An update for this algorithm is currently pending moderation. Please revisit this page shortly to access the algorithm's latest version.
Description
The Price Channel Stop custom indicator displays the current estimated trend based on the channel period and desired risk. Additionally, it presents two levels of values that can serve as stop-loss points for orders that have been opened based on this indicator.
Utilizing the indicator across three different periods (e.g., 20, 50, 200) and observing a change in trend color can serve as a signal for both opening new orders and closing orders that have already been initiated.
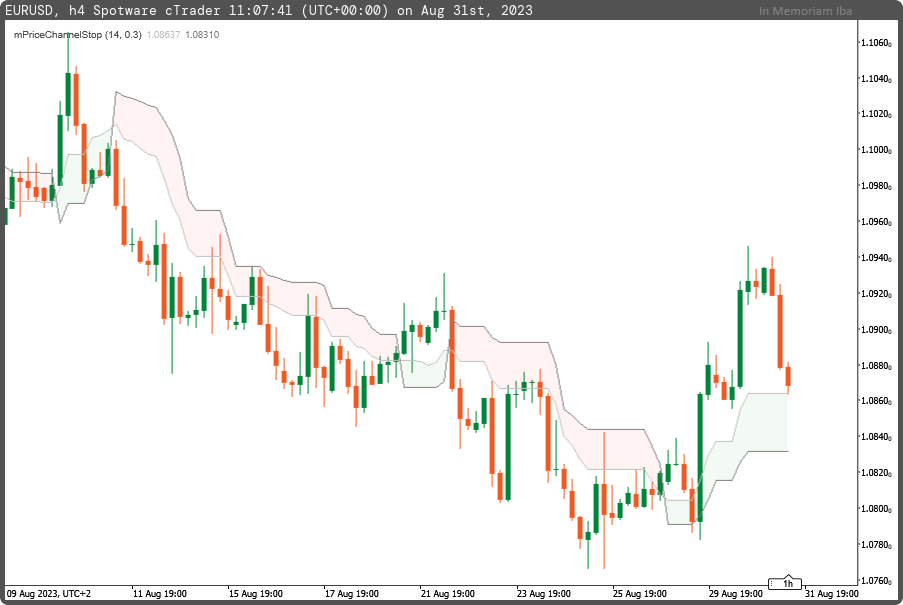
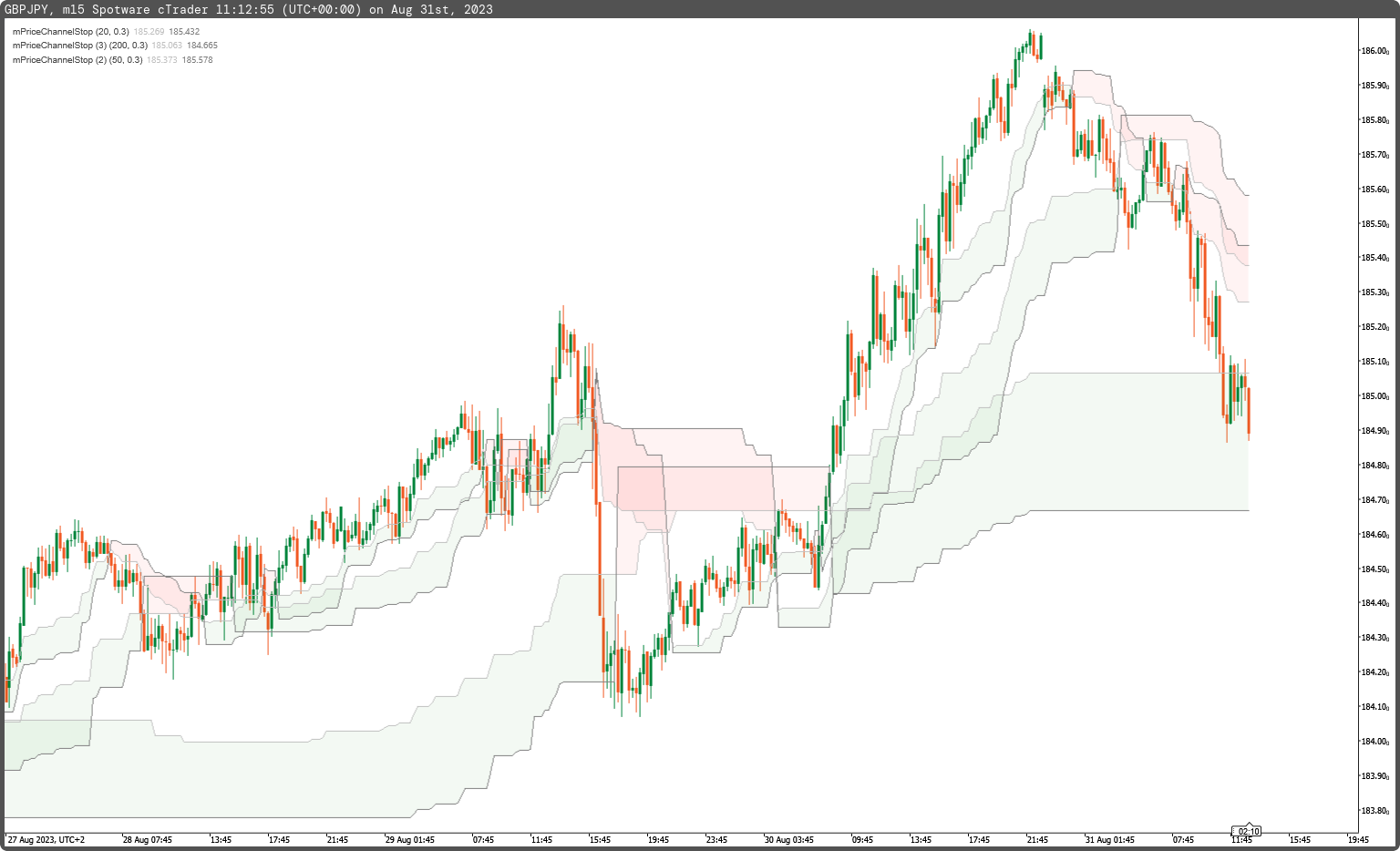
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Cloud("Channel Up", "Channel Down", FirstColor = "Green", SecondColor = "Red", Opacity = 0.05)]
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class mPriceChannelStop : Indicator
{
[Parameter("Channel period (20)", DefaultValue = 14)]
public int inpChannelPeriod { get; set; }
[Parameter("Risk (0.5)", DefaultValue = 0.3, MinValue = 0.01, MaxValue = 0.49)]
public double inpRisk { get; set; }
[Output("Channel Up", LineColor = "Silver", PlotType = PlotType.Line, Thickness = 1)]
public IndicatorDataSeries outChannelUp { get; set; }
[Output("Channel Down", LineColor = "Gray", PlotType = PlotType.Line, Thickness = 1)]
public IndicatorDataSeries outChannelDn { get; set; }
private IndicatorDataSeries _max, _min, _mid, _channelup, _channeldn, _bsmin, _bsmax, _trend;
protected override void Initialize()
{
_max = CreateDataSeries();
_min = CreateDataSeries();
_mid = CreateDataSeries();
_channelup = CreateDataSeries();
_channeldn = CreateDataSeries();
_bsmin = CreateDataSeries();
_bsmax = CreateDataSeries();
_trend = CreateDataSeries();
}
public override void Calculate(int i)
{
_max[i] = i>inpChannelPeriod ? Bars.HighPrices.Maximum(inpChannelPeriod) : Bars.HighPrices[i];
_min[i] = i>inpChannelPeriod ? Bars.LowPrices.Minimum(inpChannelPeriod) : Bars.LowPrices[i];
_mid[i] = (_max[i] + _min[i]) / 2;
_bsmin[i] = _min[i] + (_max[i] - _min[i]) * inpRisk;
_bsmax[i] = _max[i] - (_max[i] - _min[i]) * inpRisk;
_trend[i] = i>0 ? _trend[i-1] : 1;
if(inpRisk>0)
{
if(Bars.ClosePrices[i] > _bsmax[i]) _trend[i] = +1;
if(Bars.ClosePrices[i] < _bsmin[i]) _trend[i] = -1;
}
else
{
if(i>0 && Bars.ClosePrices[i] > _bsmax[i-1]) _trend[i] = +1;
if(i>0 && Bars.ClosePrices[i] < _bsmin[i-1]) _trend[i] = -1;
}
if(_trend[i]>0 && _bsmin[i] < _bsmin[i-1]) _bsmin[i] = _bsmin[i-1];
if(_trend[i]<0 && _bsmax[i] > _bsmax[i-1]) _bsmax[i] = _bsmax[i-1];
_channelup[i] = _mid[i];
_channeldn[i] = _trend[i]>0 ? _bsmin[i] : _bsmax[i];
outChannelUp[i] = _channelup[i];
outChannelDn[i] = _channeldn[i];
}
}
}
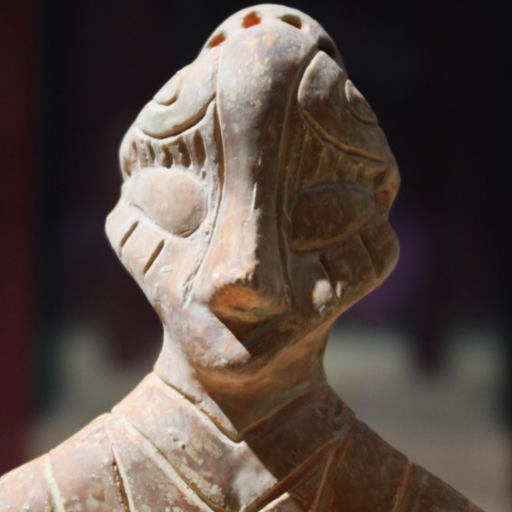
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mPriceChannelStop.algo
- Rating: 5
- Installs: 479
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.