Description
PDI is a simple signal indicator that measures the price difference between the previous and current bars.
It displays signal marks on the candlesticks where the previous data source price is higher than the current one by a pre-defined price difference. If the price difference is positive, the mark will be placed on the low price of the current candlestick. If it is negative, the mark will be placed on the high price.
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Indicator(IsOverlay = true, AccessRights = AccessRights.None)]
public class mPDI : Indicator
{
[Parameter("Difference (pips)(100)", DefaultValue = 100)]
public int inpDifference { get; set; }
[Parameter("Data Source (close)", DefaultValue = enumPriceTypes.Close)]
public enumPriceTypes inpPriceType { get; set; }
[Output("PDI Up", LineColor = "Red", PlotType = PlotType.Points, Thickness = 5)]
public IndicatorDataSeries outUp { get; set; }
[Output("PDI Down", LineColor = "Green", PlotType = PlotType.Points, Thickness = 5)]
public IndicatorDataSeries outDn { get; set; }
private IndicatorDataSeries _price;
private MovingAverage _ma;
private double diff;
protected override void Initialize()
{
_price = CreateDataSeries();
_ma = Indicators.MovingAverage(_price, 1, MovingAverageType.Simple);
diff = inpDifference * Symbol.PipSize;
}
public override void Calculate(int i)
{
switch(inpPriceType)
{
case enumPriceTypes.Open:
_price[i] = Bars.OpenPrices[i];
break;
case enumPriceTypes.Close:
_price[i] = Bars.ClosePrices[i];
break;
case enumPriceTypes.High:
_price[i] = Bars.HighPrices[i];
break;
case enumPriceTypes.Low:
_price[i] = Bars.LowPrices[i];
break;
case enumPriceTypes.Median:
_price[i] = Bars.MedianPrices[i];
break;
case enumPriceTypes.Typical:
_price[i] = Bars.TypicalPrices[i];
break;
case enumPriceTypes.Weighted:
_price[i] = Bars.WeightedPrices[i];
break;
default:
_price[i] = Bars.ClosePrices[i];
break;
}
outUp[i] = i>1 && _ma.Result[i]-_ma.Result[i-1] > diff ? Bars.LowPrices[i] : double.NaN;
outDn[i] = i>1 && _ma.Result[i]-_ma.Result[i-1] < -diff ? Bars.HighPrices[i] : double.NaN;
}
}
public enum enumPriceTypes
{
Open,
Close,
High,
Low,
Median,
Typical,
Weighted
}
}
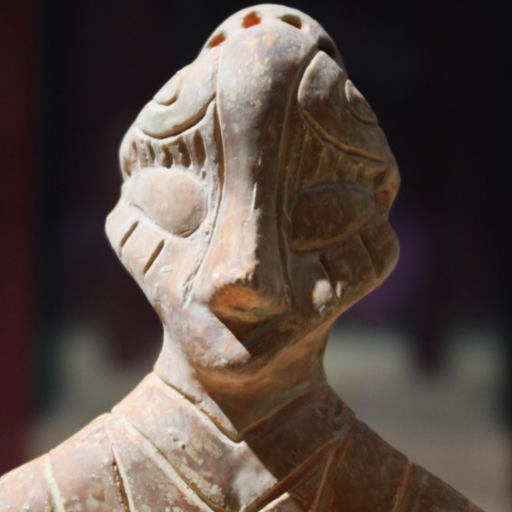
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mPDI.algo
- Rating: 5
- Installs: 473
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.