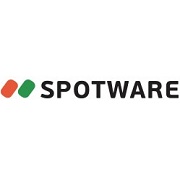
Topics
Replies
admin
07 Feb 2013, 09:56
( Updated at: 19 Mar 2025, 08:57 )
Hello,
Did you backtest with the same period with which you tested live? We need some more information to investigate this. If you wish to either share your code with us so that we can test or describe the logic by which the robot is executing a trade you may post it here or email us at support@ctrader.com.
Using OnTick as opposed to OnBar will execute on each tick instead of the beginning of the new trendbar. Depending on the logic that you use it may be required that you use the OnTick in order not to miss any trades but it is not possible to say for sure without knowing more.
@admin
admin
05 Feb 2013, 11:54
Hello,
No, there is no Heiken Ashi class in the cAlgo API. If it is useful, you may use this Heiken Ashi indicator uploaded in the indicators section.
@admin
admin
01 Feb 2013, 10:55
( Updated at: 23 Jan 2024, 13:11 )
Hello,
For Market Depth Ask and Bid you need to use MarketDeptth interface. See also the sample code for Market Depth.
For tick volume, use MarkeSeries interface. See also, this sample for [MarketSeries.TickVolume]
If you require additional help, please describe your algorithm logic.
@admin
admin
31 Jan 2013, 10:07
RE:
Hi there,
I just built a robot, and when I went to go backtest it....the "play" button was blurred out.
What might cause that to happen??
Ive just copied a demo robot and tweaked it ever so slightly.
Didnt even touch the trade logic, just changed the indicator.
Now it won't let me backtest??
Thanks
Could you check if your connection is ok when this happens? Next to the account number (top left) there should be a green steady light.
@admin
admin
30 Jan 2013, 16:42
Hello,
The robot will use the symbol that it is attached to, for creating new orders. Therefore, you cannot use any symbol.
The syntax should be:
Trade.CreateBuyMarketOrder(Symbol, posVolume);
View the API Reference for the syntax: /api/internals/itrade
The ability to use other symbols is in the list for future implementation.
@admin
admin
29 Jan 2013, 14:32
RE:
Hi again admin,
Something has come to mind and I would like to run it by you. You mentioned before in one of my other posts that count -1 represents the current values which are moving when using OnBar() until e.g an hour passes if on the 1h time frame hence finalizing the close price and so forth. So I am assuming that this might be the problem since im using non static values and the robot initializes a second, third ect position from the roughly the same price and time. I would like to know if count -2 works the same way on indicators as it is used on the MarketSeries command? So in other words I use the previous price close with the previous close values created by the indicators instead of relying on the .LastValue command.
Thanks
It is always best practice to use the previous to the last bar if the logic is based on those values. It is not certain that this is the reason why you get multiple positions. There are a number of ways you can prevent this. For instance, check if there is a position opened by the robot by assigning a global field for position which will be assigned in the OnPositionOpened event as well as assigned null in the OnPositionClosed event. Then before you create an order you can check if this field is null. This will ensure that there is only one position open at a time.
private Position _position; protected override void OnTick() { if(Trade.IsExecuting) return; if(_position == null) Trade.CreateBuyMarketOrder(Symbol, 10000); } protected override void OnPositionOpened(Position openedPosition) { _position = openedPosition; } protected override void OnPositionClosed(Position position) { _position = null; }
Alternatively, you can follow the logic in the sample martingale, which only opens a position in the OnStart and in the OnPositionClosed.
@admin
admin
29 Jan 2013, 12:56
The Sample Martingale Robot that is included in the platform has a similar logic and works properly, so the error has to be related to the code. You may test the Sample Martingale Robot to see for yourself. Also, I suggest that you modify the code of the martingale bit by bit towards your desired algorithm and this way you may be able to identify the error in your original code.
@admin
admin
29 Jan 2013, 10:39
Please use this code to compare the values at the index prior to the index of the current bar.
ParabolicSAR psar; protected override void OnStart() { psar = Indicators.ParabolicSAR(0.02,0.2); } protected override void OnBar() { int index = MarketSeries.Close.Count - 1; double psarValue = psar.Result[index-1]; Print("Open Time {0}", MarketSeries.OpenTime[index-1]); Print("P SAR Value {0}", psarValue); }
@admin
admin
11 Feb 2013, 11:30
Hello,
Thank you for your kind words and thank you for your suggestions as well. Using multiple currency pairs in a robot is currently under develpment and will be available in the future.
As far as the other suggestions, they are in the list of future possible additions to the API and it is very probable that they will be implemented as well.
Best Regards.
@admin