An update for this algorithm is currently pending moderation. Please revisit this page shortly to access the algorithm's latest version.
Description
MAMA Histogram, an occilator accelerator based on the famous MAMA indicator
Have fun, and for any collaboration, contact me !!!
On telegram : https://t.me/nimi012 (direct messaging)
On Discord: https://discord.gg/jNg7BSCh (I advise you to come and see, Money management strategies and Signals Strategies !)
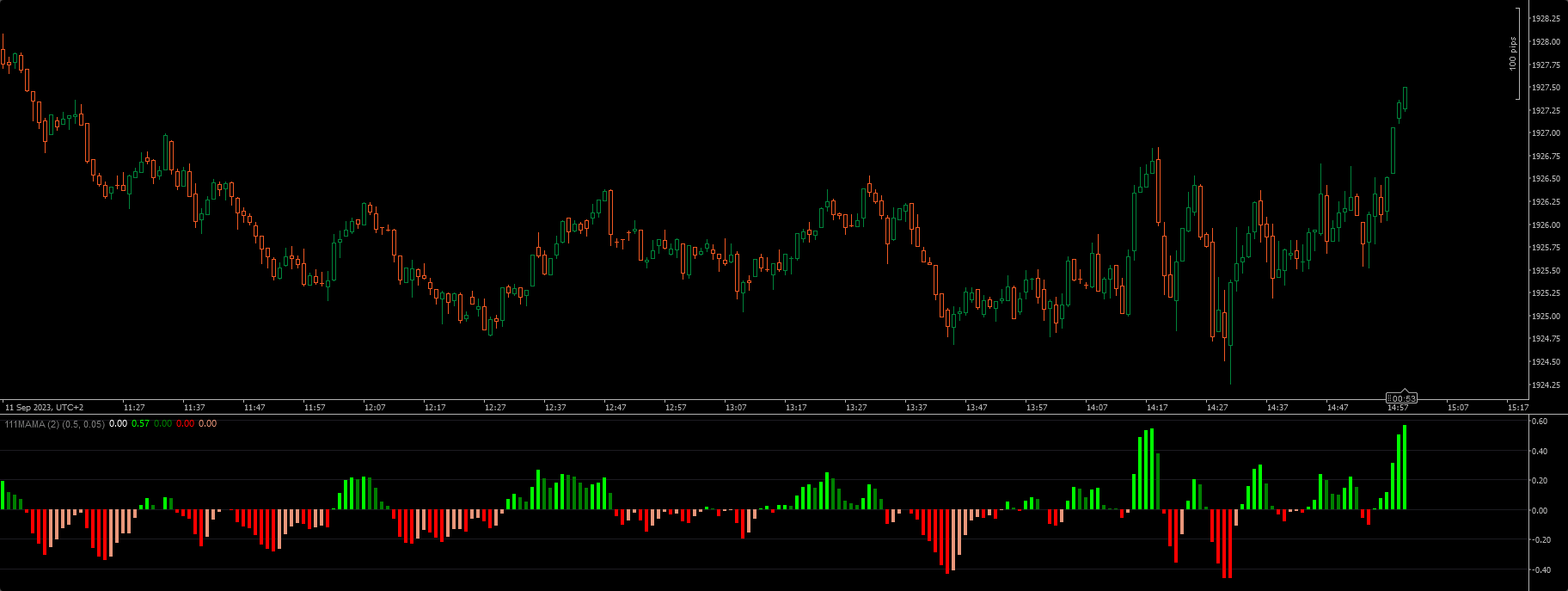
using System;
using cAlgo.API;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = false, AccessRights = AccessRights.None)]
public class Mama : Indicator
{
#region Input Parameters
[Parameter("FastLimit", DefaultValue = 0.5)]
public double FastLimit { get; set; }
[Parameter("SlowLimit", DefaultValue = 0.05)]
public double SlowLimit { get; set; }
[Output("White", PlotType = PlotType.Histogram, LineColor = "White", Thickness = 4)]
public IndicatorDataSeries White { get; set; }
[Output("Up Bullish", PlotType = PlotType.Histogram, LineColor = "Lime", Thickness = 4)]
public IndicatorDataSeries StrongBullish { get; set; }
[Output("Down Bullish", PlotType = PlotType.Histogram, LineColor = "Green", Thickness = 4)]
public IndicatorDataSeries WeakBullish { get; set; }
[Output("Down Bearish", PlotType = PlotType.Histogram, LineColor = "Red", Thickness = 4)]
public IndicatorDataSeries StrongBearish { get; set; }
[Output("Up Bearish", PlotType = PlotType.Histogram, LineColor = "DarkSalmon", Thickness = 4)]
public IndicatorDataSeries WeakBearish { get; set; }
#endregion
#region private fields
private IndicatorDataSeries _alpha;
private IndicatorDataSeries _deltaPhase;
private IndicatorDataSeries _detrender;
private IndicatorDataSeries _i1;
private IndicatorDataSeries _i2;
private IndicatorDataSeries _im;
private IndicatorDataSeries _ji;
private IndicatorDataSeries _jq;
private IndicatorDataSeries _period;
private IndicatorDataSeries _period1;
private IndicatorDataSeries _period2;
private IndicatorDataSeries _phase;
private IndicatorDataSeries _price;
private IndicatorDataSeries _q1;
private IndicatorDataSeries _q2;
private IndicatorDataSeries _re;
private IndicatorDataSeries _smooth;
private IndicatorDataSeries _smoothPeriod;
private IndicatorDataSeries MamaResult;
private IndicatorDataSeries FamaResult;
#endregion
protected override void Initialize()
{
_price = CreateDataSeries();
_smooth = CreateDataSeries();
_detrender = CreateDataSeries();
_period = CreateDataSeries();
_period1 = CreateDataSeries();
_period2 = CreateDataSeries();
_smoothPeriod = CreateDataSeries();
_phase = CreateDataSeries();
_deltaPhase = CreateDataSeries();
_alpha = CreateDataSeries();
_q1 = CreateDataSeries();
_i1 = CreateDataSeries();
_ji = CreateDataSeries();
_jq = CreateDataSeries();
_i2 = CreateDataSeries();
_q2 = CreateDataSeries();
_re = CreateDataSeries();
_im = CreateDataSeries();
MamaResult = CreateDataSeries();
FamaResult = CreateDataSeries();
}
public override void Calculate(int index)
{
if (index <= 5)
{
MamaResult[index] = 0;
FamaResult[index] = 0;
_price[index] = (Bars.HighPrices[index] + Bars.LowPrices[index]) / 2;
_smooth[index] = 0;
_detrender[index] = 0;
_period[index] = 0;
_smoothPeriod[index] = 0;
_phase[index] = 0;
_deltaPhase[index] = 0;
_alpha[index] = 0;
_q1[index] = 0;
_i1[index] = 0;
_ji[index] = 0;
_jq[index] = 0;
_i2[index] = 0;
_q2[index] = 0;
_re[index] = 0;
_im[index] = 0;
return;
}
_price[index] = (Bars.HighPrices[index] + Bars.LowPrices[index]) / 2;
_smooth[index] = (4 * _price[index] + 3 * _price[index - 1] + 2 * _price[index - 2] + _price[index - 3]) / 10;
_detrender[index] = (0.0962 * _smooth[index] + 0.5769 * _smooth[index - 2] - 0.5769 * _smooth[index - 4] - 0.0962 * _smooth[index - 6]) * (0.075 * _period[index - 1] + 0.54);
// Compute InPhase and Quadrature components
_q1[index] = (0.0962 * _detrender[index] + 0.5769 * _detrender[index - 2] - 0.5769 * _detrender[index - 4] - 0.0962 * _detrender[index - 6]) * (0.075 * _period[index - 1] + 0.54);
_i1[index] = _detrender[index - 3];
// Advance the phase of I1 and Q1 by 90 degrees
_ji[index] = (0.0962 * _i1[index] + 0.5769 * _i1[index - 2] - 0.5769 * _i1[index - 4] - 0.0962 * _i1[index - 6]) * (0.075 * _period[index - 1] + 0.54);
_jq[index] = (0.0962 * _q1[index] + 0.5769 * _q1[index - 2] - 0.5769 * _q1[index - 4] - 0.0962 * _q1[index - 6]) * (0.075 * _period[index - 1] + 0.54);
// Phasor addition for 3 bar averaging
_i2[index] = _i1[index] - _jq[index];
_q2[index] = _q1[index] + _ji[index];
// Smooth the I and Q components before applying the discriminator
_i2[index] = 0.2 * _i2[index] + 0.8 * _i2[index - 1];
_q2[index] = 0.2 * _q2[index] + 0.8 * _q2[index - 1];
// Homodyne Discriminator
_re[index] = _i2[index] * _i2[index - 1] + _q2[index] * _q2[index - 1];
_im[index] = _i2[index] * _q2[index - 1] - _q2[index] * _i2[index - 1];
_re[index] = 0.2 * _re[index] + 0.8 * _re[index - 1];
_im[index] = 0.2 * _im[index] + 0.8 * _im[index - 1];
double epsilon = Math.Pow(10, -10);
if (Math.Abs(_im[index] - 0.0) > epsilon && Math.Abs(_re[index] - 0.0) > epsilon)
if (Math.Abs(Math.Atan(_im[index] / _re[index]) - 0.0) > epsilon)
_period[index] = 360 / Math.Atan(_im[index] / _re[index]);
else
_period[index] = 0;
if (_period[index] > 1.5 * _period[index - 1])
_period[index] = 1.5 * _period[index - 1];
if (_period[index] < 0.67 * _period[index - 1])
_period[index] = 0.67 * _period[index - 1];
if (_period[index] < 6)
_period[index] = 6;
if (_period[index] > 50)
_period[index] = 50;
_period[index] = 0.2 * _period[index] + 0.8 * _period[index - 1];
_smoothPeriod[index] = 0.33 * _period[index] + 0.67 * _smoothPeriod[index - 1];
if (Math.Abs(_i1[index] - 0) > epsilon)
_phase[index] = Math.Atan(_q1[index] / _i1[index]);
if (Math.Abs(Math.Atan(_q1[index] / _i1[index]) - 0.0) > epsilon)
_phase[index] = Math.Atan(_q1[index] / _i1[index]);
else
_phase[index] = 0;
_deltaPhase[index] = _phase[index - 1] - _phase[index];
if (_deltaPhase[index] < 1)
_deltaPhase[index] = 1;
_alpha[index] = FastLimit / _deltaPhase[index];
if (_alpha[index] < SlowLimit)
_alpha[index] = SlowLimit;
MamaResult[index] = _alpha[index] * _price[index] + ((1 - _alpha[index]) * MamaResult[index - 1]);
FamaResult[index] = 0.5 * _alpha[index] * MamaResult[index] + (1 - 0.5 * _alpha[index]) * FamaResult[index - 1];
double HistogramValue = MamaResult[index] - FamaResult[index];
double prevHistogramValue = MamaResult[index - 1] - FamaResult[index - 1];
if (HistogramValue > 0)
{
if (prevHistogramValue >= HistogramValue)
{
WeakBullish[index] = HistogramValue;
StrongBullish[index] = 0;
WeakBearish[index] = 0;
StrongBearish[index] = 0;
White[index] = 0;
}
else
{
StrongBullish[index] = HistogramValue;
WeakBullish[index] = 0;
WeakBearish[index] = 0;
StrongBearish[index] = 0;
White[index] = 0;
}
}
else if (HistogramValue < -0)
{
if (HistogramValue <= prevHistogramValue)
{
StrongBearish[index] = HistogramValue;
WeakBearish[index] = 0;
StrongBullish[index] = 0;
WeakBullish[index] = 0;
White[index] = 0;
}
else
{
WeakBearish[index] = HistogramValue;
StrongBearish[index] = 0;
StrongBullish[index] = 0;
WeakBullish[index] = 0;
White[index] = 0;
}
}
else
{
if (HistogramValue <= prevHistogramValue)
{
White[index] = HistogramValue;
WeakBearish[index] = 0;
WeakBearish[index] = 0;
StrongBullish[index] = 0;
WeakBullish[index] = 0;
}
else
{
WeakBearish[index] = 0;
White[index] = HistogramValue;
StrongBearish[index] = 0;
StrongBullish[index] = 0;
WeakBullish[index] = 0;
}
}
}
}
}
YE
YesOrNot
Joined on 10.10.2022 Blocked
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: MAMA HISTOGRAM.algo
- Rating: 5
- Installs: 421
- Modified: 11/09/2023 13:01
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.