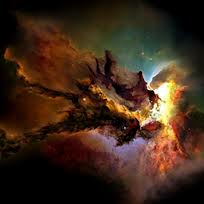
Topics
Replies
atrader
30 Oct 2013, 12:44
RE:
PCWalker said:
Will this take anymore Longer?
Have you seen this: /forum/cbot-support/1739?page=1#7
@atrader
atrader
30 Oct 2013, 12:32
RE:
jhtrader said:
Thanks for the answer.. I am sure that this will be alot of help to newbie programmers like myself.
Is this the correct and most efficient way to write this function
The following function
- gets the last 5 min range
double Rng1 = Math.Round((series1m.High[index1 - 1] - series1m.Low[index1 - 1]) / Symbol.PipSize, 2);
You mean series1m is the min 5 market series? I suggest you use better naming.
- creates a 15 min range list with cummulative values from the last bar to the 10th most recent bar (i dont use this list this is just to learn how to add and retrieve)
That is also confusing. You mean the sum of ranges of the 15 min market series? Give example with numbers.
To learn how to use lists you can see some tutorials e.g. http://www.dotnetperls.com/list
@atrader
atrader
21 Oct 2013, 10:07
RE:
cogs said:
Yes, I do.
Hichem has been great to work with. I am yet to get the end result and still concerned about intellectual property.
Sharing robots on a public forum is just insane! Indicators maybe yes. Haven't you guys heard of FapTurbo and others which ended up in too many traders trading the same system?
As soon as you let your system get into even one other persons hands, the secret is out and compound ownership is unstoppable. This is why Forex Factory and others are great food for brokers.
If I see my concept out there I will hunt down the leak and prosecute them, then visit them in person.
Please enlighten the rest of us. What is the problem if too many users trade with the same strategy? Moreover, how many is too many?
@atrader
atrader
30 Sep 2013, 16:56
RE:
stoko said:
If PendingOrder something like FOK explained in here
http://www.investopedia.com/terms/f/fok.asp
Definition of 'Fill Or Kill - FOK'
A type of time-in-force designation used in securities trading that instructs a brokerage to execute a transaction immediately and completely or not at all. This type of order is most likely to be used by active traders and is usually for a large quantity of stock. The order must be filled in its entirety or canceled (killed). The purpose of a fill or kill order is to ensure that a position is entered at a desired price.
They are GTC - Good till Cancelled.
You can read more here:
http://www.schwab.com/public/schwab/resource_center/expert_insight/investing_strategies/stocks/order_types_what_they_mean_and_how_theyre_handled.html
@atrader
atrader
25 Sep 2013, 10:17
RE: RE:
hichem said:
Have you actually tries to compile this ?
this line will throw an error:
_robot.PrintToLog(message)PrintToLog is not defined in cAlgo.API.Robot
namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC)] public class PrintRobot : Robot { public void PrintToLog(string message) { Print(message); } } public class CustomClass { private PrintRobot _robot; public CustomClass(PrintRobot robot) { _robot = robot; } public void Print(string message) { _robot.PrintToLog(message); } } }
@atrader
atrader
17 Sep 2013, 11:20
RE:
Cerunnos said:
Hi, I'm using a VPS and would like to send an e-mail with Notifications.SendEmail () every half hour to check if my robot running properly. How can I do that? Unfortunately, one cannot launch cAlgo automatically with a specific robot ...
Thanks in advance! Rainer
use the Timer Class /forum/cbot-support/357#4
Send email: /api/internals/inotifications/sendemail-6532
start cAlgo from a robot: System.Diagnostics.Process.Start("[System.Diagnostics.Process.Start("C:\\...\\cAlgo.exe]");
/forum/suggestions/1221?page=1#6
You can just use search. most questions have been answered :)
@atrader
atrader
12 Sep 2013, 16:45
RE: RE:
cAlgo_Fanatic said:
itekeste said:
Hi
I've been working on an improved martingale robot based around trading certain currency pairs at specific times of the day combined with using an OCO order for the first entry but need someone to code it up. If anyone is interested in doing so then let me know as it has proved to be profitable in manual testing and trading over the last 6 months.
The code for the martingale robot is already in cAlgo so it shouldn't take long to tweak certain parameters which is what is needed for this improved version hence I don't think it will take up much time to code up but I don't have coding experience.
Anyone who's interested just respond on this post (include your email address) or email me.
Thanks and happy trading,
Isaac
Consider posting this in the Jobs section.
Yes, do post it in the Jobs section so that we can contact you privately. Thanks.
@atrader
atrader
30 Aug 2013, 17:11
RE:
ianj said:
This is rubbish - I just managed to open a EURUSD, GBPUSD, AUDUSD positions from a single Robot on a EURUSD chart
Symbol looks to be both a property and interface (in cAlgo.API.Internals)
I implemented Symbol in my own class (MySymbol) and override Code with GBPUSD & AUDUSD and passed an instance of that into the Trade api
mySymbol.Code = "GBPUSD";
Trade.CreateMarketOrder(TradeType.Buy, mySymbol, Volume);
mySymbol.Code = "EURUSD";
Trade.CreateMarketOrder(TradeType.Buy, mySymbol, Volume);
mySymbol.Code = "AUDUSD";
Trade.CreateMarketOrder(TradeType.Buy, mySymbol, Volume);and voila ! 3 positions - 3 pairs
This is nice. Did you code the getter for the symbol properties (Ask, Bid, etc) for this class?
@atrader
atrader
30 Aug 2013, 15:53
You can have a robot that logs all the account open positions to a file and then continuously cross checks the positions in the file against the currently open positions under the account and the ones that do not correspond to the ones in the file have been closed.
Maybe you can log the closed ones to another file which can be accessed by other robots.
Or you will just have the one file which logs each time a position has been opened and then in your robots you just compare the open positions to the ones in the file.
@atrader
atrader
29 Aug 2013, 15:41
( Updated at: 21 Dec 2023, 09:20 )
RE: RE: RE:
tradermatrix said:
coolutm said:
cAlgo_Fanatic said:
When the number circled in red, which is equal to the net profit minus the commission (23.16 -15 =11.16) will be greater than 300 or less than -300, then the position will close.
protected override void OnTick() { var netProfit = 0.0; foreach (var openedPosition in Account.Positions) { netProfit += openedPosition.NetProfit + openedPosition.Commissions; } ChartObjects.DrawText("a", netProfit.ToString(), StaticPosition.BottomLeft); if (Math.Abs(netProfit) >= 300.0) { foreach (var openedPosition in Account.Positions) { Trade.Close(openedPosition); } } }
Hi cAlgo Support,
Thank you for the reply. This one works perfect.
Just one more question (or request), what if I want to set up different amount for the profit side and stop loss side?
I simply added one more if phrase....if (NetProfit <= -200) but didn't work.
Please help me.
{
var netProfit = 0.0;
foreach (var openedPosition in Account.Positions)
{
netProfit += openedPosition.NetProfit + openedPosition.Commissions;
}
ChartObjects.DrawText("a", netProfit.ToString(), StaticPosition.BottomLeft);
if ((Math.Abs(netProfit) >= 200.0) || (Math.Abs(netProfit) <= -200))
{
foreach (var openedPosition in Account.Positions)
{
Trade.Close(openedPosition);
}
}
}
Math.Abs() means the absolute or positive value. Therefore the second part of the condition
if ((Math.Abs(netProfit) >= 200.0) || (Math.Abs(netProfit) <= -200))
will always be false.
@atrader
atrader
31 Jul 2013, 10:11
I corrected a couple of things according to the paper.
using System; using cAlgo.API; using cAlgo.API.Indicators; namespace cAlgo.Indicators { [Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, ScalePrecision = 5)] public class Forum_1305 : Indicator { #region Variables private IndicatorDataSeries _filt; private IndicatorDataSeries _hp; private double a1; private double alpha1; private double b1; private double c1; private double c2; private double c3; #endregion #region Parameters [Output("Roofing", Color = Colors.Purple)] public IndicatorDataSeries Roofing { get; set; } [Parameter("Max", DefaultValue = 48)] public int Period { get; set; } [Parameter("Min", DefaultValue = 10)] public int Period2 { get; set; } [Parameter] public DataSeries Source { get; set; } #endregion protected override void Initialize() { _filt = CreateDataSeries(); _hp = CreateDataSeries(); alpha1 = (Math.Cos(Math.Sqrt(2) * Math.PI / Period) + Math.Sin(Math.Sqrt(2) * Math.PI / Period) - 1) / Math.Cos(Math.Sqrt(2) * Math.PI / Period); a1 = Math.Exp(-Math.Sqrt(2)*Math.PI/Period2); b1 = 2 * a1 * Math.Cos(Math.Sqrt(2) * Math.PI / Period2); c2 = b1; c3 = -a1*a1; c1 = 1 - c2 - c3; _hp[0] = 0; _hp[1] = 0; _filt[0] = 0; _filt[1] = 0; } public override void Calculate(int index) { if (index < 2) return; _hp[index] = (1 - alpha1/2)*(1 - alpha1/2)* ((Source[index] - 2*Source[index - 1] + Source[index - 2]) + 2*(1 - alpha1)*_hp[index - 1] - (1 - alpha1)*(1 - alpha1)*_hp[index - 2]); _filt[index] = c1 * (_hp[index] + _hp[index-1]) / 2 + c2 * _filt[index - 1] + c3 * _filt[index - 2]; Roofing[index] = _filt[index]; } } }
@atrader
atrader
29 Jul 2013, 12:33
Hi,
To identify the previous bar below the 20 ema you can compare the price (open, close, high, low whichever you want) to the ema.
e.g. if ( MarketSeries.High[index-1] < ema.Result[index-1] )
where ema is: private ExponentialMovingAverage ema;
initialized in the Initialize() method:
e.g. ema = Indicators.ExponentialMovingAverage(MarketSeries.Close, 20);
To see if it is a bear bar you compare the open to close
e.g. if ( MarketSeries.Open[index-1] > MarketSeries.Close[index-1]
You can do the same for as far back as you want with a loop
for (int i = index - Period; i < index; i++) { var lastBarBelowEma = MarketSeries.High[i - 1] < ema.Result[i - 1]; var lastBarBear = MarketSeries.Open[i - 1] > MarketSeries.Close[i - 1]; var priceActionWithinLastBar = MarketSeries.High[i] < MarketSeries.High[i - 1] && MarketSeries.Low[i] > MarketSeries.Low[i - 1]; if (lastBarBelowEma && lastBarBear && priceActionWithinLastBar) { double yValue = MarketSeries.High[i] + Symbol.PipSize*2; ChartObjects.DrawText("objectName", "Yes", i, yValue, VerticalAlignment.Center, HorizontalAlignment.Center, Colors.Red); } }
You can do something the equivalent with bars above ema.
Hope it helps.
@atrader
atrader
29 Jul 2013, 10:40
Consider these changes. The period over 10 for both fields, does not produce any results (goes to infinity). You probably need to confirm the formula. Or maybe post the formula here.
What is this indicator supposed to show?
namespace cAlgo.Indicators { [Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, ScalePrecision = 5)] public class Forum_1305 : Indicator { #region Variables private IndicatorDataSeries _filt; private IndicatorDataSeries _hp; private double a1; private double alpha1; private double b1; private double c1; private double c2; private double c3; #endregion #region Parameters [Output("Roofing", Color = Colors.Purple)] public IndicatorDataSeries Roofing { get; set; } [Parameter("Max", DefaultValue = 8)] public int Period { get; set; } [Parameter("Min", DefaultValue = 4)] public int Period2 { get; set; } [Parameter] public DataSeries Source { get; set; } #endregion protected override void Initialize() { _filt = CreateDataSeries(); _hp = CreateDataSeries(); // consider these changes: 180 = pi, 360 = 2*pi, 1.414 = Math.Sqrt(2), .707 = Math.Sqrt(2)/2 // alpha1 = (Math.Cos(.707*360/Period) + Math.Sin(.707*360/Period) - 1)/Math.Cos(.707*360/Period); alpha1 = (Math.Cos(Math.Sqrt(2) * Math.PI / Period) + Math.Sin(Math.Sqrt(2) * Math.PI / Period) - 1) / Math.Cos(Math.Sqrt(2) * Math.PI / Period); a1 = Math.Exp(-Math.Sqrt(2)*Math.PI/Period2); // b1 = 2*a1*Math.Cos(1.414*180/Period2); b1 = 2 * a1 * Math.Cos(Math.Sqrt(2) * Math.PI / Period2); c2 = b1; c3 = -a1*a1; c1 = 1 - c2 - c3; _hp[0] = 0; _hp[1] = 0; _hp[2] = 0; _filt[0] = 0; _filt[1] = 0; _filt[2] = 0; } public override void Calculate(int index) { if(index < 3) return; _hp[index] = (1 - alpha1/2)*(1 - alpha1/2)* ((Source[index] - 2*Source[index - 1] + Source[index - 2]) + 2*(1 - alpha1)*_hp[index - 2] - (1 - alpha1)*(1 - alpha1)*_hp[index - 3]); _filt[index] = c1*(_hp[index]*2)/2 + c2*_filt[index - 1] + c3*_filt[index - 2]; // Use print to check the results //Print("{0},{1},{2}", index, _hp[index], _filt[index]); Roofing[index] = _filt[index]; } } }
@atrader
atrader
25 Jul 2013, 14:55
RE:
Although there is one thing you could me me a bit more.
I want to use price channels for stop loss in some cases. Let's say long position is opened and I want to fix lower band of price channel as a stop loss. If position is profitable, I want to change from "fixed" value of stop loss to a trailing stop.
Thanks.
Hi, you can use the code from the cAlgo Sample Trailing.
@atrader
atrader
24 Jul 2013, 16:11
RE:
Hi.
How to implement Price Channel indicator in the robot, so I could set up length of periods, use it as stop loss and see indicator overlayed on the chart?
Thanks
this is a robot that uses the price channels, but it is missing a lot of functionality since you have not described the trading logic.
You can use this as a starting point or you can share the rest of the trading logic, e.g. how are trading signals triggered, based on price channels as well? How many positions does the robot open simultaneously, etc.
This robot starts a trade based on an input parameter (Buy) and volume.
The stop loss is calculated based on the channels. There is Take profit as input parameter.
//#reference: ..\Indicators\PriceChannels.algo using System; using System.Collections.Generic; using System.Linq; using System.Text; using cAlgo.API; using cAlgo.API.Requests; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot] public class PriceChannelRobot:Robot { private PriceChannels _priceChannels; [Parameter(DefaultValue = 14)] public int Period { get; set; } [Parameter(DefaultValue = "PriceChannelRobot")] public string LabelName { get; set; } [Parameter("Volume", DefaultValue = 10000, MinValue = 1000)] public int Volume { get; set; } [Parameter(DefaultValue = true)] public bool Buy { get; set; } [Parameter(DefaultValue = 10, MinValue = 1)] public int TakeProfit { get; set; } protected double StopLossPrice { get { return TradeCommand == TradeType.Buy ? LowValue : HighValue; } } protected double HighValue { get { return _priceChannels.HiChannel.LastValue; } } protected double LowValue { get { return _priceChannels.LowChannel.LastValue; } } protected override void OnStart() { _priceChannels = Indicators.GetIndicator<PriceChannels>(Period); } protected TradeType TradeCommand { get { return Buy ? TradeType.Buy : TradeType.Sell; } } protected override void OnBar() { // Your Trade condition bool tradeCondition = Account.Positions.Count(pos => pos.Label == LabelName) == 0; if (tradeCondition) ExecuteOrder(Volume, TradeCommand); } private void ExecuteOrder(int volume, TradeType tradeType) { var request = new MarketOrderRequest(tradeType, volume) { Label = LabelName, StopLossPips = (int?) (Math.Abs(StopLossPrice - Symbol.Bid)/Symbol.PipSize), TakeProfitPips = TakeProfit }; Trade.Send(request); } } }
@atrader
atrader
24 Jul 2013, 15:12
RE:
Anyone has any news about this cAlgoFX?
It seems they've just disappeared!
You may want to contact them here http://www.calgofx.com/
@atrader
atrader
07 Nov 2013, 17:32
RE: RE:Time
jhtrader said:
yes and yes. you can use MarketSeries.OpenTime.LastValue for convenience. You can also set the robot timezone.
@atrader