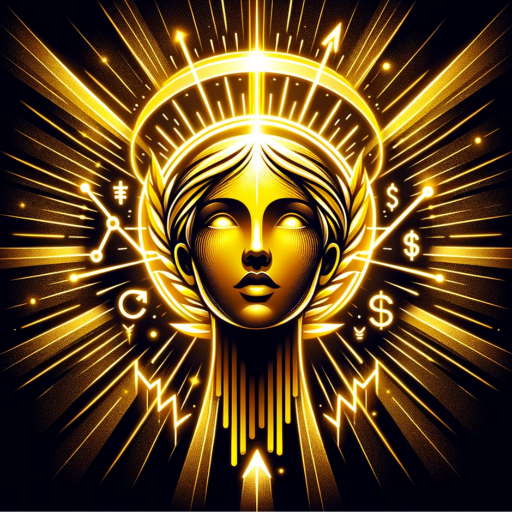
Topics
Replies
mywebsidekicks
01 Oct 2024, 13:30
( Updated at: 01 Oct 2024, 13:36 )
I'm using this script:
from ctrader_open_api import Client, Protobuf, TcpProtocol, EndPoints
from ctrader_open_api.messages.OpenApiCommonMessages_pb2 import *
from ctrader_open_api.messages.OpenApiMessages_pb2 import *
from ctrader_open_api.messages.OpenApiModelMessages_pb2 import *
from twisted.internet import reactor
import json
import datetime
import calendar
import pandas as pd
import numpy as np
credentialsFile = open("credentials.json")
credentials = json.load(credentialsFile)
host = EndPoints.PROTOBUF_LIVE_HOST if credentials["HostType"].lower() == "live" else EndPoints.PROTOBUF_DEMO_HOST
client = Client(host, EndPoints.PROTOBUF_PORT, TcpProtocol)
symbolName = "EURUSD"
## Define the trendbar period variable
# trendbar_period_str = "H1" # Set this to "D1" for daily bars or "H1" for hourly bars
trendbar_period_str = "M5"
## Map the string to the corresponding ProtoOATrendbarPeriod value
trendbar_period_map = {
"D1": ProtoOATrendbarPeriod.D1,
"H1": ProtoOATrendbarPeriod.H1,
"M30": ProtoOATrendbarPeriod.M30,
"M15": ProtoOATrendbarPeriod.M15,
"M10": ProtoOATrendbarPeriod.M10,
"M5": ProtoOATrendbarPeriod.M5,
}
trendbar_period = trendbar_period_map[trendbar_period_str]
## Set your desired 'from' and 'to' dates in format: Year, Month, Day
data_from = datetime.datetime(2021, 12, 27)
# data_to = datetime.datetime.utcnow() # until today, or:
data_to = datetime.datetime(2024, 8, 20) # any other specific date you want
dailyBars = []
symbolId = None
def connected(client): # Callback for client connection
print("\nConnected")
request = ProtoOAApplicationAuthReq()
request.clientId = credentials["ClientId"]
request.clientSecret = credentials["Secret"]
deferred = client.send(request)
deferred.addCallbacks(applicationAuthResponseCallback, onError)
def applicationAuthResponseCallback(result):
print("\nApplication authenticated")
request = ProtoOAAccountAuthReq()
request.ctidTraderAccountId = credentials["AccountId"]
request.accessToken = credentials["AccessToken"]
deferred = client.send(request)
deferred.addCallbacks(accountAuthResponseCallback, onError)
def accountAuthResponseCallback(result):
print("\nAccount authenticated")
request = ProtoOASymbolsListReq()
request.ctidTraderAccountId = credentials["AccountId"]
request.includeArchivedSymbols = False
deferred = client.send(request)
deferred.addCallbacks(symbolsResponseCallback, onError)
def symbolsResponseCallback(result):
print("\nSymbols received")
symbols = Protobuf.extract(result)
global symbolName, symbolId
symbolsFilterResult = list(filter(lambda symbol: symbol.symbolName == symbolName, symbols.symbol))
if len(symbolsFilterResult) == 0:
raise Exception(f"There is no symbol that matches your defined symbol name: {symbolName}")
elif len(symbolsFilterResult) > 1:
raise Exception(f"More than one symbol matched with your defined symbol name: {symbolName}, match result: {symbolsFilterResult}")
symbol = symbolsFilterResult[0]
symbolId = symbol.symbolId
print(f"SymbolID = {symbolId}")
request = ProtoOAGetTrendbarsReq()
request.symbolId = symbolId
request.ctidTraderAccountId = credentials["AccountId"]
request.period = trendbar_period
# request.period = ProtoOATrendbarPeriod.M5
request.fromTimestamp = int(calendar.timegm(data_from.utctimetuple())) * 1000
request.toTimestamp = int(calendar.timegm(data_to.utctimetuple())) * 1000
# to make sure it's unlimited
request.count = 9999999
deferred = client.send(request)
deferred.addCallbacks(trendbarsResponseCallback, onError)
def trendbarsResponseCallback(result):
print("\nTrendbars received")
trendbars = Protobuf.extract(result)
print(f"trendbars type {type(trendbars)}")
# print(f"trendbars = {trendbars[0]}")
barsData = list(map(transformTrendbar, trendbars.trendbar))
# print(f"barsData = {barsData}")
global dailyBars
dailyBars.clear()
dailyBars.extend(barsData)
print("\ndailyBars length:", len(dailyBars))
print("\Stopping reactor...")
reactor.stop()
def transformTrendbar(trendbar):
time_in_minutes = int(trendbar_period_str.replace("M", ""))
openTime = datetime.datetime.fromtimestamp(trendbar.utcTimestampInMinutes * 60, datetime.timezone.utc)
openPrice = (trendbar.low + trendbar.deltaOpen) / 100000.0
highPrice = (trendbar.low + trendbar.deltaHigh) / 100000.0
lowPrice = trendbar.low / 100000.0
closePrice = (trendbar.low + trendbar.deltaClose) / 100000.0
return [openTime, openPrice, highPrice, lowPrice, closePrice, trendbar.volume]
def onError(failure): # Call back for errors
print("\nMessage Error: ", failure)
def disconnected(client, reason): # Callback for client disconnection
print("\nDisconnected: ", reason)
def onMessageReceived(client, message): # Callback for receiving all messages
if message.payloadType in [ProtoHeartbeatEvent().payloadType, ProtoOAAccountAuthRes().payloadType,
ProtoOAApplicationAuthRes().payloadType, ProtoOASymbolsListRes().payloadType,
ProtoOAGetTrendbarsRes().payloadType]:
return
print("\nMessage received: \n", Protobuf.extract(message))
client.setConnectedCallback(connected)
client.setDisconnectedCallback(disconnected)
client.setMessageReceivedCallback(onMessageReceived)
client.startService()
reactor.run()
df = pd.DataFrame(np.array(dailyBars),
columns=['Time', 'Open', 'High', 'Low', 'Close', 'Volume'])
df["Open"] = pd.to_numeric(df["Open"])
df["High"] = pd.to_numeric(df["High"])
df["Low"] = pd.to_numeric(df["Low"])
df["Close"] = pd.to_numeric(df["Close"])
df["Volume"] = pd.to_numeric(df["Volume"])
print(df)
And for the “credentials.json” you obviously have to use this format:
{
"ClientId": "your-client-id",
"Secret": "your-secret",
"AccountId": your-account-id,
"AccessToken": "your-access-token",
"HostType": "live"
}
@mywebsidekicks
mywebsidekicks
11 Jun 2024, 10:42
( Updated at: 12 Jun 2024, 05:46 )
OK, finally I have found these so called ‘account IDs’! Hope this might be helpful to someone:
- Go to your cTrader apps page: https://openapi.ctrader.com/apps
- Click on the ‘Playground’ button next to an app you are working with
- Next click ‘Trading accounts’, there you'll find raw webpage with all your account data in JSON format
- You are interested in number under the "accountId"
@mywebsidekicks
mywebsidekicks
13 Mar 2024, 11:58
( Updated at: 16 Mar 2024, 16:38 )
PanagiotisCharalampous said:
mywebsidekicks said:
wonepo@gmail.com said:
Hi,
Do you have a solution since ??
Nope :(
Hi there,
You should not use the account number or the cTrader ID number in that field. You should use the ctidTraderAccountId that is included in ProtoOACtidTraderAccount
Best regards,
Panagiotis
I'm a bit confused now, can you please give a code example
@mywebsidekicks
mywebsidekicks
23 Dec 2023, 16:40
RE: Unable to find cTID trader account with id=XXXXXX
wonepo@gmail.com said:
Hi,
Do you have a solution since ??
Nope :(
@mywebsidekicks
mywebsidekicks
12 May 2023, 14:26
But quite a few indicators use Trade Volume data, as far as I know
@mywebsidekicks
mywebsidekicks
11 May 2023, 18:04
Can someone from Spotware comment on this?
How do I get Trade Volume in cTrader Automate?
@mywebsidekicks
mywebsidekicks
10 May 2023, 18:06
( Updated at: 10 May 2023, 18:12 )
What about
private TradeVolumeIndex _volume;
@mywebsidekicks
mywebsidekicks
09 May 2023, 15:19
But as far as I understand, Tick Volume is not the same as Trade Volume
@mywebsidekicks
mywebsidekicks
30 Nov 2020, 14:30
RE:
PanagiotisCharalampous said:
Hi mywebsidekicks,
Unfortunately we cannot support Open API v1.0 any more, therefore the only thing I can suggest to you is to get familiar with Open API v2.0. the soonest
Best Regards,
Panagiotis
When exactly Open API v1 will be terminated?
@mywebsidekicks
mywebsidekicks
26 Nov 2020, 22:18
( Updated at: 27 Nov 2020, 20:47 )
RE: RE: RE: It seems to work with Python
Hey mate, thanks a lot for sending me in the right direction! I was able to install ProtoBuffs and compile Spotware protos to Python files!
One more question if you don't mind:
import OpenApiCommonMessages_pb2 as CommonMessages
import OpenApiCommonModelMessages_pb2 as CommonModelMessages
import OpenApiMessages_pb2 as Messages
import OpenApiModelMessages_pb2 as ModelMessages
clientId = r"myclientId"
clientSecret = r"myclientSecret"
token = r"mytoken"
accAuth = Messages.ProtoOAAccountAuthReq()
accAuth.ctidTraderAccountId = 1234567
accAuth.accessToken = token
# checks if all the required fields have been set.
print(accAuth.IsInitialized())
# returns a human-readable representation of the message, particularly useful for debugging.
print(accAuth.__str__())
# serializes the message and returns it as a string.
print(accAuth.SerializeToString())
So how do I actually send the data?
It needs to be sent to 'live.ctraderapi.com:5035' Right?
Via regular Python socket interface or with SSL wrapper?
@mywebsidekicks
mywebsidekicks
22 Nov 2020, 18:31
Ok, I've connected with C# sample to the TRADE stream,
but still can't make it work on Python... :(
I'm doing exactly the same.
@mywebsidekicks
mywebsidekicks
19 Nov 2020, 19:58
RE:
PanagiotisCharalampous said:
Hi mywebsidekicks,
As per the error
The project file "C:\Users\irmsc\pp\FIX API Library\FIX API Library.csproj" was not found.
Can you confirm that file is there and it is not locked by any other process e.g. antivirus or something else?
Best Regards,
Panagiotis
Yes, I confirm. I first though this is due to old Microsoft Build tools 2017, but I reinstalled both Visual Studio and build tools of 2019.
May be I should try to run this ono an VPS..
@mywebsidekicks
mywebsidekicks
18 Nov 2020, 21:49
( Updated at: 21 Dec 2023, 09:22 )
RE:
@PanagiotisCharalampous
Ok, I tried to run
In the Visual Studio 2019 but got an error:
1>------ Build started: Project: FIX API Library, Configuration: Debug|AnyCPU ------
1>C:\Users\irmsc\AppData\Local\Temp\tmp4d2b9d281c5e45808cac2e0a5d03d93c.proj(23,1): error MSB3202: The project file "C:\Users\irmsc\pp\FIX API Library\FIX API Library.csproj" was not found.
Build Failure. Error: Failed to complete scanning on file ‘C:\Users\irmsc\pp\FIX API Library\FIX API Library.csproj’
The screenshot:
@mywebsidekicks
mywebsidekicks
16 Nov 2020, 14:33
RE: It seems to work with Python
@honeybadger
Right. That's some valuable info, thanks a lot!
So how did you install protobuffs on Python, with pip or?
Also, do you find Open API really any better than Fix API for trading?
PS. LOL, nice nickname by the way. I'm a big fan of HoneyBadger =)
@mywebsidekicks
mywebsidekicks
01 Oct 2024, 13:40 ( Updated at: 02 Oct 2024, 04:57 )
And make sure your ‘AccountId’ is one of these:
https://ctrader.com/forum/connect-api-support/42441/#post-110782
@mywebsidekicks