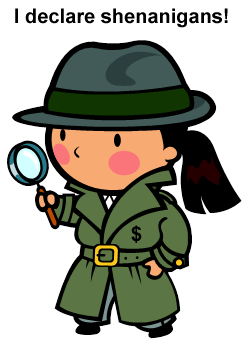
Topics
Replies
fzlogic
21 Jan 2014, 12:27
using System; using System.Linq; using cAlgo.API; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC)] public class NewRobot : Robot { private int _counter; [Parameter(DefaultValue = 5000)] public int Volume { get; set; } [Parameter(DefaultValue = 1)] public int expMinutes { get; set; } protected override void OnTick() { if (_counter < 2) { PlaceStopOrder(TradeType.Sell, Symbol, Volume, Symbol.Ask - Symbol.PipSize * 10, "Lable", 10, 10, Server.Time.AddMinutes(expMinutes)); _counter++; Print(_counter); } else { var pendingOrder = PendingOrders.FirstOrDefault(order => order.Label == "Lable"); if (pendingOrder == null) { Position position = Positions.Find("Lable"); if (position == null) { _counter = 0; Print("order expired counter = {0}", _counter); } } } } } }
@fzlogic
fzlogic
30 Dec 2013, 12:51
OnPositionsClosed will be triggered before PositionClosedByRobot and the result will be that flag_robot_close will not serve the intended purpose.
Try setting the flag_robot_close to true before calling ClosePositionAsync.
if (myPosition != null && breakeven_flag && distance_1) { flag_robot_close = true; ClosePositionAsync(myPosition, PositionClosedByRobot); }
@fzlogic
fzlogic
28 Nov 2013, 17:15
main is the percentK (green line) and signal is the percentD (red line).
StochasticOscillator stochOsc; protected override void OnStart() { stochOsc = Indicators.StochasticOscillator(9, 3, 9, MovingAverageType.Simple); } protected override void OnTick() { if (stochOsc.PercentK.LastValue > stochOsc.PercentD.LastValue) { // } }
@fzlogic
fzlogic
18 Nov 2013, 15:30
RE: RE: RE:
fzlogic said:
hichem said:
pparpas said:I am in the process of finding the best possible automated platform that covers my needs. By using cAlgo, is there any way to debug robots by using Visual Studio? I have made many trials using Visual Studio and trying to attach the debugger to calgo process with no luck.
Debugging a robot in Visual Studio is possible. But you should compile the robot to a .dll file with Visual Studio. Then you should reference the generated .dll in cAlgo and after executing the robot in cAlgo you should attach VS debugger.
Hi Hichem,
Thanks for the useful tip. I create a robot that is derived from the class of the referenced dll, I manage to debug by calling base.OnStart and base.OnTick but I am using indicators for the calculations and their values are always zero.
More specifically, I am trying to debug Artificial Intelligence robot found here: /algos/robots/show/46
So this is the code of the robot that is referencing Artificial Intelligence:
//#reference: ..\..\..\Visual Studio 2010\Projects\AI\AI\bin\Debug\AI.dll // ------------------------------------------------------------------------------- // // /algos/robots/show/46 // // ------------------------------------------------------------------------------- using System; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC)] public class AI : ArtificialIntelligence { private MacdHistogram macd; protected override void OnStart() { // Put your initialization logic here macd = Indicators.MacdHistogram(21, 20, 3); base.OnStart(); } protected override void OnTick() { // Put your core logic here Print(macd.Histogram.LastValue); base.OnTick(); } } }
I made the macd public in the base class and initialized it in the derived class and now I have values for it. I don't know if this is a good way to do this.
public class AI : ArtificialIntelligence { private MacdHistogram mcd; protected override void OnStart() { mcd = Indicators.MacdHistogram(21, 20, 3); base.macd = mcd; }
@fzlogic
fzlogic
18 Nov 2013, 15:08
RE: RE:
hichem said:
pparpas said:I am in the process of finding the best possible automated platform that covers my needs. By using cAlgo, is there any way to debug robots by using Visual Studio? I have made many trials using Visual Studio and trying to attach the debugger to calgo process with no luck.
Debugging a robot in Visual Studio is possible. But you should compile the robot to a .dll file with Visual Studio. Then you should reference the generated .dll in cAlgo and after executing the robot in cAlgo you should attach VS debugger.
Hi Hichem,
Thanks for the useful tip. I create a robot that is derived from the class of the referenced dll, I manage to debug by calling base.OnStart and base.OnTick but I am using indicators for the calculations and their values are always zero.
More specifically, I am trying to debug Artificial Intelligence robot found here: /algos/robots/show/46
So this is the code of the robot that is referencing Artificial Intelligence:
//#reference: ..\..\..\Visual Studio 2010\Projects\AI\AI\bin\Debug\AI.dll // ------------------------------------------------------------------------------- // // /algos/robots/show/46 // // ------------------------------------------------------------------------------- using System; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC)] public class AI : ArtificialIntelligence { private MacdHistogram macd; protected override void OnStart() { // Put your initialization logic here macd = Indicators.MacdHistogram(21, 20, 3); base.OnStart(); } protected override void OnTick() { // Put your core logic here Print(macd.Histogram.LastValue); base.OnTick(); } } }
@fzlogic
fzlogic
13 Nov 2013, 14:59
Sorry, that one was also wrong.
This one is correct:
double lastLong = 0; double lastShort = 0; for (int i = index; i > index - Period; i--) { if (lastLong == 0 && MarketSeries.Open[i] < MarketSeries.Close[i]) { lastLong = MarketSeries.High[i]; ChartObjects.DrawText("LL", "LastLong", i, lastLong, VerticalAlignment.Top, HorizontalAlignment.Center, Colors.LimeGreen); } else if (lastShort == 0 && MarketSeries.Open[i] > MarketSeries.Close[i]) { lastShort = MarketSeries.Low[i]; ChartObjects.DrawText("LS", "LastShort", i, lastShort, VerticalAlignment.Bottom, HorizontalAlignment.Center, Colors.Red); } if (lastLong > 0 && lastShort > 0) break; }
@fzlogic
fzlogic
13 Nov 2013, 14:56
RE:
fzlogic said:
for (int i = index; i > index - Period; i--) if (MarketSeries.Open[i] < MarketSeries.Close[i]) { ChartObjects.DrawText("LL", "LastLong", index, MarketSeries.High[i], VerticalAlignment.Top, HorizontalAlignment.Center, Colors.LimeGreen); break; } }
That one was only for last long.
double lastLong = 0; double lastShort = 0; for (int i = index; i > index - Period; i--) { if (MarketSeries.Open[i] < MarketSeries.Close[i]) { lastLong = MarketSeries.High[i]; ChartObjects.DrawText("LL", "LastLong", i, lastLong, VerticalAlignment.Top, HorizontalAlignment.Center, Colors.LimeGreen); } else if (MarketSeries.Open[i] > MarketSeries.Close[i]) { lastShort = MarketSeries.Low[i]; ChartObjects.DrawText("LS", "LastShort", i, lastShort, VerticalAlignment.Bottom, HorizontalAlignment.Center, Colors.Red); } if (lastLong > 0 && lastShort > 0) break; }
@fzlogic
fzlogic
13 Nov 2013, 14:40
private int _firstBarIndex = -1; private int _secondBar; protected override void OnStart() { // Put your initialization logic here } protected override void OnBar() { // Put your core logic here DateTime currentTime = MarketSeries.OpenTime[MarketSeries.OpenTime.Count - 1]; DateTime previousTime = MarketSeries.OpenTime[MarketSeries.OpenTime.Count - 2]; int index = MarketSeries.OpenTime.Count - 1; if (currentTime.DayOfWeek == DayOfWeek.Sunday && previousTime.DayOfWeek != DayOfWeek.Sunday) { //first bar of the week _firstBarIndex = index; } if (index == _firstBarIndex + 1) { // second bar of the week _secondBar = index; Print(MarketSeries.OpenTime[_secondBar]); } }
@fzlogic
fzlogic
31 Oct 2013, 14:22
RE:
fzlogic said:
There is this example you can elaborate on /forum/cbot-support/219
using System; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot()] public class BaseRobot : Robot { protected string symbolCode; public int Volume { get; set; } protected string SymbolCode { get { return Symbol.Code; } set { symbolCode = value; } } protected override void OnStart() { var symbol = MarketData.GetSymbol(SymbolCode); Trade.CreateBuyMarketOrder(symbol, Volume); } protected override void OnTick() { foreach (var pos in Account.Positions) Print("{0}", pos.Id); } } }
//#reference: BaseRobot.algo using System; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.API.Requests; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC)] public class ChildRobot : BaseRobot { protected override void OnStart() { // Put your initialization logic here Volume = 1000; SymbolCode = Symbol.Code; base.OnStart(); } protected override void OnTick() { // Put your core logic here base.OnTick(); } } }
Sorry, You want communication, my example is not valid...
@fzlogic
fzlogic
31 Oct 2013, 14:13
There is this example you can elaborate on /forum/cbot-support/219
using System; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot()] public class BaseRobot : Robot { protected string symbolCode; public int Volume { get; set; } protected string SymbolCode { get { return Symbol.Code; } set { symbolCode = value; } } protected override void OnStart() { var symbol = MarketData.GetSymbol(SymbolCode); Trade.CreateBuyMarketOrder(symbol, Volume); } protected override void OnTick() { foreach (var pos in Account.Positions) Print("{0}", pos.Id); } } }
//#reference: BaseRobot.algo using System; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.API.Requests; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC)] public class ChildRobot : BaseRobot { protected override void OnStart() { // Put your initialization logic here Volume = 1000; SymbolCode = Symbol.Code; base.OnStart(); } protected override void OnTick() { // Put your core logic here base.OnTick(); } } }
@fzlogic
fzlogic
30 Oct 2013, 15:23
RE: RE:
atrader said:
PCWalker said:
Will this take anymore Longer?
Have you seen this: /forum/cbot-support/1739?page=1#7
regarding the article
to avoid copy paste between VS and calgo you can set the output path in VS to the robots folder ..\..\..\..\cAlgo\Sources\Robots\ when you build it will create the executable file in that folder but then you should not rebuild the cs file in calgo.
@fzlogic
fzlogic
30 Oct 2013, 15:05
( Updated at: 21 Dec 2023, 09:20 )
RE: RE: RE:
Cerunnos said:
fzlogic said:
protected override void OnBar() { // Put your core logic here var index = heiken.closeha.Count - 1; if (heiken.closeha[index] > heiken.openha[index]) Print("green"); else Print("red"); }I think that for the last bar it may not produce correct results. Notice in the screenshot how there is both colors in certain candles.
Thanks for help. I have one more question in this context. Within my Robot I'm using OnTick () method instead of onBar (). But I need some kind of bar-counter:
If a bar has the color blue in the next three bars and a further condition is given, then the robot should enter an order. How can I do that? Thanks
What do you mean next three bars? You mean if a bar has the color blue in the previous three bars?
for (int i = index; i > index - 3; i--) { if (heiken.closeha[index] > heiken.openha[index]) continue; //blue red = true; break; } if (!red && condition) ExecuteOrder(TradeType);
@fzlogic
fzlogic
30 Oct 2013, 11:50
There doesn't seem to be a property for the minutes /api/timeframe, so yes, you need a switch statement. I hope they implement it.
@fzlogic
fzlogic
22 Jan 2014, 17:39
RE:
Isn't that the sum of all positions net profit?
kricka said:
@fzlogic