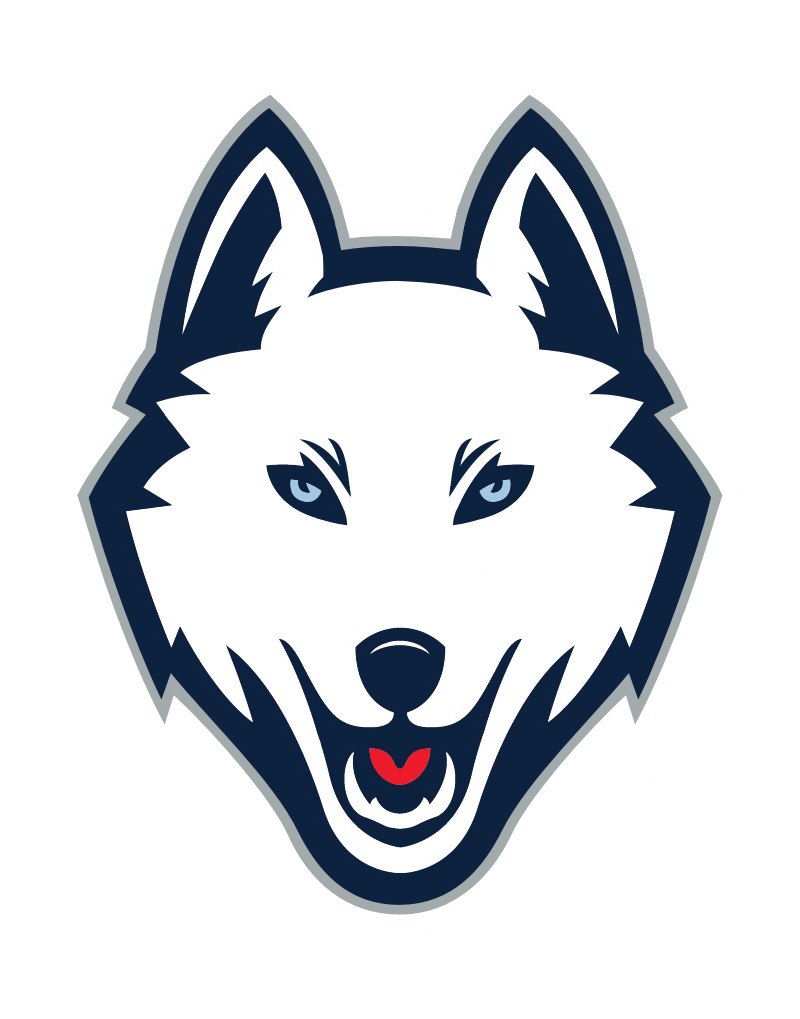
Topics
Replies
breakermind
27 Jun 2014, 23:36
RE: RE: RE:
Hi,
how it should work just in a few sentences (may be easier to write it from the beginning)?
@breakermind
breakermind
27 Jun 2014, 21:12
RE: RE: RE: RE:
If you felt offended, I did not want to offend anyone.
Bye.
@breakermind
breakermind
27 Jun 2014, 21:10
RE: RE: RE:
Forex19 said:
breakermind said:
Forex19 said:
Hi,
I need to compare the closing price of four or more candlesticks.What is the best way?
Hi,
This is one of those things whose probably can not do :D
I think you can compare only two things ;)
Bye.
Waiting to see if you get other suggestions.
How would you compare two things?
Something l;ike ...
This black car is prettier than orange one.
:D:D:D
But I meant "Strings, values etc ..."
Bye.
@breakermind
breakermind
27 Jun 2014, 13:26
RE:
Invalid said:
Why not to use VS instead of Print?
Not everyone needs to know what is VS, and second for what everything is ok in calgo debugger if you can read and think ;)
@breakermind
breakermind
27 Jun 2014, 09:03
( Updated at: 21 Dec 2023, 09:20 )
RE:
Hi,
search solutions do not expect the final solution - if you want to learn
Check line by line why conditions dont work and use Print("CCI1 " + _cci + "" + ...)
search robot which already works and compare :)
but working! conditions check it alone:
cBots is a waste of time ... :)
Regards
@breakermind
breakermind
26 Jun 2014, 23:50
RE:
Forex19 said:
Hi,
I need to compare the closing price of four or more candlesticks.What is the best way?
Hi,
This is one of those things whose probably can not do :D
I think you can compare only two things ;)
Bye.
@breakermind
breakermind
26 Jun 2014, 23:31
RE:
Hi,
try like this:
i dont test it :)
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)] public class z_cci : Robot { [Parameter("Volume", DefaultValue = 10000)] public int Volume { get; set; } [Parameter("Periods", DefaultValue = 20, MinValue = 1)] public int Periods { get; set; } [Parameter("Label", DefaultValue = "labelname")] public string label { get; set; } [Parameter("TP", DefaultValue = 50, MinValue = 10)] public int TP { get; set; } [Parameter("SL", DefaultValue = 0, MinValue = 0)] public int SL { get; set; } private CommodityChannelIndex _cci; private Position _position; protected override void OnStart() { _cci = Indicators.CommodityChannelIndex(Periods); } protected override void OnBar() { _position = Positions.Find(label); bool isLongPositionOpen = _position != null && _position.TradeType == TradeType.Buy; bool isShortPositionOpen = _position != null && _position.TradeType == TradeType.Sell; if (_cci.Result.HasCrossedBelow(0.0, 1) && _cci.Result.HasCrossedAbove(0.0, 2) && !isShortPositionOpen) { ExecuteMarketOrder(TradeType.Sell, Symbol, Volume, label, SL, TP); } if (_cci.Result.HasCrossedBelow(0.0, 2) && _cci.Result.HasCrossedAbove(0.0, 1) && !isLongPositionOpen) { ExecuteMarketOrder(TradeType.Buy, Symbol, Volume, label, SL, TP); } } protected override void OnStop() { // Put your deinitialization logic here } } }
Bye
@breakermind
breakermind
18 Jun 2014, 12:33
RE:
davidp13 said:
I need some guidance on how to close position immediately and not on the next open bar. I have tested my code in both OnTick and OnBar, but the effect stays the same. The time frame I use is 1 hour.
Code:
if (longPosition != null && _cciclose.Result.Last(1) < 0 && valueClose <= valueMa)
{
ClosePosition(longPosition);
Thanks
Hi,
set OnTick when tick change then close all positions
if the tick does not change it, nothing happens, set yourself on the chart period 1tick and see how it works(in the evening when there is little traffic), everything changes for the tick
tick is the smallest interval - probably
so I explain it myself.
Regards
@breakermind
breakermind
17 Jun 2014, 16:57
RE:
Is It possible to log in to your account cTrader from C# only using login and password for your account whitout any cTraderId or PartnerID whitout OpenApi?
Why is this whole OpenApi connection to account is so twisted?
Regards
@breakermind
breakermind
16 Jun 2014, 11:11
RE:
davidp13 said:
Correct. This code is to close the open position. The problem I have is when I add || (or) then no entries open anymore when I back test.
Hi,
think different ;)
if (currentSlowMa > currentFastMa && _cci.Result.Last(1) < _cci.Result.Last(0) && longPosition != null)
{
ClosePosition(longPosition);
}
if(_cci.Result.Last(1) < 0){
ClosePosition(longPosition);
}
if(xFastMA > valueClose)){
ClosePosition(longPosition);
]
Regards
@breakermind
breakermind
14 Jun 2014, 13:58
RE:
Hi,
look at this: (MA - movingAverage) something like this
protected override void OnTick() { foreach (var position in Positions) { if (position.TradeType == TradeType.Buy) { // TP == MA ModifyPosition(position, position.StopLoss, Convert.ToInt64(Ma)); } if (position.TradeType == TradeType.Sell) { // HighMinusLow == Ma ModifyPosition(position, Convert.ToInt64(Ma), position.TakeProfit); } } }
or:
if (Symbol.Bid > Ma) { foreach (var openedPosition in Positions) { ClosePosition(openedPosition); } Stop(); }
close all position is allways possible :D:D:D
Regards
@breakermind
breakermind
13 Jun 2014, 16:59
( Updated at: 21 Dec 2023, 09:20 )
RE:
Spotware said:
Backtesting will not stop in case if Equity goes bellow zero. Probably we will change this behavior in the future. You can post this idea to vote.spotware.com.
In cAlgo 1.23 we added Extremum points to the Equity line.
We plan to release cAlgo 1.23 next week, however it is already available on Spotware environment:
Hi,
after update "Add an Instance" to cBot dont works :)
why on this charts eguity do not refresh after some time period like tick, seconds, minute or something ... only when position close(or i have old ver).
Thanks
@breakermind
breakermind
12 Jun 2014, 17:29
( Updated at: 23 Jan 2024, 13:11 )
RE: RE: WeekStart-WeekEnd
breakermind said:
[/algos/cbots/show/503]
New with safe profits:
//================================================================================ // SimpleWeekRobo // Start at Monday 00:00 close on Friday 23:00 // Simple Monday-Friday script without any security with close when earn // If CloseWhenEarn == 0 then dont close positions //================================================================================ using System; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Requests; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)] public class SimpleWeekRobo : Robot { //================================================================================ // Parametrs //================================================================================ private double WeekStart; private double LastProfit = 0; private double StopMoneyLoss = 0; [Parameter(DefaultValue = 300000, MinValue = 1000)] public int Volume { get; set; } [Parameter(DefaultValue = 25, MinValue = 5)] public int Spacing { get; set; } [Parameter(DefaultValue = 10, MinValue = 2)] public int HowMuchPositions { get; set; } // close when earn [Parameter(DefaultValue = 0, MinValue = 0)] public int CloseWhenEarn { get; set; } // safe deposit works when earn 100% [Parameter(DefaultValue = false)] public bool MoneyStopLossOn { get; set; } [Parameter(DefaultValue = 1000, MinValue = 100)] public int StartDeposit { get; set; } [Parameter(DefaultValue = 50, MinValue = 10, MaxValue = 100)] public int StopLossPercent { get; set; } [Parameter(DefaultValue = 2, MinValue = 1, MaxValue = 100)] public int OnWhenDepositX { get; set; } //================================================================================ // OnStart //================================================================================ protected override void OnStart() { WeekStart = Symbol.Ask; for (int i = 1; i < HowMuchPositions; i++) { PlaceStopOrder(TradeType.Buy, Symbol, Volume, Symbol.Ask + Spacing * i * Symbol.PipSize); } for (int j = 1; j < HowMuchPositions; j++) { PlaceStopOrder(TradeType.Sell, Symbol, Volume, Symbol.Bid - Spacing * j * Symbol.PipSize); } } //================================================================================ // OnTick //================================================================================ protected override void OnTick() { var netProfit = 0.0; foreach (var openedPosition in Positions) { netProfit += openedPosition.NetProfit; } if (MoneyStopLossOn == true) { // safe deposit works when earn startdeposit x 1,2,3,4,5... if (LastProfit < Account.Equity && Account.Equity > (StartDeposit * OnWhenDepositX)) { LastProfit = Account.Equity; } if (Account.Equity > StartDeposit * 3) { //StopLossPercent = 90; } StopMoneyLoss = LastProfit * (StopLossPercent * 0.01); Print("LastProfit: ", LastProfit); Print("Equity: ", Account.Equity); Print("StopLossMoney: ", StopMoneyLoss); if (Account.Equity < StopMoneyLoss) { foreach (var openedPosition in Positions) { ClosePosition(openedPosition); } Stop(); } } if (netProfit >= CloseWhenEarn && CloseWhenEarn != 0) { foreach (var openedPosition in Positions) { ClosePosition(openedPosition); } Stop(); } } protected override void OnStop() { Print("cBot stop"); } //================================================================================ // Robot end //================================================================================ } }
Bye.
@breakermind
breakermind
12 Jun 2014, 16:10
( Updated at: 23 Jan 2024, 13:11 )
RE: WeekStart-WeekEnd
[/algos/cbots/show/503]
@breakermind
breakermind
12 Jun 2014, 15:13
WeekStart-WeekEnd
Update :D
// Start deposit: 1000$ Position volume: 500000 leverage 1:500 week: 14/04/2014 <-> 19/04/2014 Ending equity: 51055,30
// Start deposit: 1000$ Position volume: 300000 leverage 1:500 week: 14/04/2014 <-> 19/04/2014 Ending equity: 24,736.95
// Start deposit: 1000$ Position volume: 300000 leverage 1:500 week: 2/06/2014 <-> 7/06/2014 Ending equity: 16043.74
// Start deposit: 1000$ Position volume: 500000 leverage 1:500 week: 2/06/2014 <-> 7/06/2014 Ending equity: 26073.84
Have a nice day:
//================================================================================ // WeekStart-WeekEnd // Start at Monday 00:00 close on Friday 23:00 // Simple Monday-Friday script without any security with close when earn // If CloseWhenEarn == 0 then dont close positions //================================================================================ using System; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Requests; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)] public class SimpleWeekRobo : Robot { //================================================================================ // Parametrs //================================================================================ private Position _position; private double WeekStart; [Parameter(DefaultValue = false)] public bool CloseOnlyWithProfit { get; set; } [Parameter(DefaultValue = 300000, MinValue = 1000)] public int Volume { get; set; } [Parameter(DefaultValue = 25, MinValue = 5)] public int Spacing { get; set; } [Parameter(DefaultValue = 10, MinValue = 2)] public int HowMuchPositions { get; set; } [Parameter(DefaultValue = 0, MinValue = 0)] public int CloseWhenEarn { get; set; } //================================================================================ // OnStart //================================================================================ protected override void OnStart() { // week start price or run script price if (CloseOnlyWithProfit == true) { foreach (var openedPosition in Positions) { if (openedPosition.GrossProfit > 0) { ClosePosition(openedPosition); } } } WeekStart = Symbol.Ask; for (int i = 1; i < HowMuchPositions; i++) { PlaceStopOrder(TradeType.Buy, Symbol, Volume, Symbol.Ask + Spacing * i * Symbol.PipSize); } for (int j = 1; j < HowMuchPositions; j++) { PlaceStopOrder(TradeType.Sell, Symbol, Volume, Symbol.Bid - Spacing * j * Symbol.PipSize); } } //================================================================================ // OnTick //================================================================================ protected override void OnTick() { var netProfit = 0.0; foreach (var openedPosition in Positions) { netProfit += openedPosition.NetProfit; } if (netProfit >= CloseWhenEarn && CloseWhenEarn != 0) { foreach (var openedPosition in Positions) { ClosePosition(openedPosition); } Stop(); } } //================================================================================ // OnPositionOpened //================================================================================ protected override void OnPositionOpened(Position openedPosition) { int BuyPos = 0; int SellPos = 0; foreach (var position in Positions) { // Count opened positions if (position.TradeType == TradeType.Buy) { BuyPos = BuyPos + 1; } if (position.TradeType == TradeType.Sell) { SellPos = SellPos + 1; } } Print("All Buy positions: " + BuyPos); Print("All Sell positions: " + SellPos); } // end OnPositionOpened //================================================================================ // OnBar //================================================================================ protected override void OnBar() { } //================================================================================ // OnPositionClosed //================================================================================ protected override void OnPositionClosed(Position closedPosition) { } //================================================================================ // OnStop //================================================================================ protected override void OnStop() { } //================================================================================ // Function //================================================================================ private void Buy() { ExecuteMarketOrder(TradeType.Buy, Symbol, 10000, "", 1000, 1000); } private void Sell() { ExecuteMarketOrder(TradeType.Sell, Symbol, 10000, "", 1000, 1000); } private void ClosePosition() { if (_position != null) { ClosePosition(_position); _position = null; } } //================================================================================ // Robot end //================================================================================ } }
Bye
@breakermind
breakermind
12 Jun 2014, 09:50
RE: RE: RE: RE:
Hi Marin0ss it works ???
Why is this robot works once again dont,
what is wrong with baktestem in spotware cAlgo -- support why ???
Regards
Spotware said:
Marin0ss said:
Hi, Thank you very much! I left out te part in OnStart, as I don't want an order to be opened. (this will not cause any problems for the rest of the code?)
I would also like to Backtest this, this is not possible because of use of Server.Time; how must the code look to make this work?
I tried to replace Server.Time for MarketSeries.OpenTime.LastValue but this won't work.
Server.Time is emulated in backtesting, therefore you can backtest such cBot. Please do not forget to change TimeZones.UTC to the desired timezone.
@breakermind
breakermind
12 Jun 2014, 09:46
RE: RE: RE:
Spotware said:
breakermind said:
Hi and thanks,
I have this:
public override void Calculate(int index) { // access to different series var weeklySeries = MarketData.GetSeries(TimeFrame.Weekly); // open price bars double open = weeklySeries.Open[index]; Print(open); // If first bar is first bar of the day set open Week1Open[index] = open; }How get Week open price and draw line on this price ? what is wrong ?
index is wrong. The weeklySeries object has different indexes.
Cool,
something is wrong with this spotware cAlgo,
if do not hang it terribly slow ... shows some strange results ... in backtests
Support helpful as usual ...
... defeat.
Bye.
@breakermind
breakermind
12 Jun 2014, 09:28
RE:
Hi and thanks,
I have this:
public override void Calculate(int index) { // access to different series var weeklySeries = MarketData.GetSeries(TimeFrame.Weekly); // open price bars double open = weeklySeries.Open[index]; Print(open); // If first bar is first bar of the day set open Week1Open[index] = open; }
How get Week open price and draw line on this price ? what is wrong ?
@breakermind
breakermind
28 Jun 2014, 11:26
Modyfikacja sl and tp with label
Hi all,
Modyfikacja sl and tp with label:
It's somewhere in the examples or finished cbots.
Have a nice day.
@breakermind