Warning! This section will be deprecated on February 1st 2025. Please move all your Indicators to the cTrader Store catalogue.
An update for this algorithm is currently pending moderation. Please revisit this page shortly to access the algorithm's latest version.
Description
This indicator shows the sentiment of five Momentum Oscillators, each with different lookback periods.
Trading zones are selected or identified when all indicators have the same sentiment (green for Long, red for Short).
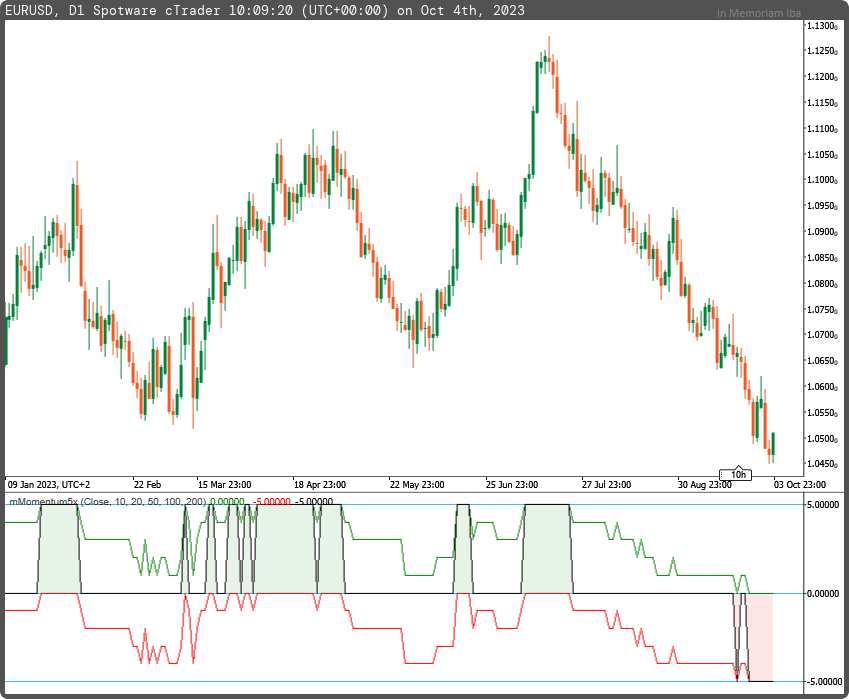
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Cloud("Polarity", "LevelZero", FirstColor = "Green", SecondColor = "Red", Opacity = 0.1)]
[Levels(-5,0,+5)]
[Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class mMomentum5x : Indicator
{
[Parameter("Data Source (close)", DefaultValue = enumPriceTypes.Close)]
public enumPriceTypes inpPriceType { get; set; }
[Parameter("Period (10)", DefaultValue = 10, MinValue = 2)]
public int inpPeriod1 { get; set; }
[Parameter("Period (20)", DefaultValue = 20, MinValue = 2)]
public int inpPeriod2 { get; set; }
[Parameter("Period (50)", DefaultValue = 50, MinValue = 2)]
public int inpPeriod3 { get; set; }
[Parameter("Period (100)", DefaultValue = 100, MinValue = 2)]
public int inpPeriod4 { get; set; }
[Parameter("Period (200)", DefaultValue = 200, MinValue = 2)]
public int inpPeriod5 { get; set; }
[Output("Polarity", LineColor = "Silver", PlotType = PlotType.Line, Thickness = 1)]
public IndicatorDataSeries outPol { get; set; }
[Output("LevelZero", LineColor = "Transparent", PlotType = PlotType.Line, Thickness = 1)]
public IndicatorDataSeries outLevelZero { get; set; }
[Output("Bulls", LineColor = "Green", PlotType = PlotType.Line, Thickness = 1)]
public IndicatorDataSeries outBulls { get; set; }
[Output("Bears", LineColor = "Red", PlotType = PlotType.Line, Thickness = 1)]
public IndicatorDataSeries outBears { get; set; }
private MomentumOscillator _i1, _i2, _i3, _i4, _i5;
private IndicatorDataSeries _price, _fir, _haclose, _pos, _neg, _pol;
protected override void Initialize()
{
_price = CreateDataSeries();
_fir = CreateDataSeries();
_haclose = CreateDataSeries();
_i1 = Indicators.MomentumOscillator(_price, inpPeriod1);
_i2 = Indicators.MomentumOscillator(_price, inpPeriod2);
_i3 = Indicators.MomentumOscillator(_price, inpPeriod3);
_i4 = Indicators.MomentumOscillator(_price, inpPeriod4);
_i5 = Indicators.MomentumOscillator(_price, inpPeriod5);
_pos = CreateDataSeries();
_neg = CreateDataSeries();
_pol = CreateDataSeries();
}
public override void Calculate(int i)
{
switch(inpPriceType)
{
case enumPriceTypes.Open:
_price[i] = Bars.OpenPrices[i];
break;
case enumPriceTypes.Close:
_price[i] = Bars.ClosePrices[i];
break;
case enumPriceTypes.High:
_price[i] = Bars.HighPrices[i];
break;
case enumPriceTypes.Low:
_price[i] = Bars.LowPrices[i];
break;
case enumPriceTypes.Median:
_price[i] = Bars.MedianPrices[i];
break;
case enumPriceTypes.Typical:
_price[i] = Bars.TypicalPrices[i];
break;
case enumPriceTypes.Weighted:
_price[i] = Bars.WeightedPrices[i];
break;
case enumPriceTypes.FIR:
_price[i] = i>3 ? (Bars.ClosePrices[i] + 2.0 * Bars.ClosePrices[i-1] + 2.0 * Bars.ClosePrices[i-2] + Bars.ClosePrices[i-3]) / 6.0 : Bars.ClosePrices[i];
break;
case enumPriceTypes.HeikinAshiClose:
_price[i] = (Bars.OpenPrices[i] + Bars.ClosePrices[i] + Bars.HighPrices[i] + Bars.LowPrices[i]) / 4;
break;
default:
_price[i] = Bars.ClosePrices[i];
break;
}
_pos[i] = _neg[i] = 0;
_pos[i] += _i1.Result[i] > 100 ? +1 : 0;
_pos[i] += _i2.Result[i] > 100 ? +1 : 0;
_pos[i] += _i3.Result[i] > 100 ? +1 : 0;
_pos[i] += _i4.Result[i] > 100 ? +1 : 0;
_pos[i] += _i5.Result[i] > 100 ? +1 : 0;
_neg[i] += _i1.Result[i] < 100 ? -1 : 0;
_neg[i] += _i2.Result[i] < 100 ? -1 : 0;
_neg[i] += _i3.Result[i] < 100 ? -1 : 0;
_neg[i] += _i4.Result[i] < 100 ? -1 : 0;
_neg[i] += _i5.Result[i] < 100 ? -1 : 0;
_pol[i] = _pos[i] == +5 && _neg[i] == 0 ? +5
: _neg[i] == -5 && _pos[i] == 0 ? -5
: 0;
outBulls[i] = _pos[i];
outBears[i] = _neg[i];
outPol[i] = _pol[i];
outLevelZero[i] = 0;
}
}
public enum enumPriceTypes
{
Open,
Close,
High,
Low,
Median,
Typical,
Weighted,
FIR,
HeikinAshiClose
}
}
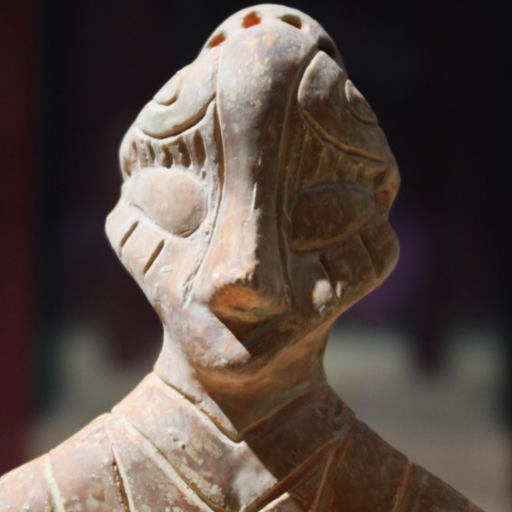
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mMomentum5x.algo
- Rating: 5
- Installs: 711
- Modified: 04/10/2023 10:14
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.