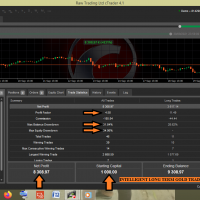
Topics
Replies
danieleyube
07 Feb 2022, 14:52
RE: RE:
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class NewcBot : Robot
{
[Parameter("Trade Size", DefaultValue = 1000, MinValue = 0.01)]
public double Size { get; set; }
[Parameter("Stop Loss (Pips)", DefaultValue = 15, MinValue = 2)]
public int SL { get; set; }
[Parameter("Take Profit (Pips)", DefaultValue = 35, MinValue = 2)]
public int TP { get; set; }
protected override void OnStart()
{
ExecuteMarketOrder(TradeType.Buy, SymbolName, Size, SymbolName, 10, 10);
}
protected override void OnTick()
{
// It iterates over all positions
foreach (var position in Positions)
{
// if position is 5 pips in profit
if (Positions[0].Pips == 5)
{
ExecuteMarketOrder(TradeType.Sell, SymbolName, Size, SymbolName, 0, 10);
}
// if position is 5 pips in lose
else if (Positions[0].Pips == -5)
{
ExecuteMarketOrder(TradeType.Buy, SymbolName, Size, SymbolName, 0, 10);
}
}
}
protected override void OnStop()
{
// Put your deinitialization logic here
}
}
}
is anything Wrong with the above code?
@danieleyube
danieleyube
07 Feb 2022, 10:26
RE:
amusleh said:
Hi,
You can use Position Pips property to get the current Pips amount of Position, ex:
using cAlgo.API; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.EasternStandardTime, AccessRights = AccessRights.FullAccess)] public class AUDJPY : Robot { [Parameter("Trade Size", DefaultValue = 1000, MinValue = 0.01)] public double Size { get; set; } [Parameter("Stop Loss (Pips)", DefaultValue = 15, MinValue = 2)] public int SL { get; set; } [Parameter("Take Profit (Pips)", DefaultValue = 35, MinValue = 2)] public int TP { get; set; } protected override void OnStart() { ExecuteMarketOrder(TradeType.Buy, SymbolName, Size, SymbolName, 0, 10); } protected override void OnTick() { // It iterates over all positions foreach (var position in Positions) { // if position is 5 pips in profit if (position.Pips == 5) { // Do something here, ex: execute a new order } // if position is 5 pips in lose else if (position.Pips == -5) { // Do something here, ex: execute a new order } } } } }
Thank you so much sir! I'll try it out and give you feedback.
@danieleyube
danieleyube
06 Feb 2022, 19:59
Multiple Entry Bot
Whenever a trade move from the entry point +5 or -5pips it should enter a new trade, please help i don't know how to go about it.
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.EasternStandardTime, AccessRights = AccessRights.FullAccess)]
public class AUDJPY : Robot
{
[Parameter("Trade Size", DefaultValue = 1000, MinValue = 0.01)]
public double Size { get; set; }
[Parameter("Stop Loss (Pips)", DefaultValue = 15, MinValue = 2)]
public int SL { get; set; }
[Parameter("Take Profit (Pips)", DefaultValue = 35, MinValue = 2)]
public int TP { get; set; }
protected override void OnStart()
{
ExecuteMarketOrder(TradeType.Buy, SymbolName, Size, SymbolName, 0, 10);
}
protected override void OnTick()
{
var position2 = Positions[0];
if (position2.EntryPrice - Symbol.Bid == -0.0005)
{
ExecuteMarketOrder(TradeType.Sell, SymbolName, Size, SymbolName, 0, 10);
}
if (position2.EntryPrice - Symbol.Bid == 0.0005)
{
ExecuteMarketOrder(TradeType.Buy, SymbolName, Size, SymbolName, 0, 10);
}
}
protected override void OnStop()
{
// Put your deinitialization logic here
}
}
}
@danieleyube
danieleyube
07 Feb 2022, 15:19 ( Updated at: 21 Dec 2023, 09:22 )
RE: RE: RE:
@danieleyube