Description
This is the Anchored VWAP with Deviation Bands + Clouds used in a strategy Known as Midas Technical Analysis.
The indicator gives you the possibility to plote multiple VWAPs by giving for each of them a different number id in the VWAP Number field.
Grupo CTrader em Portugues -->> https://t.me/ComunidadeCtrader
Make a Donation
- If you liked our work Consider making a Donation to help the Community Grow by Benefiting from more free Indicators and Trading Tools Donation Link
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Cloud("Upper Deviation 3", "Upper Deviation 2")]
[Cloud("Upper Deviation 1", "VWAP")]
[Cloud("Lower Deviation 2", "Lower Deviation 3")]
[Cloud("VWAP", "Lower Deviation 1", FirstColor = "6BFF0000")]
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class AnchoredVWAPDeviationExperto : Indicator
{
[Output("VWAP", LineColor = "Yellow")]
public IndicatorDataSeries VWAP { get; set; }
public enum PriceType
{
Typical = 1,
High = 2,
Low = 3,
Median = 4
}
[Parameter("Arrow Type", Group = "Arrows Settings", DefaultValue = ChartIconType.UpArrow)]
public ChartIconType ArrowType { get; set; }
[Parameter("VWAP Price", Group = "VWAP Calcuation Type", DefaultValue = PriceType.Typical)]
public PriceType VWAPSelectedType { get; set; }
[Parameter("Arrow Color", Group = "Arrows Settings", DefaultValue = Colors.Yellow)]
public Colors ArrowColor { get; set; }
[Parameter("VWAP Number", Group = "Use for Multiple VWAPs", DefaultValue = 0)]
public int VWAPNumber { get; set; }
[Parameter("Icon Style", Group = "Arrows Settings", DefaultValue = ChartIconType.UpArrow)]
public ChartIconType IconStyle { get; set; }
[Output("Upper Deviation 1", LineColor = "897CFC00", Thickness = 1, PlotType = PlotType.DiscontinuousLine)]
public IndicatorDataSeries STD1U { get; set; }
[Output("Upper Deviation 2", LineColor = "897CFC00", Thickness = 1, PlotType = PlotType.DiscontinuousLine)]
public IndicatorDataSeries STD2U { get; set; }
[Output("Upper Deviation 3", LineColor = "897CFC00", Thickness = 1, PlotType = PlotType.DiscontinuousLine)]
public IndicatorDataSeries STD3U { get; set; }
[Output("Lower Deviation 1", LineColor = "6BFF0000", Thickness = 1, PlotType = PlotType.DiscontinuousLine)]
public IndicatorDataSeries STD1D { get; set; }
[Output("Lower Deviation 2", LineColor = "6BFF0000", Thickness = 1, PlotType = PlotType.DiscontinuousLine)]
public IndicatorDataSeries STD2D { get; set; }
[Output("Lower Deviation 3", LineColor = "6BFF0000", Thickness = 1, PlotType = PlotType.DiscontinuousLine)]
public IndicatorDataSeries STD3D { get; set; }
[Parameter("First Multiplier", Group = "Standard Deviations", DefaultValue = 1)]
public double STD1M { get; set; }
[Parameter("Second Multiplier", Group = "Standard Deviations", DefaultValue = 2)]
public double STD2M { get; set; }
[Parameter("Third Multiplier", Group = "Standard Deviations", DefaultValue = 3)]
public double STD3M { get; set; }
public ChartIcon Arrow;
public DateTime IconTime;
public bool IconExists = false;
public int IndexIconStart;
public int ArrowCreated;
public ChartIcon VWAPIcon;
public DataSeries VWAPTPrice;
public bool ShowSTDs = true;
protected override void Initialize()
{
foreach (ChartObject o in Chart.Objects)
{
var VWAP_Name = "VWAP-Arrow" + VWAPNumber;
if (o.Name == VWAP_Name && o.ObjectType == ChartObjectType.Icon)
{
o.IsInteractive = true;
VWAPIcon = (ChartIcon)o;
IconTime = VWAPIcon.Time;
VWAPIcon.Color = ArrowColor.ToString();
VWAPIcon.IconType = IconStyle;
IconExists = true;
ArrowCreated = 1;
}
}
if (VWAPSelectedType == PriceType.Typical)
VWAPTPrice = Bars.TypicalPrices;
else if (VWAPSelectedType == PriceType.High)
VWAPTPrice = Bars.HighPrices;
else if (VWAPSelectedType == PriceType.Low)
VWAPTPrice = Bars.LowPrices;
else if (VWAPSelectedType == PriceType.Median)
VWAPTPrice = Bars.MedianPrices;
if (!IconExists)
{
IndexIconStart = Chart.FirstVisibleBarIndex + ((Chart.LastVisibleBarIndex - Chart.FirstVisibleBarIndex) / 2);
}
Chart.ObjectUpdated += OnChartObjectUpdated;
}
public override void Calculate(int index)
{
//******************** Create VWAP Arrow One *********************************//
if (index == IndexIconStart && IconExists == false)
{
var VWAP_Name = "VWAP-Arrow" + VWAPNumber;
double pr = Bars.LowPrices[index] - (Bars.HighPrices[index] - Bars.LowPrices[index]);
Arrow = Chart.DrawIcon(VWAP_Name, ChartIconType.UpArrow, index, pr, Color.Gold);
Arrow.IsInteractive = true;
Arrow.Color = ArrowColor.ToString();
ArrowCreated = 2;
}
//************************ Draw VWAP One Line **********************************//
if (IsLastBar && ArrowCreated == 2)
{
double sumP = 0.0;
double sumVl = 0.0;
for (int j = 0; j < Chart.BarsTotal; j++)
{
if (j >= IndexIconStart)
{
sumP += VWAPTPrice[j] * Bars.TickVolumes[j];
sumVl += Bars.TickVolumes[j];
VWAP[j] = sumP / sumVl;
}
if (ShowSTDs)
{
//Print("Called");
double squaredErrors = 0;
//int startIndex = Bars.OpenTimes.GetIndexByTime(IconTime);
int startIndex = IndexIconStart;
for (int i = j; i >= startIndex; --i)
{
squaredErrors += Math.Pow(Bars.ClosePrices[i] - VWAP[j], 2);
}
squaredErrors /= (j - startIndex + 1);
squaredErrors = Math.Sqrt(squaredErrors);
STD1U[j] = squaredErrors * STD1M + VWAP[j];
STD2U[j] = squaredErrors * STD2M + VWAP[j];
STD3U[j] = squaredErrors * STD3M + VWAP[j];
STD1D[j] = VWAP[j] - squaredErrors * STD1M;
STD2D[j] = VWAP[j] - squaredErrors * STD2M;
STD3D[j] = VWAP[j] - squaredErrors * STD3M;
}
}
}
else if (IsLastBar && ArrowCreated == 1)
{
double sumP = 0.0;
double sumVl = 0.0;
for (int j = 0; j < Chart.BarsTotal; j++)
{
if (Bars.OpenTimes[j] >= IconTime)
{
sumP += VWAPTPrice[j] * Bars.TickVolumes[j];
sumVl += Bars.TickVolumes[j];
VWAP[j] = sumP / sumVl;
}
if (ShowSTDs)
{
//Print("Called");
double squaredErrors = 0;
int startIndex = Bars.OpenTimes.GetIndexByTime(IconTime);
for (int i = j; i >= startIndex; --i)
{
squaredErrors += Math.Pow(Bars.ClosePrices[i] - VWAP[j], 2);
}
squaredErrors /= (j - startIndex + 1);
squaredErrors = Math.Sqrt(squaredErrors);
STD1U[j] = squaredErrors * STD1M + VWAP[j];
STD2U[j] = squaredErrors * STD2M + VWAP[j];
STD3U[j] = squaredErrors * STD3M + VWAP[j];
STD1D[j] = VWAP[j] - squaredErrors * STD1M;
STD2D[j] = VWAP[j] - squaredErrors * STD2M;
STD3D[j] = VWAP[j] - squaredErrors * STD3M;
}
}
}
}
void OnChartObjectUpdated(ChartObjectUpdatedEventArgs obj)
{
if (obj.ChartObject.ObjectType == ChartObjectType.Icon)
{
var VWAP_Name = "VWAP-Arrow" + VWAPNumber;
if (obj.ChartObject.Name == VWAP_Name)
{
UpdateIconOne();
}
}
}
private void UpdateIconOne()
{
double sumP = 0.0;
double sumVl = 0.0;
for (int i = 0; i < Chart.BarsTotal; i++)
{
VWAP[i] = double.NaN;
}
ArrowCreated = 1;
if (IconExists)
{
IconTime = VWAPIcon.Time;
VWAPIcon.Color = ArrowColor.ToString();
VWAPIcon.IconType = IconStyle;
}
else
{
IconTime = Arrow.Time;
Arrow.Color = ArrowColor.ToString();
Arrow.IconType = IconStyle;
}
for (int j = 0; j < Chart.BarsTotal; j++)
{
if (Bars.OpenTimes[j] >= IconTime)
{
sumP += VWAPTPrice[j] * Bars.TickVolumes[j];
sumVl += Bars.TickVolumes[j];
VWAP[j] = sumP / sumVl;
}
if (ShowSTDs)
{
//Print("Called");
double squaredErrors = 0;
int startIndex = Bars.OpenTimes.GetIndexByTime(IconTime);
for (int i = j; i >= startIndex; --i)
{
squaredErrors += Math.Pow(Bars.ClosePrices[i] - VWAP[j], 2);
}
squaredErrors /= (j - startIndex + 1);
squaredErrors = Math.Sqrt(squaredErrors);
STD1U[j] = squaredErrors * STD1M + VWAP[j];
STD2U[j] = squaredErrors * STD2M + VWAP[j];
STD3U[j] = squaredErrors * STD3M + VWAP[j];
STD1D[j] = VWAP[j] - squaredErrors * STD1M;
STD2D[j] = VWAP[j] - squaredErrors * STD2M;
STD3D[j] = VWAP[j] - squaredErrors * STD3M;
}
}
}
}
}
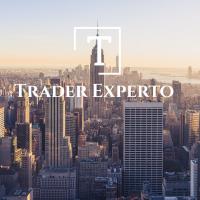
TraderExperto
Joined on 07.06.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Anchored VWAP Experto + Deviation + Clouds.algo
- Rating: 5
- Installs: 3111
- Modified: 13/10/2021 09:54
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.