Information
Username: | VEI5S6C4OUNT0 |
Member since: | 06 Dec 2022 |
Last login: | 15 Oct 2023 |
Status: | Active |
Activity
Where | Created | Comments |
---|---|---|
Algorithms | 24 | 29 |
Forum Topics | 3 | 8 |
Jobs | 0 | 0 |
Last Algorithm Comments
Also try adding this method.
BeginInvokeOnMainThread(() =>
{
Notifications.PlaySound(@"C:\Windows\Media\Windows Information Bar.wav"); } );
C: \Windows\Media\Windows Information Bar.wav
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC,AccessRights = AccessRights.None)]
public class ATRBand : Robot
{
public DataSeries Source { get; set; }
[Parameter("Start Hour", DefaultValue = 1 , Step = 1)]
public double StartTime { get; set; }
[Parameter("Stop Hour", DefaultValue = 16 , Step = 1)]
public double StopTime { get; set; }
[Parameter("MA", Group = "MA ATR", DefaultValue = 50)]
public int P1 { get; set; }
[Parameter("MA", Group = "MA ATR", DefaultValue = 50)]
public int P2 { get; set; }
[Parameter("Band Width", Group = "MA ATR", DefaultValue = 4)]
public int BW { get; set; }
[Parameter("Per Account", Group = "What", Step = 1)]
public double Q { get; set; }
[Parameter("Stop Loss ", Group = "What",DefaultValue = 10 ,Step = 1)]
public int SL { get; set; }
[Parameter("Take Profit", Group = "What",DefaultValue = 10 ,Step = 1)]
public int TP { get; set; }
[Parameter("MaxPos", Group = "What",DefaultValue = 2, MinValue = 1)]
public int MaxPos { get; set; }
MovingAverage MATR;
AverageTrueRange ATR;
private const string Lab = "ATRBand";
protected override void OnStart()
{
MATR = Indicators.WellesWilderSmoothing(Bars.ClosePrices,P1 );
ATR = Indicators.AverageTrueRange (P2,MovingAverageType.WilderSmoothing);
}
protected override void OnBar()
{
var TIMENOW = Server.Time.TimeOfDay.TotalHours;
var Aspace = ATR.Result.Last(0);
var UT1 = MATR.Result.Last (0) + (Aspace*BW);
var DT1 = MATR.Result.Last (0) - (Aspace*BW);
var BC = Bars.ClosePrices.Last(0);
var BO = Bars.OpenPrices.Last(0);
var Units = Symbol.NormalizeVolumeInUnits(Q * Account.Balance, RoundingMode.Down);
if ((TIMENOW > StartTime && TIMENOW < StopTime) && (Positions.Count < MaxPos))
{
if (BC > DT1 && BO < DT1)
{
ExecuteMarketOrder(TradeType.Buy, SymbolName, Units, Lab,SL,TP);
}
if (BC < UT1 && BO > UT1)
ExecuteMarketOrder(TradeType.Sell, SymbolName, Units, Lab,SL,TP);
}
}
}
}
I'm not sure what you are asking here
This Arrow/Bar is a Bars Close Price Crossing across an ATR Band (50 Wells Wilder plus or minus ATR Result)?
You can just add the system ATR to your robot
And use these values to in your “if” statement ?
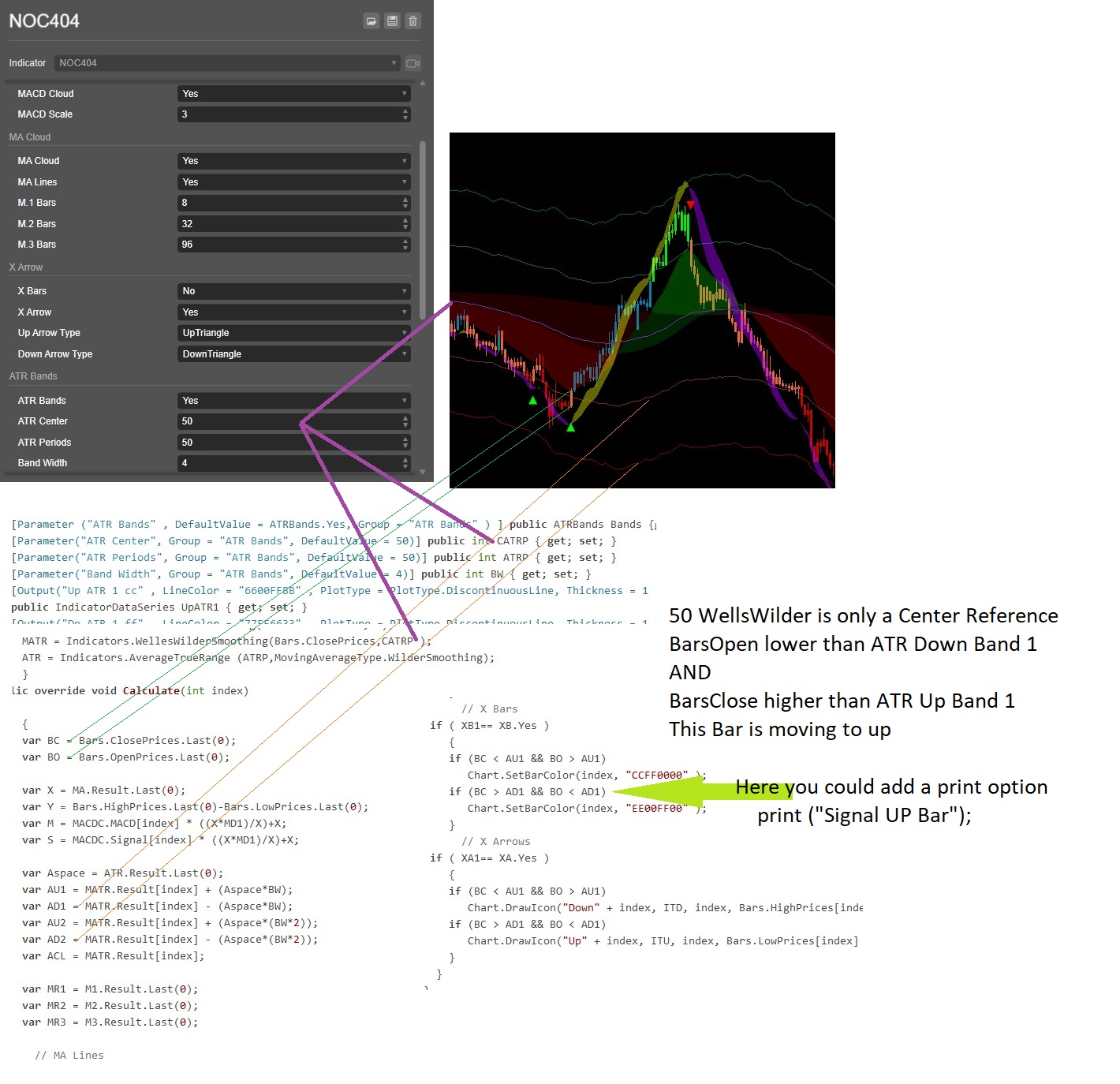
I have tested crossover bots before and they have ups and downs and they don't work as expected with back testing , but I can look into it.
So you are thinking of something like if 3 up arrows then enter buy and set SL and TP at ATR levels? (Should give an average 1:1.4 RR) Look at my other indicator > “3 line Strike P2” < for reference.
Also the ATR Bands here are not as bolinger or rsi but a reference for SL and TP
eg; you could use the inner bands for SL and outer for TP when using Quick Trade Buttons to set them faster.
But I suggest watching it for a while to see how it looks so you can see what to look for during market sessions or what ever appeals to you could work with UpDownTick ?.
Up Date Change to line 7 , 11, 12 for MACD Cloud to make this more palatable and adjustable

I wonder if you can fix a problem with my other algo's
The RSI Stochastic MACD cloud on chart, has to be manualy set for different charts would be good if it would calculate a percentage or something…
I was totaly missing an argument for the clouds
print (FACEPALM)
I fixed it and added option for cloud …(EnumCloud)
Thank you for responding.
I learned a lot of how you coded this and I like it so I made something like it with Expernatial MA and or other MA's as source for ATR however I am having trouble with the cloud part…
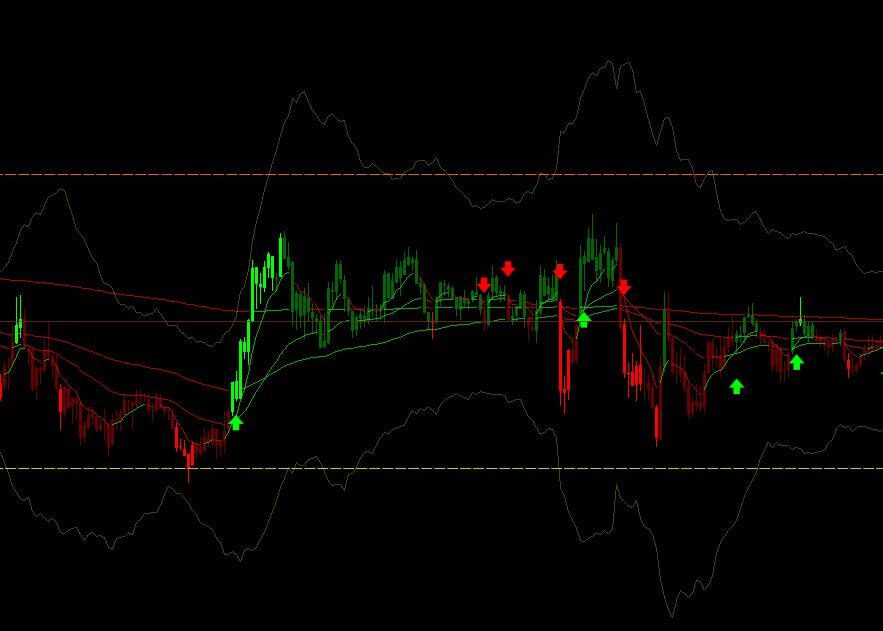
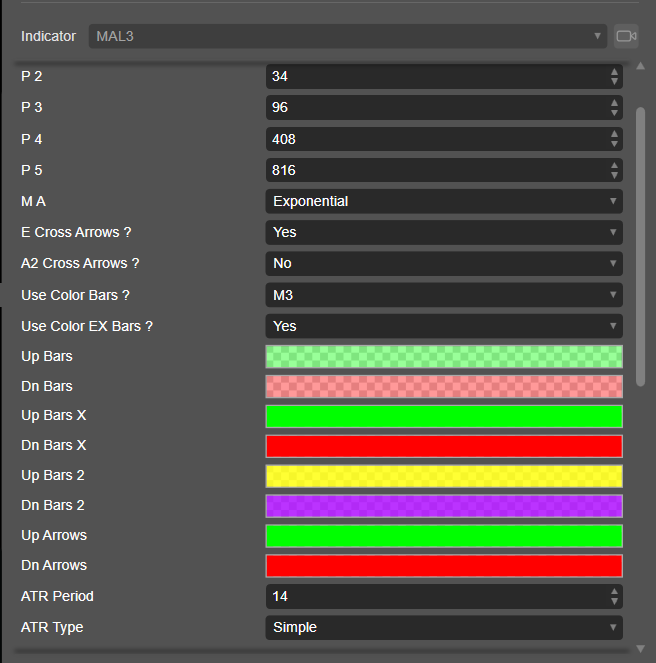
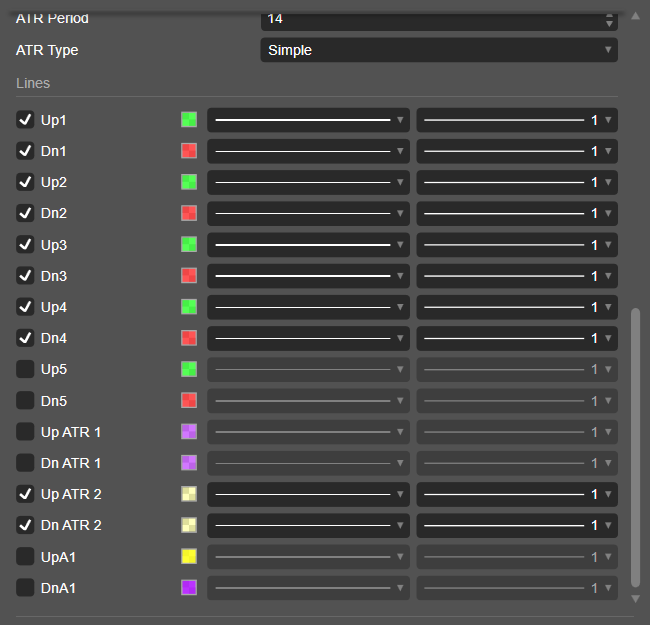
using cAlgo.API;
using cAlgo.API.Indicators;
namespace cAlgo
{
//[Cloud("Up1", "Dn5", Opacity = 1)]
//[Cloud("Up1", "Dn4", Opacity = 1)]
//[Cloud("Up1", "Dn3", Opacity = 1)]
//[Cloud("Up1", "Dn2", Opacity = 1)]
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class MAL3 : Indicator
{
public DataSeries Source { get; set; }
[Parameter("P 1", DefaultValue = 8)]
public int P1 { get; set; }
[Parameter("P 2", DefaultValue = 34)]
public int P2 { get; set; }
[Parameter("P 3", DefaultValue = 96)]
public int P3 { get; set; }
[Parameter("P 4", DefaultValue = 408)]
public int P4 { get; set; }
[Parameter("P 5", DefaultValue = 816)]
public int P5 { get; set; }
[Parameter("M A", DefaultValue = MovingAverageType.Exponential )]
public MovingAverageType MAType { get; set; }
[Output("Up1", LineColor = "AA00FF00", PlotType = PlotType.DiscontinuousLine, Thickness = 1)]
public IndicatorDataSeries Up1 { get; set; }
[Output("Dn1", LineColor = "AAFF0000", PlotType = PlotType.DiscontinuousLine, Thickness = 1)]
public IndicatorDataSeries Dn1 { get; set; }
[Output("Up2", LineColor = "AA00FF00", PlotType = PlotType.DiscontinuousLine, Thickness = 1)]
public IndicatorDataSeries Up2 { get; set; }
[Output("Dn2", LineColor = "AAFF0000", PlotType = PlotType.DiscontinuousLine, Thickness = 1)]
public IndicatorDataSeries Dn2 { get; set; }
[Output("Up3", LineColor = "AA00FF00", PlotType = PlotType.DiscontinuousLine, Thickness = 1)]
public IndicatorDataSeries Up3 { get; set; }
[Output("Dn3", LineColor = "AAFF0000", PlotType = PlotType.DiscontinuousLine, Thickness = 1)]
public IndicatorDataSeries Dn3 { get; set; }
[Output("Up4", LineColor = "AA00FF00", PlotType = PlotType.DiscontinuousLine, Thickness = 1)]
public IndicatorDataSeries Up4 { get; set; }
[Output("Dn4", LineColor = "AAFF0000", PlotType = PlotType.DiscontinuousLine, Thickness = 1)]
public IndicatorDataSeries Dn4 { get; set; }
[Output("Up5", LineColor = "AA00FF00", PlotType = PlotType.DiscontinuousLine, Thickness = 1)]
public IndicatorDataSeries Up5 { get; set; }
[Output("Dn5", LineColor = "AAFF0000", PlotType = PlotType.DiscontinuousLine, Thickness = 1)]
public IndicatorDataSeries Dn5 { get; set; }
MovingAverage M1;
MovingAverage M2;
MovingAverage M3;
MovingAverage M4;
MovingAverage M5;
MovingAverage MATR;
AverageTrueRange ATR;
AverageTrueRange ATRA;
[Parameter("E Cross Arrows ?", DefaultValue = EnumColorArrows.Yes)]
public EnumColorArrows ColorArrowsE { get; set; }
[Parameter("A2 Cross Arrows ?", DefaultValue = EnumColorArrows.Yes)]
public EnumColorArrows ColorArrowsA { get; set; }
[Parameter("Use Color Bars ?", DefaultValue = EnumColorBars.M3)]
public EnumColorBars ColorBars { get; set; }
[Parameter("Use Color EX Bars ?", DefaultValue = EnumColorXBars.Yes)]
public EnumColorXBars ColorBarsX { get; set; }
[Parameter("Up Bars",DefaultValue = "8800FF00")]
public Color UpB { get; set; }
[Parameter("Dn Bars",DefaultValue = "88FF0000")]
public Color DnB { get; set; }
[Parameter("Up Bars X",DefaultValue = "FF00FF00")]
public Color UpBX { get; set; }
[Parameter("Dn Bars X",DefaultValue = "FFFF0000")]
public Color DnBX { get; set; }
[Parameter("Up Bars 2",DefaultValue = "CCFFFF00")]
public Color UpB2 { get; set; }
[Parameter("Dn Bars 2",DefaultValue = "CCAA00FF")]
public Color DnB2 { get; set; }
[Parameter("Up Arrows",DefaultValue = "FF00FF00")]
public Color UpA { get; set; }
[Parameter("Dn Arrows",DefaultValue = "FFFF0000")]
public Color DnA { get; set; }
[Parameter("ATR Period",DefaultValue = 14)]
public int ATRP { get; set; }
[Parameter("ATR Type", DefaultValue = MovingAverageType.Simple )]
public MovingAverageType ATRType { get; set; }
[Output("Up ATR 1", LineColor = "88AA00FF", PlotType = PlotType.DiscontinuousLine, Thickness = 1)]
public IndicatorDataSeries UpATR1 { get; set; }
[Output("Dn ATR 1", LineColor = "88AA00FF", PlotType = PlotType.DiscontinuousLine, Thickness = 1)]
public IndicatorDataSeries DnATR1 { get; set; }
[Output("Up ATR 2", LineColor = "44FFFF00", PlotType = PlotType.DiscontinuousLine, Thickness = 1)]
public IndicatorDataSeries UpATR2 { get; set; }
[Output("Dn ATR 2", LineColor = "44FFFF00", PlotType = PlotType.DiscontinuousLine, Thickness = 1)]
public IndicatorDataSeries DnATR2 { get; set; }
[Output("UpA1", LineColor = "CCFFFF00", PlotType = PlotType.DiscontinuousLine, Thickness = 1)]
public IndicatorDataSeries UpA1 { get; set; }
[Output("DnA1", LineColor = "CCAA00FF", PlotType = PlotType.DiscontinuousLine, Thickness = 1)]
public IndicatorDataSeries DnA1 { get; set; }
public enum EnumColorArrows
{
Yes,No
}
public enum EnumColorBars
{
M1,M2,M3,M4,M5,ATR,OFF
}
public enum EnumColorXBars
{
Yes,No
}
protected override void Initialize()
{
M1 = Indicators.MovingAverage(Source,P1,MAType);
M2 = Indicators.MovingAverage(Source,P2,MAType);
M3 = Indicators.MovingAverage(Source,P3,MAType);
M4 = Indicators.MovingAverage(Source,P4,MAType);
M5 = Indicators.MovingAverage(Source,P5,MAType);
MATR = Indicators.MovingAverage (Source , ATRP , ATRType );
ATR = Indicators.AverageTrueRange(ATRP, ATRType);
ATRA = Indicators.AverageTrueRange(100, MovingAverageType.Exponential);
}
public override void Calculate(int index)
{
var espace = ATRA.Result.Last(0);
var Aspace = ATR.Result.Last(0);
UpATR1[index] = MATR.Result[index] - (Aspace*2);
DnATR1[index] = MATR.Result[index] + (Aspace*2);
UpATR2[index] = MATR.Result[index] - (Aspace*5);
DnATR2[index] = MATR.Result[index] + (Aspace*5);
if (MATR.Result.Last(0) >= MATR.Result.Last(1))
{
UpA1[index] = MATR.Result[index];
}
if (MATR.Result.Last(0) <= MATR.Result.Last(1))
{
DnA1[index] = MATR.Result[index];
}
if (M1.Result.Last(0) >= M1.Result.Last(1))
{
Up1[index] = M1.Result[index];
}
if (M1.Result.Last(0) <= M1.Result.Last(1))
{
Dn1[index] = M1.Result[index];
}
if (M2.Result.Last(0) >= M2.Result.Last(1))
{
Up2[index] = M2.Result[index];
}
if (M2.Result.Last(0) <= M2.Result.Last(1))
{
Dn2[index] = M2.Result[index];
}
if (M3.Result.Last(0) >= M3.Result.Last(1))
{
Up3[index] = M3.Result[index];
}
if (M3.Result.Last(0) <= M3.Result.Last(1))
{
Dn3[index] = M3.Result[index];
}
if (M4.Result.Last(0) >= M4.Result.Last(1))
{
Up4[index] = M4.Result[index];
}
if (M4.Result.Last(0) <= M4.Result.Last(1))
{
Dn4[index] = M4.Result[index];
}
if (M5.Result.Last(0) >= M5.Result.Last(1))
{
Up5[index] = M5.Result[index];
}
if (M5.Result.Last(0) <= M5.Result.Last(1))
{
Dn5[index] = M5.Result[index];
}
if (ColorBars == EnumColorBars.M1)
{
if (Bars.ClosePrices.Last(0) > M1.Result.Last(0))
Chart.SetBarColor(index, UpB);
if (Bars.ClosePrices.Last(0) < M1.Result.Last(0))
Chart.SetBarColor(index, DnB);
}
if (ColorBars == EnumColorBars.M2)
{
if (Bars.ClosePrices.Last(0) > M2.Result.Last(0))
Chart.SetBarColor(index, UpB);
if (Bars.ClosePrices.Last(0) < M2.Result.Last(0))
Chart.SetBarColor(index, DnB);
}
if (ColorBars == EnumColorBars.M3)
{
if (Bars.ClosePrices.Last(0) > M3.Result.Last(0))
Chart.SetBarColor(index, UpB);
if (Bars.ClosePrices.Last(0) < M3.Result.Last(0))
Chart.SetBarColor(index, DnB);
}
if (ColorBars == EnumColorBars.M4)
{
if (Bars.ClosePrices.Last(0) > M4.Result.Last(0))
Chart.SetBarColor(index, UpB);
if (Bars.ClosePrices.Last(0) < M4.Result.Last(0))
Chart.SetBarColor(index, DnB);
}
if (ColorBars == EnumColorBars.M5)
{
if (Bars.ClosePrices.Last(0) > M5.Result.Last(0))
Chart.SetBarColor(index, UpB);
if (Bars.ClosePrices.Last(0) < M5.Result.Last(0))
Chart.SetBarColor(index, DnB);
}
if (ColorBars == EnumColorBars.ATR)
{
if (Bars.ClosePrices.Last(0) > MATR.Result.Last(0))
Chart.SetBarColor(index, UpB2);
if (Bars.ClosePrices.Last(0) < MATR.Result.Last(0))
Chart.SetBarColor(index, DnB2);
}
if (ColorBarsX == EnumColorXBars.Yes)
{
if (Bars.HighPrices.Last(0) > (MATR.Result.Last(0)+Aspace*2))
Chart.SetBarColor(index, UpBX);
if (Bars.LowPrices.Last(0) < (MATR.Result.Last(0)-Aspace*2))
Chart.SetBarColor(index, DnBX);
}
if (ColorArrowsE == EnumColorArrows.Yes)
{
if (M1.Result.Last(0) > M2.Result.Last(0) && M1.Result.Last(1) < M2.Result.Last(1) && M1.Result.Last(1) < M3.Result.Last(1))
{
Chart.DrawIcon("UP" + index, ChartIconType.UpArrow, index, Bars.LowPrices.Last(0) - espace , UpA);
}
if (M1.Result.Last(0) < M2.Result.Last(0) && M1.Result.Last(1) > M2.Result.Last(1) && M1.Result.Last(1) > M3.Result.Last(1))
{
Chart.DrawIcon("DN" + index, ChartIconType.DownArrow, index, Bars.HighPrices.Last(0) + espace , DnA);
}
}
if (ColorArrowsA == EnumColorArrows.Yes)
{
if (Bars.LowPrices.Last(0) < (MATR.Result.Last(0)-Aspace*2))
{
Chart.DrawIcon("UP" + index, ChartIconType.UpArrow, index, Bars.LowPrices.Last(0) - espace , UpA);
}
if (Bars.HighPrices.Last(0) > (MATR.Result.Last(0)+Aspace*2))
{
Chart.DrawIcon("DN" + index, ChartIconType.DownArrow, index, Bars.HighPrices.Last(0) + espace , DnA);
}
}
}
}
}
UpDate the MA's are now selectable ... Sorry my bad
Yes thank you, that is much cleaner
I was trying to get my latest crossover indicater to trigger OnBar so I could use different wav files for different events, when Iasked C-GPT it suggested a sounplayed...previousbar... ex... but this would mostly only work in one direction, and it tryed to build a 16bit player as well (C-GPT dosn't realy understand cAlgo lmao)
Try Net.4 Legacy.
if macd crossover && rsi crossover && stochastic crossover && mafast> maslow
This could expanded in so many ways, Tick volume if (Bid => bid + 10) for higher volume notice
or
if (Bid => Bars.ClosePrices.Last(3) for direction notice
or like the 3 line strick indicator I made to notify reversals ???
I notice almost strait away I can here trades being hedged
bing bing bong = buyers being stopped with sellers
bong bong bing = sellers being stopped with buyers
It works....
or maybe....
eg; var Upsound = GenerateSouns.Wave.Sine.500,200;
... if .....
... Notifications.PlaySound(Upsound);
... ........
That is exactly what I was thinking yes. Thank you.
Does this need the sound files or can we ask , eg PlaySound.SineSave.500khz,200ms; .
I was thinking about how different tones could be used for different charts extra..
if file is needed I can make them with audacity and add / modify to (@"C:\......) ?
Using a sine generate comand then we can set any tone as needed.
using files I would need to duplicate indicator for each chart and set the file in each as needed. ?
Open 5 charts EURUSD, GBPUSD, AUDUSD, EURAUD, GBPUSD on the 1 or 5 min and you see how these markets price against each other like a game of toss the ball.
It's for Scalping as, if you just sit and watch after some time the brain starts to see paterns in market behavior ie; open, sessions, news, and like often during the news there is a raly then the hype dies of and the gap is closes back to original price like music / sports event you can hear the trend ...Maybe
It is an experiment that I wonder if I can here things instead of just staring all day like Pink Floyd...lol
Interesting Code.
I have an idea but I dont know how to do it ( tried ChatGPT failed lol )
a simple Tick indicator with audio eg; up tick "Ping" down tick "Bong" ... got any ideas ?
I have a similar indicator that shoes as clouds and or lines , it also has MACD but the output is set to "signal +50" to line up with RSI and Stochastic 50 but the scale needs to be set to each chart as needed.
Maybe you have some ideas to make this part automatic ?
Nice indicator. I should get you to fix my codes ha ha ha . keep up the great work.
Very Much appreciated.
OMG I'm so sorry yes that's the wrong one .... I will upload the write one now.
Please go to his channel to learn more about how this works
https://www.youtube.com/@TheMovingAverage/videos
I'm not realy that good at coding but this seams to be as close to what he uses on TradingView
if anyone could help update this it would be AWESOME.
The 6 Warnings are in the other indicator P2
This is great I like it.
I wonder if can we add the D line and also a MACD crossover lines or cloud to it as well ?
I found this very useful but it has a problem of trading when the RSI is flat in the middle range. Is there a way to tell it NOT to execute for example RSI 45-55 range ?
Very interesting here's something you may like…
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = false, AutoRescale = false, AccessRights = AccessRights.None)]
public class UpDownTick : Indicator
{
[Output("Up Volume", LineColor = "AAFFFF00", PlotType = PlotType.Histogram, Thickness = 3)]
public IndicatorDataSeries UpVolume { get; set; }
[Output("Down Volume", LineColor = "FFAA00FF", PlotType = PlotType.Histogram, Thickness = 1)]
public IndicatorDataSeries DownVolume { get; set; }
public override void Calculate(int index)
{
if (Bars.ClosePrices[index] >= Bars.OpenPrices[index])
{
UpVolume[index] = Bars.TickVolumes[index] ;
}
if (Bars.ClosePrices[index] <= Bars.OpenPrices[index])
{
DownVolume[index] = Bars.TickVolumes[index] ;
}
}
}
}