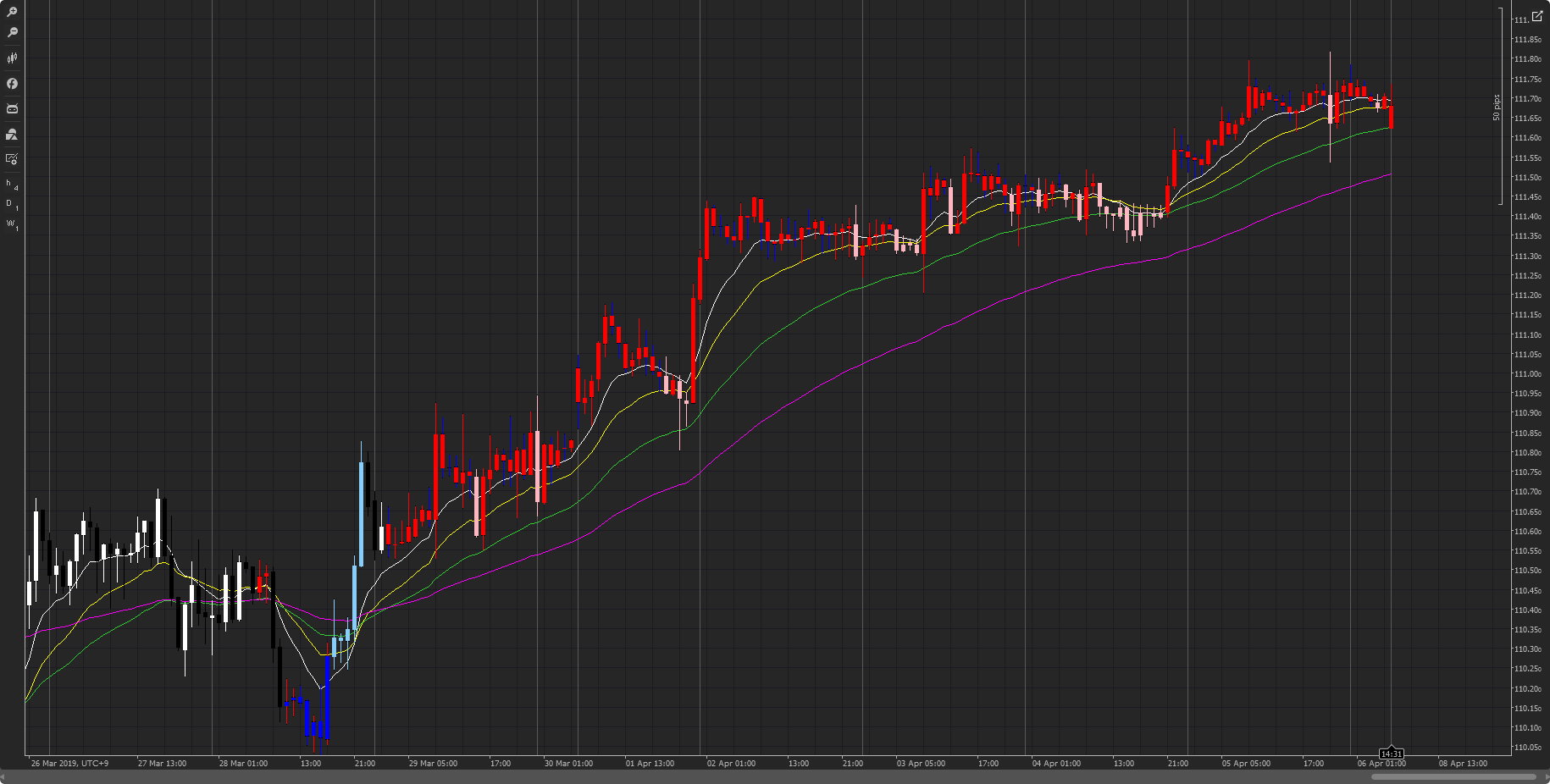
Topics
Replies
Tatsuya
07 Feb 2020, 05:58
Same problem here,Error #495654: Can not create instance and i can't run my cBot after 3.7 update.
Everytime they add something new,they break something else.
Edit:
Seems you can no longer add static to parameters,for me that was the reason.
Spotware,I know you guys are doing your best but debug harder,as I said above everytime you add something you also break something else,most of the time it is not a big problem but this time it is.
How can I trust and put money on something that changes its behavior once in a while without any notice?
@Tatsuya
Tatsuya
11 Nov 2019, 12:12
RE:
Panagiotis Charalampous said:
Hi Tatsuya,
Which cTrader do you use? Can you send some screenshots?
Best Regards,
Panagiotis
Sure thing.
On the current Axiory cTrader:
On the current Spotware cTrader:
As you can see on the axiory version,horizontal lines are thinner than the latest ones,also Fibonacci retracement and some other drawning tools lines are inconsistent.
On the Spotware version all lines are consistent now,however,lines got thicker again just like back in August.
I hope this helps.
@Tatsuya
Tatsuya
25 Aug 2019, 21:38
Hope this code helps.
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class NewcBot : Robot { [Parameter("Instance Name", DefaultValue = "001")] public string InstanceName { get; set; } [Parameter("Lot Size", DefaultValue = 0.1)] public double LotSize { get; set; } [Parameter("Source Dema")] public DataSeries SourceDema { get; set; } [Parameter("Source Tema")] public DataSeries SourceTema { get; set; } [Parameter("Period Dema", DefaultValue = 5, MinValue = 5, MaxValue = 100)] public int PeriodDema { get; set; } [Parameter("Period Tema", DefaultValue = 5, MinValue = 5, MaxValue = 100)] public int PeriodTema { get; set; } [Parameter("Calculate OnBar", DefaultValue = false)] public bool CalculateOnBar { get; set; } private DEMA Dema; private TEMA Tema; protected override void OnStart() { Dema = Indicators.GetIndicator<DEMA>(SourceDema, PeriodDema); Tema = Indicators.GetIndicator<TEMA>(SourceTema, PeriodTema); } protected override void OnTick() { if (CalculateOnBar) { return; } ManagePostions(); } protected override void OnBar() { if (!CalculateOnBar) { return; } ManagePostions(); } protected override void OnStop() { // Put your deinitialization logic here } private void ManagePostions() { // When you want to grab data from indicators you need their IndicatorDataSeries. // Result wasn't either in one of them,in your case you had tema and dema. if (Tema.tema.LastValue > Dema.dema.LastValue) { if (!IsPositionOpenByType(TradeType.Buy)) { OpenPosition(TradeType.Buy); } ClosePosition(TradeType.Sell); } if (Tema.tema.LastValue < Dema.dema.LastValue) { if (!IsPositionOpenByType(TradeType.Sell)) { OpenPosition(TradeType.Sell); } ClosePosition(TradeType.Buy); } } private void OpenPosition(TradeType type) { // QuantityToVolumeInUnits returns double,not long therefore you either have to put it into double or cast it to long. // e.g. long Volume = (long)Symbol.QuantityToVolumeInUnits(LotSize); this also works. double Volume = Symbol.QuantityToVolumeInUnits(LotSize); ExecuteMarketOrder(type, this.SymbolName, Volume, InstanceName, null, null); } private void ClosePosition(TradeType type) { var P = Positions.Find(InstanceName, this.SymbolName, type); if (P != null) { ClosePosition(P); } } private bool IsPositionOpenByType(TradeType type) { var P = Positions.FindAll(InstanceName, SymbolName, type); if (P.Count() >= 1) { return true; } return false; } } }
@Tatsuya
Tatsuya
25 Aug 2019, 10:58
You don't need get set for variables,try this one instead:
private DEMA Dema; private TEMA Tema;
I'd like to see the DEMA and TEMA code if the code above doesn't work.
Also the last error is just telling you that there is a newer API that does the same thing.
You can simply replace QuantityToVolume with QuantityToVolumeInUnits.
@Tatsuya
Tatsuya
07 Feb 2020, 08:07
RE:
PanagiotisCharalampous said:
Sure thing.
@Tatsuya