Description
This is the Tick Volume based on Volume Spread Analisys and Volume Price Analisys
You can find me by joyining this Telegram Group http://t.me/cTraderCoders
Grupo de Telegram Para Brasileiros, Portugueses e todos aqueles que falam portugues:http://t.me/ComunidadeCtrader
Grupo CTrader en Español para Latinos: http://t.me/ComunidadCtrader
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = false, AccessRights = AccessRights.None)]
public class TickVolumeVSA : Indicator
{
[Parameter("MA Length", DefaultValue = 60)]
public int Period { get; set; }
[Output("Moving Average", LineColor = "Gold")]
public IndicatorDataSeries MovingAverageLine { get; set; }
[Output("Up Ultra Volume", LineColor = "Red", PlotType = PlotType.Histogram, Thickness = 4)]
public IndicatorDataSeries UpUltraVolume { get; set; }
[Output("Down Ultra Volume", LineColor = "Red", PlotType = PlotType.Histogram, Thickness = 4)]
public IndicatorDataSeries DownUltraVolume { get; set; }
[Output("Up High Volume", LineColor = "DarkOrange", PlotType = PlotType.Histogram, Thickness = 4)]
public IndicatorDataSeries UpHighVolume { get; set; }
[Output("Down High Volume", LineColor = "DarkOrange", PlotType = PlotType.Histogram, Thickness = 4)]
public IndicatorDataSeries DownHighVolume { get; set; }
[Output("Up Average Volume", LineColor = "Yellow", PlotType = PlotType.Histogram, Thickness = 4)]
public IndicatorDataSeries UpAverageVolume { get; set; }
[Output("Down Average Volume", LineColor = "Yellow", PlotType = PlotType.Histogram, Thickness = 4)]
public IndicatorDataSeries DownAverageVolume { get; set; }
[Output("Up Low Volume", LineColor = "White", PlotType = PlotType.Histogram, Thickness = 4)]
public IndicatorDataSeries UpLowVolume { get; set; }
[Output("Down Low Volume", LineColor = "White", PlotType = PlotType.Histogram, Thickness = 4)]
public IndicatorDataSeries DownLowVolume { get; set; }
[Output("Up Low Activity", LineColor = "Aqua", PlotType = PlotType.Histogram, Thickness = 4)]
public IndicatorDataSeries UpLowActivity { get; set; }
[Output("Down Low Activity", LineColor = "Aqua", PlotType = PlotType.Histogram, Thickness = 4)]
public IndicatorDataSeries DownLowActivity { get; set; }
public IndicatorDataSeries dataUltraVolume { get; set; }
public IndicatorDataSeries dataHighVolume { get; set; }
public IndicatorDataSeries dataAverageVolume { get; set; }
public IndicatorDataSeries dataLowVolume { get; set; }
private SimpleMovingAverage smaVol;
private StandardDeviation stdDev;
protected override void Initialize()
{
smaVol = Indicators.SimpleMovingAverage(Bars.TickVolumes, Period);
stdDev = Indicators.StandardDeviation(Bars.TickVolumes, Period, MovingAverageType.Simple);
}
public override void Calculate(int index)
{
MovingAverageLine[index] = smaVol.Result[index];
double open = Bars.OpenPrices[index];
double high = Bars.HighPrices[index];
double low = Bars.LowPrices[index];
double close = Bars.ClosePrices[index];
double volume = Bars.TickVolumes[index];
double stdbar = (volume - smaVol.Result[index]) / stdDev.Result[index];
if (Bars.ClosePrices[index] >= Bars.OpenPrices[index] && stdbar > 3.5)
{
UpUltraVolume[index] = Bars.TickVolumes[index];
}
else if (Bars.ClosePrices[index] >= Bars.OpenPrices[index] && stdbar > 2.5)
{
UpHighVolume[index] = Bars.TickVolumes[index];
}
else if (Bars.ClosePrices[index] >= Bars.OpenPrices[index] && stdbar > 1)
{
UpAverageVolume[index] = Bars.TickVolumes[index];
}
else if (Bars.ClosePrices[index] >= Bars.OpenPrices[index] && stdbar > -0.5)
{
UpLowVolume[index] = Bars.TickVolumes[index];
}
else if (Bars.ClosePrices[index] >= Bars.OpenPrices[index] && stdbar <= -0.5)
{
UpLowActivity[index] = Bars.TickVolumes[index];
}
if (Bars.ClosePrices[index] < Bars.OpenPrices[index] && stdbar > 3.5)
{
DownUltraVolume[index] = Bars.TickVolumes[index];
}
else if (Bars.ClosePrices[index] >= Bars.OpenPrices[index] && stdbar > 2.5)
{
DownHighVolume[index] = Bars.TickVolumes[index];
}
else if (Bars.ClosePrices[index] < Bars.OpenPrices[index] && stdbar > 1)
{
DownAverageVolume[index] = Bars.TickVolumes[index];
}
else if (Bars.ClosePrices[index] < Bars.OpenPrices[index] && stdbar > -0.5)
{
DownLowVolume[index] = Bars.TickVolumes[index];
}
else if (Bars.ClosePrices[index] < Bars.OpenPrices[index] && stdbar <= -0.5)
{
DownLowActivity[index] = Bars.TickVolumes[index];
}
}
}
}
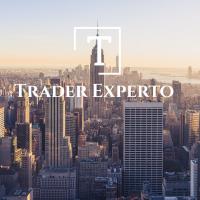
TraderExperto
Joined on 07.06.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Tick Volume VSA.algo
- Rating: 5
- Installs: 4232
- Modified: 13/10/2021 09:54
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.