Topics
Replies
levd20
07 Sep 2024, 01:30
RE: Cross line from below or above.
should be something like
//cross above
if(Bars.ClosePrices.Last(0) ≥ myIndicator.Last(0) && Bars.ClosePrices(1) < myIndicator.Last(0))
{
// do stuff
ExecuteMarketOrder…
}
rdebacker said:
Thanks for your reply.
Somebody found a code sample in the past that can be used for this?
Thank you
Rafael
@levd20
levd20
01 Sep 2024, 15:15
( Updated at: 02 Sep 2024, 05:28 )
RE: Deal Map
Hi, feel free to use this indicator.
best regards
levd20
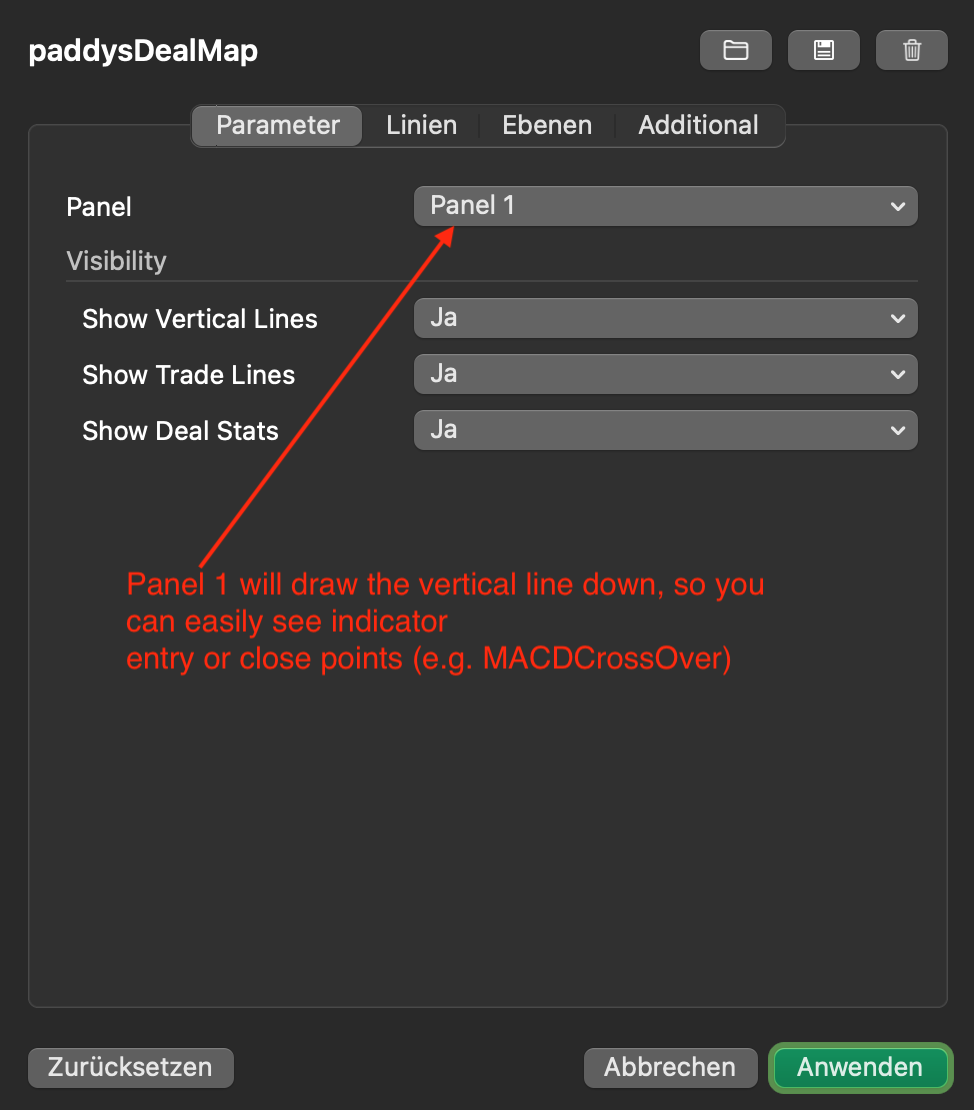
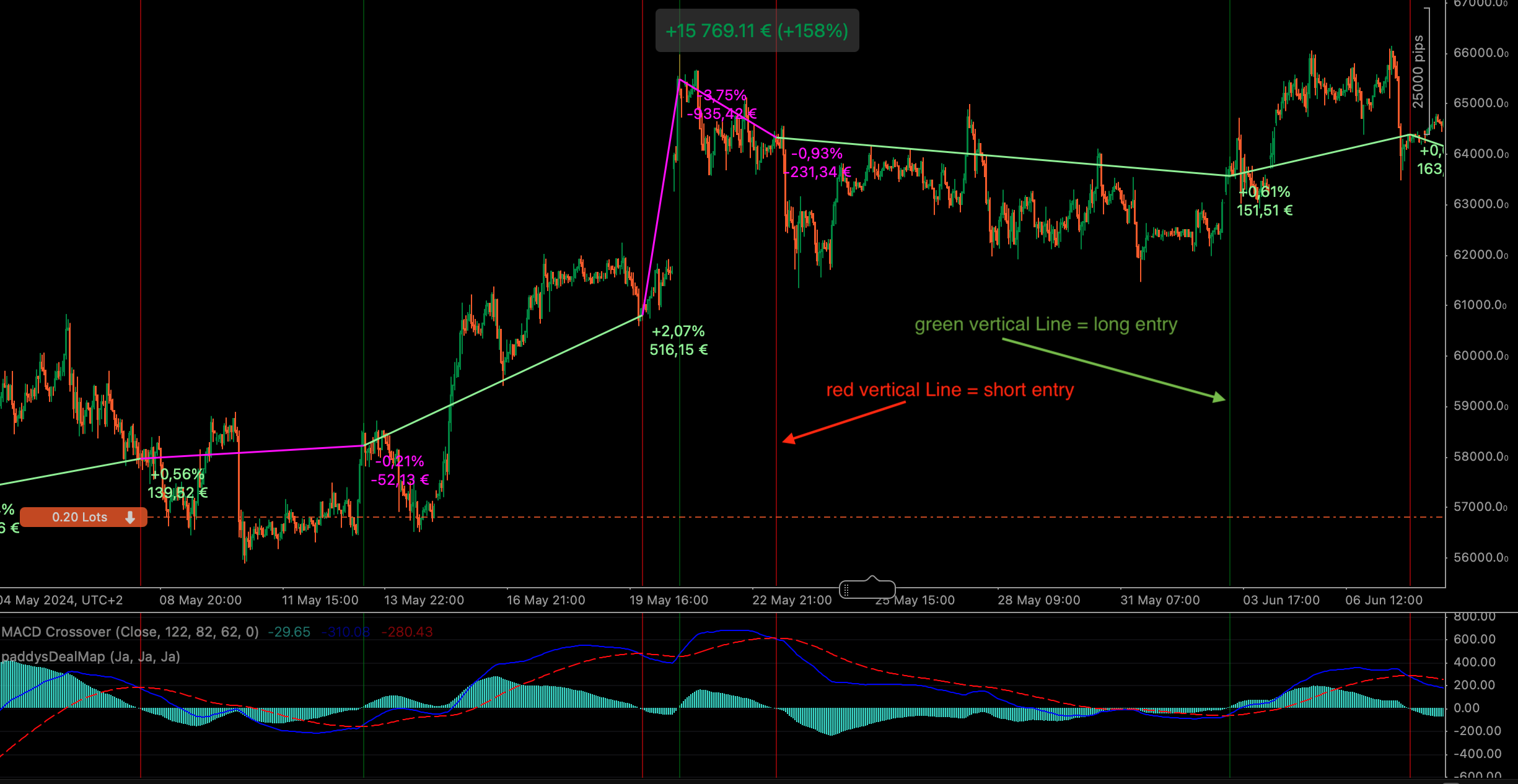
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = true, AccessRights = AccessRights.None)]
public class paddysDealMap : Indicator
{
#region Parameter
[Parameter("Show Vertical Lines", Group = "Visibility", DefaultValue = true)]
public bool showVerticalLines { get; set; }
[Parameter("Show Trade Lines", Group = "Visibility", DefaultValue = true)]
public bool showTradeLines { get; set; }
[Parameter("Show Deal Stats", Group = "Visibility", DefaultValue = true)]
public bool showDealStats { get; set; }
#endregion
protected override void Initialize()
{
Chart.RemoveAllObjects();
DrawVerticalLines();
DrawDealMap();
DrawDealStats();
}
#region own Methods
private void DrawVerticalLines()
{
foreach (var Trade in History)
{
if(showVerticalLines && Trade.TradeType == TradeType.Buy) // green Line, when it's a Long-Order
{
Chart.DrawVerticalLine(
"Open_" + Trade.PositionId,
Trade.EntryTime,
Color.Green
);
}
if(showVerticalLines && Trade.TradeType == TradeType.Sell) // red Line, when it's a Short-Order
{
Chart.DrawVerticalLine(
"Open_" + Trade.PositionId,
Trade.EntryTime,
Color.Red
);
}
}
}
private void DrawDealMap()
{
foreach (var Trade in History)
{
if(showTradeLines && Trade.NetProfit>0) // When Trade was profitable -> green dealMapLine
{
var TrendLine = Chart.DrawTrendLine(
"TradelLine " + Trade.PositionId, // unique Name of the TrendLine
Trade.EntryTime, // X1 OpenTime
Trade.EntryPrice, // Y1 OpenPrice
Trade.ClosingTime, // X2 ClosingTime
Trade.ClosingPrice, // Y2 ClosingPrice
Color.LightGreen, // Color
3, // Line Thickness
LineStyle.Solid // Line Style
);
}
if(showTradeLines && Trade.NetProfit<0) // When Trade was profitable -> green dealMapLine
{
var TrendLine = Chart.DrawTrendLine(
"TradelLine " + Trade.PositionId, // unique Name of the TrendLine
Trade.EntryTime, // X1 OpenTime
Trade.EntryPrice, // Y1 OpenPrice
Trade.ClosingTime, // X2 ClosingTime
Trade.ClosingPrice, // Y2 ClosingPrice
Color.Magenta, // Color
3, // Line Thickness
LineStyle.Solid // Line Style
);
}
}
}
private void DrawDealStats()
{
foreach (var Trade in History)
{
string sign = Trade.NetProfit > 0 ? "+" : "";
string dealStats = $"{sign}{Trade.NetProfit / Account.Balance:0.00%}\n"+Trade.NetProfit.ToString("F2")+" €";
if(showDealStats && Trade.NetProfit > 0)
{
Chart.DrawText(
"DealNetProfit" + Trade.PositionId,
dealStats,
Trade.ClosingTime,
Trade.ClosingPrice,
Color.LightGreen
);
}
if(showDealStats && Trade.NetProfit < 0)
{
Chart.DrawText(
"DealNetProfit" + Trade.PositionId,
dealStats,
Trade.ClosingTime,
Trade.ClosingPrice,
Color.Magenta
);
}
}
}
#endregion own Methods
public override void Calculate(int index) { }
}
}
@levd20
levd20
01 Sep 2024, 15:13
( Updated at: 02 Sep 2024, 05:28 )
RE: RE: RE: RE: Mac version doesn't have the Deal Map viewing option
levd20 said:
This is what i built right now (CODE3 in ATTACHEMENT)
Please tell me:
- Wheather it works in your workarount
- if it is written and commented correctly (fits all formatting standards)
- if you have further ideas to make it shorter
- if you have further ideas to expand it with more functions
Feel free to use it for whatever you like.
Best regards
levd20
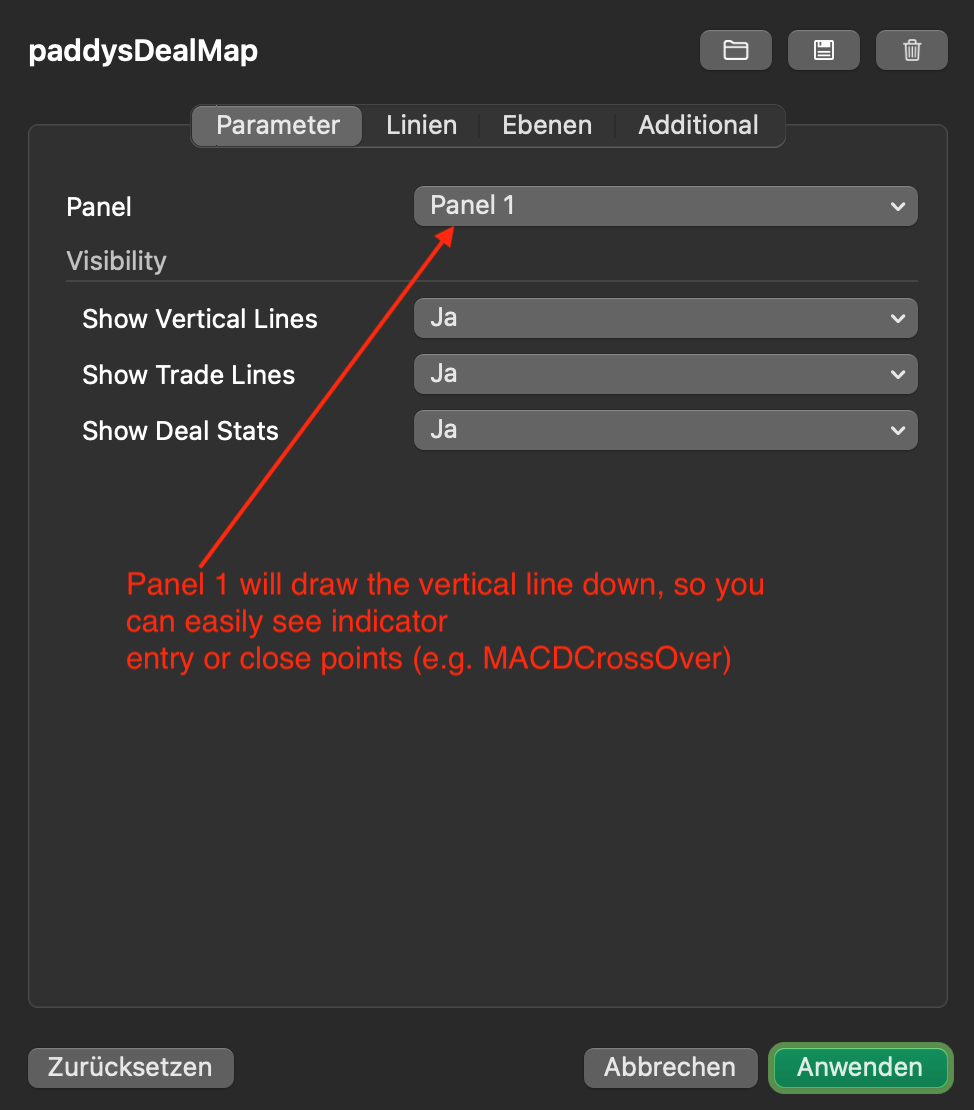
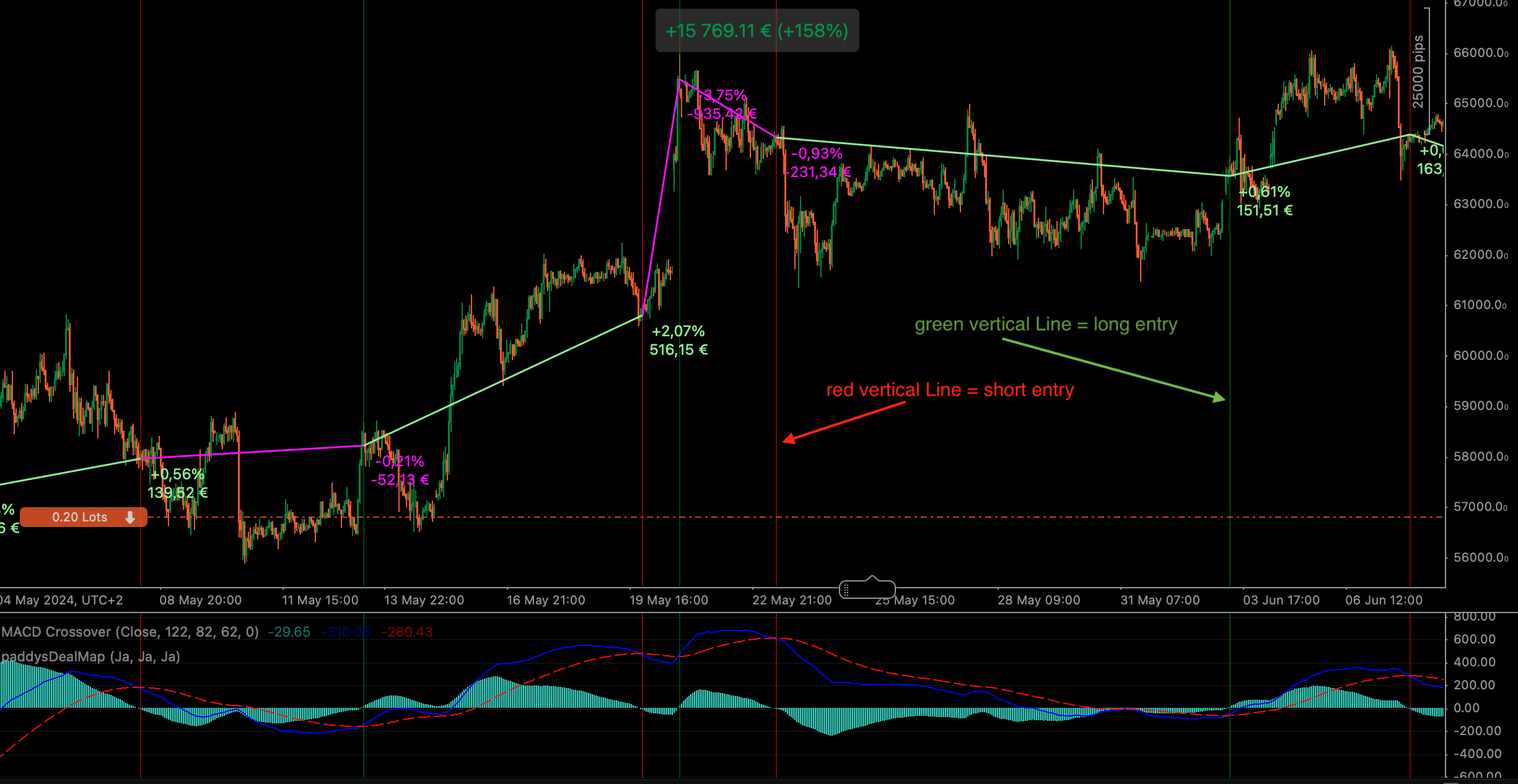
______________________________________________________________________________________________________________________________________________________________________________________________________________________________________________________________________________________________________________
ATTACHEMENT
CODE3:
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = true, AccessRights = AccessRights.None)]
public class paddysDealMap : Indicator
{
#region Parameter
[Parameter("Show Vertical Lines", Group = "Visibility", DefaultValue = true)]
public bool showVerticalLines { get; set; }
[Parameter("Show Trade Lines", Group = "Visibility", DefaultValue = true)]
public bool showTradeLines { get; set; }
[Parameter("Show Deal Stats", Group = "Visibility", DefaultValue = true)]
public bool showDealStats { get; set; }
#endregion
protected override void Initialize()
{
Chart.RemoveAllObjects();
DrawVerticalLines();
DrawDealMap();
DrawDealStats();
}
#region own Methods
private void DrawVerticalLines()
{
foreach (var Trade in History)
{
if(showVerticalLines && Trade.TradeType == TradeType.Buy) // green Line, when it's a Long-Order
{
Chart.DrawVerticalLine(
"Open_" + Trade.PositionId,
Trade.EntryTime,
Color.Green
);
}
if(showVerticalLines && Trade.TradeType == TradeType.Sell) // red Line, when it's a Short-Order
{
Chart.DrawVerticalLine(
"Open_" + Trade.PositionId,
Trade.EntryTime,
Color.Red
);
}
}
}
private void DrawDealMap()
{
foreach (var Trade in History)
{
if(showTradeLines && Trade.NetProfit>0) // When Trade was profitable -> green dealMapLine
{
var TrendLine = Chart.DrawTrendLine(
"TradelLine " + Trade.PositionId, // unique Name of the TrendLine
Trade.EntryTime, // X1 OpenTime
Trade.EntryPrice, // Y1 OpenPrice
Trade.ClosingTime, // X2 ClosingTime
Trade.ClosingPrice, // Y2 ClosingPrice
Color.LightGreen, // Color
3, // Line Thickness
LineStyle.Solid // Line Style
);
}
if(showTradeLines && Trade.NetProfit<0) // When Trade was profitable -> green dealMapLine
{
var TrendLine = Chart.DrawTrendLine(
"TradelLine " + Trade.PositionId, // unique Name of the TrendLine
Trade.EntryTime, // X1 OpenTime
Trade.EntryPrice, // Y1 OpenPrice
Trade.ClosingTime, // X2 ClosingTime
Trade.ClosingPrice, // Y2 ClosingPrice
Color.Magenta, // Color
3, // Line Thickness
LineStyle.Solid // Line Style
);
}
}
}
private void DrawDealStats()
{
foreach (var Trade in History)
{
string sign = Trade.NetProfit > 0 ? "+" : "";
string dealStats = $"{sign}{Trade.NetProfit / Account.Balance:0.00%}\n"+Trade.NetProfit.ToString("F2")+" €";
if(showDealStats && Trade.NetProfit > 0)
{
Chart.DrawText(
"DealNetProfit" + Trade.PositionId,
dealStats,
Trade.ClosingTime,
Trade.ClosingPrice,
Color.LightGreen
);
}
if(showDealStats && Trade.NetProfit < 0)
{
Chart.DrawText(
"DealNetProfit" + Trade.PositionId,
dealStats,
Trade.ClosingTime,
Trade.ClosingPrice,
Color.Magenta
);
}
}
}
#endregion own Methods
public override void Calculate(int index) { }
}
}
PanagiotisCharalampous said:
cBotTrader said:
PanagiotisCharalampous said:
Hi there,
It will be added in a future update.
Best regards,
Panagiotis
Hi!
i saw many improvemnts on mac version. It seemn now backtesting work. But the Deal Map still missing. this feature is very peaciuos when analysing things.
we still wating for this!
thanks!
Hi there,
Deal map has not been released yet for cTrader Mac.
Best regards,
Panagiotis
Anyone knows when dealMap for mac will be released?
@levd20
levd20
01 Sep 2024, 10:39
( Updated at: 02 Sep 2024, 05:28 )
RE: RE: RE: RE: Mac version doesn't have the Deal Map viewing option
levd20 said:
Hi, i am new to cTrader & c#. But thanks to PanagiotisCharalampous frequently helping and constructive answers in nearly every thread and some other research i was able to build a dealMap kinda myself as an indicator.
Little Story of how i got there:
At first i catched the Events via OnPositionClosed(PositionClosedEventArgs args), and added this into a Robot.
Unfortunately the backtests taking HUGE time (15 simulations over night with the weakest MacBookAir A1932)
So i removed the drawing-Lines from my sample-Code (standard MACDCrossOver) and ran the backtest “lean” and without any disturbing lines of code.
Result: 1300 simulations in 1h 40mins → Lesson learnt!
So i had to code the dealMap into an indicator, to run the Indicator seperately after the Optimization/Parameter Sweep. Whysoever the Events (OnPositionClosed,…) are not being triggered (see code down below, CODE2), but i was able to just draw all Lines from the history. (see below, CODE1)
This is only lean code to draw a verticalLine everytime a trade has been opened. Now it should be easy to change the code to the wanted dealMap-feature (line between open and close).
I have two further questions:
- Is sweeping the history really the most elegant way? (CODE1)
- e.g. i run a single backtest with CODE1. How is the backtesting calculation being prioritized? is the robot running through at first and than coming the indicator, or are Robot & Indicator being calculated parallely? Do i have to add the indicator to the backtest window before i run the backtest, or does it not matter when i add the indicator? → if it doesn't matter when i add it → Why? How is it being prioritized?
Greetings from Germany!
levd20
______________________________________________________________________________________________________________________________________
ATTACHEMENT
CODE 1:
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = true, AccessRights = AccessRights.None)]
public class SimpleTradeLinesFromHistory : Indicator
{
// you will FIRST have to run the Backtest and THEN add this indicator! -> only being executed while Initialize()! -> instantly after adding the Indicator to the chart. -> every new Backtest = delete and add the indicator to the chart again. I'm working on fixing this.
protected override void Initialize()
{
DrawTradeLines();
}
private void DrawTradeLines()
{
foreach (var position in History)
{
Chart.DrawVerticalLine("Open_" + position.PositionId, position.EntryTime, Color.Blue);
}
}
public override void Calculate(int index) { }
}
}
Are there some other possibilities to create dealMaps without using the history?
OnPositionsOpened did not trigger → no Print in the Log :(
CODE 2:
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = true, AccessRights = AccessRights.None)]
public class SimpleTradeLines : Indicator
{
protected override void Initialize()
{
Positions.Opened += OnPositionsOpened;
Positions.Closed += OnPositionsClosed;
}
private void OnPositionsOpened(PositionOpenedEventArgs args)
{
Chart.DrawVerticalLine("Open_" + args.Position.Id, args.Position.EntryTime, Color.Blue);
Print("POSITION OPENED!");
Print("----------------");
}
private void OnPositionsClosed(PositionClosedEventArgs args)
{
Chart.DrawVerticalLine("Close_" + args.Position.Id, args.Position.ExitTime, Color.Red);
Print("POSITION CLOSED!");
Print("----------------");
}
public override void Calculate(int index) { }
}
}
Another good inspiration was this one: https://ctrader.com/algos/indicators/show/3026/
But it didn't show all past trades on my Backtest, so we will have to put some work in it.
PanagiotisCharalampous said:
cBotTrader said:
PanagiotisCharalampous said:
Hi there,
It will be added in a future update.
Best regards,
Panagiotis
Hi!
i saw many improvemnts on mac version. It seemn now backtesting work. But the Deal Map still missing. this feature is very peaciuos when analysing things.
we still wating for this!
thanks!
Hi there,
Deal map has not been released yet for cTrader Mac.
Best regards,
Panagiotis
Anyone knows when dealMap for mac will be released?
@levd20
levd20
29 Aug 2024, 18:11
( Updated at: 29 Aug 2024, 18:15 )
RE: Mac: Scroll horizontal in timeline in backtest
PanagiotisCharalampous said:
Hi there,
You have not provided a screenshot therefore it is hard for us to understand what you are doing. If you can provide a video and also rewrite a post in a form of a question, explaining what you expect to happen, it would help us help you.
Best regards,
Panagiotis
Hello Panagiotis,
i fixed it with dialing in some settings in the "logi options+"-App. Now it is working perfectly fine.
Solution: activate horizontal scrolling in the Logi Options+-App
Thread closed :)
see you!
levd20
@levd20
levd20
27 Aug 2024, 15:16
Hi, i have the Logitech MX Master 3S. A mouse, that has a scroll wheel for scrolling vertically and horizontally.
cTrader asks me wheather i want to zoom, or scroll horizontally, when i scroll.
Is it possible to set it like this:
vertical mouse wheel turning = zoom
herizontal mouse weehl turning = horizontal scrolling (if possible without auto scale, lol)
best regards
levd20
@levd20
levd20
26 Aug 2024, 07:22
RE: RE: RE: how to write square and curly brackets in cTrader on Mac
PanagiotisCharalampous said:
levd20 said:
firemyst said:
What do i do wrong?
You're using a Mac ;-)
ok i'll buy windows then…
jokes aside - i can type via option+5 in the search bar for the indicators without any problems.
just INSIDE the Code-area it is not available. i also checked for some hotkey assignments in the settings. option+5 was assigned → i changed it, but no impact
Hi there,
What keyboard layout do you use?
Best regards,
Panagiotis
Good Morning Panagiotis,
iMac 27": mac OSVentura 13.6.9, Keyboard ("qwertz" / German), Keyboard Model A1843.
MacBook Air OS 14(I THINK), Keyboard ("qwertz"), MacBook Model A1932.
Thank you in Advance!
Best regards
levd20 :)
@levd20
levd20
25 Aug 2024, 11:01
( Updated at: 26 Aug 2024, 06:44 )
RE: RE: RE: Mac version doesn't have the Deal Map viewing option
PanagiotisCharalampous said:
cBotTrader said:
PanagiotisCharalampous said:
Hi there,
It will be added in a future update.
Best regards,
Panagiotis
Hi!
i saw many improvemnts on mac version. It seemn now backtesting work. But the Deal Map still missing. this feature is very peaciuos when analysing things.
we still wating for this!
thanks!
Hi there,
Deal map has not been released yet for cTrader Mac.
Best regards,
Panagiotis
Anyone knows when dealMap for mac will be released?
@levd20
levd20
25 Aug 2024, 11:00
( Updated at: 26 Aug 2024, 06:44 )
RE: RE: RE:
J.Lo said:
J.Lo said:
PanagiotisChar said:
Hi there,
Looks good to me
Need help? Join us on Telegram
Need premium support? Trade with us
What version are you running?
any specific settings you needed to toggle?
Ahh, i found the setting for those who run into the same issue.
The setting is called "Deal Map", it needs to be ticked
Not available on mac evtl.?
in my desktop version it doesnt work …
@levd20
levd20
22 Aug 2024, 08:03
( Updated at: 22 Aug 2024, 08:22 )
RE: how to write square and curly brackets in cTrader on Mac
firemyst said:
What do i do wrong?
You're using a Mac ;-)
ok i'll buy windows then…
jokes aside - i can type via option+5 in the search bar for the indicators without any problems.
just INSIDE the Code-area it is not available. i also checked for some hotkey assignments in the settings. option+5 was assigned → i changed it, but no impact
@levd20
levd20
07 Sep 2024, 01:31
RE: Cross line from below or above.
should be something like
//cross above
if(Bars.ClosePrices.Last(0) ≥ myIndicator.Last(0) && Bars.ClosePrices(1) < myIndicator.Last(1))
{
// do stuff
ExecuteMarketOrder…
}
rdebacker said:
@levd20