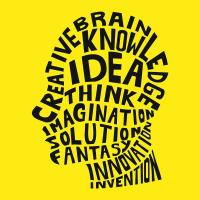
Information
Username: | albatros667 |
Member since: | 29 May 2019 |
Last login: | 21 Jan 2025 |
Status: | Active |
Activity
Where | Created | Comments |
---|---|---|
Forum Topics | 1 | 3 |
Jobs | 0 | 0 |
Username: | albatros667 |
Member since: | 29 May 2019 |
Last login: | 21 Jan 2025 |
Status: | Active |
Where | Created | Comments |
---|---|---|
Forum Topics | 1 | 3 |
Jobs | 0 | 0 |