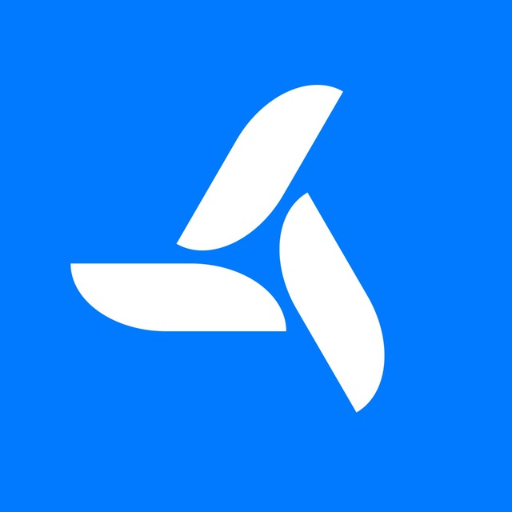
Topics
Replies
acnestis
11 Feb 2021, 10:17
RE:
PanagiotisCharalampous said:
Hi robustby,
The ask price is not available. Bars are created using bid prices. To get ask prices you need to get the tick data using MarketData.GetTicks() and loop through the ticks to find the lowest for the bar's period.
Best Regards,
Panagiotis
Thank you! Can you write some example how to use it?
@acnestis
acnestis
09 Jun 2020, 13:14
( Updated at: 21 Dec 2023, 09:22 )
RE:
PanagiotisCharalampous said:
Ηι robustby,
How do you know that these copiers are not copying your trades?
Hi! Usually I can see changing "Copying Funds (Live)" in real time. So, now this value is not changing. "Total Volume Copied (Live)" is not changing also.
What commission would you expect to get?
Volume Fee.
Why do you say that they are demo copiers but displayed as live?
Because looks like a some bug for me and this is a possible explanation.
@acnestis
acnestis
12 May 2020, 15:19
RE:
PanagiotisCharalampous said:
Hi robustby,
Try rounding the values before using them
var SellLim = Math.Round(barHigh - Dist * 1E-05, Symbol.Digits); var BuyLim = Math.Round(barLow + Dist * 1E-05, Symbol.Digits);
Best Regards,
Panagiotis
Thank you so much! It's works :)
@acnestis
acnestis
12 May 2020, 11:21
RE:
PanagiotisCharalampous said:
Hi robustby,
This message usually appears when the modified parameter is the same as the previous one. To investigate your case further, you need to provide us with the complete cBot source code and cBot parameters to reproduce this message on backtesting.
Best Regards,
Panagiotis
Here is my code.
Problem with following code
var SellLim = barHigh - Dist * 1E-05;
var BuyLim = barLow + Dist * 1E-05;
If I put it instead of
var SellLim = barHigh;
var BuyLim = barLow;
With the code above all working fine.
You can use any of parameters, it's doesn't matter. For example: bar_analyse=20, Dist=5, IndBar=8.
@acnestis
acnestis
11 Feb 2021, 12:52
RE:
PanagiotisCharalampous said:
Thank you so much for the example. Can you check if I'm right? If I want to get lower Ask price I'm doing following:
Also, I have some questions:
1) If I want to use only last 10000 ticks, how better load it? Or if I want use ticks data for only 50 last bars at the chart?
2) I must use MarketData.GetTicks() & ticks.LoadMoreHistory() for every time when new tick appears? Please explain how it works.
@acnestis