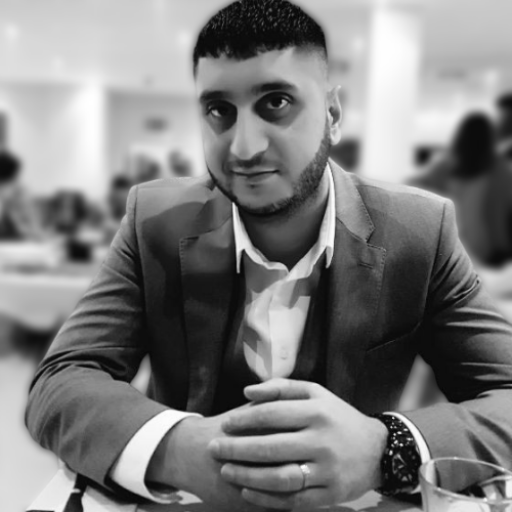
Topics
Replies
WassTima
23 Mar 2024, 03:16
RE: RE: Help with creating a cBot. Parabolic SAR
Such an amazing and helpful community
WassTima said:
firemyst said:
Why don't you post the code you have if you expect any help without having to pay someone?
using cAlgo.API;
using cAlgo.API.Indicators;
namespace cAlgo.Robots
{
// This cBot enters a position when the price reaches the Parabolic SAR value
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class ParabolicSAREntry : Robot
{
private double _volumeInUnits;
private ParabolicSAR _parabolicSAR;
[Parameter("Volume (Lots)", DefaultValue = 0.1)]
public double VolumeInLots { get; set; }
[Parameter("Stop Loss (Pips)", DefaultValue = 150)]
public double StopLossInPips { get; set; }
[Parameter("Take Profit (Pips)", DefaultValue = 20)]
public double TakeProfitInPips { get; set; }
[Parameter("Label", DefaultValue = "ParabolicSAREntry")]
public string Label { get; set; }
protected override void OnStart()
{
_volumeInUnits = Symbol.QuantityToVolumeInUnits(VolumeInLots);
_parabolicSAR = Indicators.ParabolicSAR(0.02, 0.2);
}
protected override void OnTick()
{
// Check if the current price has reached the Parabolic SAR value
if (Symbol.Ask < _parabolicSAR.Result.LastValue)
{
// If the price is below the Parabolic SAR, enter a Buy position
ExecuteMarketOrder(TradeType.Buy, SymbolName, _volumeInUnits, Label, StopLossInPips, TakeProfitInPips);
}
else if (Symbol.Bid > _parabolicSAR.Result.LastValue)
{
// If the price is above the Parabolic SAR, enter a Sell position
ExecuteMarketOrder(TradeType.Sell, SymbolName, _volumeInUnits, Label, StopLossInPips, TakeProfitInPips);
}
}
}
}
I would like the buy/sell position to start as soon as the oposite SAR is touched rather than wait and sometimes it opens a position at a random point?
Thank you in advance
@WassTima
WassTima
10 Mar 2024, 23:17
( Updated at: 10 Mar 2024, 23:18 )
RE: RE: Help with creating a cBot. Parabolic SAR
Please can someone help?
firemyst said:
Why don't you post the code you have if you expect any help without having to pay someone?
using cAlgo.API;
using cAlgo.API.Indicators;
namespace cAlgo.Robots
{
// This cBot enters a position when the price reaches the Parabolic SAR value
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class ParabolicSAREntry : Robot
{
private double _volumeInUnits;
private ParabolicSAR _parabolicSAR;
[Parameter("Volume (Lots)", DefaultValue = 0.1)]
public double VolumeInLots { get; set; }
[Parameter("Stop Loss (Pips)", DefaultValue = 150)]
public double StopLossInPips { get; set; }
[Parameter("Take Profit (Pips)", DefaultValue = 20)]
public double TakeProfitInPips { get; set; }
[Parameter("Label", DefaultValue = "ParabolicSAREntry")]
public string Label { get; set; }
protected override void OnStart()
{
_volumeInUnits = Symbol.QuantityToVolumeInUnits(VolumeInLots);
_parabolicSAR = Indicators.ParabolicSAR(0.02, 0.2);
}
protected override void OnTick()
{
// Check if the current price has reached the Parabolic SAR value
if (Symbol.Ask < _parabolicSAR.Result.LastValue)
{
// If the price is below the Parabolic SAR, enter a Buy position
ExecuteMarketOrder(TradeType.Buy, SymbolName, _volumeInUnits, Label, StopLossInPips, TakeProfitInPips);
}
else if (Symbol.Bid > _parabolicSAR.Result.LastValue)
{
// If the price is above the Parabolic SAR, enter a Sell position
ExecuteMarketOrder(TradeType.Sell, SymbolName, _volumeInUnits, Label, StopLossInPips, TakeProfitInPips);
}
}
}
}
I would like the buy/sell position to start as soon as the oposite SAR is touched rather than wait and sometimes it opens a position at a random point?
Thank you in advance
@WassTima
WassTima
10 Mar 2024, 15:03
( Updated at: 10 Mar 2024, 16:39 )
RE: Help with creating a cBot. Parabolic SAR
firemyst said:
Why don't you post the code you have if you expect any help without having to pay someone?
using cAlgo.API;
using cAlgo.API.Indicators;
namespace cAlgo.Robots
{
// This cBot enters a position when the price reaches the Parabolic SAR value
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class ParabolicSAREntry : Robot
{
private double _volumeInUnits;
private ParabolicSAR _parabolicSAR;
[Parameter("Volume (Lots)", DefaultValue = 0.1)]
public double VolumeInLots { get; set; }
[Parameter("Stop Loss (Pips)", DefaultValue = 150)]
public double StopLossInPips { get; set; }
[Parameter("Take Profit (Pips)", DefaultValue = 20)]
public double TakeProfitInPips { get; set; }
[Parameter("Label", DefaultValue = "ParabolicSAREntry")]
public string Label { get; set; }
protected override void OnStart()
{
_volumeInUnits = Symbol.QuantityToVolumeInUnits(VolumeInLots);
_parabolicSAR = Indicators.ParabolicSAR(0.02, 0.2);
}
protected override void OnTick()
{
// Check if the current price has reached the Parabolic SAR value
if (Symbol.Ask < _parabolicSAR.Result.LastValue)
{
// If the price is below the Parabolic SAR, enter a Buy position
ExecuteMarketOrder(TradeType.Buy, SymbolName, _volumeInUnits, Label, StopLossInPips, TakeProfitInPips);
}
else if (Symbol.Bid > _parabolicSAR.Result.LastValue)
{
// If the price is above the Parabolic SAR, enter a Sell position
ExecuteMarketOrder(TradeType.Sell, SymbolName, _volumeInUnits, Label, StopLossInPips, TakeProfitInPips);
}
}
}
}
I would like the buy/sell position to start as soon as the oposite SAR is touched rather than wait and sometimes it opens a position at a random point?
Thank you in advance
@WassTima
WassTima
23 Mar 2024, 03:16
RE: RE: Help with creating a cBot. Parabolic SAR
Such an amazing and helpful community
WassTima said:
@WassTima