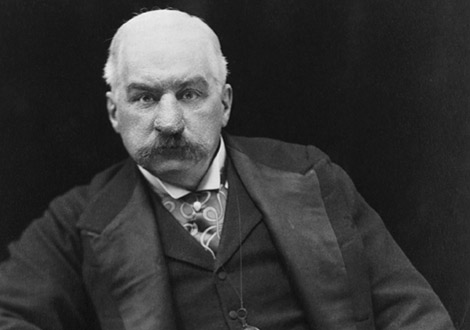
Topics
Replies
bosma
11 May 2015, 19:41
It would be great if cAlgo would override System Time while back-testing to equal the time it would have been. I'm not positive what you are trying to do in OnPositionClosed, so I don't get your functionality completely, but something like this would work (have to use 1min charts).
// ------------------------------------------------------------------------------------------------- // // This code is a cAlgo API sample. // // This cBot is intended to be used as a sample and does not guarantee any particular outcome or // profit of any kind. Use it at your own risk // // The "Sample Martingale cBot" creates a random Sell or Buy order. If the Stop loss is hit, a new // order of the same type (Buy / Sell) is created with double the Initial Volume amount. The cBot will // continue to double the volume amount for all orders created until one of them hits the take Profit. // After a Take Profit is hit, a new random Buy or Sell order is created with the Initial Volume amount. // // ------------------------------------------------------------------------------------------------- using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class SampleMartingalecBot : Robot { [Parameter("Initial Volume", DefaultValue = 10000, MinValue = 0)] public int InitialVolume { get; set; } [Parameter("Stop Loss", DefaultValue = 10)] public int StopLoss { get; set; } [Parameter("Take Profit", DefaultValue = 10)] public int TakeProfit { get; set; } [Parameter("delay minute", DefaultValue = 1)] public int Minute { get; set; } private Random random = new Random(); private DateTime StartTimer; private bool OpenAfterTimer = false; protected override void OnStart() { Positions.Closed += OnPositionsClosed; } protected override void OnBar() { // OpenAfterTimer is only true only when a position has been closed (and before another opened) if (OpenAfterTimer) { // If Minute number of minutes has passed if (MarketSeries.OpenTime.LastValue.Subtract(StartTimer).TotalMinutes >= Minute) ExecuteOrder(InitialVolume, GetRandomTradeType()); } else { // Remember when we've opened our position StartTimer = MarketSeries.OpenTime.LastValue; OpenAfterTimer = false; ExecuteOrder(InitialVolume, GetRandomTradeType()); } } private void ExecuteOrder(long volume, TradeType tradeType) { var result = ExecuteMarketOrder(tradeType, Symbol, volume, "Martingale", StopLoss, TakeProfit); if (result.Error == ErrorCode.NoMoney) Stop(); } private void OnPositionsClosed(PositionClosedEventArgs args) { var position = args.Position; { if (position.Label != "Martingale" || position.SymbolCode != Symbol.Code) return; if (position.Pips > 0) OpenAfterTimer = true; if (position.GrossProfit > 0) { ExecuteOrder(InitialVolume, GetRandomTradeType()); } else { ExecuteOrder((int)position.Volume * 2, position.TradeType); } } } private TradeType GetRandomTradeType() { return random.Next(2) == 0 ? TradeType.Buy : TradeType.Sell; } } }
@bosma
bosma
05 May 2015, 23:56
RE: RE: RE:
AccessRights = AccessRights.FileSystem
to adhere to principle of least privilege.
benco5000 said:
Fixed: AccessRights was set to AccessRights.none changed to AccessRights.FullAccess and it works now.
namespace cAlgo
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)]
public class myTest : Robot
{...
benco5000 said:
When I run the Writing to File example I get an error message:
Backtesting Started
Crashed OnStart with Security Exception: Request for the permission of the type 'System.Security.Permissions.FileIOPermission, mscorlib, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089' failed.
Backtesting Stopped
Does anyone have any insight?
Thanks in advance.
@bosma
bosma
17 May 2015, 10:05
RE:
I would like some customization to how the optimization algorithm works. Stopping after a given time limit or a certain number of passes (given no improvement in fitness). I wouldn't say it's pointless as is, because you can never be sure you've found the best possible parameters unless brute forcing.
jsuhncc said:
@bosma