Description
The Ehlers Filter uses a linear distance calculation algorithm. This indicator enables tracking the common trend and trading in its direction. Unlike the non-linear Ehlers Filter, it has a longer delay and allows for more lagged detection of entry and exit points for your positions.
The indicator allows for tracking the common trend and trading in its direction.
You can find the Ehlers Non-Linear Filter indicator here: ctrader.com/algos/indicators/show/3461.
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Indicator(IsOverlay = true, AccessRights = AccessRights.None)]
public class mEhlersFilterlinear : Indicator
{
[Parameter("Period (13)", DefaultValue = 13, MinValue = 2)]
public int inpPeriod { get; set; }
[Parameter("Momentum (5)", DefaultValue = 5, MinValue = 2)]
public int inpMomentum { get; set; }
[Parameter("Data Source (close)", DefaultValue = enumPriceTypes.Close)]
public enumPriceTypes inpPriceType { get; set; }
[Output("Ehlers Filter Linear", LineColor = "FFAA83C6", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 2)]
public IndicatorDataSeries outElhersFilterLinear { get; set; }
private IndicatorDataSeries _price, _delta, _coeff, _coeffprice, _efl;
private MovingAverage _smoothcoeff, _smoothcoeffprice;
protected override void Initialize()
{
_price = CreateDataSeries();
_delta = CreateDataSeries();
_coeff = CreateDataSeries();
_coeffprice = CreateDataSeries();
_efl = CreateDataSeries();
_smoothcoeff = Indicators.MovingAverage(_coeff, inpPeriod, MovingAverageType.Simple);
_smoothcoeffprice = Indicators.MovingAverage(_coeffprice, inpPeriod, MovingAverageType.Simple);
}
public override void Calculate(int i)
{
switch(inpPriceType)
{
case enumPriceTypes.Open:
_price[i] = Bars.OpenPrices[i];
break;
case enumPriceTypes.Close:
_price[i] = Bars.ClosePrices[i];
break;
case enumPriceTypes.High:
_price[i] = Bars.HighPrices[i];
break;
case enumPriceTypes.Low:
_price[i] = Bars.LowPrices[i];
break;
case enumPriceTypes.Median:
_price[i] = Bars.MedianPrices[i];
break;
case enumPriceTypes.Typical:
_price[i] = Bars.TypicalPrices[i];
break;
case enumPriceTypes.Weighted:
_price[i] = Bars.WeightedPrices[i];
break;
default:
_price[i] = Bars.ClosePrices[i];
break;
}
_delta[i] = i>inpMomentum ? _price[i] - _price[i-inpMomentum] : _price[i] - ((Bars.TypicalPrices[i] + Bars.WeightedPrices[i]) / 2);
_coeff[i] = Math.Abs(_delta[i]);
_coeffprice[i] = _coeff[i] * _price[i];
_efl[i] = _smoothcoeffprice.Result[i] / (_smoothcoeff.Result[i] != 0 ? _smoothcoeff.Result[i] : 1);
outElhersFilterLinear[i] = _efl[i];
}
}
public enum enumPriceTypes
{
Open,
Close,
High,
Low,
Median,
Typical,
Weighted
}
}
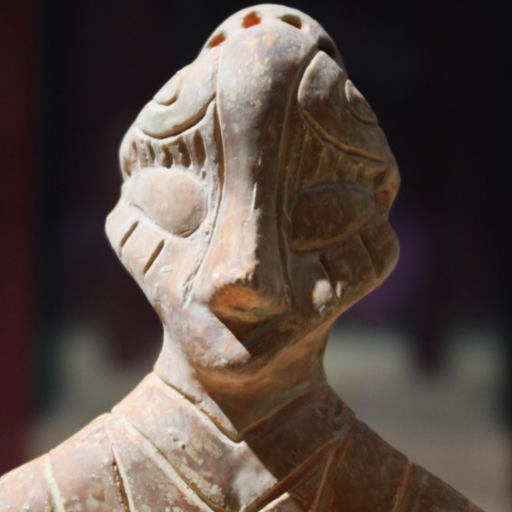
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mEhlersFilterLinear.algo
- Rating: 5
- Installs: 428
- Modified: 07/06/2023 13:31
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.