Description
The Fast Adaptive Trend Line (FATL) is calculated using a low-frequency digital filter (FLF-1).
The FLF-1 filter is necessary for suppressing high-frequency noises and reducing market cycles with very short volatility periods, which can also be considered as noise.
The FLF-1 and FLF-2 filters for low frequencies provide attenuation in the stop band of no less than 40 dB and do not distort the amplitude and phase of the entry discontinuous price series in the pass band (bandwidth). These properties of the digital filters significantly improve noise suppression compared to a simple moving average, thereby sharply reducing the probability of false buy and sell signals.
There are no analogues to FATL among widely known technical instruments. It is not a moving average, but rather adaptive line estimates of short-term trends. Unlike a moving average, FATL does not have any phase delay with respect to current prices.
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Indicator(IsOverlay = true, AccessRights = AccessRights.None)]
public class mFATL : Indicator
{
[Parameter("Data Source (close)", DefaultValue = enumPriceTypes.Close)]
public enumPriceTypes inpPriceType { get; set; }
[Output("FATL", LineColor = "Black", PlotType = PlotType.Line, Thickness = 2)]
public IndicatorDataSeries outFATL { get; set; }
private IndicatorDataSeries _price, _fatl;
protected override void Initialize()
{
_price = CreateDataSeries();
_fatl = CreateDataSeries();
}
public override void Calculate(int i)
{
switch(inpPriceType)
{
case enumPriceTypes.Open:
_price[i] = Bars.OpenPrices[i];
break;
case enumPriceTypes.Close:
_price[i] = Bars.ClosePrices[i];
break;
case enumPriceTypes.High:
_price[i] = Bars.HighPrices[i];
break;
case enumPriceTypes.Low:
_price[i] = Bars.LowPrices[i];
break;
case enumPriceTypes.Median:
_price[i] = Bars.MedianPrices[i];
break;
case enumPriceTypes.Typical:
_price[i] = Bars.TypicalPrices[i];
break;
case enumPriceTypes.Weighted:
_price[i] = Bars.WeightedPrices[i];
break;
default:
_price[i] = Bars.ClosePrices[i];
break;
}
_fatl[i] = i>38 ?
0.4360409450 * _price[i]
+ 0.3658689069 * _price[i-1]
+ 0.2460452079 * _price[i-2]
+ 0.1104506886 * _price[i-3]
- 0.0054034585 * _price[i-4]
- 0.0760367731 * _price[i-5]
- 0.0933058722 * _price[i-6]
- 0.0670110374 * _price[i-7]
- 0.0190795053 * _price[i-8]
+ 0.0259609206 * _price[i-9]
+ 0.0502044896 * _price[i-10]
+ 0.0477818607 * _price[i-11]
+ 0.0249252327 * _price[i-12]
- 0.0047706151 * _price[i-13]
- 0.0272432537 * _price[i-14]
- 0.0338917071 * _price[i-15]
- 0.0244141482 * _price[i-16]
- 0.0055774838 * _price[i-17]
+ 0.0128149838 * _price[i-18]
+ 0.0226522218 * _price[i-19]
+ 0.0208778257 * _price[i-20]
+ 0.0100299086 * _price[i-21]
- 0.0036771622 * _price[i-22]
- 0.0136744850 * _price[i-23]
- 0.0160483392 * _price[i-24]
- 0.0108597376 * _price[i-25]
- 0.0016060704 * _price[i-26]
+ 0.0069480557 * _price[i-27]
+ 0.0110573605 * _price[i-28]
+ 0.0095711419 * _price[i-29]
+ 0.0040444064 * _price[i-30]
- 0.0023824623 * _price[i-31]
- 0.0067093714 * _price[i-32]
- 0.0072003400 * _price[i-33]
- 0.0047717710 * _price[i-34]
+ 0.0005541115 * _price[i-35]
+ 0.0007860160 * _price[i-36]
+ 0.0130129076 * _price[i-37]
+ 0.0040364019 * _price[i-38]
: _price[i];
outFATL[i] = _fatl[i];
}
}
public enum enumPriceTypes
{
Open,
Close,
High,
Low,
Median,
Typical,
Weighted
}
}
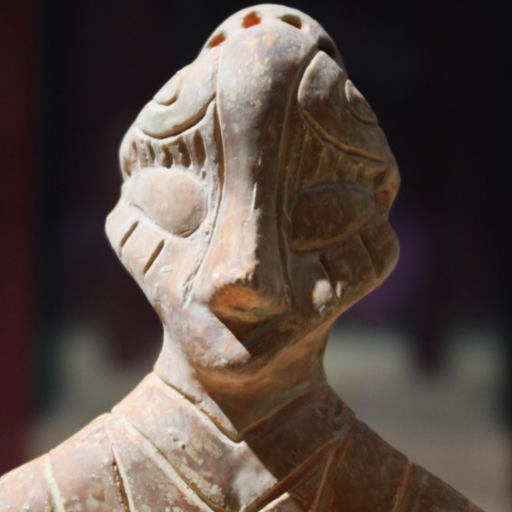
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mFATL.algo
- Rating: 5
- Installs: 497
- Modified: 05/06/2023 19:56
The market is difficult to predict
https://ctrader.com/burrito craft/algos/indicators/show/2735