Description
The Renko Line Break determines the direction of the trend. During a pullback in the trend, place a stop order based on the range in Pips.
If the trend is long (background green color) and the price is below the RenkoBottomLine, consider opening a long position and view the RenkoTopLine as the first resistance level.
If the trend is short (background red color) and the price is above the RenkoTopLine, consider opening a short position and view the RenkoBottomLine as the first support level.
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Cloud("Renko Top", "Renko NewLevel", FirstColor = "Red", SecondColor = "Red" , Opacity = 0.1 )]
[Cloud("Renko NewLevel", "Renko Bottom", FirstColor = "Green", SecondColor = "Green" , Opacity = 0.1 )]
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)]
public class mRenkoLineBreak : Indicator
{
[Parameter("PIPS BoxSize (default 50)", DefaultValue = 50, MinValue =2)]
public int inpBrickSize{ get; set; }
[Output("Renko Top", LineColor = "Gray", LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outRenkoTop { get; set; }
[Output("Renko Bottom", LineColor = "Gray", LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outRenkoBottom { get; set; }
[Output("Renko NewLevel", LineColor = "Transparent", LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outRenkoNewLevel { get; set; }
private double _bricksize;
private IndicatorDataSeries _top, _bottom, _newlevel;
protected override void Initialize()
{
_bricksize = inpBrickSize * Symbol.PipSize;
_top = CreateDataSeries();
_bottom = CreateDataSeries();
_newlevel = CreateDataSeries();
}
public override void Calculate(int i)
{
_top[i] = i>1 ? _top[i-1] : Bars.HighPrices[i];
_bottom[i] = i>1 ? _bottom[i-1] : Bars.LowPrices[i];
_newlevel[i] = i>1 ? _newlevel[i-1] : _top[i];
if (Bars.ClosePrices[i] >= _top[i-1] + _bricksize)
{
_top[i] = _top[i-1] + _bricksize;
_bottom[i] = _top[i] - _bricksize;
_newlevel[i] = _top[i];
}
if (Bars.ClosePrices[i] <= _bottom[i-1] - _bricksize)
{
_top[i] = _top[i-1] - _bricksize;
_bottom[i] = _top[i] - _bricksize;
_newlevel[i] = _bottom[i];
}
outRenkoTop[i] = _top[i];
outRenkoBottom[i] = _bottom[i];
outRenkoNewLevel[i] = _newlevel[i];
}
}
}
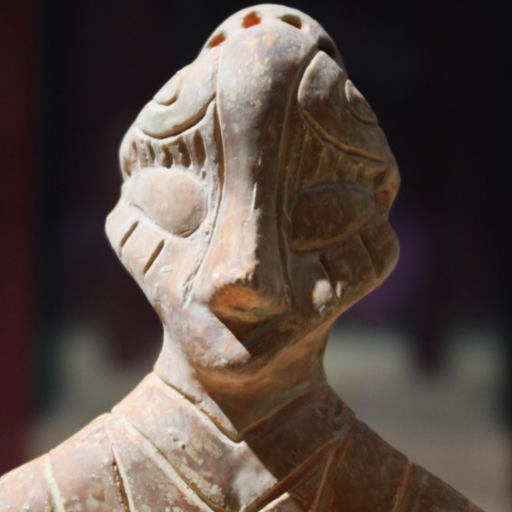
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mRenkoLineBreak.algo
- Rating: 5
- Installs: 2032
- Modified: 02/10/2022 05:07
Comments
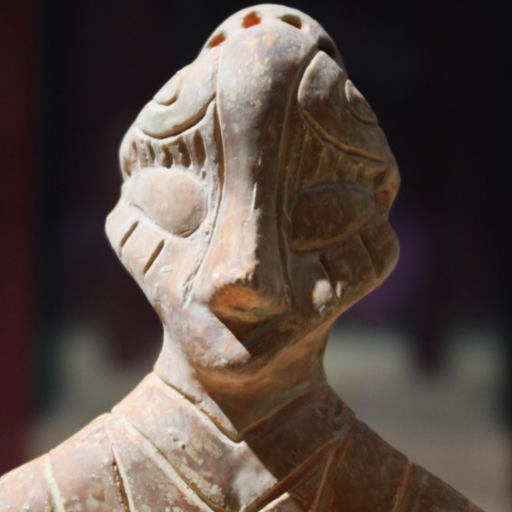
Hi privateam000
Thank you for your review and code revision. The LineBreak custom indicator is designed to track changes in price pressure.
The trigger for a new bar level occurs when the price reaches a certain distance in pips from the last level, with the size of each brick defined as BrickSize (inpBlockSize). Essentially, this indicator employs a method to smooth price movements in terms of pips.
In this version, you have the flexibility to set BrickSize in pips as a custom number. This is a feature that is not permitted in RenkoBars. For example, you can use values like inpBrickSize: 7, 15, 30, 60, or 120.
I'm confident that there are no bugs within the code. However, if you're encountering a situation where the indicator results aren't being displayed due to processing resource constraints, I recommend freeing up memory.
In your previous versions of RenkoBars, a similar solution was employed, albeit with a distinct perspective and slight variations in the results.
The output value of the indicator, outRenkoNewLevel, represents the target level. For further information and cooperation, please don't hesitate to contact me on telegram.mfejza2
After reflection, I think this is the best solution for renko
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Cloud("Renko Open", "Renko Close", FirstColor = "Green", SecondColor = "Red", Opacity = 0.5)]
[Indicator(IsOverlay = true, AccessRights = AccessRights.None)]
public class RenkoOnChart : Indicator
{
[Parameter(DefaultValue = "Renko10")]
public TimeFrame RenkoPips { get; set; }
[Output("Renko Open", LineColor = "Red")]
public IndicatorDataSeries RenkoUp { get; set; }
[Output("Renko Close", LineColor = "Green")]
public IndicatorDataSeries RenkoDown { get; set; }
Bars renko;
IndicatorDataSeries high, low, open, close;
protected override void Initialize()
{
renko = MarketData.GetBars(RenkoPips);
high = CreateDataSeries();
low = CreateDataSeries();
open = CreateDataSeries();
close = CreateDataSeries();
}
public override void Calculate(int index)
{
var indexBase = renko.OpenTimes.GetIndexByTime(Bars.OpenTimes.Last(0));
open[index] = renko.OpenPrices[indexBase];
close[index] = renko.ClosePrices[indexBase];
high[index] = renko.HighPrices[indexBase];
low[index] = renko.LowPrices[indexBase];
RenkoUp[index] = close[index] > open[index] ? high[index] : low[index];
RenkoDown[index] = close[index] < open[index] ? high[index] : low[index];
}
}
}
Hi, First of all thank you for all the codes you post! You do a lot of work !
I fixed the double renko bug. example (50 pips): if a bar makes more than 100 pips and retraces directly, there is an error. There must surely be some bugs. Enjoy
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Cloud("Renko Top", "Renko NewLevel", FirstColor = "Red", SecondColor = "Red", Opacity = 0.5)]
[Cloud("Renko NewLevel", "Renko Bottom", FirstColor = "Green", SecondColor = "Green", Opacity = 0.5)]
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class mRenkoLineBreak : Indicator
{
[Parameter("PIPS BoxSize (default 50)", DefaultValue = 50, MinValue = 2)]
public int inpBrickSize { get; set; }
[Output("Renko Top", LineColor = "Gray", LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outRenkoTop { get; set; }
[Output("Renko Bottom", LineColor = "Gray", LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outRenkoBottom { get; set; }
[Output("Renko NewLevel", LineColor = "Transparent", LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outRenkoNewLevel { get; set; }
private double _bricksize;
private IndicatorDataSeries _top, _bottom, _newlevel;
protected override void Initialize()
{
_bricksize = inpBrickSize * Symbol.PipSize;
_top = CreateDataSeries();
_bottom = CreateDataSeries();
_newlevel = CreateDataSeries();
}
public override void Calculate(int i)
{
_top[i] = i > 1 ? _top[i - 1] : Bars.HighPrices[i];
_bottom[i] = i > 1 ? _bottom[i - 1] : Bars.LowPrices[i];
_newlevel[i] = i > 1 ? _newlevel[i - 1] : _top[i];
if (Bars.ClosePrices.Last(0) >= _top[i - 1] + _bricksize)
{
var test = Math.Floor((Bars.ClosePrices.Last(0) - _top[i - 1]) / _bricksize);
_top[i] = (test == 0 || test == 1 ? _top[i - 1] + _bricksize : _top[i - 1] + _bricksize * test );
_bottom[i] = (test == 0 || test == 1 ? _top[i] - _bricksize : _top[i - 1] - _bricksize * test);
_newlevel[i] = _top[i];
}
if (Bars.ClosePrices.Last(0) <= _bottom[i - 1] - _bricksize)
{
var test = Math.Floor((_bottom[i] - Bars.ClosePrices.Last(0)) / _bricksize);
_top[i] = (test == 0 || test == 1 ? _top[i - 1] - _bricksize : _top[i - 1] - _bricksize * test );
_bottom[i] = (test == 0 || test == 1 ? _top[i] - _bricksize : _top[i - 1] - _bricksize * test- _bricksize);
_newlevel[i] = _bottom[i];
}
outRenkoTop[i] = _bottom[i] == _newlevel[i] ? _bottom[i] + _bricksize : _top[i];
outRenkoBottom[i] = _top[i] == _newlevel[i] ? _top[i] - _bricksize : _bottom[i];
outRenkoNewLevel[i] = _newlevel[i];
}
}
}
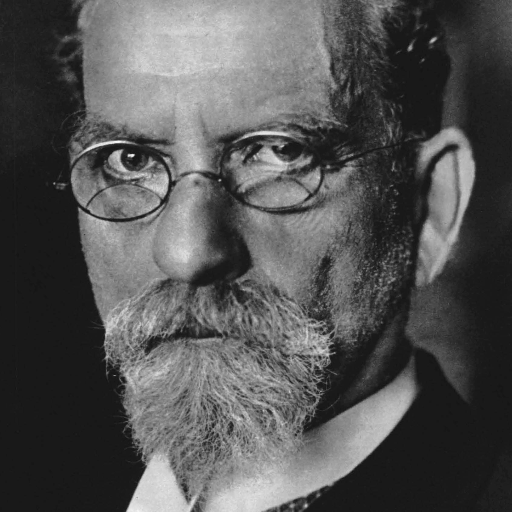
PSZ GRE \\ DR 15 **** ++ ++
%%%% + ++ \ \ /
Hi privateam000,
Thank you for your review and code revision. The LineBreak custom indicator is designed to track changes in price pressure.
The trigger for a new bar level occurs when the price reaches a certain distance in pips from the last level, with the size of each brick defined as BrickSize (inpBlockSize). Essentially, this indicator employs a method to smooth price movements in terms of pips.
In this version, you have the flexibility to set BrickSize in pips as a custom number. This is a feature that is not permitted in RenkoBars. For example, you can use values like inpBrickSize: 7, 15, 30, 60, or 120.
I'm confident that there are no bugs within the code. However, if you're encountering a situation where the indicator results aren't being displayed due to processing resource constraints, I recommend freeing up memory.
In your previous versions of RenkoBars, a similar solution was employed, albeit with a distinct perspective and slight variations in the results.
The output value of the indicator, outRenkoNewLevel, represents the target level. For further information and cooperation, please don't hesitate to contact me on telegram.mfejza2