Description
The Braid Filter oscillator determines the trend based on the maximum and minimum values between 3 different moving averages of the chosen type. The distance between those 2 moving averages must be greater than 40% of the value of ATR (Average True Range) to confirm the trend, indicated by coloring the histogram green for bullish and red for bearish.
If the histogram is above the filter, then the trend is identified; if the histogram is below the filter, then the trend is not identified.
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Levels(0)]
[Indicator(IsOverlay = false, AccessRights = AccessRights.None, AutoRescale = true)]
public class mBraidFilter : Indicator
{
[Parameter("Smooth Type (sma)", DefaultValue = MovingAverageType.Simple)]
public MovingAverageType inpSmoothType { get; set; }
[Parameter("Period Fast (3)", DefaultValue = 3)]
public int inpPeriodFast { get; set; }
[Parameter("Period Mid (7)", DefaultValue = 7)]
public int inpPeriodMid { get; set; }
[Parameter("Period Slow (14)", DefaultValue = 14)]
public int inpPeriodSlow { get; set; }
[Parameter("Pips Min Sep Percent (40)", DefaultValue = 40)]
public double inpPipsMinSepPercent { get; set; }
[Output("Braid Bulls", LineColor = "Green", PlotType = PlotType.Histogram, LineStyle = LineStyle.Solid, Thickness = 3)]
public IndicatorDataSeries outBraidBulls { get; set; }
[Output("Braid Bears", LineColor = "Red", PlotType = PlotType.Histogram, LineStyle = LineStyle.Solid, Thickness = 3)]
public IndicatorDataSeries outBraidBears { get; set; }
[Output("Braid Neutral", LineColor = "Gray", PlotType = PlotType.Histogram, LineStyle = LineStyle.Solid, Thickness = 3)]
public IndicatorDataSeries outBraidNeutral { get; set; }
[Output("Filter", LineColor = "Black", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 2)]
public IndicatorDataSeries outFilter { get; set; }
private MovingAverage _smooth1, _smooth2, _smooth3;
private AverageTrueRange _atr;
private IndicatorDataSeries _min, _max, _diff, _filter;
protected override void Initialize()
{
_smooth1 = Indicators.MovingAverage(Bars.ClosePrices, inpPeriodFast, inpSmoothType);
_smooth2 = Indicators.MovingAverage(Bars.OpenPrices, inpPeriodMid, inpSmoothType);
_smooth3 = Indicators.MovingAverage(Bars.ClosePrices, inpPeriodSlow, inpSmoothType);
_atr = Indicators.AverageTrueRange(14, MovingAverageType.Simple);
_min = CreateDataSeries();
_max = CreateDataSeries();
_diff = CreateDataSeries();
_filter = CreateDataSeries();
}
public override void Calculate(int i)
{
_min[i] = Math.Min(Math.Min(_smooth1.Result[i], _smooth2.Result[i]), _smooth3.Result[i]);
_max[i] = Math.Max(Math.Max(_smooth1.Result[i], _smooth2.Result[i]), _smooth3.Result[i]);
_diff[i] = _max[i] - _min[i];
_filter[i] = _atr.Result[i] * inpPipsMinSepPercent / 100;
outFilter[i] = _filter[i] / Symbol.TickSize;
outBraidBulls[i] = _smooth1.Result[i] > _smooth2.Result[i] && _diff[i] > _filter[i] ? _diff[i] / Symbol.TickSize : 0;
outBraidBears[i] = _smooth2.Result[i] > _smooth1.Result[i] && _diff[i] > _filter[i] ? _diff[i] / Symbol.TickSize: 0;
outBraidNeutral[i] = outBraidBulls[i] == 0 && outBraidBears[i] == 0 ? _diff[i] / Symbol.TickSize : 0;
}
}
}
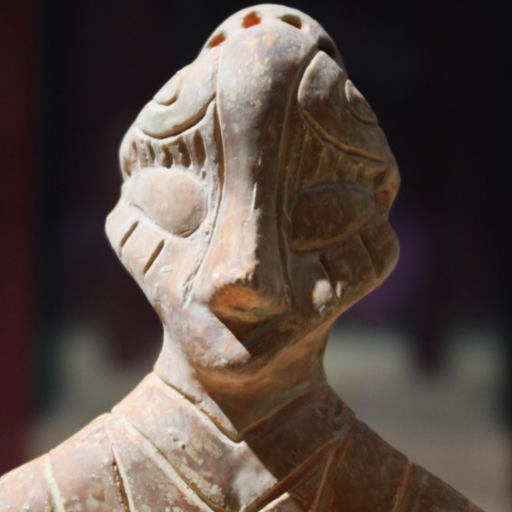
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mBraidFilter.algo
- Rating: 5
- Installs: 644
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.