Description
The Ehlers' Super Smoother Filter is a smoothing technique developed by John F. Ehlers, based on aerospace analog filters.
This filter aims at reducing noise in price data, which appears to be stronger as the high-to-low price swings increase especially when chart is plotted for greater time intervals. In theory, this filter eliminates the noise completely, as opposed to moving averages, e.g., exponential (EMA) which only offers a modest attenuation effect.
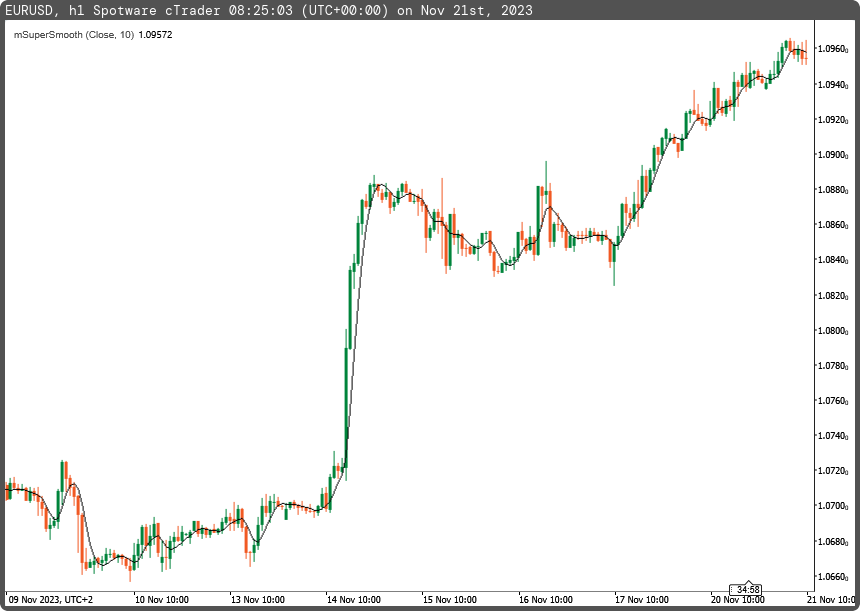
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class mSuperSmooth : Indicator
{
[Parameter("Data Source (close)", DefaultValue = enumPriceTypes.Close)]
public enumPriceTypes inpPriceType { get; set; }
[Parameter("Low Pass Period (10)", DefaultValue = 10)]
public int inpPeriod { get; set; }
[Output("Ehlers Super Smoother", LineColor = "Black", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outSSCF { get; set; }
private IndicatorDataSeries _price, _sscf;
private double a1, b1, c1, c2, c3, pi;
protected override void Initialize()
{
pi = 3.14159265358979;
a1 = Math.Exp(-1.0 * Math.Sqrt(2.0) * pi / (double)inpPeriod);
b1 = 2.0 * a1 * Math.Cos(Math.Sqrt(2.0) * pi / (double)inpPeriod);
c2 = b1;
c3 = -a1 * a1;
c1 = 1.0 - c2 - c3;
_sscf = CreateDataSeries();
_price = CreateDataSeries();
}
public override void Calculate(int i)
{
switch (inpPriceType)
{
case enumPriceTypes.Open:
_price[i] = Bars.OpenPrices[i];
break;
case enumPriceTypes.Close:
_price[i] = Bars.ClosePrices[i];
break;
case enumPriceTypes.High:
_price[i] = Bars.HighPrices[i];
break;
case enumPriceTypes.Low:
_price[i] = Bars.LowPrices[i];
break;
case enumPriceTypes.Median:
_price[i] = Bars.MedianPrices[i];
break;
case enumPriceTypes.Typical:
_price[i] = Bars.TypicalPrices[i];
break;
case enumPriceTypes.Weighted:
_price[i] = Bars.WeightedPrices[i];
break;
default:
_price[i] = Bars.ClosePrices[i];
break;
}
if (i < 3)
_sscf[i] = c1 * _price[i];
else
_sscf[i] = c1 * (_price[i] + _price[i - 1]) / 2.0 + c2 * _sscf[i - 1] + c3 * _sscf[i - 2];
outSSCF[i] = _sscf[i];
}
}
public enum enumPriceTypes
{
Open,
Close,
High,
Low,
Median,
Typical,
Weighted
}
}
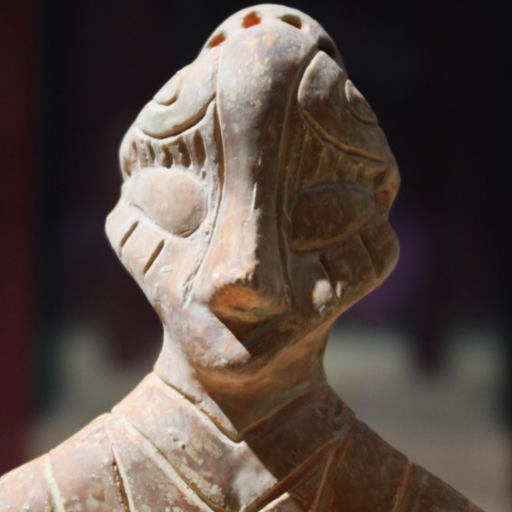
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mSuperSmooth.algo
- Rating: 5
- Installs: 158
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.