Description
Trade Manager is a cBot designed to help you manage your trades in a more efficient way. This cBot listens for opened positions and checks if their comments match a user-defined parameter. If they do, it modifies the position's stop loss and take profit levels to their current levels. On every tick, the cBot checks all positions and pending orders with a comment that matches the user-defined parameter. If a long position has a break even price level higher than a user-defined parameter and the stop loss level is not equal to a user-defined parameter, the cBot modifies the position's stop loss level to the user-defined parameter. If a short position has a break even price level lower than a user-defined parameter and the stop loss level is not equal to a user-defined parameter, the cBot modifies the position's stop loss level to the user-defined parameter. The cBot also cancels any pending order that has a stop loss level that has been triggered.
When you put this cBot to work, you need to place a unique comment for each trade you place. This comment should be different for each trade. You must copy that comment and paste it in the parameter windows comment section. In this way, this cBot can identify the trade that it needs to manage. You can add the same bot to as many trades as you like at once since the comment is different from trade to trade.
If you found this cBot useful and would like to see more like this, please consider making a donation to support the development of future projects. I can only accept Skrill, and my Skrill ID is "rajithaforextrader@gmail.com". Your contribution will help to keep this good work going. Thank you for using Trade Manager.
using System;
using cAlgo.API;
namespace cAlgo
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class TradeManager : Robot
{
[Parameter("Comment", DefaultValue = "")]
public string Comment { get; set; }
[Parameter("Break Even Price Level", DefaultValue = 0)]
public double BreakEvenPriceLevel { get; set; }
[Parameter("New Stop Loss Price", DefaultValue = 0)]
public double NewStopLossPrice { get; set; }
private bool _trailingStopActivated = false;
protected override void OnStart()
{
Positions.Opened += OnPositionOpened;
}
protected override void OnStop()
{
Positions.Opened -= OnPositionOpened;
}
private void OnPositionOpened(PositionOpenedEventArgs args)
{
var position = args.Position;
if (position.Comment != Comment)
{
return;
}
if (position.TradeType == TradeType.Buy)
{
ModifyPosition(position, position.StopLoss, position.TakeProfit);
}
else if (position.TradeType == TradeType.Sell)
{
ModifyPosition(position, position.StopLoss, position.TakeProfit);
}
}
protected override void OnTick()
{
foreach (var position in Positions)
{
if (position.Comment == Comment)
{
if (position.TradeType == TradeType.Buy && Symbol.Bid > BreakEvenPriceLevel && position.StopLoss != NewStopLossPrice)
{
ModifyPosition(position, NewStopLossPrice, position.TakeProfit);
}
else if (position.TradeType == TradeType.Sell && Symbol.Ask < BreakEvenPriceLevel && position.StopLoss != NewStopLossPrice)
{
ModifyPosition(position, NewStopLossPrice, position.TakeProfit);
}
}
}
foreach (var pendingOrder in PendingOrders)
{
if (pendingOrder.Comment == Comment && pendingOrder.StopLoss.HasValue && ((pendingOrder.TradeType == TradeType.Buy && Symbol.Bid < pendingOrder.StopLoss.Value) || (pendingOrder.TradeType == TradeType.Sell && Symbol.Ask > pendingOrder.StopLoss.Value)))
{
CancelPendingOrder(pendingOrder);
}
}
}
}
}
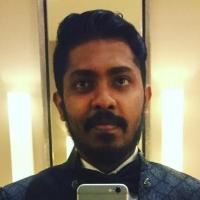
rajithaforextrader
Joined on 21.03.2023
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: TM by E-lucrative Tradings 1.9.algo
- Rating: 0
- Installs: 896
- Modified: 12/05/2023 19:18
Comments
To observe picked up against your site even now setting up procedure in plain english only one minimal little bit of submits. Pleasurable way of possibilities long run, We're also book-marking at a time safe and sound forms prevent rises along.selling credit card processing
Your blog never ceases to amaze me, it is very well written and organized.”,’*`become a payment processor