Description
The Ehlers Decycler Oscillator is a technical indicator proposed by John F. Ehlers. Its calculation involves eliminating very low-frequency components from Ehlers Simple Decycler and transforming the output into the oscillator. Low-frequency components are eliminated using the half-period Ehlers Highpass filter.
The oscillator itself is equal to the ratio of the high-pass output data to price, multiplied by a coefficient. This coefficient is equal to 1 by default, however, you might want to adjust it if you use several instances of the oscillator on the same subgraph. Crossovers of oscillators with different coefficients might help identifying important trend reversals.
//John F.Ehlers Decycler Oscillator
using System;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Levels(0)]
[Indicator(AccessRights = AccessRights.None)]
public class mDecyclerOscillator : Indicator
{
[Parameter("HP Period (125)", DefaultValue = 125)]
public int inpPeriodHP { get; set; }
[Parameter("Coefficient (1.0)", DefaultValue = 1.0)]
public double inpCoefficient { get; set; }
[Output("Decycler Oscillator", IsHistogram = false, LineColor = "Black", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outDO { get; set; }
double angle1, angle2, alpha1, alpha2;
private IndicatorDataSeries _hp, _decycle, _decycle2;
private double a1, a2, a3, a4, b1, b2, b3, b4, pr2, pr3, pr4, pr5;
protected override void Initialize()
{
angle1 = 1.414 * 3.14159265358979323846 / inpPeriodHP;
angle2 = 2.0*angle1;
alpha1 = (Math.Cos(angle1) + Math.Sin(angle1) - 1.0) / Math.Cos(angle1);
alpha2 = (Math.Cos(angle2) + Math.Sin(angle2) - 1.0) / Math.Cos(angle2);
a1 = 1.0 - alpha1;
a2 = 1.0 - alpha1 / 2.0;
a3 = a1 * a1;
a4 = a2 * a2;
b1 = 1.0 - alpha2;
b2 = 1.0 - alpha2 / 2.0;
b3 = b1 * b1;
b4 = b2 * b2;
_hp = CreateDataSeries();
_decycle = CreateDataSeries();
_decycle2 = CreateDataSeries();
}
public override void Calculate(int i)
{
pr2 = 2.0 * (i>1 ? Bars.ClosePrices[i-1] : Bars.ClosePrices[i]);
pr3 = Bars.ClosePrices[i] - pr2 + (i>2 ? Bars.ClosePrices[i-2] : Bars.ClosePrices[i]);
_hp[i] = a4 * pr3 + (2.0 * a1 * (i>1 ? _hp[i-1] : 0)) - (a3 * (i>2 ? _hp[i-2] : 0));
_decycle[i] = Bars.ClosePrices[i] - _hp[i];
pr4 = 2.0 * (i>1 ? _decycle[i-1] : 0);
pr5 = _decycle[i] - pr4 + (i>2 ? _decycle[i-2] : 0);
_decycle2[i] = b4 * pr5 + (2.0 * b1 * (i>1 ? _decycle2[i-1] : 0)) - (b3 * (i>2 ? _decycle2[i-2] : 0));
outDO[i] = (Bars.ClosePrices[i]!=0 ? 100.0 * inpCoefficient * _decycle2[i] / Bars.ClosePrices[i] : 0);
}
}
}
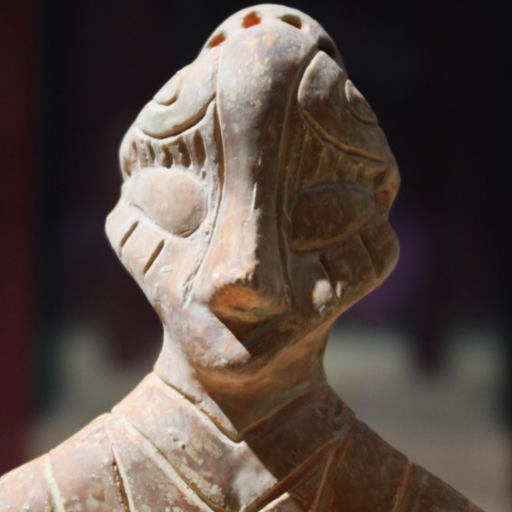
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mDecyclerOscillator.algo
- Rating: 5
- Installs: 499
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.