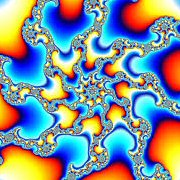
Status
Open
Budget
1.00 USD
Payment Method
Direct Payment
Job Description
I need help with a simple C# issue. I want to get the average spread stored by Pair in an associated list.
This works exactly the way I want it to, but only for one symbol:
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using System.Collections.Generic; namespace cAlgo.Robots { [Robot()] public class Robot : Robot { List SpreadList = new List(); protected override void OnStart() { } protected override void OnTick() { SpreadList.Add(Symbol.Spread); if (SpreadList.Count() >= 5) { SpreadList.RemoveAt(0); if (Symbol.Spread < SpreadList.Average()) Print("Current Spread: " + (Symbol.Spread / Symbol.PipSize) + " | Average Spread: " + (SpreadList.Average() / Symbol.PipSize) + " | Status: Better Than Average"); else Print("Current Spread: " + (Symbol.Spread / Symbol.PipSize) + " | Average Spread: " + (SpreadList.Average() / Symbol.PipSize) + " | Status: Worse Than Average"); } else Print("More Data Needed..."); } protected override void OnStop() { } } }
This does not work:
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Internals; using System.Collections.Generic; namespace cAlgo.Robots { [Robot()] public class RobotExample : Robot { string[] pPairs = new string[] { "EURUSD", "GBPUSD", "USDJPY", "AUDUSD", "EURJPY" }; List> SpreadList = new List>(); protected override void OnStart() { } protected override void OnTick() { foreach (var p in pPairs) { Symbol sSymbol = MarketData.GetSymbol(p); SpreadList.Insert(0, new KeyValuePair(p, sSymbol.Spread)); if ((SpreadList.Count() / pPairs.Count()) >= 5) { SpreadList.RemoveAt(0); // Print(sSymbol.Code + " | Current Spread: " + (sSymbol.Spread / sSymbol.PipSize) + " | Average Spread: " + SpreadList.FindAll(sSymbol.Code).Average() / sSymbol.PipSize ); } else Print("More Data Needed..."); } } protected override void OnStop() { } } }
The problem is with this:
SpreadList.FindAll(sSymbol.Code).Average()
let me implement it for you