An update for this algorithm is currently pending moderation. Please revisit this page shortly to access the algorithm's latest version.
Description
The Wave Segregation Index (WSI) oscillator is based on the ratio of two indicators: DMS (Directional Movement System) and CCI (Commodity Channel Index), both in confluence with Typical Price.
The result is displayed as a colored histogram, indicating the strength of price wave segregation in four types of conditions.
This version is the common interpretation, attempting to assess the force and velocity of price.
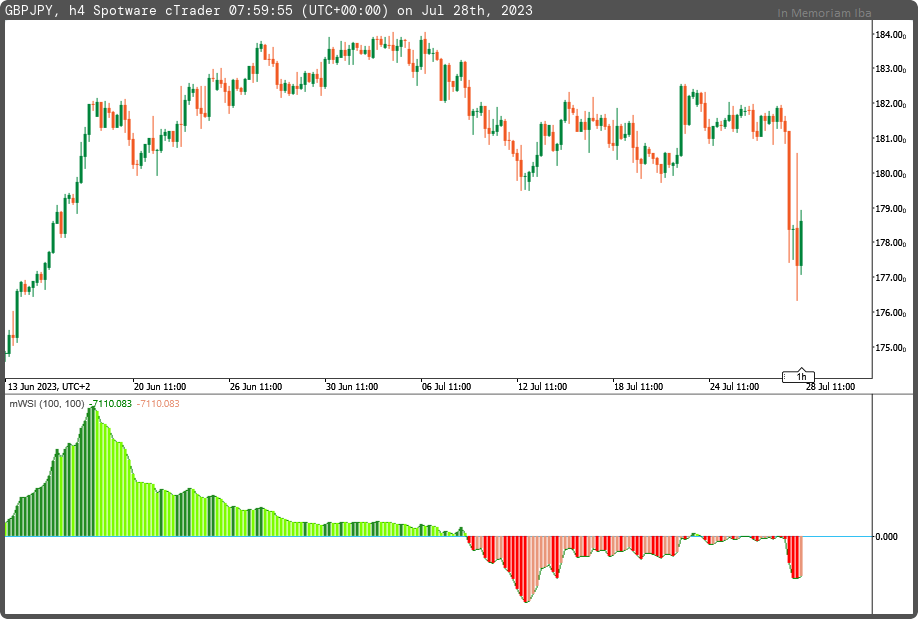
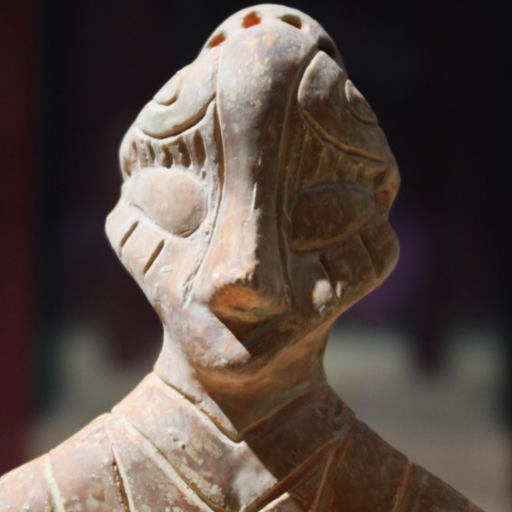
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mWSI.algo
- Rating: 5
- Installs: 530
- Modified: 28/07/2023 08:02
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
MF
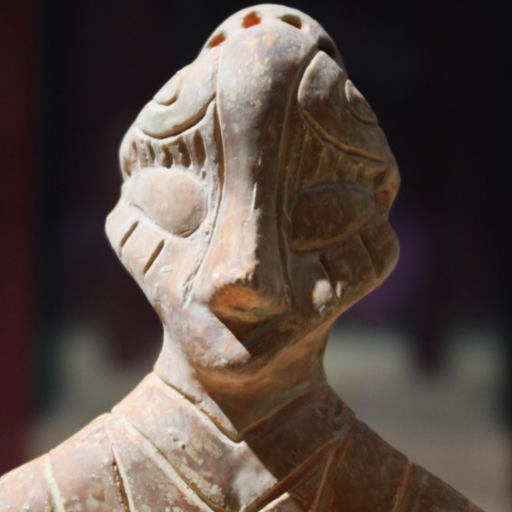
mfejza
·
1 year ago