Description
The term polarized fractal efficiency (PFE) refers to a technical indicator that is used to determine the price efficiency for an investment over a user-defined period. It fluctuates between -100 and 100 where the centerline is at zero. Traders can use the PFE to see where a security they're interested in is headed. The price shows an upward trend if the PFE goes above zero and a downward trend if it drops below zero. The indicator was developed by author, engineer, programmer, and trader Hans Hannula.
The polarized fractal efficiency indicator was developed by author, engineer, programmer, and trader Hans Hannula. The idea was first mentioned in 1994 in the January issue of Technical Analysis of Stocks & Commodities magazine. It fluctuates between -100 and +100, with zero as the centerline. Securities with a PFE greater than zero trend up, while a reading of less than zero indicates a downward trend.
The PFE indicator measures the strength of a trend by its position relative to the zero line. As a general rule, the further the PFE value is away from zero, the stronger and more efficient the given trend is. A PFE value that fluctuates around the zero line could indicate that the supply and demand for the security are in balance and the price may trade sideways.
Strategies that generally use the PFE as a signal consider a buy sign as a reversal in the direction of the indicator and its movement from its minimum value to zero. A signal to close a position arises as the value of the indicator reaches its peak above zero. An indicator shift from peak to zero presents a sell signal. As a rule of thumb, traders should buy to cover all short positions after the indicator forms a new minimum.
In this link you can find the indicator as oscillator indicator: https://ctrader.com/algos/indicators/show/3529
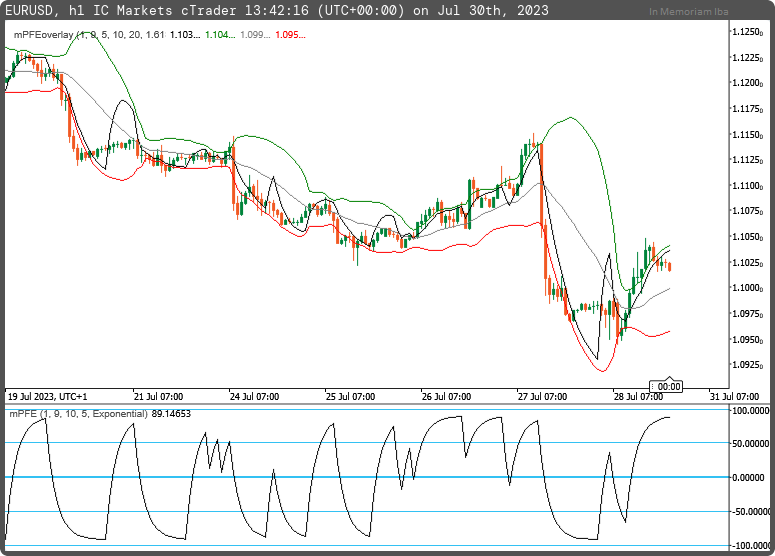
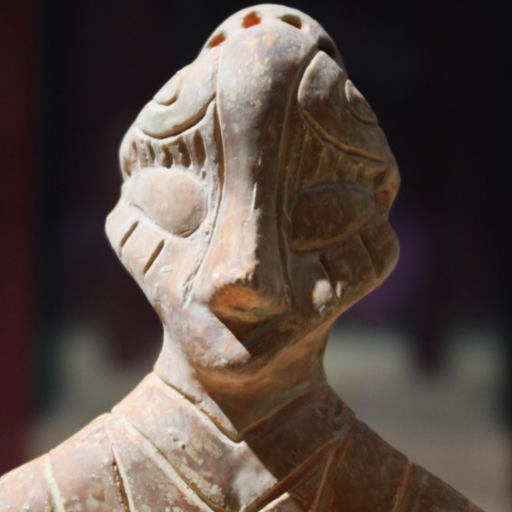
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mPFEoverlay.algo
- Rating: 5
- Installs: 346
Comments
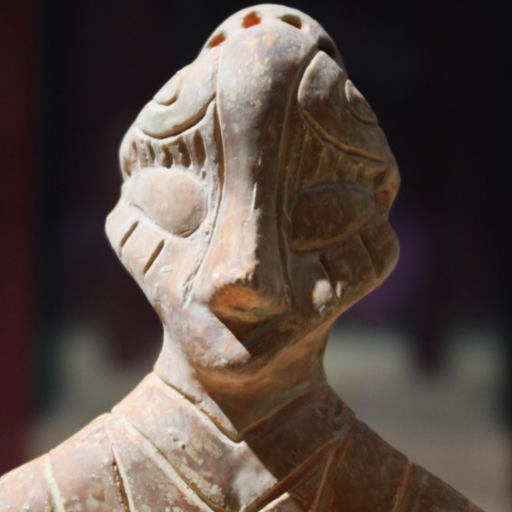