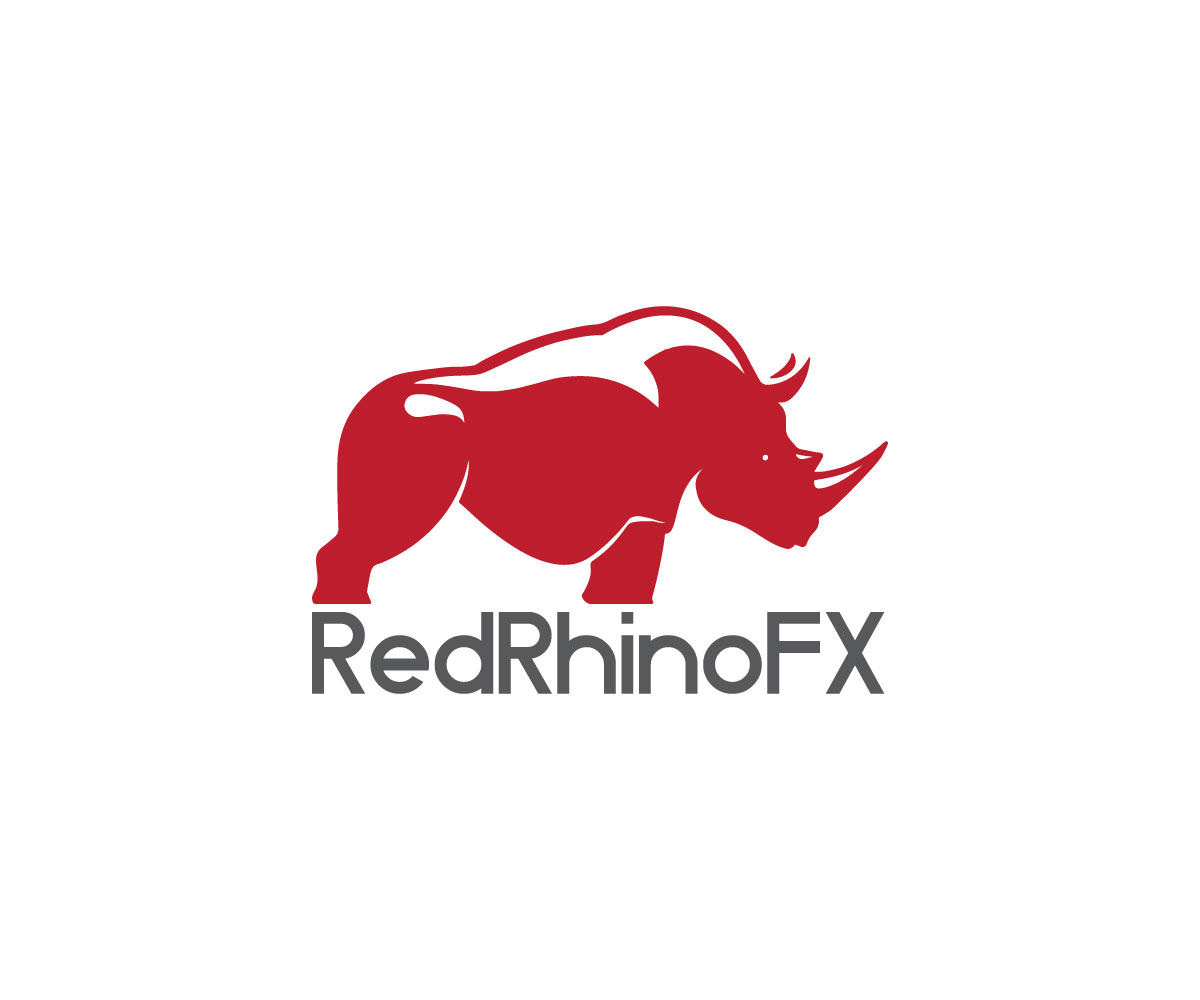
Topics
Replies
SwapBridgeCapital
22 Jun 2017, 06:25
The issue looks to be within the cBot because the above code will work fine within an indicator but when applying the same function within a cBot, both methods above mentioned to play a sound file does not work. It fails to open the file as mentioned before. Can anyone explain why sound can work within an indicator and not a cbot? FullAccess is applied in both (indicator/cbot)
namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)]
@SwapBridgeCapital
SwapBridgeCapital
22 Jun 2017, 06:14
Notification.PlaySound Error
[Parameter("Alert Buy Sound File", DefaultValue = "C:\\calgo\\buy.wav")] public string alertBuyFile { get; set; } //====== example to use within your code // if (UseNotify) { Notifications.PlaySound(alertBuyFile); } if (UsePlayer) { System.Media.SoundPlayer player = new System.Media.SoundPlayer(alertBuyFile); player.Play(); }
The system.media.soundplayer is working. Until the Notifications is fixed, I have placed a Boolean in the code to enable the SoundPlayer.
@SwapBridgeCapital
SwapBridgeCapital
21 Jun 2017, 18:03
At this moment, we have to contact our broker to remove the account from the cTID.
@SwapBridgeCapital
SwapBridgeCapital
21 Jun 2017, 17:58
cMirror Message Button is available
Hello GammaQuant,
I apologize for the losses. As you know the trading system was only a few weeks old and the longevity or robustness of the system has not been proven. I am trying my best to follow my own rules and working on solutions/tools to make it work for the longrun. My website link was in the strategy description and you can or could have contacted me via email or my trade room for questions before joining. Keep in mind that asking questions should be done before following the system because I won't be able to answer emails until my positions are closed.
You can also set a Hard Stoploss on your account to prevent A loss larger than you comfortable with within the cMirror Platform.
Also within cMirror you can send messages directly by clicking on the Signal Providers cTID, and clicking on the Message Button.
https://cmirror.com/strategy/61487
https://cmirror.com/strategy/61620
https://cmirror.com/strategy/61562
@SwapBridgeCapital
SwapBridgeCapital
21 Jun 2017, 17:41
( Updated at: 21 Dec 2023, 09:20 )
Notification Play Sound does not work.
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)] public class NewIndicator : Indicator { int i; public override void Calculate(int index) { if (IsLastBar) { i++; System.Media.SoundPlayer player = new System.Media.SoundPlayer("C:\\calgo\\buy.wav"); player.Play(); ChartObjects.DrawText("x", "test " + i, StaticPosition.BottomLeft, Colors.Yellow); } } } }
I have tried the above code. Does not work.
using System; using cAlgo.API; using cAlgo.API.Internals; using cAlgo.API.Indicators; using cAlgo.Indicators; namespace cAlgo { [Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)] public class NewIndicator : Indicator { [Parameter(DefaultValue = 0.0)] public double Parameter { get; set; } [Output("Main")] public IndicatorDataSeries Result { get; set; } protected override void Initialize() { // Initialize and create nested indicators Notifications.PlaySound("C:\\Users\\Asus\\Documents\\cAlgo\\Sources\\Robots\\buy.wav"); Notifications.PlaySound("C:\\Users\\Asus\\Documents\\cAlgo\\Sources\\Robots\\Buy.wav"); Notifications.PlaySound("C:\\calgo\\Buy.wav"); Notifications.PlaySound("C:\\calgo\\buy.wav"); } public override void Calculate(int index) { // Calculate value at specified index // Result[index] = ... Notifications.PlaySound("C:\\Users\\Asus\\Documents\\cAlgo\\Sources\\Robots\\buy.wav"); Notifications.PlaySound("C:\\Users\\Asus\\Documents\\cAlgo\\Sources\\Robots\\Buy.wav"); Notifications.PlaySound("C:\\calgo\\Buy.wav"); Notifications.PlaySound("C:\\calgo\\buy.wav"); } } }
The above code does not work.
I have tried the old API - /api/reference/internals/inotifications/playsound
Notifications.PlaySound(@"C:\SampleDestination\SampleSound.mp3");
When using PlaySound(@"location") after compiling the code, It is automatically updated to
Notifications.PlaySound("C:\\SampleDestination\\SampleSound.mp3");
On WIndows10, with microsoft .net 4.5.1 framewrok and 4.6
I've also made my wav files into mp3 and the playsound still does not work. Every time the the calgo or cbot log file will say FAILED.
The previous member had issues in March 2017. Today , JUNE 2017. Still no support? solution? Please help community.
@SwapBridgeCapital
SwapBridgeCapital
13 Jun 2017, 14:45
RE:
gitarp said:
You can reffer this help.spotware.com/ctid . And yes, there are no other choice. You must contact your broker to unlink your account.
Convenience has been removed.
@SwapBridgeCapital
SwapBridgeCapital
13 Jun 2017, 14:42
June 2017 How to remove account from cTrader ID
We were able to login to cmirror and remove them that way but it doesn't work any longer.
How to remove in 2017?
@SwapBridgeCapital
SwapBridgeCapital
17 Jul 2015, 04:09
RE:
3xfx said:
What exactly is the ib center, I have looked but found nothing.
I have only seen it in one of the brokers that I use. If your broker offers it, it will be located on the cAlgo platform under the cBots ( very button ).
Thanks for the reply.
@SwapBridgeCapital
SwapBridgeCapital
04 May 2015, 17:49
RE:
onemind said:
Hi
Quick question about what is possible with cTrader.
Cbots are great for auto trading and backtesting and indicatiors are great too but is there a way to add extra functionality to ctrader itself?
For example.
Can I create an indicator that shows max possible position size relative to balance and then have that inserted into the volume field for opening new trades with max volume?
So if i have a $1000 balance at 400:1 i would lilke to calculate the max volume i can have and have that inserted into the one click trading volume field.
Thanks for any info
you can build a cbot that can run in your ctrader platform that does all the calculations for you. You would create two cBots, One BUY and One Sell cbot. When you want to risk your account balance on 1 trade ( enter highest volume allowed, ) you select what cBot you want to trade ( buy or sell).
Simple select your cBot and let it open the position based on your calculations. It would be very similar to a one click button.
Scripts haven't been added to the cTrader platform yet,
@SwapBridgeCapital
SwapBridgeCapital
31 Mar 2015, 20:26
RE:
MZen said:
Hi,
I am new to VS, C# and cAlgo, so my question might be naive. Anyway.
How can I create custom library in VS to use in my robots and indicators? I already installed VS and I can edit cBots in VS. Yet, I need something like Money management class and methods to use in all my cBots. Where to start?
Thanks.
You need to reference the calgo api dll, then you will have intellisense and easily start your project in VS - http://redrhinofx.com/calgo
@SwapBridgeCapital
SwapBridgeCapital
31 Mar 2015, 20:21
that broker doesn't offer ctrader platform today.
Spotware hasn't released MAM/PAMM software yet. Since cMirror is finished, maybe that will be the next product on their list.
@SwapBridgeCapital
SwapBridgeCapital
28 Mar 2015, 06:28
I have been developing trading systems without any martingale tactics for 2015 - http://www.myfxbook.com/members/RedRhinoLab
RevEnginePro
RhinoEG
Caesar Signal EA
Vortex Scalper Pro
@SwapBridgeCapital
SwapBridgeCapital
01 Feb 2015, 15:59
May I ask where did you get your data from ( source) and did you need to alter the data format to make it compatible with cAlgo format
2003.06.18,16:01,1.11423,1.11428,1.11332,1.11374,19
@SwapBridgeCapital
SwapBridgeCapital
01 Feb 2015, 15:57
is dukascopy data in the right format for cAlgo, Their data is free and you download M1 or tick.
@SwapBridgeCapital
SwapBridgeCapital
01 Feb 2015, 15:22
tick
The files are in a proprietary format with scrambling (reversible encryption). The only value of looking at them is to check whether the specific date and symbol has been cached or not.
Will the tick data be available for multipurpose in the future ( without encryption).
@SwapBridgeCapital
SwapBridgeCapital
23 Jan 2015, 10:02
RE:
maninjapan said:
I have noticed a fee threads on the topic of a Trade Copier Function for cTrader. Are there any updates on this? I have also noticed a couple of trade copier bots for MT4 to cTrader. Are there any bots, that any one knows of, for cTrader to cTrader?
Thanks!
I am also interested in a cTrader to cTrader Trade Copier bridge tool, I have added a Job listing here - /jobs/105
@SwapBridgeCapital
SwapBridgeCapital
19 Nov 2014, 15:01
RE:
Spotware said:
We cannot provide a time estimate for the release, but it is planned.
1 year later,
Any update on partial close in backtesting?
@SwapBridgeCapital
SwapBridgeCapital
22 Jun 2017, 06:42
cBot code working now with the below code.
For the project that I am working on, when I attach a new Instance of my cBot, the PopUp box asks for permission, I accept the confirmation and the above code will play the sound. If I add another instance, it does not ask for the permission again ( FULLACCESS). Is this permission saved in memory? How to reset the permission? Removing all instances and re-adding them does not ask for the permission again.
@SwapBridgeCapital