume and price momentum.
You can use this indicator as a measure of trading sentiment: go Long when above the bands, and go Short when below the bands.
You can also find the Compare Price Momentum Oscillator at the following link: https://ctrader.com/algos/indicators/show/3561
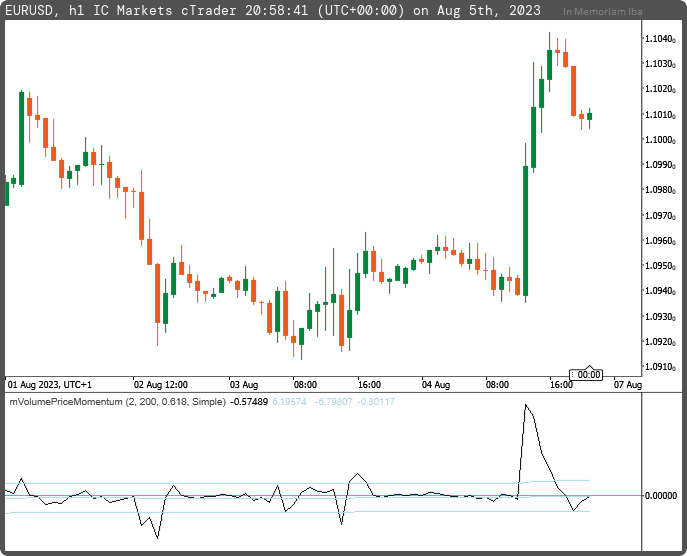
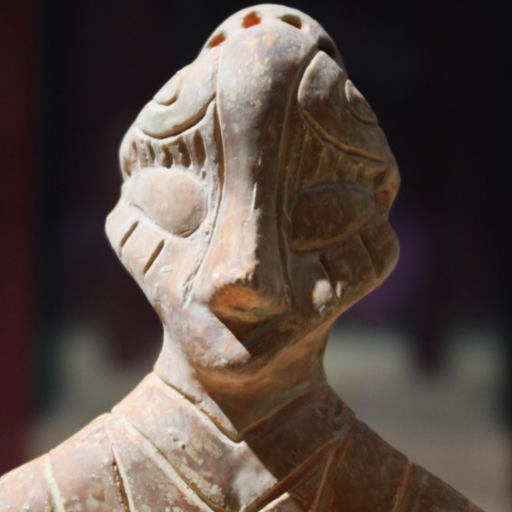
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mVolumePriceMomentum.algo
- Rating: 5
- Installs: 462
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
MF
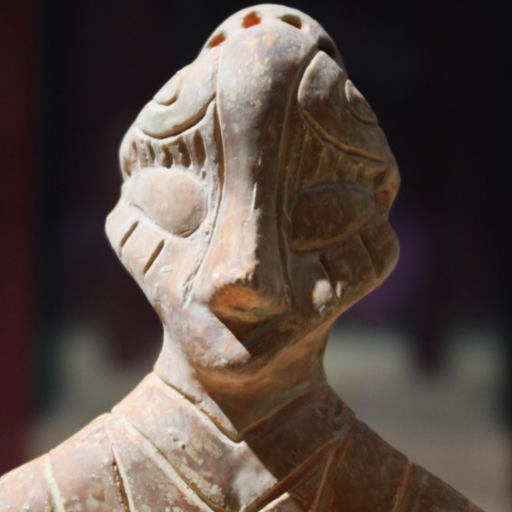
mfejza
·
10 months ago