Description
This bot creates a simple moving average band with high and low price and open orders when the price is lower than the bottom band or higher than top band.
Run Optimization to get the bests params for you.
If you like this bot, let me know, and if you can and want to help me keep creating bots for you
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class SMABands : Robot
{
[Parameter("Quantity (Lots)", Group = "Volume", DefaultValue = 0.01, MinValue = 0.01, Step = 0.01)]
public double Quantity { get; set; }
[Parameter("Simple Moving Average Value", Group = "Volume", DefaultValue = 1, MinValue = 1, Step = 1)]
public int smaValue { get; set; }
[Parameter("Minimun Take Pips", Group = "Take", DefaultValue = 1, MinValue = 1, Step = 1)]
public double minTake { get; set; }
[Parameter("Enable TakeProfit?", Group = "TakeProfit", DefaultValue = true)]
public bool useTakeProfit { get; set; }
[Parameter("Take Profit", Group = "TakeProfit", DefaultValue = 1, MinValue = 1, Step = 1)]
public int takeProfit { get; set; }
[Parameter("Enable Stop Loss?", Group = "StopLoss", DefaultValue = true)]
public bool useStopLoss { get; set; }
[Parameter("Stop Loss", Group = "StopLoss", DefaultValue = 1, MinValue = 1, Step = 1)]
public int stopLoss { get; set; }
private MovingAverage FourMinimum;
private MovingAverage FourMaximum;
private double LastValue { get; set; }
private double volumeInUnits;
protected override void OnStart()
{
volumeInUnits = Symbol.QuantityToVolumeInUnits(Quantity);
FourMinimum = Indicators.SimpleMovingAverage(Bars.LowPrices, smaValue);
FourMaximum = Indicators.SimpleMovingAverage(Bars.HighPrices, smaValue);
}
protected override void OnBar()
{
if (Positions.Count() >= 1)
{
if (Bars.LastBar.High >= FourMaximum.Result.LastValue)
{
foreach (var position in Positions)
{
if (position.Pips > minTake)
{
ClosePosition(position);
}
}
}
}
bool buyOrSell = Bars.LastBar.Close >= FourMinimum.Result.LastValue;
ExecuteMarketOrder((buyOrSell ? TradeType.Buy : TradeType.Sell), SymbolName, volumeInUnits, "SMABands", (useStopLoss ? stopLoss : double.MaxValue), (useTakeProfit ? takeProfit : double.MaxValue));
}
}
}
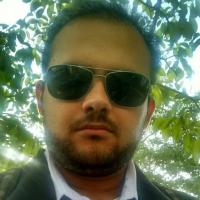
CabralTrader
Joined on 10.03.2020
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: SMABands.algo
- Rating: 5
- Installs: 2094
Warning! Running cBots downloaded from this section may lead to financial losses. Use them at your own risk.
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
thank you for your bots