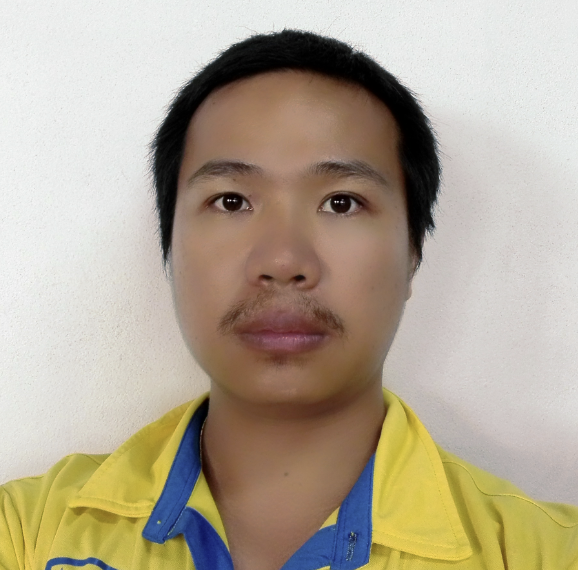
Topics
Replies
ogima.515@gmail.com
18 Feb 2021, 11:07
( Updated at: 18 Feb 2021, 11:18 )
This my cod have problem
@ogima.515@gmail.com
ogima.515@gmail.com
21 Sep 2017, 04:37
RE:
Panagiotis Charalampous said:
Hi ogima.515@gmail.com,
The Supertrend indicator has not been added yet to cTrader Desktop Indicators. You can find a supertrend indicator provided by the community here
/algos/indicators/show/136
Best Regards,
Panagiotis
Thank you for the reply and hope this indicator will come soon.
@ogima.515@gmail.com
ogima.515@gmail.com
15 Sep 2017, 11:48
RE: RE:
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; using System.Windows.Forms; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class BarrycBot : Robot { [Parameter("Position Id", DefaultValue = "Barry cBot")] public string PositionId { get; set; } [Parameter("Quantity (Lots)", DefaultValue = 0.1, MinValue = 0.01, Step = 0.01)] public double Quantity { get; set; } [Parameter("Take Profit (Pips)", DefaultValue = 500, MinValue = 0, Step = 1)] public double TP { get; set; } [Parameter("Stop Loss (Pips)", DefaultValue = 500, MinValue = 0, Step = 1)] public double SL { get; set; } private BarryBoxIndyV02midLine barry; protected override void OnStart() { barry = Indicators.GetIndicator<BarryBoxIndyV02midLine>(barryUp, barryDn, midLine); } protected override void OnBar() { var barry_openbuy1 = MarketSeries.Low.Last(2) < barry.barryDn.Last(2) && MarketSeries.Low.Last(1) > barry.barryDn.Last(1); var barry_closebuy1 = MarketSeries.High.Last(2) > barry.barryUp.Last(2) && MarketSeries.High.Last(1) < barry.barryUp.Last(1); var position = Positions.Find(PositionId, Symbol, TradeType.Buy); if (position != null && barry_closebuy1) { Close(TradeType.Buy); } if (position == null && barry_openbuy1) { Open(TradeType.Buy); } } protected override void OnTick() { } protected override void OnStop() { // Put your deinitialization logic here } private void Close(TradeType tradeType) { // if (position.NetProfit > 0.0) foreach (var position in Positions.FindAll(PositionId, Symbol, tradeType)) if (position.NetProfit > 0.0) { ClosePosition(position); } } private void Open(TradeType tradeType) { var position = Positions.Find(PositionId, Symbol, tradeType); var volumeInUnits = Symbol.QuantityToVolume(Quantity); if (position == null) { ExecuteMarketOrder(tradeType, Symbol, volumeInUnits, PositionId, SL, TP); } } } }
@ogima.515@gmail.com
ogima.515@gmail.com
15 Sep 2017, 11:42
RE:
// ------------------------------------------------------------------------------------------------- // BarryBox Indy // powered by http://stealthForex.eu - http://stealthForex.it // // ------------------------------------------------------------------------------------------------- using System; using cAlgo.API; using cAlgo.API.Internals; using cAlgo.API.Indicators; using cAlgo.Indicators; namespace cAlgo { [Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class BarryBoxIndyV02midLine : Indicator { [Parameter("Show mid line", DefaultValue = true)] public bool showMidLine { get; set; } [Parameter("Show prices", DefaultValue = true)] public bool showPrice { get; set; } [Output("BarryBox Upper", Color = Colors.Red, Thickness = 2)] public IndicatorDataSeries barryUp { get; set; } [Output("BarryBox Lower", Color = Colors.Lime, Thickness = 2)] public IndicatorDataSeries barryDn { get; set; } [Output("BarryBox mid line", Color = Colors.Yellow, Thickness = 1, LineStyle = LineStyle.Dots)] public IndicatorDataSeries midLine { get; set; } protected override void Initialize() { } public override void Calculate(int index) { if (MarketSeries.High[index] > MarketSeries.High[index - 1] && MarketSeries.High[index] > MarketSeries.High[index - 2] && MarketSeries.High[index] > MarketSeries.High[index + 1] && MarketSeries.High[index] > MarketSeries.High[index + 2]) { barryUp[index] = MarketSeries.High[index]; } else { barryUp[index] = barryUp[index - 1]; } if (MarketSeries.Low[index] < MarketSeries.Low[index - 1] && MarketSeries.Low[index] < MarketSeries.Low[index - 2] && MarketSeries.Low[index] < MarketSeries.Low[index + 1] && MarketSeries.Low[index] < MarketSeries.Low[index + 2]) { barryDn[index] = MarketSeries.Low[index]; } else { barryDn[index] = barryDn[index - 1]; } if (showMidLine) { midLine[index] = (barryDn[index] + barryUp[index]) / 2; } else { midLine[index] = double.NaN; } if (IsRealTime && showPrice) { ChartObjects.DrawText("UPPER Price", "UPPER " + Math.Round(barryUp[index], Symbol.Digits).ToString().Replace(',', '.'), index, barryUp[index] + 2 * Symbol.PipValue, VerticalAlignment.Top, HorizontalAlignment.Left); ChartObjects.DrawText("LOWER Price", "LOWER " + Math.Round(barryDn[index], Symbol.Digits).ToString().Replace(',', '.'), index, barryDn[index] - 2 * Symbol.PipValue, VerticalAlignment.Bottom, HorizontalAlignment.Left); } } } }
ogima.515@gmail.com said:
I need value of barryUp barryDn and midLine in BarryBoxIndyV02midLine : Indicator
Tell me pls
This my code
barry = Indicators.GetIndicator<BarryBoxIndyV02midLine>(barryUp, barryDn, midLine);
protected override void OnBar()
{
var barry_openbuy1 = MarketSeries.Low.Last(2) < barry.barryDn.Last(2) && MarketSeries.Low.Last(1) > barry.barryDn.Last(1);
var barry_closebuy1 = MarketSeries.High.Last(2) > barry.barryUp.Last(2) && MarketSeries.High.Last(1) < barry.barryUp.Last(1);
var position = Positions.Find(PositionId, Symbol, TradeType.Buy);
if (position != null && barry_closebuy1)
{
Close(TradeType.Buy);
}
if (position == null && barry_openbuy1)
{
if (CheckDay() && CheckTime())
{
Open(TradeType.Buy);
}
}
}
@ogima.515@gmail.com
ogima.515@gmail.com
15 Sep 2017, 11:36
RE:
Spotware said:
Dear Trader,
Thanks for posting in our forum. Could you please be more specific on what kind of assistance you need? Form the posted code, even though it is incomplete, it seems that you are already calling the indicator and getting the barryUp and barryDn values. What is the problem you are facing? Are the values you are getting not the ones you are expecting? Do you get compilation error? If you give some more information it will be easier for the community and cTrader Team to help you.
Best Regards,
cTrader Team
My first time on this forum and thank you for this suggestion.
@ogima.515@gmail.com
ogima.515@gmail.com
18 Feb 2021, 11:08
This my cod have problem
@ogima.515@gmail.com