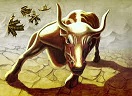
Topics
28 Aug 2012, 13:02
5447
2
Replies
sktrader
17 Sep 2012, 10:06
Hi,
You need to click Add Reference on the top next to build, locate your algo file (indicator) and then in the code:
TrendFinder trendFinder; // Declaration protected override void OnStart() { trendFinder = Indicators.GetIndicator<TrendFinder>(StdDevMultiplier, StdDevPeriod, MAType); } protected override void OnTick() { Print("{0}", trendFinder.UpTrend.LastValue); Print("{0}", trendFinder.DownTrend) .LastValue); }
@sktrader
sktrader
19 Sep 2012, 12:26
Hi,
I looked at your code and there are a few mistakes.
First of all this is not possible: position = 1. What are you trying to do here?
Here are some corrections:
@sktrader