Description
OrglobalFx_Simple_Waddar_cBOT_v1_1 with breakeven
orglobalng@gmail.com
Reference from Waddah Attah Exploasion
https://ctrader.com/algos/indicators/show/2609
Logic Update:
Buy = Histogram (Green) > Explosion Line (Yellow) for the Last Bar
Sell = Histogram (Red) > Explosion Line (Yellow) for the Last Bar
Note: Discovered that the previous version wasn't picking trades accurately.
Features:
- Different calculations
- Breakeven trigger added with trailing stop(trails the prices at breakeven).
/////////////////////////////////////////////////////////////////
//OrglobalFx_Simple_Waddar_cBOT_v1_1 with breakeven
// orglobalng@gmail.com
// Reference from Waddah Attah Exploasion
// https://ctrader.com/algos/indicators/show/2609
//
// Logic Update:
// Buy = Histogram (Green) > Explosion Line (Yellow) for the Last Bar
// Sell = Histogram (Red) > Explosion Line (Yellow) for the Last Bar
//
//Features:
// Different calculations
//Breakeven trigger added with trailing stop(trails the prices at breakeven).
////////////////////////////////////////////////////////////////
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.EasternStandardTime, AccessRights = AccessRights.None)]
public class OrglobalFx_Simple_Waddar_cBOT_v1_1 : Robot
{
[Parameter("Volume", DefaultValue = 1000, Group = "Protection")]
public double volume { get; set; }
[Parameter("Instance Name", DefaultValue = "OrglobalFx")]
public string InstanceName { get; set; }
[Parameter("Stop Loss (pips)", Group = "Protection", DefaultValue = 200)]
public double StopLossInPips { get; set; }
[Parameter("Take Profit (pips)", Group = "Protection", DefaultValue = 150)]
public double TakeProfitInPips { get; set; }
[Parameter("Include Break-Even", DefaultValue = true, Group = "Protection")]
public bool IncludeBreakEven { get; set; }
[Parameter("Break-Even Trigger (pips)", DefaultValue = 10, MinValue = 1, Group = "Protection")]
public int BreakEvenPips { get; set; }
[Parameter("Break-Even Extra (pips)", DefaultValue = 10, MinValue = 1, Group = "Protection")]
public int BreakEvenExtraPips { get; set; }
[Parameter("Trail after Break-Even", DefaultValue = true, Group = "Protection")]
public bool Includetrail { get; set; }
[Parameter("Sensitivity", DefaultValue = 150, Group = "WAE")]
public int Sensitivity { get; set; }
[Parameter("Fast EMA", DefaultValue = 26, Group = "WAE")]
public int FastEMA { get; set; }
[Parameter("Slow EMA", DefaultValue = 52, Group = "WAE")]
public int SlowEMA { get; set; }
[Parameter("Channel Period", DefaultValue = 20, Group = "WAE")]
public int BBPeriod { get; set; }
[Parameter("Channel Multiplier", DefaultValue = 4, Group = "WAE")]
public double BBMultiplier { get; set; }
[Parameter("DeadZonePip", DefaultValue = 30, Group = "WAE")]
public int DeadZonePip { get; set; }
[Output("UpTrend", LineColor = "Green", PlotType = PlotType.Histogram, Thickness = 5)]
public IndicatorDataSeries Uptrend { get; set; }
[Output("DownTrend", LineColor = "Red", PlotType = PlotType.Histogram, Thickness = 5)]
public IndicatorDataSeries Downtrend { get; set; }
[Output("Explosion Line", LineColor = "Yellow", Thickness = 1)]
public IndicatorDataSeries Explosion { get; set; }
[Output("Dead Line", LineColor = "White", Thickness = 1)]
public IndicatorDataSeries Dead { get; set; }
private OrglobalFx_Waddah_Attah_Edited_v1_1 _wae;
protected override void OnStart()
{
_wae = Indicators.GetIndicator<OrglobalFx_Waddah_Attah_Edited_v1_1>(Sensitivity, FastEMA, SlowEMA, BBPeriod, BBMultiplier, DeadZonePip);
}
#region OnBar
protected override void OnBar()
{
// Put your core logic here
var uptrend = _wae.Uptrend.Last(1);
var downtrend = _wae.Downtrend.Last(1);
var explosion = _wae.Explosion.Last(1);
var dead = _wae.Dead.Last(1);
var position = Positions.Find(InstanceName, SymbolName);
if (uptrend > explosion)
{
if (position == null)
ExecuteMarketOrder(TradeType.Buy, SymbolName, volume, InstanceName, StopLossInPips, TakeProfitInPips);
}
if (downtrend > explosion)
{
if (position == null)
ExecuteMarketOrder(TradeType.Sell, SymbolName, volume, InstanceName, StopLossInPips, TakeProfitInPips);
}
//breakeven trigger
if (IncludeBreakEven)
{
BreakEvenAdjustment();
}
}
#endregion
#region Break Even
// code from clickalgo.com
private void BreakEvenAdjustment()
{
var allPositions = Positions.FindAll(InstanceName, SymbolName);
foreach (Position position in allPositions)
{
//if (position.StopLoss != null)
//return;
var entryPrice = position.EntryPrice;
var distance = position.TradeType == TradeType.Buy ? Symbol.Bid - entryPrice : entryPrice - Symbol.Ask;
// move stop loss to break even plus and additional (x) pips
if (distance >= BreakEvenPips * Symbol.PipSize)
{
if (position.TradeType == TradeType.Buy)
{
if (position.StopLoss <= position.EntryPrice + (Symbol.PipSize * BreakEvenExtraPips) || position.StopLoss == null)
{
if (Includetrail)
{
ModifyPosition(position, position.EntryPrice + (Symbol.PipSize * BreakEvenExtraPips), position.TakeProfit, true);
Print("Stop Loss to Break Even set for BUY position {0}", position.Id);
}
else if (!Includetrail)
{
ModifyPosition(position, position.EntryPrice + (Symbol.PipSize * BreakEvenExtraPips), position.TakeProfit);
Print("Stop Loss to Break Even set for BUY position {0}", position.Id);
}
}
}
else
{
if (position.StopLoss >= position.EntryPrice - (Symbol.PipSize * BreakEvenExtraPips) || position.StopLoss == null)
{
if (Includetrail)
{
ModifyPosition(position, entryPrice - (Symbol.PipSize * BreakEvenExtraPips), position.TakeProfit, true);
Print("Stop Loss to Break Even set for SELL position {0}", position.Id);
}
else if (!Includetrail)
{
ModifyPosition(position, entryPrice - (Symbol.PipSize * BreakEvenExtraPips), position.TakeProfit);
Print("Stop Loss to Break Even set for SELL position {0}", position.Id);
}
}
}
}
}
}
#endregion
}
}
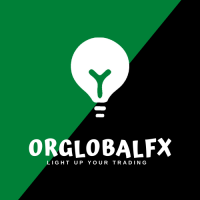
Orglobalfx01
Joined on 03.03.2021
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: OrglobalFx_Simple_Waddar_cBOT_v1_1.algo
- Rating: 5
- Installs: 2026
- Modified: 13/10/2021 09:54
Warning! Running cBots downloaded from this section may lead to financial losses. Use them at your own risk.
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
EV
Great results, thank you
DO
Thank you for this.
Awesome bot thank you.
Getting error - 19/12/2022 19:26:01.170 | → Request to amend position PID108132349 (SL: 1.06522) is REJECTED with error "New SL for BUY position should be <= current BID price. current BID: 1.06448, SL: 1.06522; "