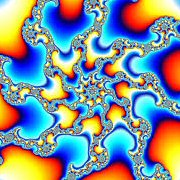
Topics
Replies
deklin
28 May 2015, 03:59
This does not work. How can I do it?
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class NewcBot : Robot { [Parameter(DefaultValue = "B")] public string[3] ABC {"A", "B", "C"} protected override void OnStart() { Print(ABC); } } }
@deklin
deklin
26 May 2015, 14:10
RE:
Sorry, I made a typo above... It should be:
using System; using cAlgo.API; using cAlgo.API.Internals; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class SampleSmartBotRobot : Robot { bool doneToday; protected override void OnStart() { doneToday = false; } protected override void OnTick() { var currentHour = int.Parse(Server.Time.ToString("%H")); if (currentHour >= 8 && doneToday == false) { doneToday = true; Print("This is done once per day at 8:00 am UTC or as close after that time as possible."); } else if (currentHour <= 1) { doneToday = false; } } } }
@deklin
deklin
26 May 2015, 14:08
using System; using cAlgo.API; using cAlgo.API.Internals; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class SampleSmartBotRobot : Robot { bool doneToday; protected override void OnStart() { doneToday = false; } protected override void OnTick() { var currentHour = int.Parse(Server.Time.ToString("%H")); if (currentHour >= 8 && doneToday == false) { doneToday = true; Print("This is done once per day at 8:00 am UTC or as close after that time as possible."); } else if (currentHour <= 1) { doneToday = true; } } } }
@deklin
deklin
03 May 2015, 15:31
Here is an example that adds a martingale approach to your bot, without changing the trading strategy:
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class SampleTrendRobot : Robot { [Parameter("MA Type")] public MovingAverageType MAType { get; set; } [Parameter("Source")] public DataSeries SourceSeries { get; set; } [Parameter("Slow Periods", DefaultValue = 8)] public int SlowPeriods { get; set; } [Parameter("Fast Periods", DefaultValue = 3)] public int FastPeriods { get; set; } [Parameter(DefaultValue = 1000, MinValue = 0)] public int Volume { get; set; } [Parameter("RSIPeriods", DefaultValue = 8)] public int RSIPeriods { get; set; } private RelativeStrengthIndex rsi; private MovingAverage slowMa; private MovingAverage fastMa; private const string label = "Trend Martangle Robot"; private double TopBalance = 0; protected override void OnStart() { TopBalance = Account.Balance; fastMa = Indicators.MovingAverage(SourceSeries, FastPeriods, MAType); slowMa = Indicators.MovingAverage(SourceSeries, SlowPeriods, MAType); rsi = Indicators.RelativeStrengthIndex(SourceSeries, RSIPeriods); } protected override void OnTick() { if(Account.Balance>TopBalance) TopBalance=Account.Balance; var longPosition = Positions.Find(label, Symbol, TradeType.Buy); var shortPosition = Positions.Find(label, Symbol, TradeType.Sell); var position = Positions.Find(label, Symbol); var currentSlowMa = slowMa.Result.Last(0); var currentFastMa = fastMa.Result.Last(0); var previousSlowMa = slowMa.Result.Last(1); var previousFastMa = fastMa.Result.Last(1); double v = Volume; if (position != null && Account.Balance < TopBalance) v = position.Volume * 2; if (previousSlowMa > previousFastMa && currentSlowMa <= currentFastMa && rsi.Result.LastValue < 30 && longPosition == null) { if (shortPosition != null) { ClosePosition(shortPosition); } ExecuteMarketOrder(TradeType.Buy, Symbol, Symbol.NormalizeVolume(v), label); } else if (previousSlowMa < previousFastMa && currentSlowMa >= currentFastMa && rsi.Result.LastValue > 70 && shortPosition == null) { if (longPosition != null) { ClosePosition(longPosition); } ExecuteMarketOrder(TradeType.Sell, Symbol, Symbol.NormalizeVolume(v), label); } } } }
Here is an example of a martingale approach to your bot with built-in stop loss and take profit levels:
// Test on USDCAD & EURUSD & AUDUSD using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class SampleTrendRobot : Robot { [Parameter("MA Type")] public MovingAverageType MAType { get; set; } [Parameter("Source")] public DataSeries SourceSeries { get; set; } [Parameter("Stop Loss", DefaultValue = 50)] public int StopLoss { get; set; } [Parameter("Take Profit", DefaultValue = 60)] public int TakeProfit { get; set; } [Parameter("Slow Periods", DefaultValue = 8)] public int SlowPeriods { get; set; } [Parameter("Fast Periods", DefaultValue = 3)] public int FastPeriods { get; set; } [Parameter(DefaultValue = 1000, MinValue = 0)] public int Volume { get; set; } [Parameter("RSIPeriods", DefaultValue = 8)] public int RSIPeriods { get; set; } private RelativeStrengthIndex rsi; private MovingAverage slowMa; private MovingAverage fastMa; private const string label = "Trend Martangle Robot"; protected override void OnStart() { Positions.Closed += OnPositionsClosed; fastMa = Indicators.MovingAverage(SourceSeries, FastPeriods, MAType); slowMa = Indicators.MovingAverage(SourceSeries, SlowPeriods, MAType); rsi = Indicators.RelativeStrengthIndex(SourceSeries, RSIPeriods); } protected override void OnTick() { var longPosition = Positions.Find(label, Symbol, TradeType.Buy); var shortPosition = Positions.Find(label, Symbol, TradeType.Sell); var currentSlowMa = slowMa.Result.Last(0); var currentFastMa = fastMa.Result.Last(0); var previousSlowMa = slowMa.Result.Last(1); var previousFastMa = fastMa.Result.Last(1); if (previousSlowMa > previousFastMa && currentSlowMa <= currentFastMa && rsi.Result.LastValue < 30 && longPosition == null) { if (shortPosition != null) ClosePosition(shortPosition); ExecuteMarketOrder(TradeType.Buy, Symbol, Symbol.NormalizeVolume(Volume), label, StopLoss, TakeProfit); } else if (previousSlowMa < previousFastMa && currentSlowMa >= currentFastMa && rsi.Result.LastValue > 70 && shortPosition == null) { if (longPosition != null) ClosePosition(longPosition); ExecuteMarketOrder(TradeType.Sell, Symbol, Symbol.NormalizeVolume(Volume), label, StopLoss, TakeProfit); } } private void OnPositionsClosed(PositionClosedEventArgs args) { var position = args.Position; if (position.GrossProfit < 0) { TradeType tt = TradeType.Sell; if(position.TradeType==TradeType.Sell) tt = TradeType.Buy; ExecuteMarketOrder(tt, Symbol, Symbol.NormalizeVolume(position.Volume * 2), "Martingale", StopLoss, TakeProfit); } } } }
I tested both of these with the USDCAD pair. I would not actually use either of these in a live-trading environment.
@deklin
deklin
11 Jan 2015, 01:31
RE: Daily pip range from 12 days ago
Thanks, HJozan!
I have posted a related question here:
/forum/cbot-support/4212
@deklin
deklin
09 Jan 2015, 19:49
Does the "Trading Session" information take holidays into consideration or does it only take the day of the week and time of day into account?
For example, at 14:00 UTC it says that London and New York sessions are both open. What if it is a bank holiday in London but not a holiday in New York? Would cTrader reflect that?
@deklin
deklin
06 Jan 2015, 00:03
Re: help me,my code error
This works with no errors:
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class NewcBot : Robot { [Parameter(DefaultValue = 0.0)] public double Parameter { get; set; } [Parameter("take profit(pips)", DefaultValue = 10, MinValue = 1)] public int takeProfit { get; set; } protected override void OnPendingOrderCreated(PendingOrder newOrder) { Print("Pending order with ID {0} was created.", newOrder.Id); } protected override void OnPositionClosed(Position closedPosition) { } protected override void OnTick() { if (1 == 2) return; } protected override void OnStop() { } } }
If you want it to do something else you will have to be more specific.
@deklin
deklin
05 Jan 2015, 23:53
Re: Please convert those MT4 codes to cAlgo
Instead of TimeCurrent() use Server.Time
Print ( Server.Time );
See:
/api/reference/internals/iserver/time
The time can be formatted using C#
http://msdn.microsoft.com/en-us/library/8kb3ddd4(v=vs.110).aspx
---
Instead of GetTickCount() I would just start a variable at 0 and add to it every tick.
Here is an example that incorporates both of these solutions:
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class simpleTest : Robot { long tickCounter = 0; [Parameter("TotalTicsToStopAt", DefaultValue = 10)] protected override void OnStart() { Print("Current Time: " + Server.Time); Print("Current Time with Custom Formatting: " + Server.Time.ToString("ddd, MMM d yyyy H':'mm':'ss tt")); } protected override void OnTick() { Print("Tick Count: " + tickCounter++); } protected override void OnStop() { } } }
@deklin
deklin
04 Jan 2015, 21:12
This:
Print(Environment.GetFolderPath(Environment.SpecialFolder.ApplicationData));
Returns this:
C:\Users\7\AppData\Roaming
However, the files I want to refference are actually in a subfolder:
C:\Users\7\AppData\Roaming\BrokerName cAlgo\
How can I get the full path of the actual folder where the associated
@deklin
deklin
04 Jan 2015, 17:47
How can I get the daily pip range from 12 days ago?
This seems to get the daily pip range from the most recent trading day:
MarketSeries m = MarketData.GetSeries(Symbol, TimeFrame.Daily); Print("Daily Pip Range: " + (m.High.LastValue - m.Low.LastValue)/Symbol.PipSize );
@deklin
deklin
01 Jan 2015, 02:37
How can I backtest a bot that randomly buys pairs? The following bot buys pairs when it is run but not when I try to backtest it.
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class simpleTest : Robot { string[] pPairs = new string[] { "EURUSD", "GBPUSD", "EURJPY", "USDJPY", "AUDUSD", "USDCHF", "GBPJPY", "USDCAD", "EURGBP", "EURCHF", "AUDJPY", "NZDUSD", "AUDNZD" }; protected override void OnStart() { } protected override void OnTick() { if(Positions.Count < 20 ) { Random rnd = new Random(); Symbol sSymbol; sSymbol = MarketData.GetSymbol(pPairs[rnd.Next(0, pPairs.Length)]); ExecuteMarketOrder(TradeType.Buy, sSymbol, 100000, sSymbol.Code, 20, 30); } } protected override void OnStop() { } } }
@deklin
deklin
01 Jul 2015, 01:44
What is wrong with this? It does not place the order. It returns an error: No Money!
@deklin