Warning! This section will be deprecated on February 1st 2025. Please move all your cBots to the cTrader Store catalogue.
Description
This robot validates the slope of the moving averages to indicate the trend and opens orders accordingly.
It uses some configurable moving averages that must be optimized for the role you want to operate.
Indicated papers: EURUSD, GBPUSD, AUDUSD, EURUSD
If you enjoy then donate us any value.
Backtest - 2020-04-10 --- 2020-03-24
Warning! Executing the following cBot may result in loss of funds. Use it at your own risk.
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class TrendMovingAveragev2 : Robot
{
[Parameter("Quantity (Lots)", Group = "Volume", DefaultValue = 0.01, MinValue = 0.01, Step = 0.01)]
public double Quantity { get; set; }
[Parameter("Source", Group = "RSI")]
public DataSeries Source { get; set; }
[Parameter("Periods", Group = "RSI", DefaultValue = 19)]
public int Periods { get; set; }
private RelativeStrengthIndex rsi;
[Parameter("MME Slow", Group = "RSI", DefaultValue = 16)]
public int mmeSlow { get; set; }
[Parameter("MME Fast", Group = "RSI", DefaultValue = 12)]
public int mmeFast { get; set; }
[Parameter("MME Ultra Slow", Group = "RSI", DefaultValue = 196)]
public int mmeUltraSlow { get; set; }
[Parameter("Take Profit", Group = "RSI", DefaultValue = 200)]
public int takeProfit { get; set; }
[Parameter("Stop Loss", Group = "RSI", DefaultValue = 95)]
public int stopLoss { get; set; }
[Parameter("Include Trailing Stop", DefaultValue = false)]
public bool IncludeTrailingStop { get; set; }
[Parameter("Trailing Stop Trigger (pips)", DefaultValue = 20)]
public int TrailingStopTrigger { get; set; }
[Parameter("Trailing Stop Step (pips)", DefaultValue = 10)]
public int TrailingStopStep { get; set; }
private MovingAverage i_MA_ultraSlow;
private MovingAverage i_MA_slow;
private MovingAverage i_MA_fast;
private double volumeInUnits;
protected override void OnTick()
{
if (IncludeTrailingStop)
{
SetTrailingStop();
}
}
protected override void OnStart()
{
volumeInUnits = Symbol.QuantityToVolumeInUnits(Quantity);
rsi = Indicators.RelativeStrengthIndex(Source, Periods);
i_MA_slow = Indicators.MovingAverage(Bars.ClosePrices, mmeSlow, MovingAverageType.Exponential);
i_MA_ultraSlow = Indicators.MovingAverage(Bars.ClosePrices, mmeUltraSlow, MovingAverageType.Exponential);
i_MA_fast = Indicators.MovingAverage(Bars.ClosePrices, mmeFast, MovingAverageType.Exponential);
}
protected override void OnBar()
{
if (rsi.Result.LastValue > 25 && rsi.Result.LastValue < 75)
{
if (i_MA_fast.Result.LastValue > i_MA_slow.Result.LastValue)
{
ExecuteMarketOrder(TradeType.Buy, SymbolName, volumeInUnits, "RSI", stopLoss, takeProfit);
}
else if (i_MA_fast.Result.LastValue < i_MA_slow.Result.LastValue)
{
ExecuteMarketOrder(TradeType.Sell, SymbolName, volumeInUnits, "RSI", stopLoss, takeProfit);
}
}
}
private void SetTrailingStop()
{
var sellPositions = Positions.FindAll("RSI", SymbolName, TradeType.Sell);
foreach (Position position in sellPositions)
{
double distance = position.EntryPrice - Symbol.Ask;
if (distance < TrailingStopTrigger * Symbol.PipSize)
continue;
double newStopLossPrice = Symbol.Ask + TrailingStopStep * Symbol.PipSize;
if (position.StopLoss == null || newStopLossPrice < position.StopLoss)
{
ModifyPosition(position, newStopLossPrice, position.TakeProfit);
}
}
var buyPositions = Positions.FindAll("RSI", SymbolName, TradeType.Buy);
foreach (Position position in buyPositions)
{
double distance = Symbol.Bid - position.EntryPrice;
if (distance < TrailingStopTrigger * Symbol.PipSize)
continue;
double newStopLossPrice = Symbol.Bid - TrailingStopStep * Symbol.PipSize;
if (position.StopLoss == null || newStopLossPrice > position.StopLoss)
{
ModifyPosition(position, newStopLossPrice, position.TakeProfit);
}
}
}
}
}
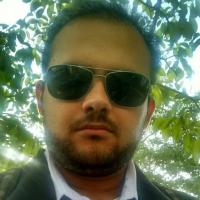
CabralTrader
Joined on 10.03.2020
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Trend Moving Average v2.algo
- Rating: 5
- Installs: 3000
- Modified: 13/10/2021 09:54
Warning! Running cBots downloaded from this section may lead to financial losses. Use them at your own risk.
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.