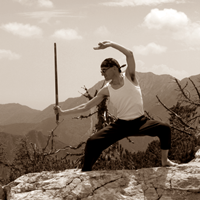
Topics
Forum Topics not found
Replies
Chistabo
02 May 2015, 14:25
RE:
ForexGump said:
atrader: your cbot is pretty good. Do you have something similar that places pending orders instead of market orders? Would place a pending stop order at the next round 5 pip level. Would actually need two cbots: one for pending buy stop and another one for pending sell stop
No need for two cBots, all you need is add another external parameter, i.e. [Parameter("0:Instant 1:Pending", DefaultValue = 0)] public int OrderType { get; set; }, and then add some logic into the code.
Until cTrader / cAlgo implements a method to Find/Get properties of the ChartObjects, the cBot as per original post (with horizontal line movements) is not easy to do; I guess one needs to have own methods to Find/Get ChartObjects properties.
Best regards,
Simon
S love nia
@Chistabo
Chistabo
02 May 2015, 10:01
Example of the code and its use:
Author: prof7bit, file: common_functions.mqh, found at: http://sites.google.com/site/prof7bit/
This code is for MT4; it checks Description field of the horizontal or trendline, and reads the 'command' in the description. Example of use is at the end. Must see original file for code comments.
//| CrossedLineS(tring)
/* Check if a line has been crossed, the line may contain a command string.
string crossedLineS(string command,bool one_shot=true,color clr_active=CLR_NONE,color clr_triggered=CLR_NONE)
{
double last_bid;// see below!
int i;
double price;
string name;
string command_line;
string command_argument;
int type;
if(clr_active==CLR_NONE)clr_active=CLR_CROSSLINE_ACTIVE;
if(clr_triggered==CLR_NONE)clr_triggered=CLR_CROSSLINE_TRIGGERED;
for(i=0;i<ObjectsTotal();i++)
{
name=ObjectName(i);
if(ObjectDescription(name)=="") // is this an object without description (newly created by the user)?
{
if(ObjectGet(name,OBJPROP_COLOR)==clr_active &&
ObjectGet(name,OBJPROP_WIDTH)== WIDTH_CROSSLINE_ACTIVE &&
ObjectGet(name,OBJPROP_STYLE)==STYLE_CROSSLINE_ACTIVE)
{
ObjectSet(name,OBJPROP_COLOR,clr_triggered);
ObjectSet(name,OBJPROP_STYLE,STYLE_CROSSLINE_TRIGGERED);
ObjectSet(name,OBJPROP_WIDTH,WIDTH_CROSSLINE_TRIGGERED);
ObjectSet(name,OBJPROP_PRICE3,0);
}
}
if(StringFind(ObjectDescription(name),command)==0) // is this an object that contains our command?
{
price=0;
type=ObjectType(name);
if(type==OBJ_HLINE)price=ObjectGet(name,OBJPROP_PRICE1); // we only care about certain types of objects
if(type==OBJ_TREND)price=ObjectGetValueByShift(name,0);
if(price>0)
{// we found a line
// ATTENTION! DIRTY HACK! MAY BREAK IN FUTURE VERSIONS OF MT4 - We store the last bid price in the unused PRICE3 field of every line,
// so we can call this function more than once per tick for multiple lines. A static variable would not work here since we could not
// call the function a second time during the same tick
last_bid=ObjectGet(name,OBJPROP_PRICE3);
ObjectSet(name,OBJPROP_COLOR,clr_active);
ObjectSet(name,OBJPROP_STYLE,STYLE_CROSSLINE_ACTIVE);
ObjectSet(name,OBJPROP_WIDTH,WIDTH_CROSSLINE_ACTIVE);
if(last_bid>0) // we have a last_bid value for this line
{
if((Close[0]>=price && last_bid<=price) || (Close[0]<=price && last_bid>=price)) // did price cross this line since the last time we checked this line?
{
command_line=ObjectDescription(name); // extract the argument
command_argument=StringSubstr(command_line,StringLen(command)+1);
if(command_argument=="")command_argument="1";// default argument is "1"
if(one_shot) // make the line triggered if it is a one shot command
{
ObjectSetText(name,"triggered: "+command_line);
ObjectSet(name,OBJPROP_COLOR,clr_triggered);
ObjectSet(name,OBJPROP_STYLE,STYLE_CROSSLINE_TRIGGERED);
ObjectSet(name,OBJPROP_WIDTH,WIDTH_CROSSLINE_TRIGGERED);
ObjectSet(name,OBJPROP_PRICE3,0);
}else{
ObjectSet(name,OBJPROP_PRICE3,Close[0]);
}
return(command_argument);
}
}
ObjectSet(name,OBJPROP_PRICE3,Close[0]); // store current price in the line itself
}
}
}
return("");// command line not crossed,return empty string (false)
}
//| CrossedLineD(ouble)
/* Check if a line has been crossed,the line may contain a numeric value. Call 'crossedLineS()' and cast it into a number */
double crossedLineD(string command,bool one_shot=true,color clr_active=CLR_NONE,color clr_triggered=CLR_NONE)
{
string arg=crossedLineS(command,one_shot,clr_active,clr_triggered);
return(StrToDouble(arg));
}
//| CrossedLine
/* Check if a line has been crossed. Call 'crossedLineS(') and cast it into a bool (true if crossed,otherwise false) */
bool crossedLine(string command,bool one_shot=true,color clr_active=CLR_NONE,color clr_triggered=CLR_NONE)
{
string arg=crossedLineS(command,one_shot,clr_active,clr_triggered);
return(arg != "");
}
// Example of use
if(crossedLine("alert")) {Alert("ALERT line crossed! "+Symbol()); Sleep(300); PlaySound(AlertSound);}
@Chistabo
Chistabo
01 May 2015, 11:51
Totally agreed with Anonymous; also (like MT4) ObjectName (already implemented) and ObjectDescripton, which can show Description text on chart together with the object.
I would also like so see Button object, so I can maximize the chart and trade/modify settings via buttons.
FindObject, and then read its parameters...
cTrader/cAlgo looks nice, I love dark theme! Thumbs up!
Best regards,
Simon
S love nia
@Chistabo
Chistabo
13 Dec 2015, 20:28
RE: RE:
magforex said:
In this case 'int i = MarketSeries.Close.Count - 1' defines a number of iritations that equals all 'close' values found on chart = back to the beginning of available chart data. So, setting this to i.e. 2 would do.
I guess. Have not tried.
Simon
@Chistabo