Description
The Pivot Oscillator is based on three average indicators of the average differences in prices.
The oscillator shows the market sentiment and the market power of typical prices.
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Levels(0)]
[Indicator(AccessRights = AccessRights.None)]
public class mPivotOscillator : Indicator
{
[Parameter("Fast Period (13)", DefaultValue = 13, MinValue = 1)]
public int inpPeriodFast { get; set; }
[Parameter("Medium Period (21)", DefaultValue = 21, MinValue = 2)]
public int inpPeriodMedium { get; set; }
[Parameter("Slow Period (34)", DefaultValue = 34, MinValue = 3)]
public int inpPeriodSlow { get; set; }
[Parameter("Smooth type (sma)", DefaultValue = MovingAverageType.Simple)]
public MovingAverageType inpSmoothType { get; set; }
[Output("Pivot Oscillator", LineColor = "Black", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outPO { get; set; }
[Output("Strong Bullish", PlotType = PlotType.Histogram, LineColor = "ForestGreen", Thickness = 3)]
public IndicatorDataSeries outStrongBullish { get; set; }
[Output("Weak Bullish", PlotType = PlotType.Histogram, LineColor = "LawnGreen", Thickness = 3)]
public IndicatorDataSeries outWeakBullish { get; set; }
[Output("Strong Bearish", PlotType = PlotType.Histogram, LineColor = "Red", Thickness = 3)]
public IndicatorDataSeries outStrongBearish { get; set; }
[Output("Weak Bearish", PlotType = PlotType.Histogram, LineColor = "DarkSalmon", Thickness = 3)]
public IndicatorDataSeries outWeakBearish { get; set; }
private MovingAverage _mafast, _mamedium, _maslow;
private IndicatorDataSeries _d1, _d2, _d3, _tp, _osc;
protected override void Initialize()
{
_mafast = Indicators.MovingAverage(Bars.TypicalPrices, inpPeriodFast, inpSmoothType);
_mamedium = Indicators.MovingAverage(Bars.TypicalPrices, inpPeriodMedium, inpSmoothType);
_maslow = Indicators.MovingAverage(Bars.TypicalPrices, inpPeriodSlow, inpSmoothType);
_d1 = CreateDataSeries();
_d2 = CreateDataSeries();
_d3 = CreateDataSeries();
_tp = CreateDataSeries();
_osc = CreateDataSeries();
}
public override void Calculate(int i)
{
_d1[i] = _mafast.Result[i] - _maslow.Result[i];
_d2[i] = _mamedium.Result[i] - _maslow.Result[i];
_d3[i] = _mafast.Result[i] - _mamedium.Result[i];
_tp[i] = (Bars.HighPrices[i] + Bars.LowPrices[i] + Bars.ClosePrices[i]) / 3;
_osc[i] = _tp[i] != 0 ? ((_d1[i] + _d2[i] + _d3[i]) / _tp[i]) / Symbol.PipSize : 0;
outPO[i] = _osc[i];
outStrongBullish[i] = _osc[i] > 0 && _osc[i] >= _osc[i-1] ? _osc[i] : double.NaN;
outWeakBullish[i] = _osc[i] > 0 && _osc[i] < _osc[i-1] ? _osc[i] : double.NaN;
outStrongBearish[i] = _osc[i] < 0 && _osc[i] <= _osc[i-1] ? _osc[i] : double.NaN;
outWeakBearish[i] = _osc[i] < 0 && _osc[i] > _osc[i-1] ? _osc[i] : double.NaN;
}
}
}
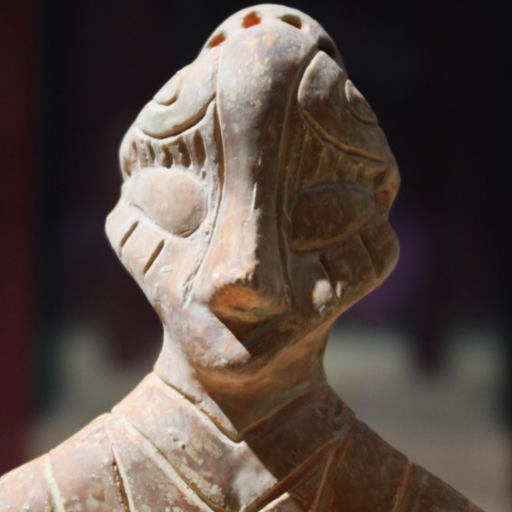
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mPivotOscillator.algo
- Rating: 5
- Installs: 671
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.