Description
re code the mt4 i-session indicator to draw session box in pure calgo code if you have code problem you can comment this post thanks
using System;
using System.Collections.Generic;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class SessionBox : Indicator
{
[Parameter(DefaultValue = "22:00")]
public string asia { get; set; }
[Parameter(DefaultValue = 12)]
public int asiaHour { get; set; }
[Parameter(DefaultValue = "08:00")]
public string euro { get; set; }
[Parameter(DefaultValue = 9)]
public int euroHour { get; set; }
[Parameter(DefaultValue = "13:00")]
public string us { get; set; }
[Parameter(DefaultValue = 10)]
public int usHour { get; set; }
// store last Session Start and Session End
public DateTime asStart;
public DateTime asEnd;
public DateTime euStart;
public DateTime euEnd;
public DateTime usStart;
public DateTime usEnd;
protected override void Initialize()
{
// Initialize and create nested indicators
}
public override void Calculate(int index)
{
var dateTime = MarketSeries.OpenTime[index];
List<Box> boxs = sbox(index);
for (int i = 0; i < boxs.Count; i++)
{
var box = boxs[i];
double[] high_low = boxHighLow(index, box);
drawBox(box.label, box.left, box.right, high_low[0], high_low[1], box.clr);
}
}
// box calcuate logic
public List<Box> sbox(int index)
{
List<Box> boxs = new List<Box>();
DateTime current = MarketSeries.OpenTime[index];
string asStartHour = asia.Split('-')[0].Split(':')[0];
string asStartMinute = asia.Split('-')[0].Split(':')[1];
string euroStartHour = euro.Split('-')[0].Split(':')[0];
string euroStartMinute = euro.Split('-')[0].Split(':')[1];
string usStartHour = us.Split('-')[0].Split(':')[0];
string usStartMinute = us.Split('-')[0].Split(':')[1];
if (current.Hour == Int32.Parse(asStartHour) && current.Minute == Int32.Parse(asStartMinute))
{
asStart = current;
asEnd = current.AddHours(asiaHour);
}
if (current.Hour == Int32.Parse(euroStartHour) && current.Minute == Int32.Parse(euroStartMinute))
{
euStart = current;
euEnd = current.AddHours(euroHour);
}
if (current.Hour == Int32.Parse(usStartHour) && current.Minute == Int32.Parse(usStartMinute))
{
usStart = current;
usEnd = current.AddHours(usHour);
}
if (current >= asStart && current <= asEnd)
{
boxs.Add(new Box(asStart.ToString(), asStart, asEnd, Colors.Green));
}
if (current >= euStart && current <= euEnd)
{
boxs.Add(new Box(euStart.ToString(), euStart, euEnd, Colors.Red));
}
if (current >= usStart && current <= usEnd)
{
boxs.Add(new Box(usStart.ToString(), usStart, usEnd, Colors.Yellow));
}
return boxs;
}
// calculate session High Low
private double[] boxHighLow(int index, Box box)
{
DateTime left = box.left;
double[] high_low = new double[2]
{
MarketSeries.High[index],
MarketSeries.Low[index]
};
while (MarketSeries.OpenTime[index] >= left)
{
high_low[0] = Math.Max(high_low[0], MarketSeries.High[index]);
high_low[1] = Math.Min(high_low[1], MarketSeries.Low[index]);
index--;
}
return high_low;
}
// draw session box
private void drawBox(String label, DateTime left, DateTime right, Double high, Double low, Colors clr)
{
ChartObjects.DrawLine(label + "_low", left, low, right, low, clr);
ChartObjects.DrawLine(label + "_high", left, high, right, high, clr);
ChartObjects.DrawLine(label + "_left", left, high, left, low, clr);
ChartObjects.DrawLine(label + "_right", right, high, right, low, clr);
}
// box data struct
public struct Box
{
public string label;
public DateTime left;
public DateTime right;
public Colors clr;
public Box(string label, DateTime left, DateTime right, Colors clr)
{
this.label = label;
this.left = left;
this.right = right;
this.clr = clr;
}
}
}
}
noodles
Joined on 30.03.2015
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: SessionBox.algo
- Rating: 3.33
- Installs: 10524
- Modified: 13/10/2021 09:54
Comments
quite amazes me that no one can get this indicator right. I finally had to break down and pay a programmer to make this in caglo. and he still has to get the asia session working right.
is calgo code that much more difficult than mt4 ?
Hi and thanks,
could you insert MidLines like Trading View indicator "AsianSessioneHightLowMidLine"?
It's most important for trading.
Thank you.
Giovanni
on the subject of wishlist, could you add the session range and ability to change box colours
there is an mt4 indicator with all the same properties if you want more info
thanks again
Hi and thanks
I use to use the mt4 version and found open close timing essential with respect to the candle opening.
If I may ask could you label top of the boxes and separate pacific time (australia) from asia time open close.
Does this indi also consider daylight savings in each country?
Once again thankyou
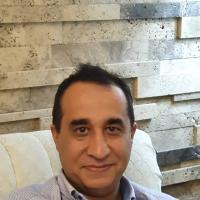
Thanks for good indicator, please let me know what change should I do to fill in the boxes. thanks
Thankyou for this indicator.
Is it possible to shade the boxes different colors with a transparency filter like on the MT4 version?
Cheers
Thanks for the value indicator,
Can you add pip range of each session below box? It will help us so much.
Hi,
This just what I was looking for. Loads OK onto chart,, except when I try and change the time. I am in the UK and London opens 1 hour ahead of Europe. I have tried to adjust the European time from 08.00 to 07.00 which would line up with the current London opening, but it just disapears of my chart.
Can you help?
Regards
Terry
Hi, thanks for indi.
Does is possible to add settings that allow changing line thickness and shape (normal line, dots etc.) ?
Works good for me. Need to adjust the times to my liking, but does exactly what I wanted - scaled boxes around each session.