Warning! This section will be deprecated on February 1st 2025. Please move all your Indicators to the cTrader Store catalogue.
An update for this algorithm is currently pending moderation. Please revisit this page shortly to access the algorithm's latest version.
Description
The Detrended Price Oscillator (DPO) is a well-known market sentiment indicator, displaying values above (bullish) and below (bearish) the zero level. This version of the custom indicator colors the values after smoothing DPO with the FIR method, indicating confident market conditions as:
- Very bullish: Above zero, colored green.
- Weak bullish: Above zero, colored red.
- Very bearish: Below zero, colored red.
- Weak bearish: Below zero, colored green.
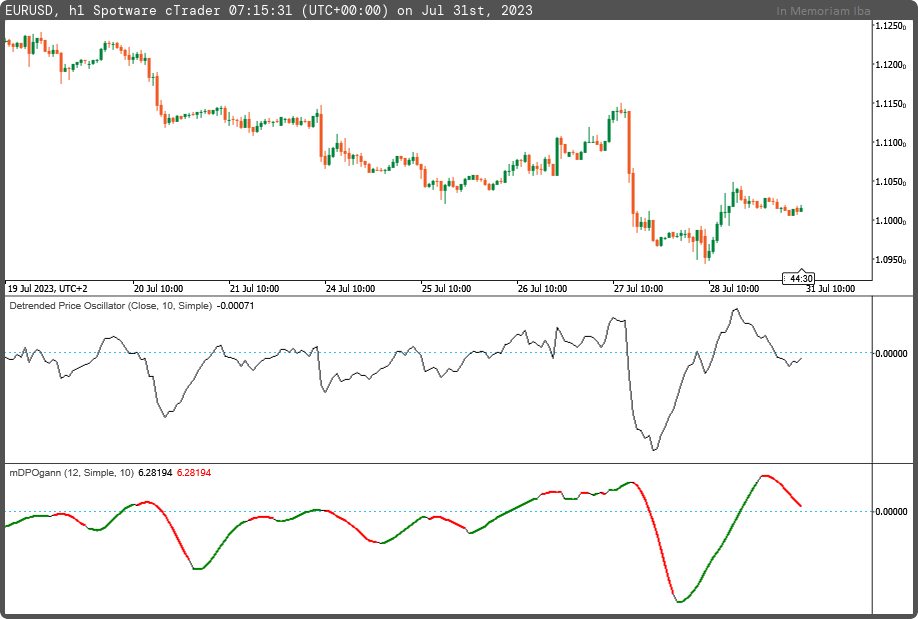
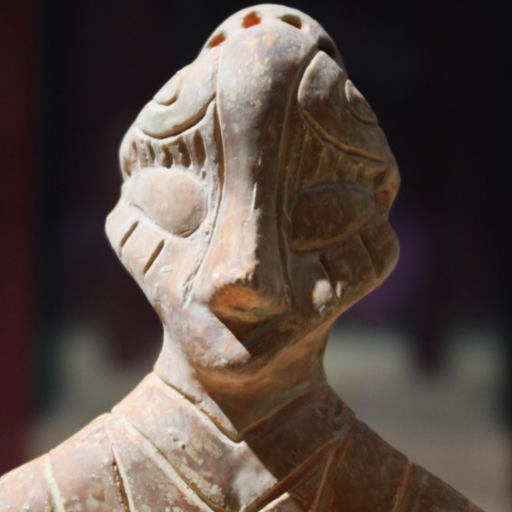
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mDPOgann.algo
- Rating: 5
- Installs: 605
- Modified: 31/07/2023 07:22
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
MF
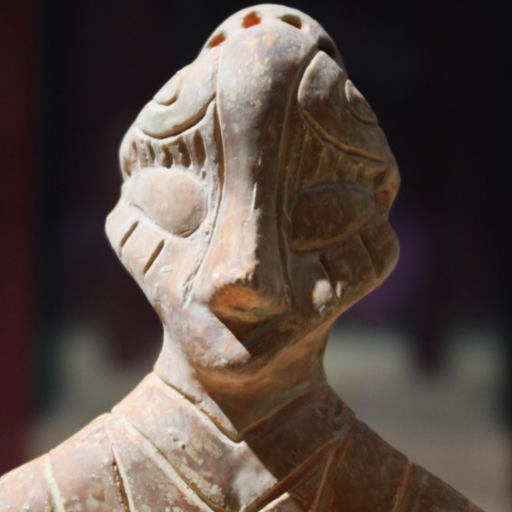
mfejza
·
1 year ago