Description
This Indicator display static rectangle and text.
Donation from free
download from Gumroad
YouTube
Another indicators:
--free--
Upper TF Heikin-ashi Bull Bear
--paid--
MTF OHLCFP Lines Candles Before
cBot:
Auto Calculate Lots V4.0 自動ロット計算
Best Regards
using System;
using cAlgo.API;
using System.Text;
using System.Collections.Generic;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = true, AccessRights = AccessRights.None, AutoRescale = false)]
public class StaticArea : Indicator
{
[Parameter("Price 1", DefaultValue = 0, MinValue = 0)]
public double Line1price { get; set; }
[Parameter("Price 2", DefaultValue = 0, MinValue = 0)]
public double Line2price { get; set; }
[Parameter("Label 1")]
public string lText1 { get; set; }
[Parameter("Label 2")]
public string lText2 { get; set; }
[Parameter("Color", DefaultValue = "Green")]
public string lColor1 { get; set; }
[Parameter("Transparency", DefaultValue = 100, MinValue = 0, MaxValue = 255)]
public int Transparency1 { get; set; }
[Parameter("Linestyle", DefaultValue = "Solid")]
public LineStyle LineStyle { get; set; }
[Parameter("Thickness", DefaultValue = "1", MinValue = 1)]
public int Thickness { get; set; }
[Parameter("Display Prices", DefaultValue = false)]
public bool _price { get; set; }
[Parameter("Area Offset", DefaultValue = -40)]
public int Offset1 { get; set; }
[Parameter("Label Offset", DefaultValue = 1)]
public int lOffset1 { get; set; }
[Parameter("Price Offset", DefaultValue = 1)]
public int pOffset1 { get; set; }
[Parameter("Fill", DefaultValue = true)]
public bool _fill { get; set; }
[Parameter("Visible", DefaultValue = true)]
public bool _visible { get; set; }
public Color alphaColor1;
protected override void Initialize()
{
Color color1 = Color.FromName(lColor1);
alphaColor1 = Color.FromArgb(Transparency1, color1.R, color1.G, color1.B);
}
public override void Calculate(int index)
{
if (_visible)
if (Line1price > 1 && Line2price == 0)
{
StringBuilder sb = new StringBuilder();
Chart.DrawTrendLine("Line1", index, Line1price, index + Offset1, Line1price, alphaColor1, Thickness, LineStyle);
if (_price)
{
sb.AppendFormat("\n{0} " + "(" + Line1price.ToString() + ")", lText1);
sb.AppendFormat("\n\n{0}", lText2);
var text1 = Chart.DrawText("Label1", sb.ToString(), index + lOffset1, Line1price, lColor1);
text1.VerticalAlignment = VerticalAlignment.Center;
text1.HorizontalAlignment = HorizontalAlignment.Right;
}
else
{
sb.AppendFormat("\n{0}", lText1);
sb.AppendFormat("\n\n{0}", lText2);
var text1 = Chart.DrawText("Label1", sb.ToString(), index + lOffset1, Line1price, lColor1);
text1.VerticalAlignment = VerticalAlignment.Center;
text1.HorizontalAlignment = HorizontalAlignment.Right;
}
}
else if (Line1price == 0 && Line2price > 1)
{
StringBuilder sb = new StringBuilder();
Chart.DrawTrendLine("Line2", index, Line2price, index + Offset1, Line2price, alphaColor1, Thickness, LineStyle);
if (_price)
{
sb.AppendFormat("\n{0} " + "(" + Line2price.ToString() + ")", lText1);
sb.AppendFormat("\n\n{0}", lText2);
var text1 = Chart.DrawText("Label1", sb.ToString(), index + lOffset1, Line2price, lColor1);
text1.VerticalAlignment = VerticalAlignment.Center;
text1.HorizontalAlignment = HorizontalAlignment.Right;
}
else
{
sb.AppendFormat("\n{0}", lText1);
sb.AppendFormat("\n\n{0}", lText2);
var text1 = Chart.DrawText("Label2", sb.ToString(), index + lOffset1, Line2price, lColor1);
text1.VerticalAlignment = VerticalAlignment.Center;
text1.HorizontalAlignment = HorizontalAlignment.Right;
}
}
else if (Line1price > 0 && Line2price > 0)
{
StringBuilder sb = new StringBuilder();
var rect = Chart.DrawRectangle("Rect", index, Line1price, index + Offset1, Line2price, alphaColor1, Thickness, LineStyle);
rect.IsFilled = _fill;
if (Line1price == Line2price)
{
if (_price)
{
sb.AppendFormat("\n{0} " + "(" + Line1price.ToString() + ")", lText1);
sb.AppendFormat("\n\n{0}", lText2);
var text1 = Chart.DrawText("Label1", sb.ToString(), index + lOffset1, Line1price, lColor1);
text1.VerticalAlignment = VerticalAlignment.Center;
text1.HorizontalAlignment = HorizontalAlignment.Right;
}
else
{
sb.AppendFormat("\n{0}", lText1);
sb.AppendFormat("\n\n{0}", lText2);
var text1 = Chart.DrawText("Label1", sb.ToString(), index + lOffset1, Line1price, lColor1);
text1.VerticalAlignment = VerticalAlignment.Center;
text1.HorizontalAlignment = HorizontalAlignment.Right;
}
}
else
{
sb.AppendFormat("\n{0}", lText1);
sb.AppendFormat("\n\n{0}", lText2);
var text1 = Chart.DrawText("Label1", sb.ToString(), index + lOffset1, (Line1price + Line2price) / 2, lColor1);
text1.VerticalAlignment = VerticalAlignment.Center;
text1.HorizontalAlignment = HorizontalAlignment.Right;
if (_price)
{
var price1 = Chart.DrawText("PriceLabel1", "(" + Line1price.ToString() + ")", index + pOffset1, Line1price, lColor1);
var price2 = Chart.DrawText("PriceLabel2", "(" + Line2price.ToString() + ")", index + pOffset1, Line2price, lColor1);
price1.HorizontalAlignment = HorizontalAlignment.Right;
price2.HorizontalAlignment = HorizontalAlignment.Right;
if (Line1price < Line2price)
{
price1.VerticalAlignment = VerticalAlignment.Bottom;
price2.VerticalAlignment = VerticalAlignment.Top;
}
else
{
price1.VerticalAlignment = VerticalAlignment.Top;
price2.VerticalAlignment = VerticalAlignment.Bottom;
}
}
}
}
}
}
}
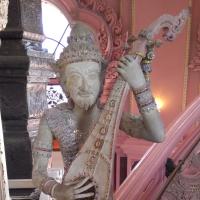
yomm0401
Joined on 11.04.2020
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Static Area.algo
- Rating: 0
- Installs: 2207
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.